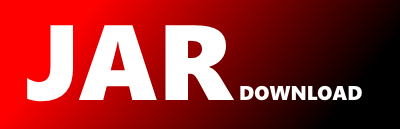
com.foursoft.vecmodel.vec120.AbstractVecContactingSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
/**
* Abstract base class for {@link VecContactingSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecContactingSpecificationAssert, A extends VecContactingSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecContactingSpecificationAssert}
to make assertions on actual VecContactingSpecification.
* @param actual the VecContactingSpecification we want to make assertions on.
*/
protected AbstractVecContactingSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecContactingSpecification's contactPoints contains the given VecContactPoint elements.
* @param contactPoints the given elements that should be contained in actual VecContactingSpecification's contactPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecContactingSpecification's contactPoints does not contain all given VecContactPoint elements.
*/
public S hasContactPoints(VecContactPoint... contactPoints) {
// check that actual VecContactingSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint varargs is not null.
if (contactPoints == null) failWithMessage("Expecting contactPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getContactPoints(), contactPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecContactingSpecification's contactPoints contains the given VecContactPoint elements in Collection.
* @param contactPoints the given elements that should be contained in actual VecContactingSpecification's contactPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecContactingSpecification's contactPoints does not contain all given VecContactPoint elements.
*/
public S hasContactPoints(java.util.Collection extends VecContactPoint> contactPoints) {
// check that actual VecContactingSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint collection is not null.
if (contactPoints == null) {
failWithMessage("Expecting contactPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getContactPoints(), contactPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecContactingSpecification's contactPoints contains only the given VecContactPoint elements and nothing else in whatever order.
* @param contactPoints the given elements that should be contained in actual VecContactingSpecification's contactPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecContactingSpecification's contactPoints does not contain all given VecContactPoint elements.
*/
public S hasOnlyContactPoints(VecContactPoint... contactPoints) {
// check that actual VecContactingSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint varargs is not null.
if (contactPoints == null) failWithMessage("Expecting contactPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getContactPoints(), contactPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecContactingSpecification's contactPoints contains only the given VecContactPoint elements in Collection and nothing else in whatever order.
* @param contactPoints the given elements that should be contained in actual VecContactingSpecification's contactPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecContactingSpecification's contactPoints does not contain all given VecContactPoint elements.
*/
public S hasOnlyContactPoints(java.util.Collection extends VecContactPoint> contactPoints) {
// check that actual VecContactingSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint collection is not null.
if (contactPoints == null) {
failWithMessage("Expecting contactPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getContactPoints(), contactPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecContactingSpecification's contactPoints does not contain the given VecContactPoint elements.
*
* @param contactPoints the given elements that should not be in actual VecContactingSpecification's contactPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecContactingSpecification's contactPoints contains any given VecContactPoint elements.
*/
public S doesNotHaveContactPoints(VecContactPoint... contactPoints) {
// check that actual VecContactingSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint varargs is not null.
if (contactPoints == null) failWithMessage("Expecting contactPoints parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getContactPoints(), contactPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecContactingSpecification's contactPoints does not contain the given VecContactPoint elements in Collection.
*
* @param contactPoints the given elements that should not be in actual VecContactingSpecification's contactPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecContactingSpecification's contactPoints contains any given VecContactPoint elements.
*/
public S doesNotHaveContactPoints(java.util.Collection extends VecContactPoint> contactPoints) {
// check that actual VecContactingSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint collection is not null.
if (contactPoints == null) {
failWithMessage("Expecting contactPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getContactPoints(), contactPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecContactingSpecification has no contactPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecContactingSpecification's contactPoints is not empty.
*/
public S hasNoContactPoints() {
// check that actual VecContactingSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have contactPoints but had :\n <%s>";
// check
if (actual.getContactPoints().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getContactPoints());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy