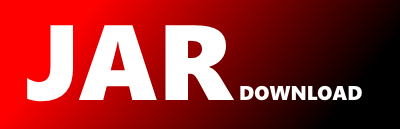
com.foursoft.vecmodel.vec120.AbstractVecLocationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecLocation} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecLocationAssert, A extends VecLocation> extends AbstractVecDimensionAnchorAssert {
/**
* Creates a new {@link AbstractVecLocationAssert}
to make assertions on actual VecLocation.
* @param actual the VecLocation we want to make assertions on.
*/
protected AbstractVecLocationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecLocation's identification is equal to the given one.
* @param identification the given identification to compare the actual VecLocation's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecLocation's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's matchingId is equal to the given one.
* @param matchingId the given matchingId to compare the actual VecLocation's matchingId to.
* @return this assertion object.
* @throws AssertionError - if the actual VecLocation's matchingId is not equal to the given one.
*/
public S hasMatchingId(String matchingId) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting matchingId of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualMatchingId = actual.getMatchingId();
if (!Objects.areEqual(actualMatchingId, matchingId)) {
failWithMessage(assertjErrorMessage, actual, matchingId, actualMatchingId);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's parentDimension is equal to the given one.
* @param parentDimension the given parentDimension to compare the actual VecLocation's parentDimension to.
* @return this assertion object.
* @throws AssertionError - if the actual VecLocation's parentDimension is not equal to the given one.
*/
public S hasParentDimension(VecDimension parentDimension) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentDimension of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecDimension actualParentDimension = actual.getParentDimension();
if (!Objects.areEqual(actualParentDimension, parentDimension)) {
failWithMessage(assertjErrorMessage, actual, parentDimension, actualParentDimension);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's parentNodeMapping is equal to the given one.
* @param parentNodeMapping the given parentNodeMapping to compare the actual VecLocation's parentNodeMapping to.
* @return this assertion object.
* @throws AssertionError - if the actual VecLocation's parentNodeMapping is not equal to the given one.
*/
public S hasParentNodeMapping(VecNodeMapping parentNodeMapping) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentNodeMapping of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNodeMapping actualParentNodeMapping = actual.getParentNodeMapping();
if (!Objects.areEqual(actualParentNodeMapping, parentNodeMapping)) {
failWithMessage(assertjErrorMessage, actual, parentNodeMapping, actualParentNodeMapping);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's parentOnPointPlacement is equal to the given one.
* @param parentOnPointPlacement the given parentOnPointPlacement to compare the actual VecLocation's parentOnPointPlacement to.
* @return this assertion object.
* @throws AssertionError - if the actual VecLocation's parentOnPointPlacement is not equal to the given one.
*/
public S hasParentOnPointPlacement(VecOnPointPlacement parentOnPointPlacement) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentOnPointPlacement of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecOnPointPlacement actualParentOnPointPlacement = actual.getParentOnPointPlacement();
if (!Objects.areEqual(actualParentOnPointPlacement, parentOnPointPlacement)) {
failWithMessage(assertjErrorMessage, actual, parentOnPointPlacement, actualParentOnPointPlacement);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's parentOnWayPlacement is equal to the given one.
* @param parentOnWayPlacement the given parentOnWayPlacement to compare the actual VecLocation's parentOnWayPlacement to.
* @return this assertion object.
* @throws AssertionError - if the actual VecLocation's parentOnWayPlacement is not equal to the given one.
*/
public S hasParentOnWayPlacement(VecOnWayPlacement parentOnWayPlacement) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentOnWayPlacement of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecOnWayPlacement actualParentOnWayPlacement = actual.getParentOnWayPlacement();
if (!Objects.areEqual(actualParentOnWayPlacement, parentOnWayPlacement)) {
failWithMessage(assertjErrorMessage, actual, parentOnWayPlacement, actualParentOnWayPlacement);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's parentZoneCoverage is equal to the given one.
* @param parentZoneCoverage the given parentZoneCoverage to compare the actual VecLocation's parentZoneCoverage to.
* @return this assertion object.
* @throws AssertionError - if the actual VecLocation's parentZoneCoverage is not equal to the given one.
*/
public S hasParentZoneCoverage(VecZoneCoverage parentZoneCoverage) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentZoneCoverage of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecZoneCoverage actualParentZoneCoverage = actual.getParentZoneCoverage();
if (!Objects.areEqual(actualParentZoneCoverage, parentZoneCoverage)) {
failWithMessage(assertjErrorMessage, actual, parentZoneCoverage, actualParentZoneCoverage);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's placedPlacementPoints contains the given VecPlacementPointReference elements.
* @param placedPlacementPoints the given elements that should be contained in actual VecLocation's placedPlacementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecLocation's placedPlacementPoints does not contain all given VecPlacementPointReference elements.
*/
public S hasPlacedPlacementPoints(VecPlacementPointReference... placedPlacementPoints) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference varargs is not null.
if (placedPlacementPoints == null) failWithMessage("Expecting placedPlacementPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlacedPlacementPoints(), placedPlacementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's placedPlacementPoints contains the given VecPlacementPointReference elements in Collection.
* @param placedPlacementPoints the given elements that should be contained in actual VecLocation's placedPlacementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecLocation's placedPlacementPoints does not contain all given VecPlacementPointReference elements.
*/
public S hasPlacedPlacementPoints(java.util.Collection extends VecPlacementPointReference> placedPlacementPoints) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference collection is not null.
if (placedPlacementPoints == null) {
failWithMessage("Expecting placedPlacementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlacedPlacementPoints(), placedPlacementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's placedPlacementPoints contains only the given VecPlacementPointReference elements and nothing else in whatever order.
* @param placedPlacementPoints the given elements that should be contained in actual VecLocation's placedPlacementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecLocation's placedPlacementPoints does not contain all given VecPlacementPointReference elements.
*/
public S hasOnlyPlacedPlacementPoints(VecPlacementPointReference... placedPlacementPoints) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference varargs is not null.
if (placedPlacementPoints == null) failWithMessage("Expecting placedPlacementPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlacedPlacementPoints(), placedPlacementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's placedPlacementPoints contains only the given VecPlacementPointReference elements in Collection and nothing else in whatever order.
* @param placedPlacementPoints the given elements that should be contained in actual VecLocation's placedPlacementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecLocation's placedPlacementPoints does not contain all given VecPlacementPointReference elements.
*/
public S hasOnlyPlacedPlacementPoints(java.util.Collection extends VecPlacementPointReference> placedPlacementPoints) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference collection is not null.
if (placedPlacementPoints == null) {
failWithMessage("Expecting placedPlacementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlacedPlacementPoints(), placedPlacementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's placedPlacementPoints does not contain the given VecPlacementPointReference elements.
*
* @param placedPlacementPoints the given elements that should not be in actual VecLocation's placedPlacementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecLocation's placedPlacementPoints contains any given VecPlacementPointReference elements.
*/
public S doesNotHavePlacedPlacementPoints(VecPlacementPointReference... placedPlacementPoints) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference varargs is not null.
if (placedPlacementPoints == null) failWithMessage("Expecting placedPlacementPoints parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlacedPlacementPoints(), placedPlacementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation's placedPlacementPoints does not contain the given VecPlacementPointReference elements in Collection.
*
* @param placedPlacementPoints the given elements that should not be in actual VecLocation's placedPlacementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecLocation's placedPlacementPoints contains any given VecPlacementPointReference elements.
*/
public S doesNotHavePlacedPlacementPoints(java.util.Collection extends VecPlacementPointReference> placedPlacementPoints) {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference collection is not null.
if (placedPlacementPoints == null) {
failWithMessage("Expecting placedPlacementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlacedPlacementPoints(), placedPlacementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecLocation has no placedPlacementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecLocation's placedPlacementPoints is not empty.
*/
public S hasNoPlacedPlacementPoints() {
// check that actual VecLocation we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have placedPlacementPoints but had :\n <%s>";
// check
if (actual.getPlacedPlacementPoints().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPlacedPlacementPoints());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy