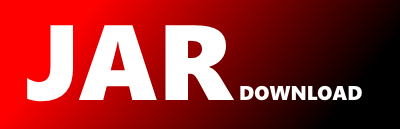
com.foursoft.vecmodel.vec120.AbstractVecNumericalValueAssert Maven / Gradle / Ivy
Show all versions of vec120-assertions Show documentation
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.Assertions;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecNumericalValue} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecNumericalValueAssert, A extends VecNumericalValue> extends AbstractVecValueWithUnitAssert {
/**
* Creates a new {@link AbstractVecNumericalValueAssert}
to make assertions on actual VecNumericalValue.
* @param actual the VecNumericalValue we want to make assertions on.
*/
protected AbstractVecNumericalValueAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecNumericalValue's tolerance is equal to the given one.
* @param tolerance the given tolerance to compare the actual VecNumericalValue's tolerance to.
* @return this assertion object.
* @throws AssertionError - if the actual VecNumericalValue's tolerance is not equal to the given one.
*/
public S hasTolerance(VecTolerance tolerance) {
// check that actual VecNumericalValue we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting tolerance of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTolerance actualTolerance = actual.getTolerance();
if (!Objects.areEqual(actualTolerance, tolerance)) {
failWithMessage(assertjErrorMessage, actual, tolerance, actualTolerance);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNumericalValue's valueComponent is equal to the given one.
* @param valueComponent the given valueComponent to compare the actual VecNumericalValue's valueComponent to.
* @return this assertion object.
* @throws AssertionError - if the actual VecNumericalValue's valueComponent is not equal to the given one.
*/
public S hasValueComponent(double valueComponent) {
// check that actual VecNumericalValue we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting valueComponent of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for valueComponent
double actualValueComponent = actual.getValueComponent();
if (actualValueComponent != valueComponent) {
failWithMessage(assertjErrorMessage, actual, valueComponent, actualValueComponent);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecNumericalValue's valueComponent is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param valueComponent the value to compare the actual VecNumericalValue's valueComponent to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecNumericalValue's valueComponent is not close enough to the given value.
*/
public S hasValueComponentCloseTo(double valueComponent, double assertjOffset) {
// check that actual VecNumericalValue we want to make assertions on is not null.
isNotNull();
double actualValueComponent = actual.getValueComponent();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting valueComponent:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualValueComponent, valueComponent, assertjOffset, Math.abs(valueComponent - actualValueComponent));
// check
Assertions.assertThat(actualValueComponent).overridingErrorMessage(assertjErrorMessage).isCloseTo(valueComponent, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
}