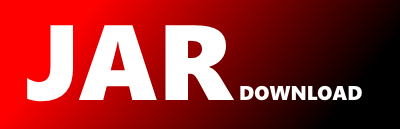
com.foursoft.vecmodel.vec120.AbstractVecPartUsageAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecPartUsage} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPartUsageAssert, A extends VecPartUsage> extends AbstractVecOccurrenceOrUsageAssert {
/**
* Creates a new {@link AbstractVecPartUsageAssert}
to make assertions on actual VecPartUsage.
* @param actual the VecPartUsage we want to make assertions on.
*/
protected AbstractVecPartUsageAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPartUsage's instanciatedUsage contains the given VecPartUsage elements.
* @param instanciatedUsage the given elements that should be contained in actual VecPartUsage's instanciatedUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's instanciatedUsage does not contain all given VecPartUsage elements.
*/
public S hasInstanciatedUsage(VecPartUsage... instanciatedUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (instanciatedUsage == null) failWithMessage("Expecting instanciatedUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInstanciatedUsage(), instanciatedUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's instanciatedUsage contains the given VecPartUsage elements in Collection.
* @param instanciatedUsage the given elements that should be contained in actual VecPartUsage's instanciatedUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's instanciatedUsage does not contain all given VecPartUsage elements.
*/
public S hasInstanciatedUsage(java.util.Collection extends VecPartUsage> instanciatedUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (instanciatedUsage == null) {
failWithMessage("Expecting instanciatedUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInstanciatedUsage(), instanciatedUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's instanciatedUsage contains only the given VecPartUsage elements and nothing else in whatever order.
* @param instanciatedUsage the given elements that should be contained in actual VecPartUsage's instanciatedUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's instanciatedUsage does not contain all given VecPartUsage elements.
*/
public S hasOnlyInstanciatedUsage(VecPartUsage... instanciatedUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (instanciatedUsage == null) failWithMessage("Expecting instanciatedUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInstanciatedUsage(), instanciatedUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's instanciatedUsage contains only the given VecPartUsage elements in Collection and nothing else in whatever order.
* @param instanciatedUsage the given elements that should be contained in actual VecPartUsage's instanciatedUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's instanciatedUsage does not contain all given VecPartUsage elements.
*/
public S hasOnlyInstanciatedUsage(java.util.Collection extends VecPartUsage> instanciatedUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (instanciatedUsage == null) {
failWithMessage("Expecting instanciatedUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInstanciatedUsage(), instanciatedUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's instanciatedUsage does not contain the given VecPartUsage elements.
*
* @param instanciatedUsage the given elements that should not be in actual VecPartUsage's instanciatedUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's instanciatedUsage contains any given VecPartUsage elements.
*/
public S doesNotHaveInstanciatedUsage(VecPartUsage... instanciatedUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (instanciatedUsage == null) failWithMessage("Expecting instanciatedUsage parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInstanciatedUsage(), instanciatedUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's instanciatedUsage does not contain the given VecPartUsage elements in Collection.
*
* @param instanciatedUsage the given elements that should not be in actual VecPartUsage's instanciatedUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's instanciatedUsage contains any given VecPartUsage elements.
*/
public S doesNotHaveInstanciatedUsage(java.util.Collection extends VecPartUsage> instanciatedUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (instanciatedUsage == null) {
failWithMessage("Expecting instanciatedUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInstanciatedUsage(), instanciatedUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage has no instanciatedUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's instanciatedUsage is not empty.
*/
public S hasNoInstanciatedUsage() {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have instanciatedUsage but had :\n <%s>";
// check
if (actual.getInstanciatedUsage().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getInstanciatedUsage());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's parentPartUsageSpecification is equal to the given one.
* @param parentPartUsageSpecification the given parentPartUsageSpecification to compare the actual VecPartUsage's parentPartUsageSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartUsage's parentPartUsageSpecification is not equal to the given one.
*/
public S hasParentPartUsageSpecification(VecPartUsageSpecification parentPartUsageSpecification) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentPartUsageSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPartUsageSpecification actualParentPartUsageSpecification = actual.getParentPartUsageSpecification();
if (!Objects.areEqual(actualParentPartUsageSpecification, parentPartUsageSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentPartUsageSpecification, actualParentPartUsageSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's partOrUsageRelatedSpecification contains the given VecPartOrUsageRelatedSpecification elements.
* @param partOrUsageRelatedSpecification the given elements that should be contained in actual VecPartUsage's partOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's partOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasPartOrUsageRelatedSpecification(VecPartOrUsageRelatedSpecification... partOrUsageRelatedSpecification) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification varargs is not null.
if (partOrUsageRelatedSpecification == null) failWithMessage("Expecting partOrUsageRelatedSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPartOrUsageRelatedSpecification(), partOrUsageRelatedSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's partOrUsageRelatedSpecification contains the given VecPartOrUsageRelatedSpecification elements in Collection.
* @param partOrUsageRelatedSpecification the given elements that should be contained in actual VecPartUsage's partOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's partOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasPartOrUsageRelatedSpecification(java.util.Collection extends VecPartOrUsageRelatedSpecification> partOrUsageRelatedSpecification) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification collection is not null.
if (partOrUsageRelatedSpecification == null) {
failWithMessage("Expecting partOrUsageRelatedSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPartOrUsageRelatedSpecification(), partOrUsageRelatedSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's partOrUsageRelatedSpecification contains only the given VecPartOrUsageRelatedSpecification elements and nothing else in whatever order.
* @param partOrUsageRelatedSpecification the given elements that should be contained in actual VecPartUsage's partOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's partOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasOnlyPartOrUsageRelatedSpecification(VecPartOrUsageRelatedSpecification... partOrUsageRelatedSpecification) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification varargs is not null.
if (partOrUsageRelatedSpecification == null) failWithMessage("Expecting partOrUsageRelatedSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPartOrUsageRelatedSpecification(), partOrUsageRelatedSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's partOrUsageRelatedSpecification contains only the given VecPartOrUsageRelatedSpecification elements in Collection and nothing else in whatever order.
* @param partOrUsageRelatedSpecification the given elements that should be contained in actual VecPartUsage's partOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's partOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasOnlyPartOrUsageRelatedSpecification(java.util.Collection extends VecPartOrUsageRelatedSpecification> partOrUsageRelatedSpecification) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification collection is not null.
if (partOrUsageRelatedSpecification == null) {
failWithMessage("Expecting partOrUsageRelatedSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPartOrUsageRelatedSpecification(), partOrUsageRelatedSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's partOrUsageRelatedSpecification does not contain the given VecPartOrUsageRelatedSpecification elements.
*
* @param partOrUsageRelatedSpecification the given elements that should not be in actual VecPartUsage's partOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's partOrUsageRelatedSpecification contains any given VecPartOrUsageRelatedSpecification elements.
*/
public S doesNotHavePartOrUsageRelatedSpecification(VecPartOrUsageRelatedSpecification... partOrUsageRelatedSpecification) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification varargs is not null.
if (partOrUsageRelatedSpecification == null) failWithMessage("Expecting partOrUsageRelatedSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPartOrUsageRelatedSpecification(), partOrUsageRelatedSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's partOrUsageRelatedSpecification does not contain the given VecPartOrUsageRelatedSpecification elements in Collection.
*
* @param partOrUsageRelatedSpecification the given elements that should not be in actual VecPartUsage's partOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's partOrUsageRelatedSpecification contains any given VecPartOrUsageRelatedSpecification elements.
*/
public S doesNotHavePartOrUsageRelatedSpecification(java.util.Collection extends VecPartOrUsageRelatedSpecification> partOrUsageRelatedSpecification) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification collection is not null.
if (partOrUsageRelatedSpecification == null) {
failWithMessage("Expecting partOrUsageRelatedSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPartOrUsageRelatedSpecification(), partOrUsageRelatedSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage has no partOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's partOrUsageRelatedSpecification is not empty.
*/
public S hasNoPartOrUsageRelatedSpecification() {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have partOrUsageRelatedSpecification but had :\n <%s>";
// check
if (actual.getPartOrUsageRelatedSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPartOrUsageRelatedSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's partSubstitution is equal to the given one.
* @param partSubstitution the given partSubstitution to compare the actual VecPartUsage's partSubstitution to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartUsage's partSubstitution is not equal to the given one.
*/
public S hasPartSubstitution(VecPartSubstitutionSpecification partSubstitution) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting partSubstitution of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPartSubstitutionSpecification actualPartSubstitution = actual.getPartSubstitution();
if (!Objects.areEqual(actualPartSubstitution, partSubstitution)) {
failWithMessage(assertjErrorMessage, actual, partSubstitution, actualPartSubstitution);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's primaryPartUsageType is equal to the given one.
* @param primaryPartUsageType the given primaryPartUsageType to compare the actual VecPartUsage's primaryPartUsageType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartUsage's primaryPartUsageType is not equal to the given one.
*/
public S hasPrimaryPartUsageType(VecPrimaryPartType primaryPartUsageType) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting primaryPartUsageType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPrimaryPartType actualPrimaryPartUsageType = actual.getPrimaryPartUsageType();
if (!Objects.areEqual(actualPrimaryPartUsageType, primaryPartUsageType)) {
failWithMessage(assertjErrorMessage, actual, primaryPartUsageType, actualPrimaryPartUsageType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartOccurrence contains the given VecPartOccurrence elements.
* @param refPartOccurrence the given elements that should be contained in actual VecPartUsage's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartOccurrence contains the given VecPartOccurrence elements in Collection.
* @param refPartOccurrence the given elements that should be contained in actual VecPartUsage's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartOccurrence contains only the given VecPartOccurrence elements and nothing else in whatever order.
* @param refPartOccurrence the given elements that should be contained in actual VecPartUsage's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartOccurrence contains only the given VecPartOccurrence elements in Collection and nothing else in whatever order.
* @param refPartOccurrence the given elements that should be contained in actual VecPartUsage's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartOccurrence does not contain the given VecPartOccurrence elements.
*
* @param refPartOccurrence the given elements that should not be in actual VecPartUsage's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartOccurrence does not contain the given VecPartOccurrence elements in Collection.
*
* @param refPartOccurrence the given elements that should not be in actual VecPartUsage's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage has no refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartOccurrence is not empty.
*/
public S hasNoRefPartOccurrence() {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPartOccurrence but had :\n <%s>";
// check
if (actual.getRefPartOccurrence().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPartOccurrence());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartUsage contains the given VecPartUsage elements.
* @param refPartUsage the given elements that should be contained in actual VecPartUsage's refPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartUsage does not contain all given VecPartUsage elements.
*/
public S hasRefPartUsage(VecPartUsage... refPartUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (refPartUsage == null) failWithMessage("Expecting refPartUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartUsage(), refPartUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartUsage contains the given VecPartUsage elements in Collection.
* @param refPartUsage the given elements that should be contained in actual VecPartUsage's refPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartUsage does not contain all given VecPartUsage elements.
*/
public S hasRefPartUsage(java.util.Collection extends VecPartUsage> refPartUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (refPartUsage == null) {
failWithMessage("Expecting refPartUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartUsage(), refPartUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartUsage contains only the given VecPartUsage elements and nothing else in whatever order.
* @param refPartUsage the given elements that should be contained in actual VecPartUsage's refPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartUsage does not contain all given VecPartUsage elements.
*/
public S hasOnlyRefPartUsage(VecPartUsage... refPartUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (refPartUsage == null) failWithMessage("Expecting refPartUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartUsage(), refPartUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartUsage contains only the given VecPartUsage elements in Collection and nothing else in whatever order.
* @param refPartUsage the given elements that should be contained in actual VecPartUsage's refPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartUsage does not contain all given VecPartUsage elements.
*/
public S hasOnlyRefPartUsage(java.util.Collection extends VecPartUsage> refPartUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (refPartUsage == null) {
failWithMessage("Expecting refPartUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartUsage(), refPartUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartUsage does not contain the given VecPartUsage elements.
*
* @param refPartUsage the given elements that should not be in actual VecPartUsage's refPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartUsage contains any given VecPartUsage elements.
*/
public S doesNotHaveRefPartUsage(VecPartUsage... refPartUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage varargs is not null.
if (refPartUsage == null) failWithMessage("Expecting refPartUsage parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartUsage(), refPartUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage's refPartUsage does not contain the given VecPartUsage elements in Collection.
*
* @param refPartUsage the given elements that should not be in actual VecPartUsage's refPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartUsage contains any given VecPartUsage elements.
*/
public S doesNotHaveRefPartUsage(java.util.Collection extends VecPartUsage> refPartUsage) {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// check that given VecPartUsage collection is not null.
if (refPartUsage == null) {
failWithMessage("Expecting refPartUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartUsage(), refPartUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartUsage has no refPartUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecPartUsage's refPartUsage is not empty.
*/
public S hasNoRefPartUsage() {
// check that actual VecPartUsage we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPartUsage but had :\n <%s>";
// check
if (actual.getRefPartUsage().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPartUsage());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy