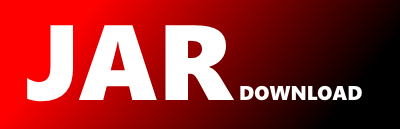
com.foursoft.vecmodel.vec120.AbstractVecEEComponentRoleAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecEEComponentRole} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecEEComponentRoleAssert, A extends VecEEComponentRole> extends AbstractVecRoleAssert {
/**
* Creates a new {@link AbstractVecEEComponentRoleAssert}
to make assertions on actual VecEEComponentRole.
* @param actual the VecEEComponentRole we want to make assertions on.
*/
protected AbstractVecEEComponentRoleAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecEEComponentRole's componentNode is equal to the given one.
* @param componentNode the given componentNode to compare the actual VecEEComponentRole's componentNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecEEComponentRole's componentNode is not equal to the given one.
*/
public S hasComponentNode(VecComponentNode componentNode) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting componentNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecComponentNode actualComponentNode = actual.getComponentNode();
if (!Objects.areEqual(actualComponentNode, componentNode)) {
failWithMessage(assertjErrorMessage, actual, componentNode, actualComponentNode);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's eEComponentSpecification is equal to the given one.
* @param eEComponentSpecification the given eEComponentSpecification to compare the actual VecEEComponentRole's eEComponentSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecEEComponentRole's eEComponentSpecification is not equal to the given one.
*/
public S hasEEComponentSpecification(VecEEComponentSpecification eEComponentSpecification) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting eEComponentSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecEEComponentSpecification actualEEComponentSpecification = actual.getEEComponentSpecification();
if (!Objects.areEqual(actualEEComponentSpecification, eEComponentSpecification)) {
failWithMessage(assertjErrorMessage, actual, eEComponentSpecification, actualEEComponentSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's extensionSlotReves contains the given VecExtensionSlotReference elements.
* @param extensionSlotReves the given elements that should be contained in actual VecEEComponentRole's extensionSlotReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's extensionSlotReves does not contain all given VecExtensionSlotReference elements.
*/
public S hasExtensionSlotReves(VecExtensionSlotReference... extensionSlotReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference varargs is not null.
if (extensionSlotReves == null) failWithMessage("Expecting extensionSlotReves parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getExtensionSlotReves(), extensionSlotReves);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's extensionSlotReves contains the given VecExtensionSlotReference elements in Collection.
* @param extensionSlotReves the given elements that should be contained in actual VecEEComponentRole's extensionSlotReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's extensionSlotReves does not contain all given VecExtensionSlotReference elements.
*/
public S hasExtensionSlotReves(java.util.Collection extends VecExtensionSlotReference> extensionSlotReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference collection is not null.
if (extensionSlotReves == null) {
failWithMessage("Expecting extensionSlotReves parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getExtensionSlotReves(), extensionSlotReves.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's extensionSlotReves contains only the given VecExtensionSlotReference elements and nothing else in whatever order.
* @param extensionSlotReves the given elements that should be contained in actual VecEEComponentRole's extensionSlotReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's extensionSlotReves does not contain all given VecExtensionSlotReference elements.
*/
public S hasOnlyExtensionSlotReves(VecExtensionSlotReference... extensionSlotReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference varargs is not null.
if (extensionSlotReves == null) failWithMessage("Expecting extensionSlotReves parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getExtensionSlotReves(), extensionSlotReves);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's extensionSlotReves contains only the given VecExtensionSlotReference elements in Collection and nothing else in whatever order.
* @param extensionSlotReves the given elements that should be contained in actual VecEEComponentRole's extensionSlotReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's extensionSlotReves does not contain all given VecExtensionSlotReference elements.
*/
public S hasOnlyExtensionSlotReves(java.util.Collection extends VecExtensionSlotReference> extensionSlotReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference collection is not null.
if (extensionSlotReves == null) {
failWithMessage("Expecting extensionSlotReves parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getExtensionSlotReves(), extensionSlotReves.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's extensionSlotReves does not contain the given VecExtensionSlotReference elements.
*
* @param extensionSlotReves the given elements that should not be in actual VecEEComponentRole's extensionSlotReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's extensionSlotReves contains any given VecExtensionSlotReference elements.
*/
public S doesNotHaveExtensionSlotReves(VecExtensionSlotReference... extensionSlotReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference varargs is not null.
if (extensionSlotReves == null) failWithMessage("Expecting extensionSlotReves parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getExtensionSlotReves(), extensionSlotReves);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's extensionSlotReves does not contain the given VecExtensionSlotReference elements in Collection.
*
* @param extensionSlotReves the given elements that should not be in actual VecEEComponentRole's extensionSlotReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's extensionSlotReves contains any given VecExtensionSlotReference elements.
*/
public S doesNotHaveExtensionSlotReves(java.util.Collection extends VecExtensionSlotReference> extensionSlotReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference collection is not null.
if (extensionSlotReves == null) {
failWithMessage("Expecting extensionSlotReves parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getExtensionSlotReves(), extensionSlotReves.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole has no extensionSlotReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's extensionSlotReves is not empty.
*/
public S hasNoExtensionSlotReves() {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have extensionSlotReves but had :\n <%s>";
// check
if (actual.getExtensionSlotReves().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getExtensionSlotReves());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's housingComponentReves contains the given VecHousingComponentReference elements.
* @param housingComponentReves the given elements that should be contained in actual VecEEComponentRole's housingComponentReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's housingComponentReves does not contain all given VecHousingComponentReference elements.
*/
public S hasHousingComponentReves(VecHousingComponentReference... housingComponentReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponentReference varargs is not null.
if (housingComponentReves == null) failWithMessage("Expecting housingComponentReves parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getHousingComponentReves(), housingComponentReves);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's housingComponentReves contains the given VecHousingComponentReference elements in Collection.
* @param housingComponentReves the given elements that should be contained in actual VecEEComponentRole's housingComponentReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's housingComponentReves does not contain all given VecHousingComponentReference elements.
*/
public S hasHousingComponentReves(java.util.Collection extends VecHousingComponentReference> housingComponentReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponentReference collection is not null.
if (housingComponentReves == null) {
failWithMessage("Expecting housingComponentReves parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getHousingComponentReves(), housingComponentReves.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's housingComponentReves contains only the given VecHousingComponentReference elements and nothing else in whatever order.
* @param housingComponentReves the given elements that should be contained in actual VecEEComponentRole's housingComponentReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's housingComponentReves does not contain all given VecHousingComponentReference elements.
*/
public S hasOnlyHousingComponentReves(VecHousingComponentReference... housingComponentReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponentReference varargs is not null.
if (housingComponentReves == null) failWithMessage("Expecting housingComponentReves parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getHousingComponentReves(), housingComponentReves);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's housingComponentReves contains only the given VecHousingComponentReference elements in Collection and nothing else in whatever order.
* @param housingComponentReves the given elements that should be contained in actual VecEEComponentRole's housingComponentReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's housingComponentReves does not contain all given VecHousingComponentReference elements.
*/
public S hasOnlyHousingComponentReves(java.util.Collection extends VecHousingComponentReference> housingComponentReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponentReference collection is not null.
if (housingComponentReves == null) {
failWithMessage("Expecting housingComponentReves parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getHousingComponentReves(), housingComponentReves.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's housingComponentReves does not contain the given VecHousingComponentReference elements.
*
* @param housingComponentReves the given elements that should not be in actual VecEEComponentRole's housingComponentReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's housingComponentReves contains any given VecHousingComponentReference elements.
*/
public S doesNotHaveHousingComponentReves(VecHousingComponentReference... housingComponentReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponentReference varargs is not null.
if (housingComponentReves == null) failWithMessage("Expecting housingComponentReves parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getHousingComponentReves(), housingComponentReves);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's housingComponentReves does not contain the given VecHousingComponentReference elements in Collection.
*
* @param housingComponentReves the given elements that should not be in actual VecEEComponentRole's housingComponentReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's housingComponentReves contains any given VecHousingComponentReference elements.
*/
public S doesNotHaveHousingComponentReves(java.util.Collection extends VecHousingComponentReference> housingComponentReves) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponentReference collection is not null.
if (housingComponentReves == null) {
failWithMessage("Expecting housingComponentReves parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getHousingComponentReves(), housingComponentReves.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole has no housingComponentReves.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's housingComponentReves is not empty.
*/
public S hasNoHousingComponentReves() {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have housingComponentReves but had :\n <%s>";
// check
if (actual.getHousingComponentReves().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getHousingComponentReves());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's refExtensionSlotReference contains the given VecExtensionSlotReference elements.
* @param refExtensionSlotReference the given elements that should be contained in actual VecEEComponentRole's refExtensionSlotReference.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's refExtensionSlotReference does not contain all given VecExtensionSlotReference elements.
*/
public S hasRefExtensionSlotReference(VecExtensionSlotReference... refExtensionSlotReference) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference varargs is not null.
if (refExtensionSlotReference == null) failWithMessage("Expecting refExtensionSlotReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefExtensionSlotReference(), refExtensionSlotReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's refExtensionSlotReference contains the given VecExtensionSlotReference elements in Collection.
* @param refExtensionSlotReference the given elements that should be contained in actual VecEEComponentRole's refExtensionSlotReference.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's refExtensionSlotReference does not contain all given VecExtensionSlotReference elements.
*/
public S hasRefExtensionSlotReference(java.util.Collection extends VecExtensionSlotReference> refExtensionSlotReference) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference collection is not null.
if (refExtensionSlotReference == null) {
failWithMessage("Expecting refExtensionSlotReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefExtensionSlotReference(), refExtensionSlotReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's refExtensionSlotReference contains only the given VecExtensionSlotReference elements and nothing else in whatever order.
* @param refExtensionSlotReference the given elements that should be contained in actual VecEEComponentRole's refExtensionSlotReference.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's refExtensionSlotReference does not contain all given VecExtensionSlotReference elements.
*/
public S hasOnlyRefExtensionSlotReference(VecExtensionSlotReference... refExtensionSlotReference) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference varargs is not null.
if (refExtensionSlotReference == null) failWithMessage("Expecting refExtensionSlotReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefExtensionSlotReference(), refExtensionSlotReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's refExtensionSlotReference contains only the given VecExtensionSlotReference elements in Collection and nothing else in whatever order.
* @param refExtensionSlotReference the given elements that should be contained in actual VecEEComponentRole's refExtensionSlotReference.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's refExtensionSlotReference does not contain all given VecExtensionSlotReference elements.
*/
public S hasOnlyRefExtensionSlotReference(java.util.Collection extends VecExtensionSlotReference> refExtensionSlotReference) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference collection is not null.
if (refExtensionSlotReference == null) {
failWithMessage("Expecting refExtensionSlotReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefExtensionSlotReference(), refExtensionSlotReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's refExtensionSlotReference does not contain the given VecExtensionSlotReference elements.
*
* @param refExtensionSlotReference the given elements that should not be in actual VecEEComponentRole's refExtensionSlotReference.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's refExtensionSlotReference contains any given VecExtensionSlotReference elements.
*/
public S doesNotHaveRefExtensionSlotReference(VecExtensionSlotReference... refExtensionSlotReference) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference varargs is not null.
if (refExtensionSlotReference == null) failWithMessage("Expecting refExtensionSlotReference parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefExtensionSlotReference(), refExtensionSlotReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole's refExtensionSlotReference does not contain the given VecExtensionSlotReference elements in Collection.
*
* @param refExtensionSlotReference the given elements that should not be in actual VecEEComponentRole's refExtensionSlotReference.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's refExtensionSlotReference contains any given VecExtensionSlotReference elements.
*/
public S doesNotHaveRefExtensionSlotReference(java.util.Collection extends VecExtensionSlotReference> refExtensionSlotReference) {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlotReference collection is not null.
if (refExtensionSlotReference == null) {
failWithMessage("Expecting refExtensionSlotReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefExtensionSlotReference(), refExtensionSlotReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentRole has no refExtensionSlotReference.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentRole's refExtensionSlotReference is not empty.
*/
public S hasNoRefExtensionSlotReference() {
// check that actual VecEEComponentRole we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refExtensionSlotReference but had :\n <%s>";
// check
if (actual.getRefExtensionSlotReference().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefExtensionSlotReference());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy