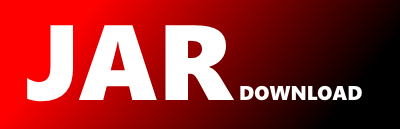
com.foursoft.vecmodel.vec120.AbstractVecEEComponentSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
/**
* Abstract base class for {@link VecEEComponentSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecEEComponentSpecificationAssert, A extends VecEEComponentSpecification> extends AbstractVecPartOrUsageRelatedSpecificationAssert {
/**
* Creates a new {@link AbstractVecEEComponentSpecificationAssert}
to make assertions on actual VecEEComponentSpecification.
* @param actual the VecEEComponentSpecification we want to make assertions on.
*/
protected AbstractVecEEComponentSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecEEComponentSpecification's connections contains the given VecInternalComponentConnection elements.
* @param connections the given elements that should be contained in actual VecEEComponentSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's connections does not contain all given VecInternalComponentConnection elements.
*/
public S hasConnections(VecInternalComponentConnection... connections) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (connections == null) failWithMessage("Expecting connections parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnections(), connections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's connections contains the given VecInternalComponentConnection elements in Collection.
* @param connections the given elements that should be contained in actual VecEEComponentSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's connections does not contain all given VecInternalComponentConnection elements.
*/
public S hasConnections(java.util.Collection extends VecInternalComponentConnection> connections) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (connections == null) {
failWithMessage("Expecting connections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getConnections(), connections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's connections contains only the given VecInternalComponentConnection elements and nothing else in whatever order.
* @param connections the given elements that should be contained in actual VecEEComponentSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's connections does not contain all given VecInternalComponentConnection elements.
*/
public S hasOnlyConnections(VecInternalComponentConnection... connections) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (connections == null) failWithMessage("Expecting connections parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnections(), connections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's connections contains only the given VecInternalComponentConnection elements in Collection and nothing else in whatever order.
* @param connections the given elements that should be contained in actual VecEEComponentSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's connections does not contain all given VecInternalComponentConnection elements.
*/
public S hasOnlyConnections(java.util.Collection extends VecInternalComponentConnection> connections) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (connections == null) {
failWithMessage("Expecting connections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getConnections(), connections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's connections does not contain the given VecInternalComponentConnection elements.
*
* @param connections the given elements that should not be in actual VecEEComponentSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's connections contains any given VecInternalComponentConnection elements.
*/
public S doesNotHaveConnections(VecInternalComponentConnection... connections) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (connections == null) failWithMessage("Expecting connections parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnections(), connections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's connections does not contain the given VecInternalComponentConnection elements in Collection.
*
* @param connections the given elements that should not be in actual VecEEComponentSpecification's connections.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's connections contains any given VecInternalComponentConnection elements.
*/
public S doesNotHaveConnections(java.util.Collection extends VecInternalComponentConnection> connections) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (connections == null) {
failWithMessage("Expecting connections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getConnections(), connections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification has no connections.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's connections is not empty.
*/
public S hasNoConnections() {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have connections but had :\n <%s>";
// check
if (actual.getConnections().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getConnections());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's extensionSlots contains the given VecExtensionSlot elements.
* @param extensionSlots the given elements that should be contained in actual VecEEComponentSpecification's extensionSlots.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's extensionSlots does not contain all given VecExtensionSlot elements.
*/
public S hasExtensionSlots(VecExtensionSlot... extensionSlots) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlot varargs is not null.
if (extensionSlots == null) failWithMessage("Expecting extensionSlots parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getExtensionSlots(), extensionSlots);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's extensionSlots contains the given VecExtensionSlot elements in Collection.
* @param extensionSlots the given elements that should be contained in actual VecEEComponentSpecification's extensionSlots.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's extensionSlots does not contain all given VecExtensionSlot elements.
*/
public S hasExtensionSlots(java.util.Collection extends VecExtensionSlot> extensionSlots) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlot collection is not null.
if (extensionSlots == null) {
failWithMessage("Expecting extensionSlots parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getExtensionSlots(), extensionSlots.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's extensionSlots contains only the given VecExtensionSlot elements and nothing else in whatever order.
* @param extensionSlots the given elements that should be contained in actual VecEEComponentSpecification's extensionSlots.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's extensionSlots does not contain all given VecExtensionSlot elements.
*/
public S hasOnlyExtensionSlots(VecExtensionSlot... extensionSlots) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlot varargs is not null.
if (extensionSlots == null) failWithMessage("Expecting extensionSlots parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getExtensionSlots(), extensionSlots);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's extensionSlots contains only the given VecExtensionSlot elements in Collection and nothing else in whatever order.
* @param extensionSlots the given elements that should be contained in actual VecEEComponentSpecification's extensionSlots.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's extensionSlots does not contain all given VecExtensionSlot elements.
*/
public S hasOnlyExtensionSlots(java.util.Collection extends VecExtensionSlot> extensionSlots) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlot collection is not null.
if (extensionSlots == null) {
failWithMessage("Expecting extensionSlots parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getExtensionSlots(), extensionSlots.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's extensionSlots does not contain the given VecExtensionSlot elements.
*
* @param extensionSlots the given elements that should not be in actual VecEEComponentSpecification's extensionSlots.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's extensionSlots contains any given VecExtensionSlot elements.
*/
public S doesNotHaveExtensionSlots(VecExtensionSlot... extensionSlots) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlot varargs is not null.
if (extensionSlots == null) failWithMessage("Expecting extensionSlots parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getExtensionSlots(), extensionSlots);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's extensionSlots does not contain the given VecExtensionSlot elements in Collection.
*
* @param extensionSlots the given elements that should not be in actual VecEEComponentSpecification's extensionSlots.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's extensionSlots contains any given VecExtensionSlot elements.
*/
public S doesNotHaveExtensionSlots(java.util.Collection extends VecExtensionSlot> extensionSlots) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecExtensionSlot collection is not null.
if (extensionSlots == null) {
failWithMessage("Expecting extensionSlots parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getExtensionSlots(), extensionSlots.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification has no extensionSlots.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's extensionSlots is not empty.
*/
public S hasNoExtensionSlots() {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have extensionSlots but had :\n <%s>";
// check
if (actual.getExtensionSlots().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getExtensionSlots());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's housingComponents contains the given VecHousingComponent elements.
* @param housingComponents the given elements that should be contained in actual VecEEComponentSpecification's housingComponents.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's housingComponents does not contain all given VecHousingComponent elements.
*/
public S hasHousingComponents(VecHousingComponent... housingComponents) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponent varargs is not null.
if (housingComponents == null) failWithMessage("Expecting housingComponents parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getHousingComponents(), housingComponents);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's housingComponents contains the given VecHousingComponent elements in Collection.
* @param housingComponents the given elements that should be contained in actual VecEEComponentSpecification's housingComponents.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's housingComponents does not contain all given VecHousingComponent elements.
*/
public S hasHousingComponents(java.util.Collection extends VecHousingComponent> housingComponents) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponent collection is not null.
if (housingComponents == null) {
failWithMessage("Expecting housingComponents parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getHousingComponents(), housingComponents.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's housingComponents contains only the given VecHousingComponent elements and nothing else in whatever order.
* @param housingComponents the given elements that should be contained in actual VecEEComponentSpecification's housingComponents.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's housingComponents does not contain all given VecHousingComponent elements.
*/
public S hasOnlyHousingComponents(VecHousingComponent... housingComponents) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponent varargs is not null.
if (housingComponents == null) failWithMessage("Expecting housingComponents parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getHousingComponents(), housingComponents);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's housingComponents contains only the given VecHousingComponent elements in Collection and nothing else in whatever order.
* @param housingComponents the given elements that should be contained in actual VecEEComponentSpecification's housingComponents.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's housingComponents does not contain all given VecHousingComponent elements.
*/
public S hasOnlyHousingComponents(java.util.Collection extends VecHousingComponent> housingComponents) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponent collection is not null.
if (housingComponents == null) {
failWithMessage("Expecting housingComponents parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getHousingComponents(), housingComponents.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's housingComponents does not contain the given VecHousingComponent elements.
*
* @param housingComponents the given elements that should not be in actual VecEEComponentSpecification's housingComponents.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's housingComponents contains any given VecHousingComponent elements.
*/
public S doesNotHaveHousingComponents(VecHousingComponent... housingComponents) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponent varargs is not null.
if (housingComponents == null) failWithMessage("Expecting housingComponents parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getHousingComponents(), housingComponents);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's housingComponents does not contain the given VecHousingComponent elements in Collection.
*
* @param housingComponents the given elements that should not be in actual VecEEComponentSpecification's housingComponents.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's housingComponents contains any given VecHousingComponent elements.
*/
public S doesNotHaveHousingComponents(java.util.Collection extends VecHousingComponent> housingComponents) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecHousingComponent collection is not null.
if (housingComponents == null) {
failWithMessage("Expecting housingComponents parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getHousingComponents(), housingComponents.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification has no housingComponents.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's housingComponents is not empty.
*/
public S hasNoHousingComponents() {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have housingComponents but had :\n <%s>";
// check
if (actual.getHousingComponents().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getHousingComponents());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's powerConsumptions contains the given VecPowerConsumption elements.
* @param powerConsumptions the given elements that should be contained in actual VecEEComponentSpecification's powerConsumptions.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's powerConsumptions does not contain all given VecPowerConsumption elements.
*/
public S hasPowerConsumptions(VecPowerConsumption... powerConsumptions) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPowerConsumption varargs is not null.
if (powerConsumptions == null) failWithMessage("Expecting powerConsumptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPowerConsumptions(), powerConsumptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's powerConsumptions contains the given VecPowerConsumption elements in Collection.
* @param powerConsumptions the given elements that should be contained in actual VecEEComponentSpecification's powerConsumptions.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's powerConsumptions does not contain all given VecPowerConsumption elements.
*/
public S hasPowerConsumptions(java.util.Collection extends VecPowerConsumption> powerConsumptions) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPowerConsumption collection is not null.
if (powerConsumptions == null) {
failWithMessage("Expecting powerConsumptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPowerConsumptions(), powerConsumptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's powerConsumptions contains only the given VecPowerConsumption elements and nothing else in whatever order.
* @param powerConsumptions the given elements that should be contained in actual VecEEComponentSpecification's powerConsumptions.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's powerConsumptions does not contain all given VecPowerConsumption elements.
*/
public S hasOnlyPowerConsumptions(VecPowerConsumption... powerConsumptions) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPowerConsumption varargs is not null.
if (powerConsumptions == null) failWithMessage("Expecting powerConsumptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPowerConsumptions(), powerConsumptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's powerConsumptions contains only the given VecPowerConsumption elements in Collection and nothing else in whatever order.
* @param powerConsumptions the given elements that should be contained in actual VecEEComponentSpecification's powerConsumptions.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's powerConsumptions does not contain all given VecPowerConsumption elements.
*/
public S hasOnlyPowerConsumptions(java.util.Collection extends VecPowerConsumption> powerConsumptions) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPowerConsumption collection is not null.
if (powerConsumptions == null) {
failWithMessage("Expecting powerConsumptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPowerConsumptions(), powerConsumptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's powerConsumptions does not contain the given VecPowerConsumption elements.
*
* @param powerConsumptions the given elements that should not be in actual VecEEComponentSpecification's powerConsumptions.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's powerConsumptions contains any given VecPowerConsumption elements.
*/
public S doesNotHavePowerConsumptions(VecPowerConsumption... powerConsumptions) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPowerConsumption varargs is not null.
if (powerConsumptions == null) failWithMessage("Expecting powerConsumptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPowerConsumptions(), powerConsumptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's powerConsumptions does not contain the given VecPowerConsumption elements in Collection.
*
* @param powerConsumptions the given elements that should not be in actual VecEEComponentSpecification's powerConsumptions.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's powerConsumptions contains any given VecPowerConsumption elements.
*/
public S doesNotHavePowerConsumptions(java.util.Collection extends VecPowerConsumption> powerConsumptions) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPowerConsumption collection is not null.
if (powerConsumptions == null) {
failWithMessage("Expecting powerConsumptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPowerConsumptions(), powerConsumptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification has no powerConsumptions.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's powerConsumptions is not empty.
*/
public S hasNoPowerConsumptions() {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have powerConsumptions but had :\n <%s>";
// check
if (actual.getPowerConsumptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPowerConsumptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's refEEComponentRole contains the given VecEEComponentRole elements.
* @param refEEComponentRole the given elements that should be contained in actual VecEEComponentSpecification's refEEComponentRole.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's refEEComponentRole does not contain all given VecEEComponentRole elements.
*/
public S hasRefEEComponentRole(VecEEComponentRole... refEEComponentRole) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole varargs is not null.
if (refEEComponentRole == null) failWithMessage("Expecting refEEComponentRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefEEComponentRole(), refEEComponentRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's refEEComponentRole contains the given VecEEComponentRole elements in Collection.
* @param refEEComponentRole the given elements that should be contained in actual VecEEComponentSpecification's refEEComponentRole.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's refEEComponentRole does not contain all given VecEEComponentRole elements.
*/
public S hasRefEEComponentRole(java.util.Collection extends VecEEComponentRole> refEEComponentRole) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole collection is not null.
if (refEEComponentRole == null) {
failWithMessage("Expecting refEEComponentRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefEEComponentRole(), refEEComponentRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's refEEComponentRole contains only the given VecEEComponentRole elements and nothing else in whatever order.
* @param refEEComponentRole the given elements that should be contained in actual VecEEComponentSpecification's refEEComponentRole.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's refEEComponentRole does not contain all given VecEEComponentRole elements.
*/
public S hasOnlyRefEEComponentRole(VecEEComponentRole... refEEComponentRole) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole varargs is not null.
if (refEEComponentRole == null) failWithMessage("Expecting refEEComponentRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefEEComponentRole(), refEEComponentRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's refEEComponentRole contains only the given VecEEComponentRole elements in Collection and nothing else in whatever order.
* @param refEEComponentRole the given elements that should be contained in actual VecEEComponentSpecification's refEEComponentRole.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's refEEComponentRole does not contain all given VecEEComponentRole elements.
*/
public S hasOnlyRefEEComponentRole(java.util.Collection extends VecEEComponentRole> refEEComponentRole) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole collection is not null.
if (refEEComponentRole == null) {
failWithMessage("Expecting refEEComponentRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefEEComponentRole(), refEEComponentRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's refEEComponentRole does not contain the given VecEEComponentRole elements.
*
* @param refEEComponentRole the given elements that should not be in actual VecEEComponentSpecification's refEEComponentRole.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's refEEComponentRole contains any given VecEEComponentRole elements.
*/
public S doesNotHaveRefEEComponentRole(VecEEComponentRole... refEEComponentRole) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole varargs is not null.
if (refEEComponentRole == null) failWithMessage("Expecting refEEComponentRole parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefEEComponentRole(), refEEComponentRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's refEEComponentRole does not contain the given VecEEComponentRole elements in Collection.
*
* @param refEEComponentRole the given elements that should not be in actual VecEEComponentSpecification's refEEComponentRole.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's refEEComponentRole contains any given VecEEComponentRole elements.
*/
public S doesNotHaveRefEEComponentRole(java.util.Collection extends VecEEComponentRole> refEEComponentRole) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole collection is not null.
if (refEEComponentRole == null) {
failWithMessage("Expecting refEEComponentRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefEEComponentRole(), refEEComponentRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification has no refEEComponentRole.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's refEEComponentRole is not empty.
*/
public S hasNoRefEEComponentRole() {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refEEComponentRole but had :\n <%s>";
// check
if (actual.getRefEEComponentRole().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefEEComponentRole());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's states contains the given VecSwitchingState elements.
* @param states the given elements that should be contained in actual VecEEComponentSpecification's states.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's states does not contain all given VecSwitchingState elements.
*/
public S hasStates(VecSwitchingState... states) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSwitchingState varargs is not null.
if (states == null) failWithMessage("Expecting states parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getStates(), states);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's states contains the given VecSwitchingState elements in Collection.
* @param states the given elements that should be contained in actual VecEEComponentSpecification's states.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's states does not contain all given VecSwitchingState elements.
*/
public S hasStates(java.util.Collection extends VecSwitchingState> states) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSwitchingState collection is not null.
if (states == null) {
failWithMessage("Expecting states parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getStates(), states.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's states contains only the given VecSwitchingState elements and nothing else in whatever order.
* @param states the given elements that should be contained in actual VecEEComponentSpecification's states.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's states does not contain all given VecSwitchingState elements.
*/
public S hasOnlyStates(VecSwitchingState... states) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSwitchingState varargs is not null.
if (states == null) failWithMessage("Expecting states parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getStates(), states);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's states contains only the given VecSwitchingState elements in Collection and nothing else in whatever order.
* @param states the given elements that should be contained in actual VecEEComponentSpecification's states.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's states does not contain all given VecSwitchingState elements.
*/
public S hasOnlyStates(java.util.Collection extends VecSwitchingState> states) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSwitchingState collection is not null.
if (states == null) {
failWithMessage("Expecting states parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getStates(), states.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's states does not contain the given VecSwitchingState elements.
*
* @param states the given elements that should not be in actual VecEEComponentSpecification's states.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's states contains any given VecSwitchingState elements.
*/
public S doesNotHaveStates(VecSwitchingState... states) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSwitchingState varargs is not null.
if (states == null) failWithMessage("Expecting states parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getStates(), states);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification's states does not contain the given VecSwitchingState elements in Collection.
*
* @param states the given elements that should not be in actual VecEEComponentSpecification's states.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's states contains any given VecSwitchingState elements.
*/
public S doesNotHaveStates(java.util.Collection extends VecSwitchingState> states) {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSwitchingState collection is not null.
if (states == null) {
failWithMessage("Expecting states parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getStates(), states.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecEEComponentSpecification has no states.
* @return this assertion object.
* @throws AssertionError if the actual VecEEComponentSpecification's states is not empty.
*/
public S hasNoStates() {
// check that actual VecEEComponentSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have states but had :\n <%s>";
// check
if (actual.getStates().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getStates());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy