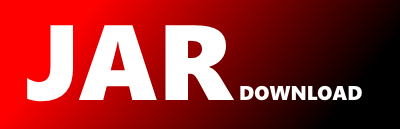
com.foursoft.vecmodel.vec120.AbstractVecItemVersionAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecItemVersion} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecItemVersionAssert, A extends VecItemVersion> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecItemVersionAssert}
to make assertions on actual VecItemVersion.
* @param actual the VecItemVersion we want to make assertions on.
*/
protected AbstractVecItemVersionAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecItemVersion's abbreviations contains the given VecLocalizedString elements.
* @param abbreviations the given elements that should be contained in actual VecItemVersion's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasAbbreviations(VecLocalizedString... abbreviations) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (abbreviations == null) failWithMessage("Expecting abbreviations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAbbreviations(), abbreviations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's abbreviations contains the given VecLocalizedString elements in Collection.
* @param abbreviations the given elements that should be contained in actual VecItemVersion's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasAbbreviations(java.util.Collection extends VecLocalizedString> abbreviations) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (abbreviations == null) {
failWithMessage("Expecting abbreviations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAbbreviations(), abbreviations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's abbreviations contains only the given VecLocalizedString elements and nothing else in whatever order.
* @param abbreviations the given elements that should be contained in actual VecItemVersion's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasOnlyAbbreviations(VecLocalizedString... abbreviations) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (abbreviations == null) failWithMessage("Expecting abbreviations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAbbreviations(), abbreviations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's abbreviations contains only the given VecLocalizedString elements in Collection and nothing else in whatever order.
* @param abbreviations the given elements that should be contained in actual VecItemVersion's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasOnlyAbbreviations(java.util.Collection extends VecLocalizedString> abbreviations) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (abbreviations == null) {
failWithMessage("Expecting abbreviations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAbbreviations(), abbreviations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's abbreviations does not contain the given VecLocalizedString elements.
*
* @param abbreviations the given elements that should not be in actual VecItemVersion's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's abbreviations contains any given VecLocalizedString elements.
*/
public S doesNotHaveAbbreviations(VecLocalizedString... abbreviations) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (abbreviations == null) failWithMessage("Expecting abbreviations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAbbreviations(), abbreviations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's abbreviations does not contain the given VecLocalizedString elements in Collection.
*
* @param abbreviations the given elements that should not be in actual VecItemVersion's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's abbreviations contains any given VecLocalizedString elements.
*/
public S doesNotHaveAbbreviations(java.util.Collection extends VecLocalizedString> abbreviations) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (abbreviations == null) {
failWithMessage("Expecting abbreviations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAbbreviations(), abbreviations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's abbreviations is not empty.
*/
public S hasNoAbbreviations() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have abbreviations but had :\n <%s>";
// check
if (actual.getAbbreviations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAbbreviations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's approvals contains the given VecApproval elements.
* @param approvals the given elements that should be contained in actual VecItemVersion's approvals.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's approvals does not contain all given VecApproval elements.
*/
public S hasApprovals(VecApproval... approvals) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecApproval varargs is not null.
if (approvals == null) failWithMessage("Expecting approvals parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getApprovals(), approvals);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's approvals contains the given VecApproval elements in Collection.
* @param approvals the given elements that should be contained in actual VecItemVersion's approvals.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's approvals does not contain all given VecApproval elements.
*/
public S hasApprovals(java.util.Collection extends VecApproval> approvals) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecApproval collection is not null.
if (approvals == null) {
failWithMessage("Expecting approvals parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getApprovals(), approvals.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's approvals contains only the given VecApproval elements and nothing else in whatever order.
* @param approvals the given elements that should be contained in actual VecItemVersion's approvals.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's approvals does not contain all given VecApproval elements.
*/
public S hasOnlyApprovals(VecApproval... approvals) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecApproval varargs is not null.
if (approvals == null) failWithMessage("Expecting approvals parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getApprovals(), approvals);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's approvals contains only the given VecApproval elements in Collection and nothing else in whatever order.
* @param approvals the given elements that should be contained in actual VecItemVersion's approvals.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's approvals does not contain all given VecApproval elements.
*/
public S hasOnlyApprovals(java.util.Collection extends VecApproval> approvals) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecApproval collection is not null.
if (approvals == null) {
failWithMessage("Expecting approvals parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getApprovals(), approvals.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's approvals does not contain the given VecApproval elements.
*
* @param approvals the given elements that should not be in actual VecItemVersion's approvals.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's approvals contains any given VecApproval elements.
*/
public S doesNotHaveApprovals(VecApproval... approvals) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecApproval varargs is not null.
if (approvals == null) failWithMessage("Expecting approvals parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getApprovals(), approvals);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's approvals does not contain the given VecApproval elements in Collection.
*
* @param approvals the given elements that should not be in actual VecItemVersion's approvals.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's approvals contains any given VecApproval elements.
*/
public S doesNotHaveApprovals(java.util.Collection extends VecApproval> approvals) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecApproval collection is not null.
if (approvals == null) {
failWithMessage("Expecting approvals parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getApprovals(), approvals.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no approvals.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's approvals is not empty.
*/
public S hasNoApprovals() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have approvals but had :\n <%s>";
// check
if (actual.getApprovals().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getApprovals());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's changeDescriptions contains the given VecChangeDescription elements.
* @param changeDescriptions the given elements that should be contained in actual VecItemVersion's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasChangeDescriptions(VecChangeDescription... changeDescriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription varargs is not null.
if (changeDescriptions == null) failWithMessage("Expecting changeDescriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getChangeDescriptions(), changeDescriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's changeDescriptions contains the given VecChangeDescription elements in Collection.
* @param changeDescriptions the given elements that should be contained in actual VecItemVersion's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasChangeDescriptions(java.util.Collection extends VecChangeDescription> changeDescriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription collection is not null.
if (changeDescriptions == null) {
failWithMessage("Expecting changeDescriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getChangeDescriptions(), changeDescriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's changeDescriptions contains only the given VecChangeDescription elements and nothing else in whatever order.
* @param changeDescriptions the given elements that should be contained in actual VecItemVersion's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasOnlyChangeDescriptions(VecChangeDescription... changeDescriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription varargs is not null.
if (changeDescriptions == null) failWithMessage("Expecting changeDescriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getChangeDescriptions(), changeDescriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's changeDescriptions contains only the given VecChangeDescription elements in Collection and nothing else in whatever order.
* @param changeDescriptions the given elements that should be contained in actual VecItemVersion's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasOnlyChangeDescriptions(java.util.Collection extends VecChangeDescription> changeDescriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription collection is not null.
if (changeDescriptions == null) {
failWithMessage("Expecting changeDescriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getChangeDescriptions(), changeDescriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's changeDescriptions does not contain the given VecChangeDescription elements.
*
* @param changeDescriptions the given elements that should not be in actual VecItemVersion's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's changeDescriptions contains any given VecChangeDescription elements.
*/
public S doesNotHaveChangeDescriptions(VecChangeDescription... changeDescriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription varargs is not null.
if (changeDescriptions == null) failWithMessage("Expecting changeDescriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getChangeDescriptions(), changeDescriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's changeDescriptions does not contain the given VecChangeDescription elements in Collection.
*
* @param changeDescriptions the given elements that should not be in actual VecItemVersion's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's changeDescriptions contains any given VecChangeDescription elements.
*/
public S doesNotHaveChangeDescriptions(java.util.Collection extends VecChangeDescription> changeDescriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription collection is not null.
if (changeDescriptions == null) {
failWithMessage("Expecting changeDescriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getChangeDescriptions(), changeDescriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's changeDescriptions is not empty.
*/
public S hasNoChangeDescriptions() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have changeDescriptions but had :\n <%s>";
// check
if (actual.getChangeDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getChangeDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's companyName is equal to the given one.
* @param companyName the given companyName to compare the actual VecItemVersion's companyName to.
* @return this assertion object.
* @throws AssertionError - if the actual VecItemVersion's companyName is not equal to the given one.
*/
public S hasCompanyName(String companyName) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting companyName of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualCompanyName = actual.getCompanyName();
if (!Objects.areEqual(actualCompanyName, companyName)) {
failWithMessage(assertjErrorMessage, actual, companyName, actualCompanyName);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's contract contains the given VecContract elements.
* @param contract the given elements that should be contained in actual VecItemVersion's contract.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's contract does not contain all given VecContract elements.
*/
public S hasContract(VecContract... contract) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecContract varargs is not null.
if (contract == null) failWithMessage("Expecting contract parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getContract(), contract);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's contract contains the given VecContract elements in Collection.
* @param contract the given elements that should be contained in actual VecItemVersion's contract.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's contract does not contain all given VecContract elements.
*/
public S hasContract(java.util.Collection extends VecContract> contract) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecContract collection is not null.
if (contract == null) {
failWithMessage("Expecting contract parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getContract(), contract.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's contract contains only the given VecContract elements and nothing else in whatever order.
* @param contract the given elements that should be contained in actual VecItemVersion's contract.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's contract does not contain all given VecContract elements.
*/
public S hasOnlyContract(VecContract... contract) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecContract varargs is not null.
if (contract == null) failWithMessage("Expecting contract parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getContract(), contract);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's contract contains only the given VecContract elements in Collection and nothing else in whatever order.
* @param contract the given elements that should be contained in actual VecItemVersion's contract.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's contract does not contain all given VecContract elements.
*/
public S hasOnlyContract(java.util.Collection extends VecContract> contract) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecContract collection is not null.
if (contract == null) {
failWithMessage("Expecting contract parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getContract(), contract.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's contract does not contain the given VecContract elements.
*
* @param contract the given elements that should not be in actual VecItemVersion's contract.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's contract contains any given VecContract elements.
*/
public S doesNotHaveContract(VecContract... contract) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecContract varargs is not null.
if (contract == null) failWithMessage("Expecting contract parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getContract(), contract);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's contract does not contain the given VecContract elements in Collection.
*
* @param contract the given elements that should not be in actual VecItemVersion's contract.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's contract contains any given VecContract elements.
*/
public S doesNotHaveContract(java.util.Collection extends VecContract> contract) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecContract collection is not null.
if (contract == null) {
failWithMessage("Expecting contract parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getContract(), contract.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no contract.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's contract is not empty.
*/
public S hasNoContract() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have contract but had :\n <%s>";
// check
if (actual.getContract().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getContract());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's copyrightInformation is equal to the given one.
* @param copyrightInformation the given copyrightInformation to compare the actual VecItemVersion's copyrightInformation to.
* @return this assertion object.
* @throws AssertionError - if the actual VecItemVersion's copyrightInformation is not equal to the given one.
*/
public S hasCopyrightInformation(VecCopyrightInformation copyrightInformation) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting copyrightInformation of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCopyrightInformation actualCopyrightInformation = actual.getCopyrightInformation();
if (!Objects.areEqual(actualCopyrightInformation, copyrightInformation)) {
failWithMessage(assertjErrorMessage, actual, copyrightInformation, actualCopyrightInformation);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's creation is equal to the given one.
* @param creation the given creation to compare the actual VecItemVersion's creation to.
* @return this assertion object.
* @throws AssertionError - if the actual VecItemVersion's creation is not equal to the given one.
*/
public S hasCreation(VecCreation creation) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting creation of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCreation actualCreation = actual.getCreation();
if (!Objects.areEqual(actualCreation, creation)) {
failWithMessage(assertjErrorMessage, actual, creation, actualCreation);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's descriptions contains the given VecAbstractLocalizedString elements.
* @param descriptions the given elements that should be contained in actual VecItemVersion's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's descriptions contains the given VecAbstractLocalizedString elements in Collection.
* @param descriptions the given elements that should be contained in actual VecItemVersion's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's descriptions contains only the given VecAbstractLocalizedString elements and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecItemVersion's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's descriptions contains only the given VecAbstractLocalizedString elements in Collection and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecItemVersion's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's descriptions does not contain the given VecAbstractLocalizedString elements.
*
* @param descriptions the given elements that should not be in actual VecItemVersion's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's descriptions does not contain the given VecAbstractLocalizedString elements in Collection.
*
* @param descriptions the given elements that should not be in actual VecItemVersion's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's descriptions is not empty.
*/
public S hasNoDescriptions() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have descriptions but had :\n <%s>";
// check
if (actual.getDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's processingInstructions contains the given VecInstruction elements.
* @param processingInstructions the given elements that should be contained in actual VecItemVersion's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's processingInstructions does not contain all given VecInstruction elements.
*/
public S hasProcessingInstructions(VecInstruction... processingInstructions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's processingInstructions contains the given VecInstruction elements in Collection.
* @param processingInstructions the given elements that should be contained in actual VecItemVersion's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's processingInstructions does not contain all given VecInstruction elements.
*/
public S hasProcessingInstructions(java.util.Collection extends VecInstruction> processingInstructions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's processingInstructions contains only the given VecInstruction elements and nothing else in whatever order.
* @param processingInstructions the given elements that should be contained in actual VecItemVersion's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's processingInstructions does not contain all given VecInstruction elements.
*/
public S hasOnlyProcessingInstructions(VecInstruction... processingInstructions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's processingInstructions contains only the given VecInstruction elements in Collection and nothing else in whatever order.
* @param processingInstructions the given elements that should be contained in actual VecItemVersion's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's processingInstructions does not contain all given VecInstruction elements.
*/
public S hasOnlyProcessingInstructions(java.util.Collection extends VecInstruction> processingInstructions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's processingInstructions does not contain the given VecInstruction elements.
*
* @param processingInstructions the given elements that should not be in actual VecItemVersion's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's processingInstructions contains any given VecInstruction elements.
*/
public S doesNotHaveProcessingInstructions(VecInstruction... processingInstructions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's processingInstructions does not contain the given VecInstruction elements in Collection.
*
* @param processingInstructions the given elements that should not be in actual VecItemVersion's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's processingInstructions contains any given VecInstruction elements.
*/
public S doesNotHaveProcessingInstructions(java.util.Collection extends VecInstruction> processingInstructions) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecInstruction collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's processingInstructions is not empty.
*/
public S hasNoProcessingInstructions() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have processingInstructions but had :\n <%s>";
// check
if (actual.getProcessingInstructions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getProcessingInstructions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refBaselineSpecification contains the given VecBaselineSpecification elements.
* @param refBaselineSpecification the given elements that should be contained in actual VecItemVersion's refBaselineSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refBaselineSpecification does not contain all given VecBaselineSpecification elements.
*/
public S hasRefBaselineSpecification(VecBaselineSpecification... refBaselineSpecification) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecBaselineSpecification varargs is not null.
if (refBaselineSpecification == null) failWithMessage("Expecting refBaselineSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBaselineSpecification(), refBaselineSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refBaselineSpecification contains the given VecBaselineSpecification elements in Collection.
* @param refBaselineSpecification the given elements that should be contained in actual VecItemVersion's refBaselineSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refBaselineSpecification does not contain all given VecBaselineSpecification elements.
*/
public S hasRefBaselineSpecification(java.util.Collection extends VecBaselineSpecification> refBaselineSpecification) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecBaselineSpecification collection is not null.
if (refBaselineSpecification == null) {
failWithMessage("Expecting refBaselineSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBaselineSpecification(), refBaselineSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refBaselineSpecification contains only the given VecBaselineSpecification elements and nothing else in whatever order.
* @param refBaselineSpecification the given elements that should be contained in actual VecItemVersion's refBaselineSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refBaselineSpecification does not contain all given VecBaselineSpecification elements.
*/
public S hasOnlyRefBaselineSpecification(VecBaselineSpecification... refBaselineSpecification) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecBaselineSpecification varargs is not null.
if (refBaselineSpecification == null) failWithMessage("Expecting refBaselineSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBaselineSpecification(), refBaselineSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refBaselineSpecification contains only the given VecBaselineSpecification elements in Collection and nothing else in whatever order.
* @param refBaselineSpecification the given elements that should be contained in actual VecItemVersion's refBaselineSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refBaselineSpecification does not contain all given VecBaselineSpecification elements.
*/
public S hasOnlyRefBaselineSpecification(java.util.Collection extends VecBaselineSpecification> refBaselineSpecification) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecBaselineSpecification collection is not null.
if (refBaselineSpecification == null) {
failWithMessage("Expecting refBaselineSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBaselineSpecification(), refBaselineSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refBaselineSpecification does not contain the given VecBaselineSpecification elements.
*
* @param refBaselineSpecification the given elements that should not be in actual VecItemVersion's refBaselineSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refBaselineSpecification contains any given VecBaselineSpecification elements.
*/
public S doesNotHaveRefBaselineSpecification(VecBaselineSpecification... refBaselineSpecification) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecBaselineSpecification varargs is not null.
if (refBaselineSpecification == null) failWithMessage("Expecting refBaselineSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBaselineSpecification(), refBaselineSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refBaselineSpecification does not contain the given VecBaselineSpecification elements in Collection.
*
* @param refBaselineSpecification the given elements that should not be in actual VecItemVersion's refBaselineSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refBaselineSpecification contains any given VecBaselineSpecification elements.
*/
public S doesNotHaveRefBaselineSpecification(java.util.Collection extends VecBaselineSpecification> refBaselineSpecification) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecBaselineSpecification collection is not null.
if (refBaselineSpecification == null) {
failWithMessage("Expecting refBaselineSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBaselineSpecification(), refBaselineSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no refBaselineSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refBaselineSpecification is not empty.
*/
public S hasNoRefBaselineSpecification() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refBaselineSpecification but had :\n <%s>";
// check
if (actual.getRefBaselineSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefBaselineSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemEquivalence contains the given VecItemEquivalence elements.
* @param refItemEquivalence the given elements that should be contained in actual VecItemVersion's refItemEquivalence.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemEquivalence does not contain all given VecItemEquivalence elements.
*/
public S hasRefItemEquivalence(VecItemEquivalence... refItemEquivalence) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence varargs is not null.
if (refItemEquivalence == null) failWithMessage("Expecting refItemEquivalence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefItemEquivalence(), refItemEquivalence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemEquivalence contains the given VecItemEquivalence elements in Collection.
* @param refItemEquivalence the given elements that should be contained in actual VecItemVersion's refItemEquivalence.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemEquivalence does not contain all given VecItemEquivalence elements.
*/
public S hasRefItemEquivalence(java.util.Collection extends VecItemEquivalence> refItemEquivalence) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence collection is not null.
if (refItemEquivalence == null) {
failWithMessage("Expecting refItemEquivalence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefItemEquivalence(), refItemEquivalence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemEquivalence contains only the given VecItemEquivalence elements and nothing else in whatever order.
* @param refItemEquivalence the given elements that should be contained in actual VecItemVersion's refItemEquivalence.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemEquivalence does not contain all given VecItemEquivalence elements.
*/
public S hasOnlyRefItemEquivalence(VecItemEquivalence... refItemEquivalence) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence varargs is not null.
if (refItemEquivalence == null) failWithMessage("Expecting refItemEquivalence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefItemEquivalence(), refItemEquivalence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemEquivalence contains only the given VecItemEquivalence elements in Collection and nothing else in whatever order.
* @param refItemEquivalence the given elements that should be contained in actual VecItemVersion's refItemEquivalence.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemEquivalence does not contain all given VecItemEquivalence elements.
*/
public S hasOnlyRefItemEquivalence(java.util.Collection extends VecItemEquivalence> refItemEquivalence) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence collection is not null.
if (refItemEquivalence == null) {
failWithMessage("Expecting refItemEquivalence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefItemEquivalence(), refItemEquivalence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemEquivalence does not contain the given VecItemEquivalence elements.
*
* @param refItemEquivalence the given elements that should not be in actual VecItemVersion's refItemEquivalence.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemEquivalence contains any given VecItemEquivalence elements.
*/
public S doesNotHaveRefItemEquivalence(VecItemEquivalence... refItemEquivalence) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence varargs is not null.
if (refItemEquivalence == null) failWithMessage("Expecting refItemEquivalence parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefItemEquivalence(), refItemEquivalence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemEquivalence does not contain the given VecItemEquivalence elements in Collection.
*
* @param refItemEquivalence the given elements that should not be in actual VecItemVersion's refItemEquivalence.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemEquivalence contains any given VecItemEquivalence elements.
*/
public S doesNotHaveRefItemEquivalence(java.util.Collection extends VecItemEquivalence> refItemEquivalence) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemEquivalence collection is not null.
if (refItemEquivalence == null) {
failWithMessage("Expecting refItemEquivalence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefItemEquivalence(), refItemEquivalence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no refItemEquivalence.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemEquivalence is not empty.
*/
public S hasNoRefItemEquivalence() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refItemEquivalence but had :\n <%s>";
// check
if (actual.getRefItemEquivalence().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefItemEquivalence());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemHistoryEntry contains the given VecItemHistoryEntry elements.
* @param refItemHistoryEntry the given elements that should be contained in actual VecItemVersion's refItemHistoryEntry.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemHistoryEntry does not contain all given VecItemHistoryEntry elements.
*/
public S hasRefItemHistoryEntry(VecItemHistoryEntry... refItemHistoryEntry) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemHistoryEntry varargs is not null.
if (refItemHistoryEntry == null) failWithMessage("Expecting refItemHistoryEntry parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefItemHistoryEntry(), refItemHistoryEntry);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemHistoryEntry contains the given VecItemHistoryEntry elements in Collection.
* @param refItemHistoryEntry the given elements that should be contained in actual VecItemVersion's refItemHistoryEntry.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemHistoryEntry does not contain all given VecItemHistoryEntry elements.
*/
public S hasRefItemHistoryEntry(java.util.Collection extends VecItemHistoryEntry> refItemHistoryEntry) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemHistoryEntry collection is not null.
if (refItemHistoryEntry == null) {
failWithMessage("Expecting refItemHistoryEntry parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefItemHistoryEntry(), refItemHistoryEntry.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemHistoryEntry contains only the given VecItemHistoryEntry elements and nothing else in whatever order.
* @param refItemHistoryEntry the given elements that should be contained in actual VecItemVersion's refItemHistoryEntry.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemHistoryEntry does not contain all given VecItemHistoryEntry elements.
*/
public S hasOnlyRefItemHistoryEntry(VecItemHistoryEntry... refItemHistoryEntry) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemHistoryEntry varargs is not null.
if (refItemHistoryEntry == null) failWithMessage("Expecting refItemHistoryEntry parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefItemHistoryEntry(), refItemHistoryEntry);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemHistoryEntry contains only the given VecItemHistoryEntry elements in Collection and nothing else in whatever order.
* @param refItemHistoryEntry the given elements that should be contained in actual VecItemVersion's refItemHistoryEntry.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemHistoryEntry does not contain all given VecItemHistoryEntry elements.
*/
public S hasOnlyRefItemHistoryEntry(java.util.Collection extends VecItemHistoryEntry> refItemHistoryEntry) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemHistoryEntry collection is not null.
if (refItemHistoryEntry == null) {
failWithMessage("Expecting refItemHistoryEntry parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefItemHistoryEntry(), refItemHistoryEntry.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemHistoryEntry does not contain the given VecItemHistoryEntry elements.
*
* @param refItemHistoryEntry the given elements that should not be in actual VecItemVersion's refItemHistoryEntry.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemHistoryEntry contains any given VecItemHistoryEntry elements.
*/
public S doesNotHaveRefItemHistoryEntry(VecItemHistoryEntry... refItemHistoryEntry) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemHistoryEntry varargs is not null.
if (refItemHistoryEntry == null) failWithMessage("Expecting refItemHistoryEntry parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefItemHistoryEntry(), refItemHistoryEntry);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion's refItemHistoryEntry does not contain the given VecItemHistoryEntry elements in Collection.
*
* @param refItemHistoryEntry the given elements that should not be in actual VecItemVersion's refItemHistoryEntry.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemHistoryEntry contains any given VecItemHistoryEntry elements.
*/
public S doesNotHaveRefItemHistoryEntry(java.util.Collection extends VecItemHistoryEntry> refItemHistoryEntry) {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// check that given VecItemHistoryEntry collection is not null.
if (refItemHistoryEntry == null) {
failWithMessage("Expecting refItemHistoryEntry parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefItemHistoryEntry(), refItemHistoryEntry.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecItemVersion has no refItemHistoryEntry.
* @return this assertion object.
* @throws AssertionError if the actual VecItemVersion's refItemHistoryEntry is not empty.
*/
public S hasNoRefItemHistoryEntry() {
// check that actual VecItemVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refItemHistoryEntry but had :\n <%s>";
// check
if (actual.getRefItemHistoryEntry().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefItemHistoryEntry());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy