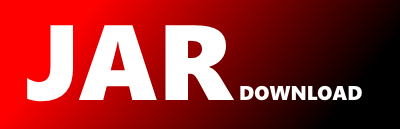
com.foursoft.vecmodel.vec120.AbstractVecNURBSCurveAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecNURBSCurve} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecNURBSCurveAssert, A extends VecNURBSCurve> extends AbstractVecCurve3DAssert {
/**
* Creates a new {@link AbstractVecNURBSCurveAssert}
to make assertions on actual VecNURBSCurve.
* @param actual the VecNURBSCurve we want to make assertions on.
*/
protected AbstractVecNURBSCurveAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecNURBSCurve's controlPoints contains the given VecNURBSControlPoint elements.
* @param controlPoints the given elements that should be contained in actual VecNURBSCurve's controlPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's controlPoints does not contain all given VecNURBSControlPoint elements.
*/
public S hasControlPoints(VecNURBSControlPoint... controlPoints) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given VecNURBSControlPoint varargs is not null.
if (controlPoints == null) failWithMessage("Expecting controlPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getControlPoints(), controlPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's controlPoints contains the given VecNURBSControlPoint elements in Collection.
* @param controlPoints the given elements that should be contained in actual VecNURBSCurve's controlPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's controlPoints does not contain all given VecNURBSControlPoint elements.
*/
public S hasControlPoints(java.util.Collection extends VecNURBSControlPoint> controlPoints) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given VecNURBSControlPoint collection is not null.
if (controlPoints == null) {
failWithMessage("Expecting controlPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getControlPoints(), controlPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's controlPoints contains only the given VecNURBSControlPoint elements and nothing else in whatever order.
* @param controlPoints the given elements that should be contained in actual VecNURBSCurve's controlPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's controlPoints does not contain all given VecNURBSControlPoint elements.
*/
public S hasOnlyControlPoints(VecNURBSControlPoint... controlPoints) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given VecNURBSControlPoint varargs is not null.
if (controlPoints == null) failWithMessage("Expecting controlPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getControlPoints(), controlPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's controlPoints contains only the given VecNURBSControlPoint elements in Collection and nothing else in whatever order.
* @param controlPoints the given elements that should be contained in actual VecNURBSCurve's controlPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's controlPoints does not contain all given VecNURBSControlPoint elements.
*/
public S hasOnlyControlPoints(java.util.Collection extends VecNURBSControlPoint> controlPoints) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given VecNURBSControlPoint collection is not null.
if (controlPoints == null) {
failWithMessage("Expecting controlPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getControlPoints(), controlPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's controlPoints does not contain the given VecNURBSControlPoint elements.
*
* @param controlPoints the given elements that should not be in actual VecNURBSCurve's controlPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's controlPoints contains any given VecNURBSControlPoint elements.
*/
public S doesNotHaveControlPoints(VecNURBSControlPoint... controlPoints) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given VecNURBSControlPoint varargs is not null.
if (controlPoints == null) failWithMessage("Expecting controlPoints parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getControlPoints(), controlPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's controlPoints does not contain the given VecNURBSControlPoint elements in Collection.
*
* @param controlPoints the given elements that should not be in actual VecNURBSCurve's controlPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's controlPoints contains any given VecNURBSControlPoint elements.
*/
public S doesNotHaveControlPoints(java.util.Collection extends VecNURBSControlPoint> controlPoints) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given VecNURBSControlPoint collection is not null.
if (controlPoints == null) {
failWithMessage("Expecting controlPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getControlPoints(), controlPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve has no controlPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's controlPoints is not empty.
*/
public S hasNoControlPoints() {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have controlPoints but had :\n <%s>";
// check
if (actual.getControlPoints().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getControlPoints());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's degree is equal to the given one.
* @param degree the given degree to compare the actual VecNURBSCurve's degree to.
* @return this assertion object.
* @throws AssertionError - if the actual VecNURBSCurve's degree is not equal to the given one.
*/
public S hasDegree(java.math.BigInteger degree) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting degree of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
java.math.BigInteger actualDegree = actual.getDegree();
if (!Objects.areEqual(actualDegree, degree)) {
failWithMessage(assertjErrorMessage, actual, degree, actualDegree);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's knots contains the given Double elements.
* @param knots the given elements that should be contained in actual VecNURBSCurve's knots.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's knots does not contain all given Double elements.
*/
public S hasKnots(Double... knots) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given Double varargs is not null.
if (knots == null) failWithMessage("Expecting knots parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getKnots(), knots);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's knots contains the given Double elements in Collection.
* @param knots the given elements that should be contained in actual VecNURBSCurve's knots.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's knots does not contain all given Double elements.
*/
public S hasKnots(java.util.Collection extends Double> knots) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given Double collection is not null.
if (knots == null) {
failWithMessage("Expecting knots parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getKnots(), knots.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's knots contains only the given Double elements and nothing else in whatever order.
* @param knots the given elements that should be contained in actual VecNURBSCurve's knots.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's knots does not contain all given Double elements.
*/
public S hasOnlyKnots(Double... knots) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given Double varargs is not null.
if (knots == null) failWithMessage("Expecting knots parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getKnots(), knots);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's knots contains only the given Double elements in Collection and nothing else in whatever order.
* @param knots the given elements that should be contained in actual VecNURBSCurve's knots.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's knots does not contain all given Double elements.
*/
public S hasOnlyKnots(java.util.Collection extends Double> knots) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given Double collection is not null.
if (knots == null) {
failWithMessage("Expecting knots parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getKnots(), knots.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's knots does not contain the given Double elements.
*
* @param knots the given elements that should not be in actual VecNURBSCurve's knots.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's knots contains any given Double elements.
*/
public S doesNotHaveKnots(Double... knots) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given Double varargs is not null.
if (knots == null) failWithMessage("Expecting knots parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getKnots(), knots);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve's knots does not contain the given Double elements in Collection.
*
* @param knots the given elements that should not be in actual VecNURBSCurve's knots.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's knots contains any given Double elements.
*/
public S doesNotHaveKnots(java.util.Collection extends Double> knots) {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// check that given Double collection is not null.
if (knots == null) {
failWithMessage("Expecting knots parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getKnots(), knots.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNURBSCurve has no knots.
* @return this assertion object.
* @throws AssertionError if the actual VecNURBSCurve's knots is not empty.
*/
public S hasNoKnots() {
// check that actual VecNURBSCurve we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have knots but had :\n <%s>";
// check
if (actual.getKnots().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getKnots());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy