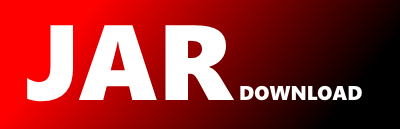
com.foursoft.vecmodel.vec120.AbstractVecInternalTerminalConnectionAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecInternalTerminalConnection} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecInternalTerminalConnectionAssert, A extends VecInternalTerminalConnection> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecInternalTerminalConnectionAssert}
to make assertions on actual VecInternalTerminalConnection.
* @param actual the VecInternalTerminalConnection we want to make assertions on.
*/
protected AbstractVecInternalTerminalConnectionAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecInternalTerminalConnection's identification is equal to the given one.
* @param identification the given identification to compare the actual VecInternalTerminalConnection's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecInternalTerminalConnection's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's parentTerminalSpecification is equal to the given one.
* @param parentTerminalSpecification the given parentTerminalSpecification to compare the actual VecInternalTerminalConnection's parentTerminalSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecInternalTerminalConnection's parentTerminalSpecification is not equal to the given one.
*/
public S hasParentTerminalSpecification(VecTerminalSpecification parentTerminalSpecification) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentTerminalSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTerminalSpecification actualParentTerminalSpecification = actual.getParentTerminalSpecification();
if (!Objects.areEqual(actualParentTerminalSpecification, parentTerminalSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentTerminalSpecification, actualParentTerminalSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's terminalReception contains the given VecTerminalReception elements.
* @param terminalReception the given elements that should be contained in actual VecInternalTerminalConnection's terminalReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's terminalReception does not contain all given VecTerminalReception elements.
*/
public S hasTerminalReception(VecTerminalReception... terminalReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception varargs is not null.
if (terminalReception == null) failWithMessage("Expecting terminalReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTerminalReception(), terminalReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's terminalReception contains the given VecTerminalReception elements in Collection.
* @param terminalReception the given elements that should be contained in actual VecInternalTerminalConnection's terminalReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's terminalReception does not contain all given VecTerminalReception elements.
*/
public S hasTerminalReception(java.util.Collection extends VecTerminalReception> terminalReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception collection is not null.
if (terminalReception == null) {
failWithMessage("Expecting terminalReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTerminalReception(), terminalReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's terminalReception contains only the given VecTerminalReception elements and nothing else in whatever order.
* @param terminalReception the given elements that should be contained in actual VecInternalTerminalConnection's terminalReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's terminalReception does not contain all given VecTerminalReception elements.
*/
public S hasOnlyTerminalReception(VecTerminalReception... terminalReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception varargs is not null.
if (terminalReception == null) failWithMessage("Expecting terminalReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTerminalReception(), terminalReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's terminalReception contains only the given VecTerminalReception elements in Collection and nothing else in whatever order.
* @param terminalReception the given elements that should be contained in actual VecInternalTerminalConnection's terminalReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's terminalReception does not contain all given VecTerminalReception elements.
*/
public S hasOnlyTerminalReception(java.util.Collection extends VecTerminalReception> terminalReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception collection is not null.
if (terminalReception == null) {
failWithMessage("Expecting terminalReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTerminalReception(), terminalReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's terminalReception does not contain the given VecTerminalReception elements.
*
* @param terminalReception the given elements that should not be in actual VecInternalTerminalConnection's terminalReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's terminalReception contains any given VecTerminalReception elements.
*/
public S doesNotHaveTerminalReception(VecTerminalReception... terminalReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception varargs is not null.
if (terminalReception == null) failWithMessage("Expecting terminalReception parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTerminalReception(), terminalReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's terminalReception does not contain the given VecTerminalReception elements in Collection.
*
* @param terminalReception the given elements that should not be in actual VecInternalTerminalConnection's terminalReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's terminalReception contains any given VecTerminalReception elements.
*/
public S doesNotHaveTerminalReception(java.util.Collection extends VecTerminalReception> terminalReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception collection is not null.
if (terminalReception == null) {
failWithMessage("Expecting terminalReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTerminalReception(), terminalReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection has no terminalReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's terminalReception is not empty.
*/
public S hasNoTerminalReception() {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have terminalReception but had :\n <%s>";
// check
if (actual.getTerminalReception().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getTerminalReception());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's wireReception contains the given VecWireReception elements.
* @param wireReception the given elements that should be contained in actual VecInternalTerminalConnection's wireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's wireReception does not contain all given VecWireReception elements.
*/
public S hasWireReception(VecWireReception... wireReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (wireReception == null) failWithMessage("Expecting wireReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireReception(), wireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's wireReception contains the given VecWireReception elements in Collection.
* @param wireReception the given elements that should be contained in actual VecInternalTerminalConnection's wireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's wireReception does not contain all given VecWireReception elements.
*/
public S hasWireReception(java.util.Collection extends VecWireReception> wireReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (wireReception == null) {
failWithMessage("Expecting wireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireReception(), wireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's wireReception contains only the given VecWireReception elements and nothing else in whatever order.
* @param wireReception the given elements that should be contained in actual VecInternalTerminalConnection's wireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's wireReception does not contain all given VecWireReception elements.
*/
public S hasOnlyWireReception(VecWireReception... wireReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (wireReception == null) failWithMessage("Expecting wireReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireReception(), wireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's wireReception contains only the given VecWireReception elements in Collection and nothing else in whatever order.
* @param wireReception the given elements that should be contained in actual VecInternalTerminalConnection's wireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's wireReception does not contain all given VecWireReception elements.
*/
public S hasOnlyWireReception(java.util.Collection extends VecWireReception> wireReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (wireReception == null) {
failWithMessage("Expecting wireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireReception(), wireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's wireReception does not contain the given VecWireReception elements.
*
* @param wireReception the given elements that should not be in actual VecInternalTerminalConnection's wireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's wireReception contains any given VecWireReception elements.
*/
public S doesNotHaveWireReception(VecWireReception... wireReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (wireReception == null) failWithMessage("Expecting wireReception parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireReception(), wireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection's wireReception does not contain the given VecWireReception elements in Collection.
*
* @param wireReception the given elements that should not be in actual VecInternalTerminalConnection's wireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's wireReception contains any given VecWireReception elements.
*/
public S doesNotHaveWireReception(java.util.Collection extends VecWireReception> wireReception) {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (wireReception == null) {
failWithMessage("Expecting wireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireReception(), wireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecInternalTerminalConnection has no wireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecInternalTerminalConnection's wireReception is not empty.
*/
public S hasNoWireReception() {
// check that actual VecInternalTerminalConnection we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have wireReception but had :\n <%s>";
// check
if (actual.getWireReception().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getWireReception());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy