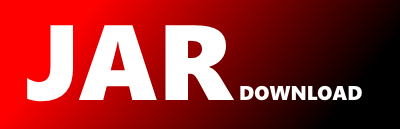
com.foursoft.vecmodel.vec120.AbstractVecConnectionSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
/**
* Abstract base class for {@link VecConnectionSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecConnectionSpecificationAssert, A extends VecConnectionSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecConnectionSpecificationAssert}
to make assertions on actual VecConnectionSpecification.
* @param actual the VecConnectionSpecification we want to make assertions on.
*/
protected AbstractVecConnectionSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecConnectionSpecification's componentNodes contains the given VecComponentNode elements.
* @param componentNodes the given elements that should be contained in actual VecConnectionSpecification's componentNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's componentNodes does not contain all given VecComponentNode elements.
*/
public S hasComponentNodes(VecComponentNode... componentNodes) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode varargs is not null.
if (componentNodes == null) failWithMessage("Expecting componentNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getComponentNodes(), componentNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's componentNodes contains the given VecComponentNode elements in Collection.
* @param componentNodes the given elements that should be contained in actual VecConnectionSpecification's componentNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's componentNodes does not contain all given VecComponentNode elements.
*/
public S hasComponentNodes(java.util.Collection extends VecComponentNode> componentNodes) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode collection is not null.
if (componentNodes == null) {
failWithMessage("Expecting componentNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getComponentNodes(), componentNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's componentNodes contains only the given VecComponentNode elements and nothing else in whatever order.
* @param componentNodes the given elements that should be contained in actual VecConnectionSpecification's componentNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's componentNodes does not contain all given VecComponentNode elements.
*/
public S hasOnlyComponentNodes(VecComponentNode... componentNodes) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode varargs is not null.
if (componentNodes == null) failWithMessage("Expecting componentNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getComponentNodes(), componentNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's componentNodes contains only the given VecComponentNode elements in Collection and nothing else in whatever order.
* @param componentNodes the given elements that should be contained in actual VecConnectionSpecification's componentNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's componentNodes does not contain all given VecComponentNode elements.
*/
public S hasOnlyComponentNodes(java.util.Collection extends VecComponentNode> componentNodes) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode collection is not null.
if (componentNodes == null) {
failWithMessage("Expecting componentNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getComponentNodes(), componentNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's componentNodes does not contain the given VecComponentNode elements.
*
* @param componentNodes the given elements that should not be in actual VecConnectionSpecification's componentNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's componentNodes contains any given VecComponentNode elements.
*/
public S doesNotHaveComponentNodes(VecComponentNode... componentNodes) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode varargs is not null.
if (componentNodes == null) failWithMessage("Expecting componentNodes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getComponentNodes(), componentNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's componentNodes does not contain the given VecComponentNode elements in Collection.
*
* @param componentNodes the given elements that should not be in actual VecConnectionSpecification's componentNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's componentNodes contains any given VecComponentNode elements.
*/
public S doesNotHaveComponentNodes(java.util.Collection extends VecComponentNode> componentNodes) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode collection is not null.
if (componentNodes == null) {
failWithMessage("Expecting componentNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getComponentNodes(), componentNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification has no componentNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's componentNodes is not empty.
*/
public S hasNoComponentNodes() {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have componentNodes but had :\n <%s>";
// check
if (actual.getComponentNodes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getComponentNodes());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connectionGroups contains the given VecConnectionGroup elements.
* @param connectionGroups the given elements that should be contained in actual VecConnectionSpecification's connectionGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connectionGroups does not contain all given VecConnectionGroup elements.
*/
public S hasConnectionGroups(VecConnectionGroup... connectionGroups) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup varargs is not null.
if (connectionGroups == null) failWithMessage("Expecting connectionGroups parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnectionGroups(), connectionGroups);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connectionGroups contains the given VecConnectionGroup elements in Collection.
* @param connectionGroups the given elements that should be contained in actual VecConnectionSpecification's connectionGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connectionGroups does not contain all given VecConnectionGroup elements.
*/
public S hasConnectionGroups(java.util.Collection extends VecConnectionGroup> connectionGroups) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup collection is not null.
if (connectionGroups == null) {
failWithMessage("Expecting connectionGroups parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnectionGroups(), connectionGroups.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connectionGroups contains only the given VecConnectionGroup elements and nothing else in whatever order.
* @param connectionGroups the given elements that should be contained in actual VecConnectionSpecification's connectionGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connectionGroups does not contain all given VecConnectionGroup elements.
*/
public S hasOnlyConnectionGroups(VecConnectionGroup... connectionGroups) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup varargs is not null.
if (connectionGroups == null) failWithMessage("Expecting connectionGroups parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnectionGroups(), connectionGroups);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connectionGroups contains only the given VecConnectionGroup elements in Collection and nothing else in whatever order.
* @param connectionGroups the given elements that should be contained in actual VecConnectionSpecification's connectionGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connectionGroups does not contain all given VecConnectionGroup elements.
*/
public S hasOnlyConnectionGroups(java.util.Collection extends VecConnectionGroup> connectionGroups) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup collection is not null.
if (connectionGroups == null) {
failWithMessage("Expecting connectionGroups parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnectionGroups(), connectionGroups.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connectionGroups does not contain the given VecConnectionGroup elements.
*
* @param connectionGroups the given elements that should not be in actual VecConnectionSpecification's connectionGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connectionGroups contains any given VecConnectionGroup elements.
*/
public S doesNotHaveConnectionGroups(VecConnectionGroup... connectionGroups) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup varargs is not null.
if (connectionGroups == null) failWithMessage("Expecting connectionGroups parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnectionGroups(), connectionGroups);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connectionGroups does not contain the given VecConnectionGroup elements in Collection.
*
* @param connectionGroups the given elements that should not be in actual VecConnectionSpecification's connectionGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connectionGroups contains any given VecConnectionGroup elements.
*/
public S doesNotHaveConnectionGroups(java.util.Collection extends VecConnectionGroup> connectionGroups) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionGroup collection is not null.
if (connectionGroups == null) {
failWithMessage("Expecting connectionGroups parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnectionGroups(), connectionGroups.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification has no connectionGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connectionGroups is not empty.
*/
public S hasNoConnectionGroups() {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have connectionGroups but had :\n <%s>";
// check
if (actual.getConnectionGroups().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getConnectionGroups());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connections contains the given VecConnection elements.
* @param connections the given elements that should be contained in actual VecConnectionSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connections does not contain all given VecConnection elements.
*/
public S hasConnections(VecConnection... connections) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnection varargs is not null.
if (connections == null) failWithMessage("Expecting connections parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnections(), connections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connections contains the given VecConnection elements in Collection.
* @param connections the given elements that should be contained in actual VecConnectionSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connections does not contain all given VecConnection elements.
*/
public S hasConnections(java.util.Collection extends VecConnection> connections) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnection collection is not null.
if (connections == null) {
failWithMessage("Expecting connections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnections(), connections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connections contains only the given VecConnection elements and nothing else in whatever order.
* @param connections the given elements that should be contained in actual VecConnectionSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connections does not contain all given VecConnection elements.
*/
public S hasOnlyConnections(VecConnection... connections) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnection varargs is not null.
if (connections == null) failWithMessage("Expecting connections parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnections(), connections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connections contains only the given VecConnection elements in Collection and nothing else in whatever order.
* @param connections the given elements that should be contained in actual VecConnectionSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connections does not contain all given VecConnection elements.
*/
public S hasOnlyConnections(java.util.Collection extends VecConnection> connections) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnection collection is not null.
if (connections == null) {
failWithMessage("Expecting connections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnections(), connections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connections does not contain the given VecConnection elements.
*
* @param connections the given elements that should not be in actual VecConnectionSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connections contains any given VecConnection elements.
*/
public S doesNotHaveConnections(VecConnection... connections) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnection varargs is not null.
if (connections == null) failWithMessage("Expecting connections parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnections(), connections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification's connections does not contain the given VecConnection elements in Collection.
*
* @param connections the given elements that should not be in actual VecConnectionSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connections contains any given VecConnection elements.
*/
public S doesNotHaveConnections(java.util.Collection extends VecConnection> connections) {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConnection collection is not null.
if (connections == null) {
failWithMessage("Expecting connections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnections(), connections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConnectionSpecification has no connections.
* @return this assertion object.
* @throws AssertionError if the actual VecConnectionSpecification's connections is not empty.
*/
public S hasNoConnections() {
// check that actual VecConnectionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have connections but had :\n <%s>";
// check
if (actual.getConnections().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getConnections());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy