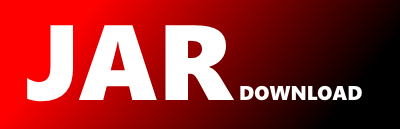
com.foursoft.vecmodel.vec120.AbstractVecGeometrySegment2DAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecGeometrySegment2D} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecGeometrySegment2DAssert, A extends VecGeometrySegment2D> extends AbstractVecGeometrySegmentAssert {
/**
* Creates a new {@link AbstractVecGeometrySegment2DAssert}
to make assertions on actual VecGeometrySegment2D.
* @param actual the VecGeometrySegment2D we want to make assertions on.
*/
protected AbstractVecGeometrySegment2DAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecGeometrySegment2D's endNode is equal to the given one.
* @param endNode the given endNode to compare the actual VecGeometrySegment2D's endNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeometrySegment2D's endNode is not equal to the given one.
*/
public S hasEndNode(VecGeometryNode2D endNode) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting endNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecGeometryNode2D actualEndNode = actual.getEndNode();
if (!Objects.areEqual(actualEndNode, endNode)) {
failWithMessage(assertjErrorMessage, actual, endNode, actualEndNode);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's endVector is equal to the given one.
* @param endVector the given endVector to compare the actual VecGeometrySegment2D's endVector to.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeometrySegment2D's endVector is not equal to the given one.
*/
public S hasEndVector(VecCartesianVector2D endVector) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting endVector of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCartesianVector2D actualEndVector = actual.getEndVector();
if (!Objects.areEqual(actualEndVector, endVector)) {
failWithMessage(assertjErrorMessage, actual, endVector, actualEndVector);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's parentBuildingBlockSpecification2D is equal to the given one.
* @param parentBuildingBlockSpecification2D the given parentBuildingBlockSpecification2D to compare the actual VecGeometrySegment2D's parentBuildingBlockSpecification2D to.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeometrySegment2D's parentBuildingBlockSpecification2D is not equal to the given one.
*/
public S hasParentBuildingBlockSpecification2D(VecBuildingBlockSpecification2D parentBuildingBlockSpecification2D) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentBuildingBlockSpecification2D of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecBuildingBlockSpecification2D actualParentBuildingBlockSpecification2D = actual.getParentBuildingBlockSpecification2D();
if (!Objects.areEqual(actualParentBuildingBlockSpecification2D, parentBuildingBlockSpecification2D)) {
failWithMessage(assertjErrorMessage, actual, parentBuildingBlockSpecification2D, actualParentBuildingBlockSpecification2D);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's pathSegments contains the given VecPathSegment elements.
* @param pathSegments the given elements that should be contained in actual VecGeometrySegment2D's pathSegments.
* @return this assertion object.
* @throws AssertionError if the actual VecGeometrySegment2D's pathSegments does not contain all given VecPathSegment elements.
*/
public S hasPathSegments(VecPathSegment... pathSegments) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// check that given VecPathSegment varargs is not null.
if (pathSegments == null) failWithMessage("Expecting pathSegments parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPathSegments(), pathSegments);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's pathSegments contains the given VecPathSegment elements in Collection.
* @param pathSegments the given elements that should be contained in actual VecGeometrySegment2D's pathSegments.
* @return this assertion object.
* @throws AssertionError if the actual VecGeometrySegment2D's pathSegments does not contain all given VecPathSegment elements.
*/
public S hasPathSegments(java.util.Collection extends VecPathSegment> pathSegments) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// check that given VecPathSegment collection is not null.
if (pathSegments == null) {
failWithMessage("Expecting pathSegments parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPathSegments(), pathSegments.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's pathSegments contains only the given VecPathSegment elements and nothing else in whatever order.
* @param pathSegments the given elements that should be contained in actual VecGeometrySegment2D's pathSegments.
* @return this assertion object.
* @throws AssertionError if the actual VecGeometrySegment2D's pathSegments does not contain all given VecPathSegment elements.
*/
public S hasOnlyPathSegments(VecPathSegment... pathSegments) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// check that given VecPathSegment varargs is not null.
if (pathSegments == null) failWithMessage("Expecting pathSegments parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPathSegments(), pathSegments);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's pathSegments contains only the given VecPathSegment elements in Collection and nothing else in whatever order.
* @param pathSegments the given elements that should be contained in actual VecGeometrySegment2D's pathSegments.
* @return this assertion object.
* @throws AssertionError if the actual VecGeometrySegment2D's pathSegments does not contain all given VecPathSegment elements.
*/
public S hasOnlyPathSegments(java.util.Collection extends VecPathSegment> pathSegments) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// check that given VecPathSegment collection is not null.
if (pathSegments == null) {
failWithMessage("Expecting pathSegments parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPathSegments(), pathSegments.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's pathSegments does not contain the given VecPathSegment elements.
*
* @param pathSegments the given elements that should not be in actual VecGeometrySegment2D's pathSegments.
* @return this assertion object.
* @throws AssertionError if the actual VecGeometrySegment2D's pathSegments contains any given VecPathSegment elements.
*/
public S doesNotHavePathSegments(VecPathSegment... pathSegments) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// check that given VecPathSegment varargs is not null.
if (pathSegments == null) failWithMessage("Expecting pathSegments parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPathSegments(), pathSegments);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's pathSegments does not contain the given VecPathSegment elements in Collection.
*
* @param pathSegments the given elements that should not be in actual VecGeometrySegment2D's pathSegments.
* @return this assertion object.
* @throws AssertionError if the actual VecGeometrySegment2D's pathSegments contains any given VecPathSegment elements.
*/
public S doesNotHavePathSegments(java.util.Collection extends VecPathSegment> pathSegments) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// check that given VecPathSegment collection is not null.
if (pathSegments == null) {
failWithMessage("Expecting pathSegments parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPathSegments(), pathSegments.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D has no pathSegments.
* @return this assertion object.
* @throws AssertionError if the actual VecGeometrySegment2D's pathSegments is not empty.
*/
public S hasNoPathSegments() {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have pathSegments but had :\n <%s>";
// check
if (actual.getPathSegments().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPathSegments());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's startNode is equal to the given one.
* @param startNode the given startNode to compare the actual VecGeometrySegment2D's startNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeometrySegment2D's startNode is not equal to the given one.
*/
public S hasStartNode(VecGeometryNode2D startNode) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting startNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecGeometryNode2D actualStartNode = actual.getStartNode();
if (!Objects.areEqual(actualStartNode, startNode)) {
failWithMessage(assertjErrorMessage, actual, startNode, actualStartNode);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecGeometrySegment2D's startVector is equal to the given one.
* @param startVector the given startVector to compare the actual VecGeometrySegment2D's startVector to.
* @return this assertion object.
* @throws AssertionError - if the actual VecGeometrySegment2D's startVector is not equal to the given one.
*/
public S hasStartVector(VecCartesianVector2D startVector) {
// check that actual VecGeometrySegment2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting startVector of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCartesianVector2D actualStartVector = actual.getStartVector();
if (!Objects.areEqual(actualStartVector, startVector)) {
failWithMessage(assertjErrorMessage, actual, startVector, actualStartVector);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy