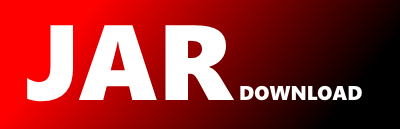
com.foursoft.vecmodel.vec120.AbstractVecPartOccurrenceAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecPartOccurrence} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPartOccurrenceAssert, A extends VecPartOccurrence> extends AbstractVecOccurrenceOrUsageAssert {
/**
* Creates a new {@link AbstractVecPartOccurrenceAssert}
to make assertions on actual VecPartOccurrence.
* @param actual the VecPartOccurrence we want to make assertions on.
*/
protected AbstractVecPartOccurrenceAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPartOccurrence's alternativeOccurrence contains the given VecPartOccurrence elements.
* @param alternativeOccurrence the given elements that should be contained in actual VecPartOccurrence's alternativeOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's alternativeOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasAlternativeOccurrence(VecPartOccurrence... alternativeOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (alternativeOccurrence == null) failWithMessage("Expecting alternativeOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAlternativeOccurrence(), alternativeOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's alternativeOccurrence contains the given VecPartOccurrence elements in Collection.
* @param alternativeOccurrence the given elements that should be contained in actual VecPartOccurrence's alternativeOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's alternativeOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasAlternativeOccurrence(java.util.Collection extends VecPartOccurrence> alternativeOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (alternativeOccurrence == null) {
failWithMessage("Expecting alternativeOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAlternativeOccurrence(), alternativeOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's alternativeOccurrence contains only the given VecPartOccurrence elements and nothing else in whatever order.
* @param alternativeOccurrence the given elements that should be contained in actual VecPartOccurrence's alternativeOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's alternativeOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyAlternativeOccurrence(VecPartOccurrence... alternativeOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (alternativeOccurrence == null) failWithMessage("Expecting alternativeOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAlternativeOccurrence(), alternativeOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's alternativeOccurrence contains only the given VecPartOccurrence elements in Collection and nothing else in whatever order.
* @param alternativeOccurrence the given elements that should be contained in actual VecPartOccurrence's alternativeOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's alternativeOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyAlternativeOccurrence(java.util.Collection extends VecPartOccurrence> alternativeOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (alternativeOccurrence == null) {
failWithMessage("Expecting alternativeOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAlternativeOccurrence(), alternativeOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's alternativeOccurrence does not contain the given VecPartOccurrence elements.
*
* @param alternativeOccurrence the given elements that should not be in actual VecPartOccurrence's alternativeOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's alternativeOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveAlternativeOccurrence(VecPartOccurrence... alternativeOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (alternativeOccurrence == null) failWithMessage("Expecting alternativeOccurrence parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAlternativeOccurrence(), alternativeOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's alternativeOccurrence does not contain the given VecPartOccurrence elements in Collection.
*
* @param alternativeOccurrence the given elements that should not be in actual VecPartOccurrence's alternativeOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's alternativeOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveAlternativeOccurrence(java.util.Collection extends VecPartOccurrence> alternativeOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (alternativeOccurrence == null) {
failWithMessage("Expecting alternativeOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAlternativeOccurrence(), alternativeOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence has no alternativeOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's alternativeOccurrence is not empty.
*/
public S hasNoAlternativeOccurrence() {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have alternativeOccurrence but had :\n <%s>";
// check
if (actual.getAlternativeOccurrence().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAlternativeOccurrence());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's instanciatedOccurrence contains the given VecPartOccurrence elements.
* @param instanciatedOccurrence the given elements that should be contained in actual VecPartOccurrence's instanciatedOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's instanciatedOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasInstanciatedOccurrence(VecPartOccurrence... instanciatedOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (instanciatedOccurrence == null) failWithMessage("Expecting instanciatedOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInstanciatedOccurrence(), instanciatedOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's instanciatedOccurrence contains the given VecPartOccurrence elements in Collection.
* @param instanciatedOccurrence the given elements that should be contained in actual VecPartOccurrence's instanciatedOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's instanciatedOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasInstanciatedOccurrence(java.util.Collection extends VecPartOccurrence> instanciatedOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (instanciatedOccurrence == null) {
failWithMessage("Expecting instanciatedOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInstanciatedOccurrence(), instanciatedOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's instanciatedOccurrence contains only the given VecPartOccurrence elements and nothing else in whatever order.
* @param instanciatedOccurrence the given elements that should be contained in actual VecPartOccurrence's instanciatedOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's instanciatedOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyInstanciatedOccurrence(VecPartOccurrence... instanciatedOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (instanciatedOccurrence == null) failWithMessage("Expecting instanciatedOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInstanciatedOccurrence(), instanciatedOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's instanciatedOccurrence contains only the given VecPartOccurrence elements in Collection and nothing else in whatever order.
* @param instanciatedOccurrence the given elements that should be contained in actual VecPartOccurrence's instanciatedOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's instanciatedOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyInstanciatedOccurrence(java.util.Collection extends VecPartOccurrence> instanciatedOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (instanciatedOccurrence == null) {
failWithMessage("Expecting instanciatedOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInstanciatedOccurrence(), instanciatedOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's instanciatedOccurrence does not contain the given VecPartOccurrence elements.
*
* @param instanciatedOccurrence the given elements that should not be in actual VecPartOccurrence's instanciatedOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's instanciatedOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveInstanciatedOccurrence(VecPartOccurrence... instanciatedOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (instanciatedOccurrence == null) failWithMessage("Expecting instanciatedOccurrence parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInstanciatedOccurrence(), instanciatedOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's instanciatedOccurrence does not contain the given VecPartOccurrence elements in Collection.
*
* @param instanciatedOccurrence the given elements that should not be in actual VecPartOccurrence's instanciatedOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's instanciatedOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveInstanciatedOccurrence(java.util.Collection extends VecPartOccurrence> instanciatedOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (instanciatedOccurrence == null) {
failWithMessage("Expecting instanciatedOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInstanciatedOccurrence(), instanciatedOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence has no instanciatedOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's instanciatedOccurrence is not empty.
*/
public S hasNoInstanciatedOccurrence() {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have instanciatedOccurrence but had :\n <%s>";
// check
if (actual.getInstanciatedOccurrence().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getInstanciatedOccurrence());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence is is secondary alternative.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartOccurrence is not is secondary alternative.
*/
public S isIsSecondaryAlternative() {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isIsSecondaryAlternative())) {
failWithMessage("\nExpecting that actual VecPartOccurrence is is secondary alternative but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence is not is secondary alternative.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartOccurrence is is secondary alternative.
*/
public S isNotIsSecondaryAlternative() {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isIsSecondaryAlternative())) {
failWithMessage("\nExpecting that actual VecPartOccurrence is not is secondary alternative but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's parentCompositionSpecification is equal to the given one.
* @param parentCompositionSpecification the given parentCompositionSpecification to compare the actual VecPartOccurrence's parentCompositionSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartOccurrence's parentCompositionSpecification is not equal to the given one.
*/
public S hasParentCompositionSpecification(VecCompositionSpecification parentCompositionSpecification) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentCompositionSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCompositionSpecification actualParentCompositionSpecification = actual.getParentCompositionSpecification();
if (!Objects.areEqual(actualParentCompositionSpecification, parentCompositionSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentCompositionSpecification, actualParentCompositionSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's part is equal to the given one.
* @param part the given part to compare the actual VecPartOccurrence's part to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartOccurrence's part is not equal to the given one.
*/
public S hasPart(VecPartVersion part) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting part of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPartVersion actualPart = actual.getPart();
if (!Objects.areEqual(actualPart, part)) {
failWithMessage(assertjErrorMessage, actual, part, actualPart);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's realizedPartUsage contains the given VecPartUsage elements.
* @param realizedPartUsage the given elements that should be contained in actual VecPartOccurrence's realizedPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's realizedPartUsage does not contain all given VecPartUsage elements.
*/
public S hasRealizedPartUsage(VecPartUsage... realizedPartUsage) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (realizedPartUsage == null) failWithMessage("Expecting realizedPartUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRealizedPartUsage(), realizedPartUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's realizedPartUsage contains the given VecPartUsage elements in Collection.
* @param realizedPartUsage the given elements that should be contained in actual VecPartOccurrence's realizedPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's realizedPartUsage does not contain all given VecPartUsage elements.
*/
public S hasRealizedPartUsage(java.util.Collection extends VecPartUsage> realizedPartUsage) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (realizedPartUsage == null) {
failWithMessage("Expecting realizedPartUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRealizedPartUsage(), realizedPartUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's realizedPartUsage contains only the given VecPartUsage elements and nothing else in whatever order.
* @param realizedPartUsage the given elements that should be contained in actual VecPartOccurrence's realizedPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's realizedPartUsage does not contain all given VecPartUsage elements.
*/
public S hasOnlyRealizedPartUsage(VecPartUsage... realizedPartUsage) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (realizedPartUsage == null) failWithMessage("Expecting realizedPartUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRealizedPartUsage(), realizedPartUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's realizedPartUsage contains only the given VecPartUsage elements in Collection and nothing else in whatever order.
* @param realizedPartUsage the given elements that should be contained in actual VecPartOccurrence's realizedPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's realizedPartUsage does not contain all given VecPartUsage elements.
*/
public S hasOnlyRealizedPartUsage(java.util.Collection extends VecPartUsage> realizedPartUsage) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (realizedPartUsage == null) {
failWithMessage("Expecting realizedPartUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRealizedPartUsage(), realizedPartUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's realizedPartUsage does not contain the given VecPartUsage elements.
*
* @param realizedPartUsage the given elements that should not be in actual VecPartOccurrence's realizedPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's realizedPartUsage contains any given VecPartUsage elements.
*/
public S doesNotHaveRealizedPartUsage(VecPartUsage... realizedPartUsage) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (realizedPartUsage == null) failWithMessage("Expecting realizedPartUsage parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRealizedPartUsage(), realizedPartUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's realizedPartUsage does not contain the given VecPartUsage elements in Collection.
*
* @param realizedPartUsage the given elements that should not be in actual VecPartOccurrence's realizedPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's realizedPartUsage contains any given VecPartUsage elements.
*/
public S doesNotHaveRealizedPartUsage(java.util.Collection extends VecPartUsage> realizedPartUsage) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (realizedPartUsage == null) {
failWithMessage("Expecting realizedPartUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRealizedPartUsage(), realizedPartUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence has no realizedPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's realizedPartUsage is not empty.
*/
public S hasNoRealizedPartUsage() {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have realizedPartUsage but had :\n <%s>";
// check
if (actual.getRealizedPartUsage().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRealizedPartUsage());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's refPartOccurrence contains the given VecPartOccurrence elements.
* @param refPartOccurrence the given elements that should be contained in actual VecPartOccurrence's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's refPartOccurrence contains the given VecPartOccurrence elements in Collection.
* @param refPartOccurrence the given elements that should be contained in actual VecPartOccurrence's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's refPartOccurrence contains only the given VecPartOccurrence elements and nothing else in whatever order.
* @param refPartOccurrence the given elements that should be contained in actual VecPartOccurrence's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's refPartOccurrence contains only the given VecPartOccurrence elements in Collection and nothing else in whatever order.
* @param refPartOccurrence the given elements that should be contained in actual VecPartOccurrence's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's refPartOccurrence does not contain the given VecPartOccurrence elements.
*
* @param refPartOccurrence the given elements that should not be in actual VecPartOccurrence's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's refPartOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence's refPartOccurrence does not contain the given VecPartOccurrence elements in Collection.
*
* @param refPartOccurrence the given elements that should not be in actual VecPartOccurrence's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's refPartOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartOccurrence has no refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartOccurrence's refPartOccurrence is not empty.
*/
public S hasNoRefPartOccurrence() {
// check that actual VecPartOccurrence we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPartOccurrence but had :\n <%s>";
// check
if (actual.getRefPartOccurrence().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPartOccurrence());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy