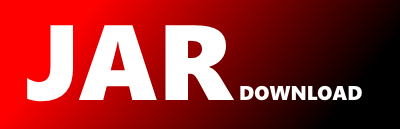
com.foursoft.vecmodel.vec120.AbstractVecDefaultDimensionAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.AbstractObjectAssert;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecDefaultDimension} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecDefaultDimensionAssert, A extends VecDefaultDimension> extends AbstractObjectAssert {
/**
* Creates a new {@link AbstractVecDefaultDimensionAssert}
to make assertions on actual VecDefaultDimension.
* @param actual the VecDefaultDimension we want to make assertions on.
*/
protected AbstractVecDefaultDimensionAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecDefaultDimension's dimensionType is equal to the given one.
* @param dimensionType the given dimensionType to compare the actual VecDefaultDimension's dimensionType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDefaultDimension's dimensionType is not equal to the given one.
*/
public S hasDimensionType(String dimensionType) {
// check that actual VecDefaultDimension we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting dimensionType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualDimensionType = actual.getDimensionType();
if (!Objects.areEqual(actualDimensionType, dimensionType)) {
failWithMessage(assertjErrorMessage, actual, dimensionType, actualDimensionType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDefaultDimension's dimensionValueRange is equal to the given one.
* @param dimensionValueRange the given dimensionValueRange to compare the actual VecDefaultDimension's dimensionValueRange to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDefaultDimension's dimensionValueRange is not equal to the given one.
*/
public S hasDimensionValueRange(VecValueRange dimensionValueRange) {
// check that actual VecDefaultDimension we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting dimensionValueRange of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecValueRange actualDimensionValueRange = actual.getDimensionValueRange();
if (!Objects.areEqual(actualDimensionValueRange, dimensionValueRange)) {
failWithMessage(assertjErrorMessage, actual, dimensionValueRange, actualDimensionValueRange);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDefaultDimension's parentDefaultDimensionSpecification is equal to the given one.
* @param parentDefaultDimensionSpecification the given parentDefaultDimensionSpecification to compare the actual VecDefaultDimension's parentDefaultDimensionSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDefaultDimension's parentDefaultDimensionSpecification is not equal to the given one.
*/
public S hasParentDefaultDimensionSpecification(VecDefaultDimensionSpecification parentDefaultDimensionSpecification) {
// check that actual VecDefaultDimension we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentDefaultDimensionSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecDefaultDimensionSpecification actualParentDefaultDimensionSpecification = actual.getParentDefaultDimensionSpecification();
if (!Objects.areEqual(actualParentDefaultDimensionSpecification, parentDefaultDimensionSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentDefaultDimensionSpecification, actualParentDefaultDimensionSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDefaultDimension's toleranceIndication is equal to the given one.
* @param toleranceIndication the given toleranceIndication to compare the actual VecDefaultDimension's toleranceIndication to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDefaultDimension's toleranceIndication is not equal to the given one.
*/
public S hasToleranceIndication(VecTolerance toleranceIndication) {
// check that actual VecDefaultDimension we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting toleranceIndication of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTolerance actualToleranceIndication = actual.getToleranceIndication();
if (!Objects.areEqual(actualToleranceIndication, toleranceIndication)) {
failWithMessage(assertjErrorMessage, actual, toleranceIndication, actualToleranceIndication);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDefaultDimension's xmlId is equal to the given one.
* @param xmlId the given xmlId to compare the actual VecDefaultDimension's xmlId to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDefaultDimension's xmlId is not equal to the given one.
*/
public S hasXmlId(String xmlId) {
// check that actual VecDefaultDimension we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting xmlId of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualXmlId = actual.getXmlId();
if (!Objects.areEqual(actualXmlId, xmlId)) {
failWithMessage(assertjErrorMessage, actual, xmlId, actualXmlId);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy