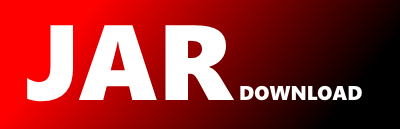
com.foursoft.vecmodel.vec120.AbstractVecNetSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
/**
* Abstract base class for {@link VecNetSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecNetSpecificationAssert, A extends VecNetSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecNetSpecificationAssert}
to make assertions on actual VecNetSpecification.
* @param actual the VecNetSpecification we want to make assertions on.
*/
protected AbstractVecNetSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecNetSpecification's netGroups contains the given VecNetGroup elements.
* @param netGroups the given elements that should be contained in actual VecNetSpecification's netGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netGroups does not contain all given VecNetGroup elements.
*/
public S hasNetGroups(VecNetGroup... netGroups) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetGroup varargs is not null.
if (netGroups == null) failWithMessage("Expecting netGroups parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNetGroups(), netGroups);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netGroups contains the given VecNetGroup elements in Collection.
* @param netGroups the given elements that should be contained in actual VecNetSpecification's netGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netGroups does not contain all given VecNetGroup elements.
*/
public S hasNetGroups(java.util.Collection extends VecNetGroup> netGroups) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetGroup collection is not null.
if (netGroups == null) {
failWithMessage("Expecting netGroups parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNetGroups(), netGroups.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netGroups contains only the given VecNetGroup elements and nothing else in whatever order.
* @param netGroups the given elements that should be contained in actual VecNetSpecification's netGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netGroups does not contain all given VecNetGroup elements.
*/
public S hasOnlyNetGroups(VecNetGroup... netGroups) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetGroup varargs is not null.
if (netGroups == null) failWithMessage("Expecting netGroups parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNetGroups(), netGroups);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netGroups contains only the given VecNetGroup elements in Collection and nothing else in whatever order.
* @param netGroups the given elements that should be contained in actual VecNetSpecification's netGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netGroups does not contain all given VecNetGroup elements.
*/
public S hasOnlyNetGroups(java.util.Collection extends VecNetGroup> netGroups) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetGroup collection is not null.
if (netGroups == null) {
failWithMessage("Expecting netGroups parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNetGroups(), netGroups.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netGroups does not contain the given VecNetGroup elements.
*
* @param netGroups the given elements that should not be in actual VecNetSpecification's netGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netGroups contains any given VecNetGroup elements.
*/
public S doesNotHaveNetGroups(VecNetGroup... netGroups) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetGroup varargs is not null.
if (netGroups == null) failWithMessage("Expecting netGroups parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNetGroups(), netGroups);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netGroups does not contain the given VecNetGroup elements in Collection.
*
* @param netGroups the given elements that should not be in actual VecNetSpecification's netGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netGroups contains any given VecNetGroup elements.
*/
public S doesNotHaveNetGroups(java.util.Collection extends VecNetGroup> netGroups) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetGroup collection is not null.
if (netGroups == null) {
failWithMessage("Expecting netGroups parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNetGroups(), netGroups.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification has no netGroups.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netGroups is not empty.
*/
public S hasNoNetGroups() {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have netGroups but had :\n <%s>";
// check
if (actual.getNetGroups().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getNetGroups());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netTypes contains the given VecNetType elements.
* @param netTypes the given elements that should be contained in actual VecNetSpecification's netTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netTypes does not contain all given VecNetType elements.
*/
public S hasNetTypes(VecNetType... netTypes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetType varargs is not null.
if (netTypes == null) failWithMessage("Expecting netTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNetTypes(), netTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netTypes contains the given VecNetType elements in Collection.
* @param netTypes the given elements that should be contained in actual VecNetSpecification's netTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netTypes does not contain all given VecNetType elements.
*/
public S hasNetTypes(java.util.Collection extends VecNetType> netTypes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetType collection is not null.
if (netTypes == null) {
failWithMessage("Expecting netTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNetTypes(), netTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netTypes contains only the given VecNetType elements and nothing else in whatever order.
* @param netTypes the given elements that should be contained in actual VecNetSpecification's netTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netTypes does not contain all given VecNetType elements.
*/
public S hasOnlyNetTypes(VecNetType... netTypes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetType varargs is not null.
if (netTypes == null) failWithMessage("Expecting netTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNetTypes(), netTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netTypes contains only the given VecNetType elements in Collection and nothing else in whatever order.
* @param netTypes the given elements that should be contained in actual VecNetSpecification's netTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netTypes does not contain all given VecNetType elements.
*/
public S hasOnlyNetTypes(java.util.Collection extends VecNetType> netTypes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetType collection is not null.
if (netTypes == null) {
failWithMessage("Expecting netTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNetTypes(), netTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netTypes does not contain the given VecNetType elements.
*
* @param netTypes the given elements that should not be in actual VecNetSpecification's netTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netTypes contains any given VecNetType elements.
*/
public S doesNotHaveNetTypes(VecNetType... netTypes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetType varargs is not null.
if (netTypes == null) failWithMessage("Expecting netTypes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNetTypes(), netTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's netTypes does not contain the given VecNetType elements in Collection.
*
* @param netTypes the given elements that should not be in actual VecNetSpecification's netTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netTypes contains any given VecNetType elements.
*/
public S doesNotHaveNetTypes(java.util.Collection extends VecNetType> netTypes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetType collection is not null.
if (netTypes == null) {
failWithMessage("Expecting netTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNetTypes(), netTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification has no netTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's netTypes is not empty.
*/
public S hasNoNetTypes() {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have netTypes but had :\n <%s>";
// check
if (actual.getNetTypes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getNetTypes());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's nets contains the given VecNet elements.
* @param nets the given elements that should be contained in actual VecNetSpecification's nets.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's nets does not contain all given VecNet elements.
*/
public S hasNets(VecNet... nets) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNet varargs is not null.
if (nets == null) failWithMessage("Expecting nets parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNets(), nets);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's nets contains the given VecNet elements in Collection.
* @param nets the given elements that should be contained in actual VecNetSpecification's nets.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's nets does not contain all given VecNet elements.
*/
public S hasNets(java.util.Collection extends VecNet> nets) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNet collection is not null.
if (nets == null) {
failWithMessage("Expecting nets parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNets(), nets.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's nets contains only the given VecNet elements and nothing else in whatever order.
* @param nets the given elements that should be contained in actual VecNetSpecification's nets.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's nets does not contain all given VecNet elements.
*/
public S hasOnlyNets(VecNet... nets) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNet varargs is not null.
if (nets == null) failWithMessage("Expecting nets parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNets(), nets);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's nets contains only the given VecNet elements in Collection and nothing else in whatever order.
* @param nets the given elements that should be contained in actual VecNetSpecification's nets.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's nets does not contain all given VecNet elements.
*/
public S hasOnlyNets(java.util.Collection extends VecNet> nets) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNet collection is not null.
if (nets == null) {
failWithMessage("Expecting nets parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNets(), nets.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's nets does not contain the given VecNet elements.
*
* @param nets the given elements that should not be in actual VecNetSpecification's nets.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's nets contains any given VecNet elements.
*/
public S doesNotHaveNets(VecNet... nets) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNet varargs is not null.
if (nets == null) failWithMessage("Expecting nets parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNets(), nets);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's nets does not contain the given VecNet elements in Collection.
*
* @param nets the given elements that should not be in actual VecNetSpecification's nets.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's nets contains any given VecNet elements.
*/
public S doesNotHaveNets(java.util.Collection extends VecNet> nets) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNet collection is not null.
if (nets == null) {
failWithMessage("Expecting nets parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNets(), nets.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification has no nets.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's nets is not empty.
*/
public S hasNoNets() {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have nets but had :\n <%s>";
// check
if (actual.getNets().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getNets());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's networkNodes contains the given VecNetworkNode elements.
* @param networkNodes the given elements that should be contained in actual VecNetSpecification's networkNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's networkNodes does not contain all given VecNetworkNode elements.
*/
public S hasNetworkNodes(VecNetworkNode... networkNodes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode varargs is not null.
if (networkNodes == null) failWithMessage("Expecting networkNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNetworkNodes(), networkNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's networkNodes contains the given VecNetworkNode elements in Collection.
* @param networkNodes the given elements that should be contained in actual VecNetSpecification's networkNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's networkNodes does not contain all given VecNetworkNode elements.
*/
public S hasNetworkNodes(java.util.Collection extends VecNetworkNode> networkNodes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode collection is not null.
if (networkNodes == null) {
failWithMessage("Expecting networkNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getNetworkNodes(), networkNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's networkNodes contains only the given VecNetworkNode elements and nothing else in whatever order.
* @param networkNodes the given elements that should be contained in actual VecNetSpecification's networkNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's networkNodes does not contain all given VecNetworkNode elements.
*/
public S hasOnlyNetworkNodes(VecNetworkNode... networkNodes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode varargs is not null.
if (networkNodes == null) failWithMessage("Expecting networkNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNetworkNodes(), networkNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's networkNodes contains only the given VecNetworkNode elements in Collection and nothing else in whatever order.
* @param networkNodes the given elements that should be contained in actual VecNetSpecification's networkNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's networkNodes does not contain all given VecNetworkNode elements.
*/
public S hasOnlyNetworkNodes(java.util.Collection extends VecNetworkNode> networkNodes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode collection is not null.
if (networkNodes == null) {
failWithMessage("Expecting networkNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getNetworkNodes(), networkNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's networkNodes does not contain the given VecNetworkNode elements.
*
* @param networkNodes the given elements that should not be in actual VecNetSpecification's networkNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's networkNodes contains any given VecNetworkNode elements.
*/
public S doesNotHaveNetworkNodes(VecNetworkNode... networkNodes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode varargs is not null.
if (networkNodes == null) failWithMessage("Expecting networkNodes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNetworkNodes(), networkNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification's networkNodes does not contain the given VecNetworkNode elements in Collection.
*
* @param networkNodes the given elements that should not be in actual VecNetSpecification's networkNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's networkNodes contains any given VecNetworkNode elements.
*/
public S doesNotHaveNetworkNodes(java.util.Collection extends VecNetworkNode> networkNodes) {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode collection is not null.
if (networkNodes == null) {
failWithMessage("Expecting networkNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getNetworkNodes(), networkNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNetSpecification has no networkNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecNetSpecification's networkNodes is not empty.
*/
public S hasNoNetworkNodes() {
// check that actual VecNetSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have networkNodes but had :\n <%s>";
// check
if (actual.getNetworkNodes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getNetworkNodes());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy