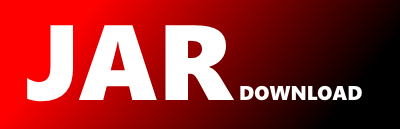
com.foursoft.vecmodel.vec120.AbstractVecExtensionSlotReferenceAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecExtensionSlotReference} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecExtensionSlotReferenceAssert, A extends VecExtensionSlotReference> extends AbstractVecConfigurableElementAssert {
/**
* Creates a new {@link AbstractVecExtensionSlotReferenceAssert}
to make assertions on actual VecExtensionSlotReference.
* @param actual the VecExtensionSlotReference we want to make assertions on.
*/
protected AbstractVecExtensionSlotReferenceAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecExtensionSlotReference's extensionSlot is equal to the given one.
* @param extensionSlot the given extensionSlot to compare the actual VecExtensionSlotReference's extensionSlot to.
* @return this assertion object.
* @throws AssertionError - if the actual VecExtensionSlotReference's extensionSlot is not equal to the given one.
*/
public S hasExtensionSlot(VecExtensionSlot extensionSlot) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting extensionSlot of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecExtensionSlot actualExtensionSlot = actual.getExtensionSlot();
if (!Objects.areEqual(actualExtensionSlot, extensionSlot)) {
failWithMessage(assertjErrorMessage, actual, extensionSlot, actualExtensionSlot);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's identification is equal to the given one.
* @param identification the given identification to compare the actual VecExtensionSlotReference's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecExtensionSlotReference's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's parentEEComponentRole is equal to the given one.
* @param parentEEComponentRole the given parentEEComponentRole to compare the actual VecExtensionSlotReference's parentEEComponentRole to.
* @return this assertion object.
* @throws AssertionError - if the actual VecExtensionSlotReference's parentEEComponentRole is not equal to the given one.
*/
public S hasParentEEComponentRole(VecEEComponentRole parentEEComponentRole) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentEEComponentRole of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecEEComponentRole actualParentEEComponentRole = actual.getParentEEComponentRole();
if (!Objects.areEqual(actualParentEEComponentRole, parentEEComponentRole)) {
failWithMessage(assertjErrorMessage, actual, parentEEComponentRole, actualParentEEComponentRole);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's usedInserts contains the given VecEEComponentRole elements.
* @param usedInserts the given elements that should be contained in actual VecExtensionSlotReference's usedInserts.
* @return this assertion object.
* @throws AssertionError if the actual VecExtensionSlotReference's usedInserts does not contain all given VecEEComponentRole elements.
*/
public S hasUsedInserts(VecEEComponentRole... usedInserts) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole varargs is not null.
if (usedInserts == null) failWithMessage("Expecting usedInserts parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedInserts(), usedInserts);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's usedInserts contains the given VecEEComponentRole elements in Collection.
* @param usedInserts the given elements that should be contained in actual VecExtensionSlotReference's usedInserts.
* @return this assertion object.
* @throws AssertionError if the actual VecExtensionSlotReference's usedInserts does not contain all given VecEEComponentRole elements.
*/
public S hasUsedInserts(java.util.Collection extends VecEEComponentRole> usedInserts) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole collection is not null.
if (usedInserts == null) {
failWithMessage("Expecting usedInserts parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedInserts(), usedInserts.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's usedInserts contains only the given VecEEComponentRole elements and nothing else in whatever order.
* @param usedInserts the given elements that should be contained in actual VecExtensionSlotReference's usedInserts.
* @return this assertion object.
* @throws AssertionError if the actual VecExtensionSlotReference's usedInserts does not contain all given VecEEComponentRole elements.
*/
public S hasOnlyUsedInserts(VecEEComponentRole... usedInserts) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole varargs is not null.
if (usedInserts == null) failWithMessage("Expecting usedInserts parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedInserts(), usedInserts);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's usedInserts contains only the given VecEEComponentRole elements in Collection and nothing else in whatever order.
* @param usedInserts the given elements that should be contained in actual VecExtensionSlotReference's usedInserts.
* @return this assertion object.
* @throws AssertionError if the actual VecExtensionSlotReference's usedInserts does not contain all given VecEEComponentRole elements.
*/
public S hasOnlyUsedInserts(java.util.Collection extends VecEEComponentRole> usedInserts) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole collection is not null.
if (usedInserts == null) {
failWithMessage("Expecting usedInserts parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedInserts(), usedInserts.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's usedInserts does not contain the given VecEEComponentRole elements.
*
* @param usedInserts the given elements that should not be in actual VecExtensionSlotReference's usedInserts.
* @return this assertion object.
* @throws AssertionError if the actual VecExtensionSlotReference's usedInserts contains any given VecEEComponentRole elements.
*/
public S doesNotHaveUsedInserts(VecEEComponentRole... usedInserts) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole varargs is not null.
if (usedInserts == null) failWithMessage("Expecting usedInserts parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedInserts(), usedInserts);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference's usedInserts does not contain the given VecEEComponentRole elements in Collection.
*
* @param usedInserts the given elements that should not be in actual VecExtensionSlotReference's usedInserts.
* @return this assertion object.
* @throws AssertionError if the actual VecExtensionSlotReference's usedInserts contains any given VecEEComponentRole elements.
*/
public S doesNotHaveUsedInserts(java.util.Collection extends VecEEComponentRole> usedInserts) {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// check that given VecEEComponentRole collection is not null.
if (usedInserts == null) {
failWithMessage("Expecting usedInserts parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedInserts(), usedInserts.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecExtensionSlotReference has no usedInserts.
* @return this assertion object.
* @throws AssertionError if the actual VecExtensionSlotReference's usedInserts is not empty.
*/
public S hasNoUsedInserts() {
// check that actual VecExtensionSlotReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have usedInserts but had :\n <%s>";
// check
if (actual.getUsedInserts().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getUsedInserts());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy