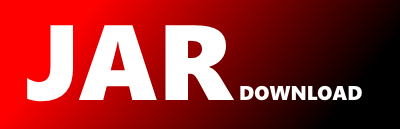
com.foursoft.vecmodel.vec120.AbstractVecConnectionAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecConnection} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecConnectionAssert, A extends VecConnection> extends AbstractVecRoutableElementAssert {
/**
* Creates a new {@link AbstractVecConnectionAssert}
to make assertions on actual VecConnection.
* @param actual the VecConnection we want to make assertions on.
*/
protected AbstractVecConnectionAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecConnection's connectionEnds contains the given VecConnectionEnd elements.
* @param connectionEnds the given elements that should be contained in actual VecConnection's connectionEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's connectionEnds does not contain all given VecConnectionEnd elements.
*/
public S hasConnectionEnds(VecConnectionEnd... connectionEnds) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd varargs is not null.
if (connectionEnds == null) failWithMessage("Expecting connectionEnds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnectionEnds(), connectionEnds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's connectionEnds contains the given VecConnectionEnd elements in Collection.
* @param connectionEnds the given elements that should be contained in actual VecConnection's connectionEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's connectionEnds does not contain all given VecConnectionEnd elements.
*/
public S hasConnectionEnds(java.util.Collection extends VecConnectionEnd> connectionEnds) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd collection is not null.
if (connectionEnds == null) {
failWithMessage("Expecting connectionEnds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnectionEnds(), connectionEnds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's connectionEnds contains only the given VecConnectionEnd elements and nothing else in whatever order.
* @param connectionEnds the given elements that should be contained in actual VecConnection's connectionEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's connectionEnds does not contain all given VecConnectionEnd elements.
*/
public S hasOnlyConnectionEnds(VecConnectionEnd... connectionEnds) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd varargs is not null.
if (connectionEnds == null) failWithMessage("Expecting connectionEnds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnectionEnds(), connectionEnds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's connectionEnds contains only the given VecConnectionEnd elements in Collection and nothing else in whatever order.
* @param connectionEnds the given elements that should be contained in actual VecConnection's connectionEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's connectionEnds does not contain all given VecConnectionEnd elements.
*/
public S hasOnlyConnectionEnds(java.util.Collection extends VecConnectionEnd> connectionEnds) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd collection is not null.
if (connectionEnds == null) {
failWithMessage("Expecting connectionEnds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnectionEnds(), connectionEnds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's connectionEnds does not contain the given VecConnectionEnd elements.
*
* @param connectionEnds the given elements that should not be in actual VecConnection's connectionEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's connectionEnds contains any given VecConnectionEnd elements.
*/
public S doesNotHaveConnectionEnds(VecConnectionEnd... connectionEnds) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd varargs is not null.
if (connectionEnds == null) failWithMessage("Expecting connectionEnds parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnectionEnds(), connectionEnds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's connectionEnds does not contain the given VecConnectionEnd elements in Collection.
*
* @param connectionEnds the given elements that should not be in actual VecConnection's connectionEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's connectionEnds contains any given VecConnectionEnd elements.
*/
public S doesNotHaveConnectionEnds(java.util.Collection extends VecConnectionEnd> connectionEnds) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd collection is not null.
if (connectionEnds == null) {
failWithMessage("Expecting connectionEnds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnectionEnds(), connectionEnds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no connectionEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's connectionEnds is not empty.
*/
public S hasNoConnectionEnds() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have connectionEnds but had :\n <%s>";
// check
if (actual.getConnectionEnds().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getConnectionEnds());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's descriptions contains the given VecAbstractLocalizedString elements.
* @param descriptions the given elements that should be contained in actual VecConnection's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's descriptions contains the given VecAbstractLocalizedString elements in Collection.
* @param descriptions the given elements that should be contained in actual VecConnection's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's descriptions contains only the given VecAbstractLocalizedString elements and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecConnection's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's descriptions contains only the given VecAbstractLocalizedString elements in Collection and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecConnection's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's descriptions does not contain the given VecAbstractLocalizedString elements.
*
* @param descriptions the given elements that should not be in actual VecConnection's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's descriptions does not contain the given VecAbstractLocalizedString elements in Collection.
*
* @param descriptions the given elements that should not be in actual VecConnection's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's descriptions is not empty.
*/
public S hasNoDescriptions() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have descriptions but had :\n <%s>";
// check
if (actual.getDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's identification is equal to the given one.
* @param identification the given identification to compare the actual VecConnection's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConnection's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's installationInstructions contains the given VecInstruction elements.
* @param installationInstructions the given elements that should be contained in actual VecConnection's installationInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's installationInstructions does not contain all given VecInstruction elements.
*/
public S hasInstallationInstructions(VecInstruction... installationInstructions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction varargs is not null.
if (installationInstructions == null) failWithMessage("Expecting installationInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInstallationInstructions(), installationInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's installationInstructions contains the given VecInstruction elements in Collection.
* @param installationInstructions the given elements that should be contained in actual VecConnection's installationInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's installationInstructions does not contain all given VecInstruction elements.
*/
public S hasInstallationInstructions(java.util.Collection extends VecInstruction> installationInstructions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction collection is not null.
if (installationInstructions == null) {
failWithMessage("Expecting installationInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInstallationInstructions(), installationInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's installationInstructions contains only the given VecInstruction elements and nothing else in whatever order.
* @param installationInstructions the given elements that should be contained in actual VecConnection's installationInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's installationInstructions does not contain all given VecInstruction elements.
*/
public S hasOnlyInstallationInstructions(VecInstruction... installationInstructions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction varargs is not null.
if (installationInstructions == null) failWithMessage("Expecting installationInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInstallationInstructions(), installationInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's installationInstructions contains only the given VecInstruction elements in Collection and nothing else in whatever order.
* @param installationInstructions the given elements that should be contained in actual VecConnection's installationInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's installationInstructions does not contain all given VecInstruction elements.
*/
public S hasOnlyInstallationInstructions(java.util.Collection extends VecInstruction> installationInstructions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction collection is not null.
if (installationInstructions == null) {
failWithMessage("Expecting installationInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInstallationInstructions(), installationInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's installationInstructions does not contain the given VecInstruction elements.
*
* @param installationInstructions the given elements that should not be in actual VecConnection's installationInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's installationInstructions contains any given VecInstruction elements.
*/
public S doesNotHaveInstallationInstructions(VecInstruction... installationInstructions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction varargs is not null.
if (installationInstructions == null) failWithMessage("Expecting installationInstructions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInstallationInstructions(), installationInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's installationInstructions does not contain the given VecInstruction elements in Collection.
*
* @param installationInstructions the given elements that should not be in actual VecConnection's installationInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's installationInstructions contains any given VecInstruction elements.
*/
public S doesNotHaveInstallationInstructions(java.util.Collection extends VecInstruction> installationInstructions) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction collection is not null.
if (installationInstructions == null) {
failWithMessage("Expecting installationInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInstallationInstructions(), installationInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no installationInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's installationInstructions is not empty.
*/
public S hasNoInstallationInstructions() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have installationInstructions but had :\n <%s>";
// check
if (actual.getInstallationInstructions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getInstallationInstructions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's net is equal to the given one.
* @param net the given net to compare the actual VecConnection's net to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConnection's net is not equal to the given one.
*/
public S hasNet(VecNet net) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting net of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNet actualNet = actual.getNet();
if (!Objects.areEqual(actualNet, net)) {
failWithMessage(assertjErrorMessage, actual, net, actualNet);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's parentConnectionSpecification is equal to the given one.
* @param parentConnectionSpecification the given parentConnectionSpecification to compare the actual VecConnection's parentConnectionSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConnection's parentConnectionSpecification is not equal to the given one.
*/
public S hasParentConnectionSpecification(VecConnectionSpecification parentConnectionSpecification) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentConnectionSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecConnectionSpecification actualParentConnectionSpecification = actual.getParentConnectionSpecification();
if (!Objects.areEqual(actualParentConnectionSpecification, parentConnectionSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentConnectionSpecification, actualParentConnectionSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refBridgeTerminalRole contains the given VecBridgeTerminalRole elements.
* @param refBridgeTerminalRole the given elements that should be contained in actual VecConnection's refBridgeTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refBridgeTerminalRole does not contain all given VecBridgeTerminalRole elements.
*/
public S hasRefBridgeTerminalRole(VecBridgeTerminalRole... refBridgeTerminalRole) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecBridgeTerminalRole varargs is not null.
if (refBridgeTerminalRole == null) failWithMessage("Expecting refBridgeTerminalRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBridgeTerminalRole(), refBridgeTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refBridgeTerminalRole contains the given VecBridgeTerminalRole elements in Collection.
* @param refBridgeTerminalRole the given elements that should be contained in actual VecConnection's refBridgeTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refBridgeTerminalRole does not contain all given VecBridgeTerminalRole elements.
*/
public S hasRefBridgeTerminalRole(java.util.Collection extends VecBridgeTerminalRole> refBridgeTerminalRole) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecBridgeTerminalRole collection is not null.
if (refBridgeTerminalRole == null) {
failWithMessage("Expecting refBridgeTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBridgeTerminalRole(), refBridgeTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refBridgeTerminalRole contains only the given VecBridgeTerminalRole elements and nothing else in whatever order.
* @param refBridgeTerminalRole the given elements that should be contained in actual VecConnection's refBridgeTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refBridgeTerminalRole does not contain all given VecBridgeTerminalRole elements.
*/
public S hasOnlyRefBridgeTerminalRole(VecBridgeTerminalRole... refBridgeTerminalRole) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecBridgeTerminalRole varargs is not null.
if (refBridgeTerminalRole == null) failWithMessage("Expecting refBridgeTerminalRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBridgeTerminalRole(), refBridgeTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refBridgeTerminalRole contains only the given VecBridgeTerminalRole elements in Collection and nothing else in whatever order.
* @param refBridgeTerminalRole the given elements that should be contained in actual VecConnection's refBridgeTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refBridgeTerminalRole does not contain all given VecBridgeTerminalRole elements.
*/
public S hasOnlyRefBridgeTerminalRole(java.util.Collection extends VecBridgeTerminalRole> refBridgeTerminalRole) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecBridgeTerminalRole collection is not null.
if (refBridgeTerminalRole == null) {
failWithMessage("Expecting refBridgeTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBridgeTerminalRole(), refBridgeTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refBridgeTerminalRole does not contain the given VecBridgeTerminalRole elements.
*
* @param refBridgeTerminalRole the given elements that should not be in actual VecConnection's refBridgeTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refBridgeTerminalRole contains any given VecBridgeTerminalRole elements.
*/
public S doesNotHaveRefBridgeTerminalRole(VecBridgeTerminalRole... refBridgeTerminalRole) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecBridgeTerminalRole varargs is not null.
if (refBridgeTerminalRole == null) failWithMessage("Expecting refBridgeTerminalRole parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBridgeTerminalRole(), refBridgeTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refBridgeTerminalRole does not contain the given VecBridgeTerminalRole elements in Collection.
*
* @param refBridgeTerminalRole the given elements that should not be in actual VecConnection's refBridgeTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refBridgeTerminalRole contains any given VecBridgeTerminalRole elements.
*/
public S doesNotHaveRefBridgeTerminalRole(java.util.Collection extends VecBridgeTerminalRole> refBridgeTerminalRole) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecBridgeTerminalRole collection is not null.
if (refBridgeTerminalRole == null) {
failWithMessage("Expecting refBridgeTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBridgeTerminalRole(), refBridgeTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no refBridgeTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refBridgeTerminalRole is not empty.
*/
public S hasNoRefBridgeTerminalRole() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refBridgeTerminalRole but had :\n <%s>";
// check
if (actual.getRefBridgeTerminalRole().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefBridgeTerminalRole());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refConnectionGroup contains the given VecConnectionGroup elements.
* @param refConnectionGroup the given elements that should be contained in actual VecConnection's refConnectionGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refConnectionGroup does not contain all given VecConnectionGroup elements.
*/
public S hasRefConnectionGroup(VecConnectionGroup... refConnectionGroup) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup varargs is not null.
if (refConnectionGroup == null) failWithMessage("Expecting refConnectionGroup parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConnectionGroup(), refConnectionGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refConnectionGroup contains the given VecConnectionGroup elements in Collection.
* @param refConnectionGroup the given elements that should be contained in actual VecConnection's refConnectionGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refConnectionGroup does not contain all given VecConnectionGroup elements.
*/
public S hasRefConnectionGroup(java.util.Collection extends VecConnectionGroup> refConnectionGroup) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup collection is not null.
if (refConnectionGroup == null) {
failWithMessage("Expecting refConnectionGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConnectionGroup(), refConnectionGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refConnectionGroup contains only the given VecConnectionGroup elements and nothing else in whatever order.
* @param refConnectionGroup the given elements that should be contained in actual VecConnection's refConnectionGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refConnectionGroup does not contain all given VecConnectionGroup elements.
*/
public S hasOnlyRefConnectionGroup(VecConnectionGroup... refConnectionGroup) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup varargs is not null.
if (refConnectionGroup == null) failWithMessage("Expecting refConnectionGroup parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConnectionGroup(), refConnectionGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refConnectionGroup contains only the given VecConnectionGroup elements in Collection and nothing else in whatever order.
* @param refConnectionGroup the given elements that should be contained in actual VecConnection's refConnectionGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refConnectionGroup does not contain all given VecConnectionGroup elements.
*/
public S hasOnlyRefConnectionGroup(java.util.Collection extends VecConnectionGroup> refConnectionGroup) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup collection is not null.
if (refConnectionGroup == null) {
failWithMessage("Expecting refConnectionGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConnectionGroup(), refConnectionGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refConnectionGroup does not contain the given VecConnectionGroup elements.
*
* @param refConnectionGroup the given elements that should not be in actual VecConnection's refConnectionGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refConnectionGroup contains any given VecConnectionGroup elements.
*/
public S doesNotHaveRefConnectionGroup(VecConnectionGroup... refConnectionGroup) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup varargs is not null.
if (refConnectionGroup == null) failWithMessage("Expecting refConnectionGroup parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConnectionGroup(), refConnectionGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refConnectionGroup does not contain the given VecConnectionGroup elements in Collection.
*
* @param refConnectionGroup the given elements that should not be in actual VecConnection's refConnectionGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refConnectionGroup contains any given VecConnectionGroup elements.
*/
public S doesNotHaveRefConnectionGroup(java.util.Collection extends VecConnectionGroup> refConnectionGroup) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup collection is not null.
if (refConnectionGroup == null) {
failWithMessage("Expecting refConnectionGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConnectionGroup(), refConnectionGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no refConnectionGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refConnectionGroup is not empty.
*/
public S hasNoRefConnectionGroup() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refConnectionGroup but had :\n <%s>";
// check
if (actual.getRefConnectionGroup().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefConnectionGroup());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingDetail contains the given VecMatingDetail elements.
* @param refMatingDetail the given elements that should be contained in actual VecConnection's refMatingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingDetail does not contain all given VecMatingDetail elements.
*/
public S hasRefMatingDetail(VecMatingDetail... refMatingDetail) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingDetail varargs is not null.
if (refMatingDetail == null) failWithMessage("Expecting refMatingDetail parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMatingDetail(), refMatingDetail);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingDetail contains the given VecMatingDetail elements in Collection.
* @param refMatingDetail the given elements that should be contained in actual VecConnection's refMatingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingDetail does not contain all given VecMatingDetail elements.
*/
public S hasRefMatingDetail(java.util.Collection extends VecMatingDetail> refMatingDetail) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingDetail collection is not null.
if (refMatingDetail == null) {
failWithMessage("Expecting refMatingDetail parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMatingDetail(), refMatingDetail.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingDetail contains only the given VecMatingDetail elements and nothing else in whatever order.
* @param refMatingDetail the given elements that should be contained in actual VecConnection's refMatingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingDetail does not contain all given VecMatingDetail elements.
*/
public S hasOnlyRefMatingDetail(VecMatingDetail... refMatingDetail) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingDetail varargs is not null.
if (refMatingDetail == null) failWithMessage("Expecting refMatingDetail parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMatingDetail(), refMatingDetail);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingDetail contains only the given VecMatingDetail elements in Collection and nothing else in whatever order.
* @param refMatingDetail the given elements that should be contained in actual VecConnection's refMatingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingDetail does not contain all given VecMatingDetail elements.
*/
public S hasOnlyRefMatingDetail(java.util.Collection extends VecMatingDetail> refMatingDetail) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingDetail collection is not null.
if (refMatingDetail == null) {
failWithMessage("Expecting refMatingDetail parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMatingDetail(), refMatingDetail.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingDetail does not contain the given VecMatingDetail elements.
*
* @param refMatingDetail the given elements that should not be in actual VecConnection's refMatingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingDetail contains any given VecMatingDetail elements.
*/
public S doesNotHaveRefMatingDetail(VecMatingDetail... refMatingDetail) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingDetail varargs is not null.
if (refMatingDetail == null) failWithMessage("Expecting refMatingDetail parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMatingDetail(), refMatingDetail);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingDetail does not contain the given VecMatingDetail elements in Collection.
*
* @param refMatingDetail the given elements that should not be in actual VecConnection's refMatingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingDetail contains any given VecMatingDetail elements.
*/
public S doesNotHaveRefMatingDetail(java.util.Collection extends VecMatingDetail> refMatingDetail) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingDetail collection is not null.
if (refMatingDetail == null) {
failWithMessage("Expecting refMatingDetail parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMatingDetail(), refMatingDetail.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no refMatingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingDetail is not empty.
*/
public S hasNoRefMatingDetail() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refMatingDetail but had :\n <%s>";
// check
if (actual.getRefMatingDetail().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefMatingDetail());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingPoint contains the given VecMatingPoint elements.
* @param refMatingPoint the given elements that should be contained in actual VecConnection's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasRefMatingPoint(VecMatingPoint... refMatingPoint) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint varargs is not null.
if (refMatingPoint == null) failWithMessage("Expecting refMatingPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMatingPoint(), refMatingPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingPoint contains the given VecMatingPoint elements in Collection.
* @param refMatingPoint the given elements that should be contained in actual VecConnection's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasRefMatingPoint(java.util.Collection extends VecMatingPoint> refMatingPoint) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint collection is not null.
if (refMatingPoint == null) {
failWithMessage("Expecting refMatingPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMatingPoint(), refMatingPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingPoint contains only the given VecMatingPoint elements and nothing else in whatever order.
* @param refMatingPoint the given elements that should be contained in actual VecConnection's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasOnlyRefMatingPoint(VecMatingPoint... refMatingPoint) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint varargs is not null.
if (refMatingPoint == null) failWithMessage("Expecting refMatingPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMatingPoint(), refMatingPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingPoint contains only the given VecMatingPoint elements in Collection and nothing else in whatever order.
* @param refMatingPoint the given elements that should be contained in actual VecConnection's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasOnlyRefMatingPoint(java.util.Collection extends VecMatingPoint> refMatingPoint) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint collection is not null.
if (refMatingPoint == null) {
failWithMessage("Expecting refMatingPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMatingPoint(), refMatingPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingPoint does not contain the given VecMatingPoint elements.
*
* @param refMatingPoint the given elements that should not be in actual VecConnection's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingPoint contains any given VecMatingPoint elements.
*/
public S doesNotHaveRefMatingPoint(VecMatingPoint... refMatingPoint) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint varargs is not null.
if (refMatingPoint == null) failWithMessage("Expecting refMatingPoint parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMatingPoint(), refMatingPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refMatingPoint does not contain the given VecMatingPoint elements in Collection.
*
* @param refMatingPoint the given elements that should not be in actual VecConnection's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingPoint contains any given VecMatingPoint elements.
*/
public S doesNotHaveRefMatingPoint(java.util.Collection extends VecMatingPoint> refMatingPoint) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint collection is not null.
if (refMatingPoint == null) {
failWithMessage("Expecting refMatingPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMatingPoint(), refMatingPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refMatingPoint is not empty.
*/
public S hasNoRefMatingPoint() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refMatingPoint but had :\n <%s>";
// check
if (actual.getRefMatingPoint().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefMatingPoint());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refWireElementReference contains the given VecWireElementReference elements.
* @param refWireElementReference the given elements that should be contained in actual VecConnection's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasRefWireElementReference(VecWireElementReference... refWireElementReference) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference varargs is not null.
if (refWireElementReference == null) failWithMessage("Expecting refWireElementReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementReference(), refWireElementReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refWireElementReference contains the given VecWireElementReference elements in Collection.
* @param refWireElementReference the given elements that should be contained in actual VecConnection's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasRefWireElementReference(java.util.Collection extends VecWireElementReference> refWireElementReference) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference collection is not null.
if (refWireElementReference == null) {
failWithMessage("Expecting refWireElementReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementReference(), refWireElementReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refWireElementReference contains only the given VecWireElementReference elements and nothing else in whatever order.
* @param refWireElementReference the given elements that should be contained in actual VecConnection's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasOnlyRefWireElementReference(VecWireElementReference... refWireElementReference) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference varargs is not null.
if (refWireElementReference == null) failWithMessage("Expecting refWireElementReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementReference(), refWireElementReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refWireElementReference contains only the given VecWireElementReference elements in Collection and nothing else in whatever order.
* @param refWireElementReference the given elements that should be contained in actual VecConnection's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasOnlyRefWireElementReference(java.util.Collection extends VecWireElementReference> refWireElementReference) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference collection is not null.
if (refWireElementReference == null) {
failWithMessage("Expecting refWireElementReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementReference(), refWireElementReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refWireElementReference does not contain the given VecWireElementReference elements.
*
* @param refWireElementReference the given elements that should not be in actual VecConnection's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refWireElementReference contains any given VecWireElementReference elements.
*/
public S doesNotHaveRefWireElementReference(VecWireElementReference... refWireElementReference) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference varargs is not null.
if (refWireElementReference == null) failWithMessage("Expecting refWireElementReference parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementReference(), refWireElementReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's refWireElementReference does not contain the given VecWireElementReference elements in Collection.
*
* @param refWireElementReference the given elements that should not be in actual VecConnection's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refWireElementReference contains any given VecWireElementReference elements.
*/
public S doesNotHaveRefWireElementReference(java.util.Collection extends VecWireElementReference> refWireElementReference) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference collection is not null.
if (refWireElementReference == null) {
failWithMessage("Expecting refWireElementReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementReference(), refWireElementReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection has no refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecConnection's refWireElementReference is not empty.
*/
public S hasNoRefWireElementReference() {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireElementReference but had :\n <%s>";
// check
if (actual.getRefWireElementReference().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireElementReference());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnection's signal is equal to the given one.
* @param signal the given signal to compare the actual VecConnection's signal to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConnection's signal is not equal to the given one.
*/
public S hasSignal(VecSignal signal) {
// check that actual VecConnection we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signal of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSignal actualSignal = actual.getSignal();
if (!Objects.areEqual(actualSignal, signal)) {
failWithMessage(assertjErrorMessage, actual, signal, actualSignal);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy