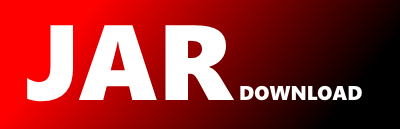
com.foursoft.vecmodel.vec120.AbstractVecDocumentVersionAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecDocumentVersion} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecDocumentVersionAssert, A extends VecDocumentVersion> extends AbstractVecItemVersionAssert {
/**
* Creates a new {@link AbstractVecDocumentVersionAssert}
to make assertions on actual VecDocumentVersion.
* @param actual the VecDocumentVersion we want to make assertions on.
*/
protected AbstractVecDocumentVersionAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecDocumentVersion's creatingSystem is equal to the given one.
* @param creatingSystem the given creatingSystem to compare the actual VecDocumentVersion's creatingSystem to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's creatingSystem is not equal to the given one.
*/
public S hasCreatingSystem(String creatingSystem) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting creatingSystem of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualCreatingSystem = actual.getCreatingSystem();
if (!Objects.areEqual(actualCreatingSystem, creatingSystem)) {
failWithMessage(assertjErrorMessage, actual, creatingSystem, actualCreatingSystem);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's dataFormat is equal to the given one.
* @param dataFormat the given dataFormat to compare the actual VecDocumentVersion's dataFormat to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's dataFormat is not equal to the given one.
*/
public S hasDataFormat(String dataFormat) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting dataFormat of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualDataFormat = actual.getDataFormat();
if (!Objects.areEqual(actualDataFormat, dataFormat)) {
failWithMessage(assertjErrorMessage, actual, dataFormat, actualDataFormat);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's digitalRepresentationIndex is equal to the given one.
* @param digitalRepresentationIndex the given digitalRepresentationIndex to compare the actual VecDocumentVersion's digitalRepresentationIndex to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's digitalRepresentationIndex is not equal to the given one.
*/
public S hasDigitalRepresentationIndex(String digitalRepresentationIndex) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting digitalRepresentationIndex of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualDigitalRepresentationIndex = actual.getDigitalRepresentationIndex();
if (!Objects.areEqual(actualDigitalRepresentationIndex, digitalRepresentationIndex)) {
failWithMessage(assertjErrorMessage, actual, digitalRepresentationIndex, actualDigitalRepresentationIndex);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's documentNumber is equal to the given one.
* @param documentNumber the given documentNumber to compare the actual VecDocumentVersion's documentNumber to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's documentNumber is not equal to the given one.
*/
public S hasDocumentNumber(String documentNumber) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting documentNumber of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualDocumentNumber = actual.getDocumentNumber();
if (!Objects.areEqual(actualDocumentNumber, documentNumber)) {
failWithMessage(assertjErrorMessage, actual, documentNumber, actualDocumentNumber);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's documentType is equal to the given one.
* @param documentType the given documentType to compare the actual VecDocumentVersion's documentType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's documentType is not equal to the given one.
*/
public S hasDocumentType(String documentType) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting documentType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualDocumentType = actual.getDocumentType();
if (!Objects.areEqual(actualDocumentType, documentType)) {
failWithMessage(assertjErrorMessage, actual, documentType, actualDocumentType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's documentVersion is equal to the given one.
* @param documentVersion the given documentVersion to compare the actual VecDocumentVersion's documentVersion to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's documentVersion is not equal to the given one.
*/
public S hasDocumentVersion(String documentVersion) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting documentVersion of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualDocumentVersion = actual.getDocumentVersion();
if (!Objects.areEqual(actualDocumentVersion, documentVersion)) {
failWithMessage(assertjErrorMessage, actual, documentVersion, actualDocumentVersion);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's fileName is equal to the given one.
* @param fileName the given fileName to compare the actual VecDocumentVersion's fileName to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's fileName is not equal to the given one.
*/
public S hasFileName(String fileName) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting fileName of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualFileName = actual.getFileName();
if (!Objects.areEqual(actualFileName, fileName)) {
failWithMessage(assertjErrorMessage, actual, fileName, actualFileName);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's itemEquivalences contains the given VecItemEquivalence elements.
* @param itemEquivalences the given elements that should be contained in actual VecDocumentVersion's itemEquivalences.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's itemEquivalences does not contain all given VecItemEquivalence elements.
*/
public S hasItemEquivalences(VecItemEquivalence... itemEquivalences) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence varargs is not null.
if (itemEquivalences == null) failWithMessage("Expecting itemEquivalences parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getItemEquivalences(), itemEquivalences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's itemEquivalences contains the given VecItemEquivalence elements in Collection.
* @param itemEquivalences the given elements that should be contained in actual VecDocumentVersion's itemEquivalences.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's itemEquivalences does not contain all given VecItemEquivalence elements.
*/
public S hasItemEquivalences(java.util.Collection extends VecItemEquivalence> itemEquivalences) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence collection is not null.
if (itemEquivalences == null) {
failWithMessage("Expecting itemEquivalences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getItemEquivalences(), itemEquivalences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's itemEquivalences contains only the given VecItemEquivalence elements and nothing else in whatever order.
* @param itemEquivalences the given elements that should be contained in actual VecDocumentVersion's itemEquivalences.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's itemEquivalences does not contain all given VecItemEquivalence elements.
*/
public S hasOnlyItemEquivalences(VecItemEquivalence... itemEquivalences) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence varargs is not null.
if (itemEquivalences == null) failWithMessage("Expecting itemEquivalences parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getItemEquivalences(), itemEquivalences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's itemEquivalences contains only the given VecItemEquivalence elements in Collection and nothing else in whatever order.
* @param itemEquivalences the given elements that should be contained in actual VecDocumentVersion's itemEquivalences.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's itemEquivalences does not contain all given VecItemEquivalence elements.
*/
public S hasOnlyItemEquivalences(java.util.Collection extends VecItemEquivalence> itemEquivalences) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence collection is not null.
if (itemEquivalences == null) {
failWithMessage("Expecting itemEquivalences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getItemEquivalences(), itemEquivalences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's itemEquivalences does not contain the given VecItemEquivalence elements.
*
* @param itemEquivalences the given elements that should not be in actual VecDocumentVersion's itemEquivalences.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's itemEquivalences contains any given VecItemEquivalence elements.
*/
public S doesNotHaveItemEquivalences(VecItemEquivalence... itemEquivalences) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence varargs is not null.
if (itemEquivalences == null) failWithMessage("Expecting itemEquivalences parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getItemEquivalences(), itemEquivalences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's itemEquivalences does not contain the given VecItemEquivalence elements in Collection.
*
* @param itemEquivalences the given elements that should not be in actual VecDocumentVersion's itemEquivalences.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's itemEquivalences contains any given VecItemEquivalence elements.
*/
public S doesNotHaveItemEquivalences(java.util.Collection extends VecItemEquivalence> itemEquivalences) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence collection is not null.
if (itemEquivalences == null) {
failWithMessage("Expecting itemEquivalences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getItemEquivalences(), itemEquivalences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no itemEquivalences.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's itemEquivalences is not empty.
*/
public S hasNoItemEquivalences() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have itemEquivalences but had :\n <%s>";
// check
if (actual.getItemEquivalences().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getItemEquivalences());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's location is equal to the given one.
* @param location the given location to compare the actual VecDocumentVersion's location to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's location is not equal to the given one.
*/
public S hasLocation(String location) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting location of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualLocation = actual.getLocation();
if (!Objects.areEqual(actualLocation, location)) {
failWithMessage(assertjErrorMessage, actual, location, actualLocation);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's numberOfSheets is equal to the given one.
* @param numberOfSheets the given numberOfSheets to compare the actual VecDocumentVersion's numberOfSheets to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's numberOfSheets is not equal to the given one.
*/
public S hasNumberOfSheets(String numberOfSheets) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting numberOfSheets of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualNumberOfSheets = actual.getNumberOfSheets();
if (!Objects.areEqual(actualNumberOfSheets, numberOfSheets)) {
failWithMessage(assertjErrorMessage, actual, numberOfSheets, actualNumberOfSheets);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's parentVecContent is equal to the given one.
* @param parentVecContent the given parentVecContent to compare the actual VecDocumentVersion's parentVecContent to.
* @return this assertion object.
* @throws AssertionError - if the actual VecDocumentVersion's parentVecContent is not equal to the given one.
*/
public S hasParentVecContent(VecContent parentVecContent) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentVecContent of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecContent actualParentVecContent = actual.getParentVecContent();
if (!Objects.areEqual(actualParentVecContent, parentVecContent)) {
failWithMessage(assertjErrorMessage, actual, parentVecContent, actualParentVecContent);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentBasedInstruction contains the given VecDocumentBasedInstruction elements.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecDocumentVersion's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasRefDocumentBasedInstruction(VecDocumentBasedInstruction... refDocumentBasedInstruction) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction varargs is not null.
if (refDocumentBasedInstruction == null) failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentBasedInstruction contains the given VecDocumentBasedInstruction elements in Collection.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecDocumentVersion's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasRefDocumentBasedInstruction(java.util.Collection extends VecDocumentBasedInstruction> refDocumentBasedInstruction) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction collection is not null.
if (refDocumentBasedInstruction == null) {
failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentBasedInstruction contains only the given VecDocumentBasedInstruction elements and nothing else in whatever order.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecDocumentVersion's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasOnlyRefDocumentBasedInstruction(VecDocumentBasedInstruction... refDocumentBasedInstruction) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction varargs is not null.
if (refDocumentBasedInstruction == null) failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentBasedInstruction contains only the given VecDocumentBasedInstruction elements in Collection and nothing else in whatever order.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecDocumentVersion's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasOnlyRefDocumentBasedInstruction(java.util.Collection extends VecDocumentBasedInstruction> refDocumentBasedInstruction) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction collection is not null.
if (refDocumentBasedInstruction == null) {
failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentBasedInstruction does not contain the given VecDocumentBasedInstruction elements.
*
* @param refDocumentBasedInstruction the given elements that should not be in actual VecDocumentVersion's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentBasedInstruction contains any given VecDocumentBasedInstruction elements.
*/
public S doesNotHaveRefDocumentBasedInstruction(VecDocumentBasedInstruction... refDocumentBasedInstruction) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction varargs is not null.
if (refDocumentBasedInstruction == null) failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentBasedInstruction does not contain the given VecDocumentBasedInstruction elements in Collection.
*
* @param refDocumentBasedInstruction the given elements that should not be in actual VecDocumentVersion's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentBasedInstruction contains any given VecDocumentBasedInstruction elements.
*/
public S doesNotHaveRefDocumentBasedInstruction(java.util.Collection extends VecDocumentBasedInstruction> refDocumentBasedInstruction) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction collection is not null.
if (refDocumentBasedInstruction == null) {
failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentBasedInstruction is not empty.
*/
public S hasNoRefDocumentBasedInstruction() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDocumentBasedInstruction but had :\n <%s>";
// check
if (actual.getRefDocumentBasedInstruction().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDocumentBasedInstruction());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup contains the given VecDocumentRelatedAssignmentGroup elements.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecDocumentVersion's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasRefDocumentRelatedAssignmentGroup(VecDocumentRelatedAssignmentGroup... refDocumentRelatedAssignmentGroup) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup varargs is not null.
if (refDocumentRelatedAssignmentGroup == null) failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup contains the given VecDocumentRelatedAssignmentGroup elements in Collection.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecDocumentVersion's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasRefDocumentRelatedAssignmentGroup(java.util.Collection extends VecDocumentRelatedAssignmentGroup> refDocumentRelatedAssignmentGroup) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup collection is not null.
if (refDocumentRelatedAssignmentGroup == null) {
failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup contains only the given VecDocumentRelatedAssignmentGroup elements and nothing else in whatever order.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecDocumentVersion's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasOnlyRefDocumentRelatedAssignmentGroup(VecDocumentRelatedAssignmentGroup... refDocumentRelatedAssignmentGroup) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup varargs is not null.
if (refDocumentRelatedAssignmentGroup == null) failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup contains only the given VecDocumentRelatedAssignmentGroup elements in Collection and nothing else in whatever order.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecDocumentVersion's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasOnlyRefDocumentRelatedAssignmentGroup(java.util.Collection extends VecDocumentRelatedAssignmentGroup> refDocumentRelatedAssignmentGroup) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup collection is not null.
if (refDocumentRelatedAssignmentGroup == null) {
failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup does not contain the given VecDocumentRelatedAssignmentGroup elements.
*
* @param refDocumentRelatedAssignmentGroup the given elements that should not be in actual VecDocumentVersion's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup contains any given VecDocumentRelatedAssignmentGroup elements.
*/
public S doesNotHaveRefDocumentRelatedAssignmentGroup(VecDocumentRelatedAssignmentGroup... refDocumentRelatedAssignmentGroup) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup varargs is not null.
if (refDocumentRelatedAssignmentGroup == null) failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup does not contain the given VecDocumentRelatedAssignmentGroup elements in Collection.
*
* @param refDocumentRelatedAssignmentGroup the given elements that should not be in actual VecDocumentVersion's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup contains any given VecDocumentRelatedAssignmentGroup elements.
*/
public S doesNotHaveRefDocumentRelatedAssignmentGroup(java.util.Collection extends VecDocumentRelatedAssignmentGroup> refDocumentRelatedAssignmentGroup) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup collection is not null.
if (refDocumentRelatedAssignmentGroup == null) {
failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentRelatedAssignmentGroup is not empty.
*/
public S hasNoRefDocumentRelatedAssignmentGroup() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDocumentRelatedAssignmentGroup but had :\n <%s>";
// check
if (actual.getRefDocumentRelatedAssignmentGroup().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDocumentRelatedAssignmentGroup());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentVersion contains the given VecDocumentVersion elements.
* @param refDocumentVersion the given elements that should be contained in actual VecDocumentVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasRefDocumentVersion(VecDocumentVersion... refDocumentVersion) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (refDocumentVersion == null) failWithMessage("Expecting refDocumentVersion parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentVersion(), refDocumentVersion);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentVersion contains the given VecDocumentVersion elements in Collection.
* @param refDocumentVersion the given elements that should be contained in actual VecDocumentVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasRefDocumentVersion(java.util.Collection extends VecDocumentVersion> refDocumentVersion) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (refDocumentVersion == null) {
failWithMessage("Expecting refDocumentVersion parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentVersion(), refDocumentVersion.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentVersion contains only the given VecDocumentVersion elements and nothing else in whatever order.
* @param refDocumentVersion the given elements that should be contained in actual VecDocumentVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasOnlyRefDocumentVersion(VecDocumentVersion... refDocumentVersion) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (refDocumentVersion == null) failWithMessage("Expecting refDocumentVersion parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentVersion(), refDocumentVersion);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentVersion contains only the given VecDocumentVersion elements in Collection and nothing else in whatever order.
* @param refDocumentVersion the given elements that should be contained in actual VecDocumentVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasOnlyRefDocumentVersion(java.util.Collection extends VecDocumentVersion> refDocumentVersion) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (refDocumentVersion == null) {
failWithMessage("Expecting refDocumentVersion parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentVersion(), refDocumentVersion.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentVersion does not contain the given VecDocumentVersion elements.
*
* @param refDocumentVersion the given elements that should not be in actual VecDocumentVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentVersion contains any given VecDocumentVersion elements.
*/
public S doesNotHaveRefDocumentVersion(VecDocumentVersion... refDocumentVersion) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (refDocumentVersion == null) failWithMessage("Expecting refDocumentVersion parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentVersion(), refDocumentVersion);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refDocumentVersion does not contain the given VecDocumentVersion elements in Collection.
*
* @param refDocumentVersion the given elements that should not be in actual VecDocumentVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentVersion contains any given VecDocumentVersion elements.
*/
public S doesNotHaveRefDocumentVersion(java.util.Collection extends VecDocumentVersion> refDocumentVersion) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (refDocumentVersion == null) {
failWithMessage("Expecting refDocumentVersion parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentVersion(), refDocumentVersion.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refDocumentVersion is not empty.
*/
public S hasNoRefDocumentVersion() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDocumentVersion but had :\n <%s>";
// check
if (actual.getRefDocumentVersion().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDocumentVersion());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExtendableElement contains the given VecExtendableElement elements.
* @param refExtendableElement the given elements that should be contained in actual VecDocumentVersion's refExtendableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExtendableElement does not contain all given VecExtendableElement elements.
*/
public S hasRefExtendableElement(VecExtendableElement... refExtendableElement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExtendableElement varargs is not null.
if (refExtendableElement == null) failWithMessage("Expecting refExtendableElement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefExtendableElement(), refExtendableElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExtendableElement contains the given VecExtendableElement elements in Collection.
* @param refExtendableElement the given elements that should be contained in actual VecDocumentVersion's refExtendableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExtendableElement does not contain all given VecExtendableElement elements.
*/
public S hasRefExtendableElement(java.util.Collection extends VecExtendableElement> refExtendableElement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExtendableElement collection is not null.
if (refExtendableElement == null) {
failWithMessage("Expecting refExtendableElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefExtendableElement(), refExtendableElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExtendableElement contains only the given VecExtendableElement elements and nothing else in whatever order.
* @param refExtendableElement the given elements that should be contained in actual VecDocumentVersion's refExtendableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExtendableElement does not contain all given VecExtendableElement elements.
*/
public S hasOnlyRefExtendableElement(VecExtendableElement... refExtendableElement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExtendableElement varargs is not null.
if (refExtendableElement == null) failWithMessage("Expecting refExtendableElement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefExtendableElement(), refExtendableElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExtendableElement contains only the given VecExtendableElement elements in Collection and nothing else in whatever order.
* @param refExtendableElement the given elements that should be contained in actual VecDocumentVersion's refExtendableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExtendableElement does not contain all given VecExtendableElement elements.
*/
public S hasOnlyRefExtendableElement(java.util.Collection extends VecExtendableElement> refExtendableElement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExtendableElement collection is not null.
if (refExtendableElement == null) {
failWithMessage("Expecting refExtendableElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefExtendableElement(), refExtendableElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExtendableElement does not contain the given VecExtendableElement elements.
*
* @param refExtendableElement the given elements that should not be in actual VecDocumentVersion's refExtendableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExtendableElement contains any given VecExtendableElement elements.
*/
public S doesNotHaveRefExtendableElement(VecExtendableElement... refExtendableElement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExtendableElement varargs is not null.
if (refExtendableElement == null) failWithMessage("Expecting refExtendableElement parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefExtendableElement(), refExtendableElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExtendableElement does not contain the given VecExtendableElement elements in Collection.
*
* @param refExtendableElement the given elements that should not be in actual VecDocumentVersion's refExtendableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExtendableElement contains any given VecExtendableElement elements.
*/
public S doesNotHaveRefExtendableElement(java.util.Collection extends VecExtendableElement> refExtendableElement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExtendableElement collection is not null.
if (refExtendableElement == null) {
failWithMessage("Expecting refExtendableElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefExtendableElement(), refExtendableElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no refExtendableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExtendableElement is not empty.
*/
public S hasNoRefExtendableElement() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refExtendableElement but had :\n <%s>";
// check
if (actual.getRefExtendableElement().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefExtendableElement());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExternalMappingSpecification contains the given VecExternalMappingSpecification elements.
* @param refExternalMappingSpecification the given elements that should be contained in actual VecDocumentVersion's refExternalMappingSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExternalMappingSpecification does not contain all given VecExternalMappingSpecification elements.
*/
public S hasRefExternalMappingSpecification(VecExternalMappingSpecification... refExternalMappingSpecification) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExternalMappingSpecification varargs is not null.
if (refExternalMappingSpecification == null) failWithMessage("Expecting refExternalMappingSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefExternalMappingSpecification(), refExternalMappingSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExternalMappingSpecification contains the given VecExternalMappingSpecification elements in Collection.
* @param refExternalMappingSpecification the given elements that should be contained in actual VecDocumentVersion's refExternalMappingSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExternalMappingSpecification does not contain all given VecExternalMappingSpecification elements.
*/
public S hasRefExternalMappingSpecification(java.util.Collection extends VecExternalMappingSpecification> refExternalMappingSpecification) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExternalMappingSpecification collection is not null.
if (refExternalMappingSpecification == null) {
failWithMessage("Expecting refExternalMappingSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefExternalMappingSpecification(), refExternalMappingSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExternalMappingSpecification contains only the given VecExternalMappingSpecification elements and nothing else in whatever order.
* @param refExternalMappingSpecification the given elements that should be contained in actual VecDocumentVersion's refExternalMappingSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExternalMappingSpecification does not contain all given VecExternalMappingSpecification elements.
*/
public S hasOnlyRefExternalMappingSpecification(VecExternalMappingSpecification... refExternalMappingSpecification) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExternalMappingSpecification varargs is not null.
if (refExternalMappingSpecification == null) failWithMessage("Expecting refExternalMappingSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefExternalMappingSpecification(), refExternalMappingSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExternalMappingSpecification contains only the given VecExternalMappingSpecification elements in Collection and nothing else in whatever order.
* @param refExternalMappingSpecification the given elements that should be contained in actual VecDocumentVersion's refExternalMappingSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExternalMappingSpecification does not contain all given VecExternalMappingSpecification elements.
*/
public S hasOnlyRefExternalMappingSpecification(java.util.Collection extends VecExternalMappingSpecification> refExternalMappingSpecification) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExternalMappingSpecification collection is not null.
if (refExternalMappingSpecification == null) {
failWithMessage("Expecting refExternalMappingSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefExternalMappingSpecification(), refExternalMappingSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExternalMappingSpecification does not contain the given VecExternalMappingSpecification elements.
*
* @param refExternalMappingSpecification the given elements that should not be in actual VecDocumentVersion's refExternalMappingSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExternalMappingSpecification contains any given VecExternalMappingSpecification elements.
*/
public S doesNotHaveRefExternalMappingSpecification(VecExternalMappingSpecification... refExternalMappingSpecification) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExternalMappingSpecification varargs is not null.
if (refExternalMappingSpecification == null) failWithMessage("Expecting refExternalMappingSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefExternalMappingSpecification(), refExternalMappingSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refExternalMappingSpecification does not contain the given VecExternalMappingSpecification elements in Collection.
*
* @param refExternalMappingSpecification the given elements that should not be in actual VecDocumentVersion's refExternalMappingSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExternalMappingSpecification contains any given VecExternalMappingSpecification elements.
*/
public S doesNotHaveRefExternalMappingSpecification(java.util.Collection extends VecExternalMappingSpecification> refExternalMappingSpecification) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecExternalMappingSpecification collection is not null.
if (refExternalMappingSpecification == null) {
failWithMessage("Expecting refExternalMappingSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefExternalMappingSpecification(), refExternalMappingSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no refExternalMappingSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refExternalMappingSpecification is not empty.
*/
public S hasNoRefExternalMappingSpecification() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refExternalMappingSpecification but had :\n <%s>";
// check
if (actual.getRefExternalMappingSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefExternalMappingSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refRequirementsConformanceStatement contains the given VecRequirementsConformanceStatement elements.
* @param refRequirementsConformanceStatement the given elements that should be contained in actual VecDocumentVersion's refRequirementsConformanceStatement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refRequirementsConformanceStatement does not contain all given VecRequirementsConformanceStatement elements.
*/
public S hasRefRequirementsConformanceStatement(VecRequirementsConformanceStatement... refRequirementsConformanceStatement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecRequirementsConformanceStatement varargs is not null.
if (refRequirementsConformanceStatement == null) failWithMessage("Expecting refRequirementsConformanceStatement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefRequirementsConformanceStatement(), refRequirementsConformanceStatement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refRequirementsConformanceStatement contains the given VecRequirementsConformanceStatement elements in Collection.
* @param refRequirementsConformanceStatement the given elements that should be contained in actual VecDocumentVersion's refRequirementsConformanceStatement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refRequirementsConformanceStatement does not contain all given VecRequirementsConformanceStatement elements.
*/
public S hasRefRequirementsConformanceStatement(java.util.Collection extends VecRequirementsConformanceStatement> refRequirementsConformanceStatement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecRequirementsConformanceStatement collection is not null.
if (refRequirementsConformanceStatement == null) {
failWithMessage("Expecting refRequirementsConformanceStatement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefRequirementsConformanceStatement(), refRequirementsConformanceStatement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refRequirementsConformanceStatement contains only the given VecRequirementsConformanceStatement elements and nothing else in whatever order.
* @param refRequirementsConformanceStatement the given elements that should be contained in actual VecDocumentVersion's refRequirementsConformanceStatement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refRequirementsConformanceStatement does not contain all given VecRequirementsConformanceStatement elements.
*/
public S hasOnlyRefRequirementsConformanceStatement(VecRequirementsConformanceStatement... refRequirementsConformanceStatement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecRequirementsConformanceStatement varargs is not null.
if (refRequirementsConformanceStatement == null) failWithMessage("Expecting refRequirementsConformanceStatement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefRequirementsConformanceStatement(), refRequirementsConformanceStatement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refRequirementsConformanceStatement contains only the given VecRequirementsConformanceStatement elements in Collection and nothing else in whatever order.
* @param refRequirementsConformanceStatement the given elements that should be contained in actual VecDocumentVersion's refRequirementsConformanceStatement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refRequirementsConformanceStatement does not contain all given VecRequirementsConformanceStatement elements.
*/
public S hasOnlyRefRequirementsConformanceStatement(java.util.Collection extends VecRequirementsConformanceStatement> refRequirementsConformanceStatement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecRequirementsConformanceStatement collection is not null.
if (refRequirementsConformanceStatement == null) {
failWithMessage("Expecting refRequirementsConformanceStatement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefRequirementsConformanceStatement(), refRequirementsConformanceStatement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refRequirementsConformanceStatement does not contain the given VecRequirementsConformanceStatement elements.
*
* @param refRequirementsConformanceStatement the given elements that should not be in actual VecDocumentVersion's refRequirementsConformanceStatement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refRequirementsConformanceStatement contains any given VecRequirementsConformanceStatement elements.
*/
public S doesNotHaveRefRequirementsConformanceStatement(VecRequirementsConformanceStatement... refRequirementsConformanceStatement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecRequirementsConformanceStatement varargs is not null.
if (refRequirementsConformanceStatement == null) failWithMessage("Expecting refRequirementsConformanceStatement parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefRequirementsConformanceStatement(), refRequirementsConformanceStatement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's refRequirementsConformanceStatement does not contain the given VecRequirementsConformanceStatement elements in Collection.
*
* @param refRequirementsConformanceStatement the given elements that should not be in actual VecDocumentVersion's refRequirementsConformanceStatement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refRequirementsConformanceStatement contains any given VecRequirementsConformanceStatement elements.
*/
public S doesNotHaveRefRequirementsConformanceStatement(java.util.Collection extends VecRequirementsConformanceStatement> refRequirementsConformanceStatement) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecRequirementsConformanceStatement collection is not null.
if (refRequirementsConformanceStatement == null) {
failWithMessage("Expecting refRequirementsConformanceStatement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefRequirementsConformanceStatement(), refRequirementsConformanceStatement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no refRequirementsConformanceStatement.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's refRequirementsConformanceStatement is not empty.
*/
public S hasNoRefRequirementsConformanceStatement() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refRequirementsConformanceStatement but had :\n <%s>";
// check
if (actual.getRefRequirementsConformanceStatement().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefRequirementsConformanceStatement());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's referencedPart contains the given VecPartVersion elements.
* @param referencedPart the given elements that should be contained in actual VecDocumentVersion's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasReferencedPart(VecPartVersion... referencedPart) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion varargs is not null.
if (referencedPart == null) failWithMessage("Expecting referencedPart parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getReferencedPart(), referencedPart);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's referencedPart contains the given VecPartVersion elements in Collection.
* @param referencedPart the given elements that should be contained in actual VecDocumentVersion's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasReferencedPart(java.util.Collection extends VecPartVersion> referencedPart) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion collection is not null.
if (referencedPart == null) {
failWithMessage("Expecting referencedPart parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getReferencedPart(), referencedPart.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's referencedPart contains only the given VecPartVersion elements and nothing else in whatever order.
* @param referencedPart the given elements that should be contained in actual VecDocumentVersion's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasOnlyReferencedPart(VecPartVersion... referencedPart) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion varargs is not null.
if (referencedPart == null) failWithMessage("Expecting referencedPart parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getReferencedPart(), referencedPart);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's referencedPart contains only the given VecPartVersion elements in Collection and nothing else in whatever order.
* @param referencedPart the given elements that should be contained in actual VecDocumentVersion's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasOnlyReferencedPart(java.util.Collection extends VecPartVersion> referencedPart) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion collection is not null.
if (referencedPart == null) {
failWithMessage("Expecting referencedPart parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getReferencedPart(), referencedPart.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's referencedPart does not contain the given VecPartVersion elements.
*
* @param referencedPart the given elements that should not be in actual VecDocumentVersion's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's referencedPart contains any given VecPartVersion elements.
*/
public S doesNotHaveReferencedPart(VecPartVersion... referencedPart) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion varargs is not null.
if (referencedPart == null) failWithMessage("Expecting referencedPart parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getReferencedPart(), referencedPart);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's referencedPart does not contain the given VecPartVersion elements in Collection.
*
* @param referencedPart the given elements that should not be in actual VecDocumentVersion's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's referencedPart contains any given VecPartVersion elements.
*/
public S doesNotHaveReferencedPart(java.util.Collection extends VecPartVersion> referencedPart) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion collection is not null.
if (referencedPart == null) {
failWithMessage("Expecting referencedPart parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getReferencedPart(), referencedPart.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's referencedPart is not empty.
*/
public S hasNoReferencedPart() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have referencedPart but had :\n <%s>";
// check
if (actual.getReferencedPart().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getReferencedPart());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's relatedDocument contains the given VecDocumentVersion elements.
* @param relatedDocument the given elements that should be contained in actual VecDocumentVersion's relatedDocument.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's relatedDocument does not contain all given VecDocumentVersion elements.
*/
public S hasRelatedDocument(VecDocumentVersion... relatedDocument) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (relatedDocument == null) failWithMessage("Expecting relatedDocument parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRelatedDocument(), relatedDocument);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's relatedDocument contains the given VecDocumentVersion elements in Collection.
* @param relatedDocument the given elements that should be contained in actual VecDocumentVersion's relatedDocument.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's relatedDocument does not contain all given VecDocumentVersion elements.
*/
public S hasRelatedDocument(java.util.Collection extends VecDocumentVersion> relatedDocument) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (relatedDocument == null) {
failWithMessage("Expecting relatedDocument parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRelatedDocument(), relatedDocument.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's relatedDocument contains only the given VecDocumentVersion elements and nothing else in whatever order.
* @param relatedDocument the given elements that should be contained in actual VecDocumentVersion's relatedDocument.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's relatedDocument does not contain all given VecDocumentVersion elements.
*/
public S hasOnlyRelatedDocument(VecDocumentVersion... relatedDocument) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (relatedDocument == null) failWithMessage("Expecting relatedDocument parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRelatedDocument(), relatedDocument);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's relatedDocument contains only the given VecDocumentVersion elements in Collection and nothing else in whatever order.
* @param relatedDocument the given elements that should be contained in actual VecDocumentVersion's relatedDocument.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's relatedDocument does not contain all given VecDocumentVersion elements.
*/
public S hasOnlyRelatedDocument(java.util.Collection extends VecDocumentVersion> relatedDocument) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (relatedDocument == null) {
failWithMessage("Expecting relatedDocument parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRelatedDocument(), relatedDocument.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's relatedDocument does not contain the given VecDocumentVersion elements.
*
* @param relatedDocument the given elements that should not be in actual VecDocumentVersion's relatedDocument.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's relatedDocument contains any given VecDocumentVersion elements.
*/
public S doesNotHaveRelatedDocument(VecDocumentVersion... relatedDocument) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (relatedDocument == null) failWithMessage("Expecting relatedDocument parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRelatedDocument(), relatedDocument);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's relatedDocument does not contain the given VecDocumentVersion elements in Collection.
*
* @param relatedDocument the given elements that should not be in actual VecDocumentVersion's relatedDocument.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's relatedDocument contains any given VecDocumentVersion elements.
*/
public S doesNotHaveRelatedDocument(java.util.Collection extends VecDocumentVersion> relatedDocument) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (relatedDocument == null) {
failWithMessage("Expecting relatedDocument parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRelatedDocument(), relatedDocument.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no relatedDocument.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's relatedDocument is not empty.
*/
public S hasNoRelatedDocument() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have relatedDocument but had :\n <%s>";
// check
if (actual.getRelatedDocument().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRelatedDocument());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's sheetOrChapters contains the given VecSheetOrChapter elements.
* @param sheetOrChapters the given elements that should be contained in actual VecDocumentVersion's sheetOrChapters.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's sheetOrChapters does not contain all given VecSheetOrChapter elements.
*/
public S hasSheetOrChapters(VecSheetOrChapter... sheetOrChapters) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter varargs is not null.
if (sheetOrChapters == null) failWithMessage("Expecting sheetOrChapters parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSheetOrChapters(), sheetOrChapters);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's sheetOrChapters contains the given VecSheetOrChapter elements in Collection.
* @param sheetOrChapters the given elements that should be contained in actual VecDocumentVersion's sheetOrChapters.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's sheetOrChapters does not contain all given VecSheetOrChapter elements.
*/
public S hasSheetOrChapters(java.util.Collection extends VecSheetOrChapter> sheetOrChapters) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter collection is not null.
if (sheetOrChapters == null) {
failWithMessage("Expecting sheetOrChapters parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSheetOrChapters(), sheetOrChapters.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's sheetOrChapters contains only the given VecSheetOrChapter elements and nothing else in whatever order.
* @param sheetOrChapters the given elements that should be contained in actual VecDocumentVersion's sheetOrChapters.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's sheetOrChapters does not contain all given VecSheetOrChapter elements.
*/
public S hasOnlySheetOrChapters(VecSheetOrChapter... sheetOrChapters) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter varargs is not null.
if (sheetOrChapters == null) failWithMessage("Expecting sheetOrChapters parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSheetOrChapters(), sheetOrChapters);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's sheetOrChapters contains only the given VecSheetOrChapter elements in Collection and nothing else in whatever order.
* @param sheetOrChapters the given elements that should be contained in actual VecDocumentVersion's sheetOrChapters.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's sheetOrChapters does not contain all given VecSheetOrChapter elements.
*/
public S hasOnlySheetOrChapters(java.util.Collection extends VecSheetOrChapter> sheetOrChapters) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter collection is not null.
if (sheetOrChapters == null) {
failWithMessage("Expecting sheetOrChapters parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSheetOrChapters(), sheetOrChapters.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's sheetOrChapters does not contain the given VecSheetOrChapter elements.
*
* @param sheetOrChapters the given elements that should not be in actual VecDocumentVersion's sheetOrChapters.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's sheetOrChapters contains any given VecSheetOrChapter elements.
*/
public S doesNotHaveSheetOrChapters(VecSheetOrChapter... sheetOrChapters) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter varargs is not null.
if (sheetOrChapters == null) failWithMessage("Expecting sheetOrChapters parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSheetOrChapters(), sheetOrChapters);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's sheetOrChapters does not contain the given VecSheetOrChapter elements in Collection.
*
* @param sheetOrChapters the given elements that should not be in actual VecDocumentVersion's sheetOrChapters.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's sheetOrChapters contains any given VecSheetOrChapter elements.
*/
public S doesNotHaveSheetOrChapters(java.util.Collection extends VecSheetOrChapter> sheetOrChapters) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter collection is not null.
if (sheetOrChapters == null) {
failWithMessage("Expecting sheetOrChapters parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSheetOrChapters(), sheetOrChapters.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no sheetOrChapters.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's sheetOrChapters is not empty.
*/
public S hasNoSheetOrChapters() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have sheetOrChapters but had :\n <%s>";
// check
if (actual.getSheetOrChapters().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getSheetOrChapters());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's specifications contains the given VecSpecification elements.
* @param specifications the given elements that should be contained in actual VecDocumentVersion's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's specifications does not contain all given VecSpecification elements.
*/
public S hasSpecifications(VecSpecification... specifications) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification varargs is not null.
if (specifications == null) failWithMessage("Expecting specifications parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSpecifications(), specifications);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's specifications contains the given VecSpecification elements in Collection.
* @param specifications the given elements that should be contained in actual VecDocumentVersion's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's specifications does not contain all given VecSpecification elements.
*/
public S hasSpecifications(java.util.Collection extends VecSpecification> specifications) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification collection is not null.
if (specifications == null) {
failWithMessage("Expecting specifications parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSpecifications(), specifications.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's specifications contains only the given VecSpecification elements and nothing else in whatever order.
* @param specifications the given elements that should be contained in actual VecDocumentVersion's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's specifications does not contain all given VecSpecification elements.
*/
public S hasOnlySpecifications(VecSpecification... specifications) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification varargs is not null.
if (specifications == null) failWithMessage("Expecting specifications parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSpecifications(), specifications);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's specifications contains only the given VecSpecification elements in Collection and nothing else in whatever order.
* @param specifications the given elements that should be contained in actual VecDocumentVersion's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's specifications does not contain all given VecSpecification elements.
*/
public S hasOnlySpecifications(java.util.Collection extends VecSpecification> specifications) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification collection is not null.
if (specifications == null) {
failWithMessage("Expecting specifications parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSpecifications(), specifications.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's specifications does not contain the given VecSpecification elements.
*
* @param specifications the given elements that should not be in actual VecDocumentVersion's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's specifications contains any given VecSpecification elements.
*/
public S doesNotHaveSpecifications(VecSpecification... specifications) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification varargs is not null.
if (specifications == null) failWithMessage("Expecting specifications parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSpecifications(), specifications);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion's specifications does not contain the given VecSpecification elements in Collection.
*
* @param specifications the given elements that should not be in actual VecDocumentVersion's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's specifications contains any given VecSpecification elements.
*/
public S doesNotHaveSpecifications(java.util.Collection extends VecSpecification> specifications) {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification collection is not null.
if (specifications == null) {
failWithMessage("Expecting specifications parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSpecifications(), specifications.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecDocumentVersion has no specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecDocumentVersion's specifications is not empty.
*/
public S hasNoSpecifications() {
// check that actual VecDocumentVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have specifications but had :\n <%s>";
// check
if (actual.getSpecifications().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getSpecifications());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy