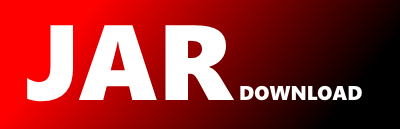
com.foursoft.vecmodel.vec120.AbstractVecGeneralTechnicalPartSpecificationAssert Maven / Gradle / Ivy
Show all versions of vec120-assertions Show documentation
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.Assertions;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecGeneralTechnicalPartSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecGeneralTechnicalPartSpecificationAssert, A extends VecGeneralTechnicalPartSpecification> extends AbstractVecPartOrUsageRelatedSpecificationAssert {
/**
* Creates a new {@link AbstractVecGeneralTechnicalPartSpecificationAssert}
to make assertions on actual VecGeneralTechnicalPartSpecification.
* @param actual the VecGeneralTechnicalPartSpecification we want to make assertions on.
*/
protected AbstractVecGeneralTechnicalPartSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's boundingBox is equal to the given one.
* @param boundingBox the given boundingBox to compare the actual VecGeneralTechnicalPartSpecification's boundingBox to.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeneralTechnicalPartSpecification's boundingBox is not equal to the given one.
*/
public S hasBoundingBox(VecBoundingBox boundingBox) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting boundingBox of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecBoundingBox actualBoundingBox = actual.getBoundingBox();
if (!Objects.areEqual(actualBoundingBox, boundingBox)) {
failWithMessage(assertjErrorMessage, actual, boundingBox, actualBoundingBox);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's colorInformations contains the given VecColor elements.
* @param colorInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's colorInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's colorInformations does not contain all given VecColor elements.
*/
public S hasColorInformations(VecColor... colorInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecColor varargs is not null.
if (colorInformations == null) failWithMessage("Expecting colorInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getColorInformations(), colorInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's colorInformations contains the given VecColor elements in Collection.
* @param colorInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's colorInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's colorInformations does not contain all given VecColor elements.
*/
public S hasColorInformations(java.util.Collection extends VecColor> colorInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecColor collection is not null.
if (colorInformations == null) {
failWithMessage("Expecting colorInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getColorInformations(), colorInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's colorInformations contains only the given VecColor elements and nothing else in whatever order.
* @param colorInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's colorInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's colorInformations does not contain all given VecColor elements.
*/
public S hasOnlyColorInformations(VecColor... colorInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecColor varargs is not null.
if (colorInformations == null) failWithMessage("Expecting colorInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getColorInformations(), colorInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's colorInformations contains only the given VecColor elements in Collection and nothing else in whatever order.
* @param colorInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's colorInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's colorInformations does not contain all given VecColor elements.
*/
public S hasOnlyColorInformations(java.util.Collection extends VecColor> colorInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecColor collection is not null.
if (colorInformations == null) {
failWithMessage("Expecting colorInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getColorInformations(), colorInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's colorInformations does not contain the given VecColor elements.
*
* @param colorInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's colorInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's colorInformations contains any given VecColor elements.
*/
public S doesNotHaveColorInformations(VecColor... colorInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecColor varargs is not null.
if (colorInformations == null) failWithMessage("Expecting colorInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getColorInformations(), colorInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's colorInformations does not contain the given VecColor elements in Collection.
*
* @param colorInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's colorInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's colorInformations contains any given VecColor elements.
*/
public S doesNotHaveColorInformations(java.util.Collection extends VecColor> colorInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecColor collection is not null.
if (colorInformations == null) {
failWithMessage("Expecting colorInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getColorInformations(), colorInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification has no colorInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's colorInformations is not empty.
*/
public S hasNoColorInformations() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have colorInformations but had :\n <%s>";
// check
if (actual.getColorInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getColorInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's fitRate is equal to the given one.
* @param fitRate the given fitRate to compare the actual VecGeneralTechnicalPartSpecification's fitRate to.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeneralTechnicalPartSpecification's fitRate is not equal to the given one.
*/
public S hasFitRate(Double fitRate) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting fitRate of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Double actualFitRate = actual.getFitRate();
if (!Objects.areEqual(actualFitRate, fitRate)) {
failWithMessage(assertjErrorMessage, actual, fitRate, actualFitRate);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's fitRate is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param fitRate the value to compare the actual VecGeneralTechnicalPartSpecification's fitRate to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeneralTechnicalPartSpecification's fitRate is not close enough to the given value.
*/
public S hasFitRateCloseTo(Double fitRate, Double assertjOffset) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
Double actualFitRate = actual.getFitRate();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting fitRate:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualFitRate, fitRate, assertjOffset, Math.abs(fitRate - actualFitRate));
// check
Assertions.assertThat(actualFitRate).overridingErrorMessage(assertjErrorMessage).isCloseTo(fitRate, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's massInformations contains the given VecMassInformation elements.
* @param massInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's massInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's massInformations does not contain all given VecMassInformation elements.
*/
public S hasMassInformations(VecMassInformation... massInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMassInformation varargs is not null.
if (massInformations == null) failWithMessage("Expecting massInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMassInformations(), massInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's massInformations contains the given VecMassInformation elements in Collection.
* @param massInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's massInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's massInformations does not contain all given VecMassInformation elements.
*/
public S hasMassInformations(java.util.Collection extends VecMassInformation> massInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMassInformation collection is not null.
if (massInformations == null) {
failWithMessage("Expecting massInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMassInformations(), massInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's massInformations contains only the given VecMassInformation elements and nothing else in whatever order.
* @param massInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's massInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's massInformations does not contain all given VecMassInformation elements.
*/
public S hasOnlyMassInformations(VecMassInformation... massInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMassInformation varargs is not null.
if (massInformations == null) failWithMessage("Expecting massInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMassInformations(), massInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's massInformations contains only the given VecMassInformation elements in Collection and nothing else in whatever order.
* @param massInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's massInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's massInformations does not contain all given VecMassInformation elements.
*/
public S hasOnlyMassInformations(java.util.Collection extends VecMassInformation> massInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMassInformation collection is not null.
if (massInformations == null) {
failWithMessage("Expecting massInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMassInformations(), massInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's massInformations does not contain the given VecMassInformation elements.
*
* @param massInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's massInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's massInformations contains any given VecMassInformation elements.
*/
public S doesNotHaveMassInformations(VecMassInformation... massInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMassInformation varargs is not null.
if (massInformations == null) failWithMessage("Expecting massInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMassInformations(), massInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's massInformations does not contain the given VecMassInformation elements in Collection.
*
* @param massInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's massInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's massInformations contains any given VecMassInformation elements.
*/
public S doesNotHaveMassInformations(java.util.Collection extends VecMassInformation> massInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMassInformation collection is not null.
if (massInformations == null) {
failWithMessage("Expecting massInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMassInformations(), massInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification has no massInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's massInformations is not empty.
*/
public S hasNoMassInformations() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have massInformations but had :\n <%s>";
// check
if (actual.getMassInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getMassInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's materialInformations contains the given VecMaterial elements.
* @param materialInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's materialInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's materialInformations does not contain all given VecMaterial elements.
*/
public S hasMaterialInformations(VecMaterial... materialInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (materialInformations == null) failWithMessage("Expecting materialInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMaterialInformations(), materialInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's materialInformations contains the given VecMaterial elements in Collection.
* @param materialInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's materialInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's materialInformations does not contain all given VecMaterial elements.
*/
public S hasMaterialInformations(java.util.Collection extends VecMaterial> materialInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (materialInformations == null) {
failWithMessage("Expecting materialInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMaterialInformations(), materialInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's materialInformations contains only the given VecMaterial elements and nothing else in whatever order.
* @param materialInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's materialInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's materialInformations does not contain all given VecMaterial elements.
*/
public S hasOnlyMaterialInformations(VecMaterial... materialInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (materialInformations == null) failWithMessage("Expecting materialInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMaterialInformations(), materialInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's materialInformations contains only the given VecMaterial elements in Collection and nothing else in whatever order.
* @param materialInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's materialInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's materialInformations does not contain all given VecMaterial elements.
*/
public S hasOnlyMaterialInformations(java.util.Collection extends VecMaterial> materialInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (materialInformations == null) {
failWithMessage("Expecting materialInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMaterialInformations(), materialInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's materialInformations does not contain the given VecMaterial elements.
*
* @param materialInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's materialInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's materialInformations contains any given VecMaterial elements.
*/
public S doesNotHaveMaterialInformations(VecMaterial... materialInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (materialInformations == null) failWithMessage("Expecting materialInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMaterialInformations(), materialInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's materialInformations does not contain the given VecMaterial elements in Collection.
*
* @param materialInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's materialInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's materialInformations contains any given VecMaterial elements.
*/
public S doesNotHaveMaterialInformations(java.util.Collection extends VecMaterial> materialInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (materialInformations == null) {
failWithMessage("Expecting materialInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMaterialInformations(), materialInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification has no materialInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's materialInformations is not empty.
*/
public S hasNoMaterialInformations() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have materialInformations but had :\n <%s>";
// check
if (actual.getMaterialInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getMaterialInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's partRelations contains the given VecPartRelation elements.
* @param partRelations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's partRelations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's partRelations does not contain all given VecPartRelation elements.
*/
public S hasPartRelations(VecPartRelation... partRelations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation varargs is not null.
if (partRelations == null) failWithMessage("Expecting partRelations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPartRelations(), partRelations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's partRelations contains the given VecPartRelation elements in Collection.
* @param partRelations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's partRelations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's partRelations does not contain all given VecPartRelation elements.
*/
public S hasPartRelations(java.util.Collection extends VecPartRelation> partRelations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation collection is not null.
if (partRelations == null) {
failWithMessage("Expecting partRelations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPartRelations(), partRelations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's partRelations contains only the given VecPartRelation elements and nothing else in whatever order.
* @param partRelations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's partRelations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's partRelations does not contain all given VecPartRelation elements.
*/
public S hasOnlyPartRelations(VecPartRelation... partRelations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation varargs is not null.
if (partRelations == null) failWithMessage("Expecting partRelations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPartRelations(), partRelations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's partRelations contains only the given VecPartRelation elements in Collection and nothing else in whatever order.
* @param partRelations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's partRelations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's partRelations does not contain all given VecPartRelation elements.
*/
public S hasOnlyPartRelations(java.util.Collection extends VecPartRelation> partRelations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation collection is not null.
if (partRelations == null) {
failWithMessage("Expecting partRelations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPartRelations(), partRelations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's partRelations does not contain the given VecPartRelation elements.
*
* @param partRelations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's partRelations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's partRelations contains any given VecPartRelation elements.
*/
public S doesNotHavePartRelations(VecPartRelation... partRelations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation varargs is not null.
if (partRelations == null) failWithMessage("Expecting partRelations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPartRelations(), partRelations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's partRelations does not contain the given VecPartRelation elements in Collection.
*
* @param partRelations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's partRelations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's partRelations contains any given VecPartRelation elements.
*/
public S doesNotHavePartRelations(java.util.Collection extends VecPartRelation> partRelations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation collection is not null.
if (partRelations == null) {
failWithMessage("Expecting partRelations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPartRelations(), partRelations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification has no partRelations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's partRelations is not empty.
*/
public S hasNoPartRelations() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have partRelations but had :\n <%s>";
// check
if (actual.getPartRelations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPartRelations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's robustnessProperties contains the given VecRobustnessProperties elements.
* @param robustnessProperties the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's robustnessProperties.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's robustnessProperties does not contain all given VecRobustnessProperties elements.
*/
public S hasRobustnessProperties(VecRobustnessProperties... robustnessProperties) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecRobustnessProperties varargs is not null.
if (robustnessProperties == null) failWithMessage("Expecting robustnessProperties parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRobustnessProperties(), robustnessProperties);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's robustnessProperties contains the given VecRobustnessProperties elements in Collection.
* @param robustnessProperties the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's robustnessProperties.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's robustnessProperties does not contain all given VecRobustnessProperties elements.
*/
public S hasRobustnessProperties(java.util.Collection extends VecRobustnessProperties> robustnessProperties) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecRobustnessProperties collection is not null.
if (robustnessProperties == null) {
failWithMessage("Expecting robustnessProperties parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRobustnessProperties(), robustnessProperties.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's robustnessProperties contains only the given VecRobustnessProperties elements and nothing else in whatever order.
* @param robustnessProperties the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's robustnessProperties.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's robustnessProperties does not contain all given VecRobustnessProperties elements.
*/
public S hasOnlyRobustnessProperties(VecRobustnessProperties... robustnessProperties) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecRobustnessProperties varargs is not null.
if (robustnessProperties == null) failWithMessage("Expecting robustnessProperties parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRobustnessProperties(), robustnessProperties);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's robustnessProperties contains only the given VecRobustnessProperties elements in Collection and nothing else in whatever order.
* @param robustnessProperties the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's robustnessProperties.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's robustnessProperties does not contain all given VecRobustnessProperties elements.
*/
public S hasOnlyRobustnessProperties(java.util.Collection extends VecRobustnessProperties> robustnessProperties) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecRobustnessProperties collection is not null.
if (robustnessProperties == null) {
failWithMessage("Expecting robustnessProperties parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRobustnessProperties(), robustnessProperties.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's robustnessProperties does not contain the given VecRobustnessProperties elements.
*
* @param robustnessProperties the given elements that should not be in actual VecGeneralTechnicalPartSpecification's robustnessProperties.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's robustnessProperties contains any given VecRobustnessProperties elements.
*/
public S doesNotHaveRobustnessProperties(VecRobustnessProperties... robustnessProperties) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecRobustnessProperties varargs is not null.
if (robustnessProperties == null) failWithMessage("Expecting robustnessProperties parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRobustnessProperties(), robustnessProperties);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's robustnessProperties does not contain the given VecRobustnessProperties elements in Collection.
*
* @param robustnessProperties the given elements that should not be in actual VecGeneralTechnicalPartSpecification's robustnessProperties.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's robustnessProperties contains any given VecRobustnessProperties elements.
*/
public S doesNotHaveRobustnessProperties(java.util.Collection extends VecRobustnessProperties> robustnessProperties) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecRobustnessProperties collection is not null.
if (robustnessProperties == null) {
failWithMessage("Expecting robustnessProperties parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRobustnessProperties(), robustnessProperties.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification has no robustnessProperties.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's robustnessProperties is not empty.
*/
public S hasNoRobustnessProperties() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have robustnessProperties but had :\n <%s>";
// check
if (actual.getRobustnessProperties().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRobustnessProperties());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's temperatureInformations contains the given VecTemperatureInformation elements.
* @param temperatureInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's temperatureInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's temperatureInformations does not contain all given VecTemperatureInformation elements.
*/
public S hasTemperatureInformations(VecTemperatureInformation... temperatureInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTemperatureInformation varargs is not null.
if (temperatureInformations == null) failWithMessage("Expecting temperatureInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTemperatureInformations(), temperatureInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's temperatureInformations contains the given VecTemperatureInformation elements in Collection.
* @param temperatureInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's temperatureInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's temperatureInformations does not contain all given VecTemperatureInformation elements.
*/
public S hasTemperatureInformations(java.util.Collection extends VecTemperatureInformation> temperatureInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTemperatureInformation collection is not null.
if (temperatureInformations == null) {
failWithMessage("Expecting temperatureInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTemperatureInformations(), temperatureInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's temperatureInformations contains only the given VecTemperatureInformation elements and nothing else in whatever order.
* @param temperatureInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's temperatureInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's temperatureInformations does not contain all given VecTemperatureInformation elements.
*/
public S hasOnlyTemperatureInformations(VecTemperatureInformation... temperatureInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTemperatureInformation varargs is not null.
if (temperatureInformations == null) failWithMessage("Expecting temperatureInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTemperatureInformations(), temperatureInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's temperatureInformations contains only the given VecTemperatureInformation elements in Collection and nothing else in whatever order.
* @param temperatureInformations the given elements that should be contained in actual VecGeneralTechnicalPartSpecification's temperatureInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's temperatureInformations does not contain all given VecTemperatureInformation elements.
*/
public S hasOnlyTemperatureInformations(java.util.Collection extends VecTemperatureInformation> temperatureInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTemperatureInformation collection is not null.
if (temperatureInformations == null) {
failWithMessage("Expecting temperatureInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTemperatureInformations(), temperatureInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's temperatureInformations does not contain the given VecTemperatureInformation elements.
*
* @param temperatureInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's temperatureInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's temperatureInformations contains any given VecTemperatureInformation elements.
*/
public S doesNotHaveTemperatureInformations(VecTemperatureInformation... temperatureInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTemperatureInformation varargs is not null.
if (temperatureInformations == null) failWithMessage("Expecting temperatureInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTemperatureInformations(), temperatureInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification's temperatureInformations does not contain the given VecTemperatureInformation elements in Collection.
*
* @param temperatureInformations the given elements that should not be in actual VecGeneralTechnicalPartSpecification's temperatureInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's temperatureInformations contains any given VecTemperatureInformation elements.
*/
public S doesNotHaveTemperatureInformations(java.util.Collection extends VecTemperatureInformation> temperatureInformations) {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTemperatureInformation collection is not null.
if (temperatureInformations == null) {
failWithMessage("Expecting temperatureInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTemperatureInformations(), temperatureInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification has no temperatureInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecGeneralTechnicalPartSpecification's temperatureInformations is not empty.
*/
public S hasNoTemperatureInformations() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have temperatureInformations but had :\n <%s>";
// check
if (actual.getTemperatureInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getTemperatureInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification is unspecified accessory permitted.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeneralTechnicalPartSpecification is not unspecified accessory permitted.
*/
public S isUnspecifiedAccessoryPermitted() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isUnspecifiedAccessoryPermitted())) {
failWithMessage("\nExpecting that actual VecGeneralTechnicalPartSpecification is unspecified accessory permitted but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeneralTechnicalPartSpecification is not unspecified accessory permitted.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeneralTechnicalPartSpecification is unspecified accessory permitted.
*/
public S isNotUnspecifiedAccessoryPermitted() {
// check that actual VecGeneralTechnicalPartSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isUnspecifiedAccessoryPermitted())) {
failWithMessage("\nExpecting that actual VecGeneralTechnicalPartSpecification is not unspecified accessory permitted but is.");
}
// return the current assertion for method chaining
return myself;
}
}