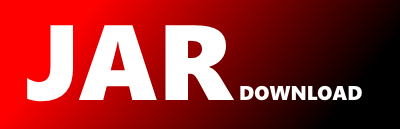
com.foursoft.vecmodel.vec120.AbstractVecCreationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecCreation} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCreationAssert, A extends VecCreation> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecCreationAssert}
to make assertions on actual VecCreation.
* @param actual the VecCreation we want to make assertions on.
*/
protected AbstractVecCreationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCreation's creationDate is equal to the given one.
* @param creationDate the given creationDate to compare the actual VecCreation's creationDate to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCreation's creationDate is not equal to the given one.
*/
public S hasCreationDate(javax.xml.datatype.XMLGregorianCalendar creationDate) {
// check that actual VecCreation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting creationDate of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
javax.xml.datatype.XMLGregorianCalendar actualCreationDate = actual.getCreationDate();
if (!Objects.areEqual(actualCreationDate, creationDate)) {
failWithMessage(assertjErrorMessage, actual, creationDate, actualCreationDate);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCreation's creator is equal to the given one.
* @param creator the given creator to compare the actual VecCreation's creator to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCreation's creator is not equal to the given one.
*/
public S hasCreator(VecPerson creator) {
// check that actual VecCreation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting creator of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPerson actualCreator = actual.getCreator();
if (!Objects.areEqual(actualCreator, creator)) {
failWithMessage(assertjErrorMessage, actual, creator, actualCreator);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCreation's parentItemVersion is equal to the given one.
* @param parentItemVersion the given parentItemVersion to compare the actual VecCreation's parentItemVersion to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCreation's parentItemVersion is not equal to the given one.
*/
public S hasParentItemVersion(VecItemVersion parentItemVersion) {
// check that actual VecCreation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentItemVersion of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecItemVersion actualParentItemVersion = actual.getParentItemVersion();
if (!Objects.areEqual(actualParentItemVersion, parentItemVersion)) {
failWithMessage(assertjErrorMessage, actual, parentItemVersion, actualParentItemVersion);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCreation's responsibleDesigner is equal to the given one.
* @param responsibleDesigner the given responsibleDesigner to compare the actual VecCreation's responsibleDesigner to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCreation's responsibleDesigner is not equal to the given one.
*/
public S hasResponsibleDesigner(VecPerson responsibleDesigner) {
// check that actual VecCreation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting responsibleDesigner of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPerson actualResponsibleDesigner = actual.getResponsibleDesigner();
if (!Objects.areEqual(actualResponsibleDesigner, responsibleDesigner)) {
failWithMessage(assertjErrorMessage, actual, responsibleDesigner, actualResponsibleDesigner);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy