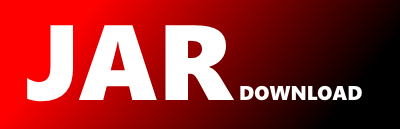
com.foursoft.vecmodel.vec120.AbstractVecPartVersionAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecPartVersion} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPartVersionAssert, A extends VecPartVersion> extends AbstractVecItemVersionAssert {
/**
* Creates a new {@link AbstractVecPartVersionAssert}
to make assertions on actual VecPartVersion.
* @param actual the VecPartVersion we want to make assertions on.
*/
protected AbstractVecPartVersionAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPartVersion's aliasIds contains the given VecAliasIdentification elements.
* @param aliasIds the given elements that should be contained in actual VecPartVersion's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's aliasIds contains the given VecAliasIdentification elements in Collection.
* @param aliasIds the given elements that should be contained in actual VecPartVersion's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's aliasIds contains only the given VecAliasIdentification elements and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecPartVersion's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's aliasIds contains only the given VecAliasIdentification elements in Collection and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecPartVersion's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's aliasIds does not contain the given VecAliasIdentification elements.
*
* @param aliasIds the given elements that should not be in actual VecPartVersion's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's aliasIds does not contain the given VecAliasIdentification elements in Collection.
*
* @param aliasIds the given elements that should not be in actual VecPartVersion's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's aliasIds is not empty.
*/
public S hasNoAliasIds() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have aliasIds but had :\n <%s>";
// check
if (actual.getAliasIds().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAliasIds());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's isPreferredPart is equal to the given one.
* @param isPreferredPart the given isPreferredPart to compare the actual VecPartVersion's isPreferredPart to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's isPreferredPart is not equal to the given one.
*/
public S hasIsPreferredPart(String isPreferredPart) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting isPreferredPart of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIsPreferredPart = actual.getIsPreferredPart();
if (!Objects.areEqual(actualIsPreferredPart, isPreferredPart)) {
failWithMessage(assertjErrorMessage, actual, isPreferredPart, actualIsPreferredPart);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's nature is equal to the given one.
* @param nature the given nature to compare the actual VecPartVersion's nature to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's nature is not equal to the given one.
*/
public S hasNature(String nature) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting nature of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualNature = actual.getNature();
if (!Objects.areEqual(actualNature, nature)) {
failWithMessage(assertjErrorMessage, actual, nature, actualNature);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's parentVecContent is equal to the given one.
* @param parentVecContent the given parentVecContent to compare the actual VecPartVersion's parentVecContent to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's parentVecContent is not equal to the given one.
*/
public S hasParentVecContent(VecContent parentVecContent) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentVecContent of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecContent actualParentVecContent = actual.getParentVecContent();
if (!Objects.areEqual(actualParentVecContent, parentVecContent)) {
failWithMessage(assertjErrorMessage, actual, parentVecContent, actualParentVecContent);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's partNumber is equal to the given one.
* @param partNumber the given partNumber to compare the actual VecPartVersion's partNumber to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's partNumber is not equal to the given one.
*/
public S hasPartNumber(String partNumber) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting partNumber of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualPartNumber = actual.getPartNumber();
if (!Objects.areEqual(actualPartNumber, partNumber)) {
failWithMessage(assertjErrorMessage, actual, partNumber, actualPartNumber);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's partVersion is equal to the given one.
* @param partVersion the given partVersion to compare the actual VecPartVersion's partVersion to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's partVersion is not equal to the given one.
*/
public S hasPartVersion(String partVersion) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting partVersion of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualPartVersion = actual.getPartVersion();
if (!Objects.areEqual(actualPartVersion, partVersion)) {
failWithMessage(assertjErrorMessage, actual, partVersion, actualPartVersion);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's preferredUseCase is equal to the given one.
* @param preferredUseCase the given preferredUseCase to compare the actual VecPartVersion's preferredUseCase to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's preferredUseCase is not equal to the given one.
*/
public S hasPreferredUseCase(String preferredUseCase) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting preferredUseCase of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualPreferredUseCase = actual.getPreferredUseCase();
if (!Objects.areEqual(actualPreferredUseCase, preferredUseCase)) {
failWithMessage(assertjErrorMessage, actual, preferredUseCase, actualPreferredUseCase);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's primaryPartType is equal to the given one.
* @param primaryPartType the given primaryPartType to compare the actual VecPartVersion's primaryPartType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's primaryPartType is not equal to the given one.
*/
public S hasPrimaryPartType(VecPrimaryPartType primaryPartType) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting primaryPartType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPrimaryPartType actualPrimaryPartType = actual.getPrimaryPartType();
if (!Objects.areEqual(actualPrimaryPartType, primaryPartType)) {
failWithMessage(assertjErrorMessage, actual, primaryPartType, actualPrimaryPartType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's project is equal to the given one.
* @param project the given project to compare the actual VecPartVersion's project to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPartVersion's project is not equal to the given one.
*/
public S hasProject(VecProject project) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting project of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecProject actualProject = actual.getProject();
if (!Objects.areEqual(actualProject, project)) {
failWithMessage(assertjErrorMessage, actual, project, actualProject);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refDocumentVersion contains the given VecDocumentVersion elements.
* @param refDocumentVersion the given elements that should be contained in actual VecPartVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasRefDocumentVersion(VecDocumentVersion... refDocumentVersion) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (refDocumentVersion == null) failWithMessage("Expecting refDocumentVersion parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentVersion(), refDocumentVersion);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refDocumentVersion contains the given VecDocumentVersion elements in Collection.
* @param refDocumentVersion the given elements that should be contained in actual VecPartVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasRefDocumentVersion(java.util.Collection extends VecDocumentVersion> refDocumentVersion) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (refDocumentVersion == null) {
failWithMessage("Expecting refDocumentVersion parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentVersion(), refDocumentVersion.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refDocumentVersion contains only the given VecDocumentVersion elements and nothing else in whatever order.
* @param refDocumentVersion the given elements that should be contained in actual VecPartVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasOnlyRefDocumentVersion(VecDocumentVersion... refDocumentVersion) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (refDocumentVersion == null) failWithMessage("Expecting refDocumentVersion parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentVersion(), refDocumentVersion);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refDocumentVersion contains only the given VecDocumentVersion elements in Collection and nothing else in whatever order.
* @param refDocumentVersion the given elements that should be contained in actual VecPartVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refDocumentVersion does not contain all given VecDocumentVersion elements.
*/
public S hasOnlyRefDocumentVersion(java.util.Collection extends VecDocumentVersion> refDocumentVersion) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (refDocumentVersion == null) {
failWithMessage("Expecting refDocumentVersion parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentVersion(), refDocumentVersion.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refDocumentVersion does not contain the given VecDocumentVersion elements.
*
* @param refDocumentVersion the given elements that should not be in actual VecPartVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refDocumentVersion contains any given VecDocumentVersion elements.
*/
public S doesNotHaveRefDocumentVersion(VecDocumentVersion... refDocumentVersion) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion varargs is not null.
if (refDocumentVersion == null) failWithMessage("Expecting refDocumentVersion parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentVersion(), refDocumentVersion);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refDocumentVersion does not contain the given VecDocumentVersion elements in Collection.
*
* @param refDocumentVersion the given elements that should not be in actual VecPartVersion's refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refDocumentVersion contains any given VecDocumentVersion elements.
*/
public S doesNotHaveRefDocumentVersion(java.util.Collection extends VecDocumentVersion> refDocumentVersion) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentVersion collection is not null.
if (refDocumentVersion == null) {
failWithMessage("Expecting refDocumentVersion parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentVersion(), refDocumentVersion.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refDocumentVersion.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refDocumentVersion is not empty.
*/
public S hasNoRefDocumentVersion() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDocumentVersion but had :\n <%s>";
// check
if (actual.getRefDocumentVersion().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDocumentVersion());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refMapping contains the given VecMapping elements.
* @param refMapping the given elements that should be contained in actual VecPartVersion's refMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refMapping does not contain all given VecMapping elements.
*/
public S hasRefMapping(VecMapping... refMapping) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecMapping varargs is not null.
if (refMapping == null) failWithMessage("Expecting refMapping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMapping(), refMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refMapping contains the given VecMapping elements in Collection.
* @param refMapping the given elements that should be contained in actual VecPartVersion's refMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refMapping does not contain all given VecMapping elements.
*/
public S hasRefMapping(java.util.Collection extends VecMapping> refMapping) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecMapping collection is not null.
if (refMapping == null) {
failWithMessage("Expecting refMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMapping(), refMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refMapping contains only the given VecMapping elements and nothing else in whatever order.
* @param refMapping the given elements that should be contained in actual VecPartVersion's refMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refMapping does not contain all given VecMapping elements.
*/
public S hasOnlyRefMapping(VecMapping... refMapping) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecMapping varargs is not null.
if (refMapping == null) failWithMessage("Expecting refMapping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMapping(), refMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refMapping contains only the given VecMapping elements in Collection and nothing else in whatever order.
* @param refMapping the given elements that should be contained in actual VecPartVersion's refMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refMapping does not contain all given VecMapping elements.
*/
public S hasOnlyRefMapping(java.util.Collection extends VecMapping> refMapping) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecMapping collection is not null.
if (refMapping == null) {
failWithMessage("Expecting refMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMapping(), refMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refMapping does not contain the given VecMapping elements.
*
* @param refMapping the given elements that should not be in actual VecPartVersion's refMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refMapping contains any given VecMapping elements.
*/
public S doesNotHaveRefMapping(VecMapping... refMapping) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecMapping varargs is not null.
if (refMapping == null) failWithMessage("Expecting refMapping parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMapping(), refMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refMapping does not contain the given VecMapping elements in Collection.
*
* @param refMapping the given elements that should not be in actual VecPartVersion's refMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refMapping contains any given VecMapping elements.
*/
public S doesNotHaveRefMapping(java.util.Collection extends VecMapping> refMapping) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecMapping collection is not null.
if (refMapping == null) {
failWithMessage("Expecting refMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMapping(), refMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refMapping is not empty.
*/
public S hasNoRefMapping() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refMapping but had :\n <%s>";
// check
if (actual.getRefMapping().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefMapping());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOccurrence contains the given VecPartOccurrence elements.
* @param refPartOccurrence the given elements that should be contained in actual VecPartVersion's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOccurrence contains the given VecPartOccurrence elements in Collection.
* @param refPartOccurrence the given elements that should be contained in actual VecPartVersion's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOccurrence contains only the given VecPartOccurrence elements and nothing else in whatever order.
* @param refPartOccurrence the given elements that should be contained in actual VecPartVersion's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOccurrence contains only the given VecPartOccurrence elements in Collection and nothing else in whatever order.
* @param refPartOccurrence the given elements that should be contained in actual VecPartVersion's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOccurrence does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOccurrence does not contain the given VecPartOccurrence elements.
*
* @param refPartOccurrence the given elements that should not be in actual VecPartVersion's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveRefPartOccurrence(VecPartOccurrence... refPartOccurrence) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (refPartOccurrence == null) failWithMessage("Expecting refPartOccurrence parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOccurrence(), refPartOccurrence);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOccurrence does not contain the given VecPartOccurrence elements in Collection.
*
* @param refPartOccurrence the given elements that should not be in actual VecPartVersion's refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOccurrence contains any given VecPartOccurrence elements.
*/
public S doesNotHaveRefPartOccurrence(java.util.Collection extends VecPartOccurrence> refPartOccurrence) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (refPartOccurrence == null) {
failWithMessage("Expecting refPartOccurrence parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOccurrence(), refPartOccurrence.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refPartOccurrence.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOccurrence is not empty.
*/
public S hasNoRefPartOccurrence() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPartOccurrence but had :\n <%s>";
// check
if (actual.getRefPartOccurrence().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPartOccurrence());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOrUsageRelatedSpecification contains the given VecPartOrUsageRelatedSpecification elements.
* @param refPartOrUsageRelatedSpecification the given elements that should be contained in actual VecPartVersion's refPartOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasRefPartOrUsageRelatedSpecification(VecPartOrUsageRelatedSpecification... refPartOrUsageRelatedSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification varargs is not null.
if (refPartOrUsageRelatedSpecification == null) failWithMessage("Expecting refPartOrUsageRelatedSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOrUsageRelatedSpecification(), refPartOrUsageRelatedSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOrUsageRelatedSpecification contains the given VecPartOrUsageRelatedSpecification elements in Collection.
* @param refPartOrUsageRelatedSpecification the given elements that should be contained in actual VecPartVersion's refPartOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasRefPartOrUsageRelatedSpecification(java.util.Collection extends VecPartOrUsageRelatedSpecification> refPartOrUsageRelatedSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification collection is not null.
if (refPartOrUsageRelatedSpecification == null) {
failWithMessage("Expecting refPartOrUsageRelatedSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartOrUsageRelatedSpecification(), refPartOrUsageRelatedSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOrUsageRelatedSpecification contains only the given VecPartOrUsageRelatedSpecification elements and nothing else in whatever order.
* @param refPartOrUsageRelatedSpecification the given elements that should be contained in actual VecPartVersion's refPartOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasOnlyRefPartOrUsageRelatedSpecification(VecPartOrUsageRelatedSpecification... refPartOrUsageRelatedSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification varargs is not null.
if (refPartOrUsageRelatedSpecification == null) failWithMessage("Expecting refPartOrUsageRelatedSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOrUsageRelatedSpecification(), refPartOrUsageRelatedSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOrUsageRelatedSpecification contains only the given VecPartOrUsageRelatedSpecification elements in Collection and nothing else in whatever order.
* @param refPartOrUsageRelatedSpecification the given elements that should be contained in actual VecPartVersion's refPartOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOrUsageRelatedSpecification does not contain all given VecPartOrUsageRelatedSpecification elements.
*/
public S hasOnlyRefPartOrUsageRelatedSpecification(java.util.Collection extends VecPartOrUsageRelatedSpecification> refPartOrUsageRelatedSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification collection is not null.
if (refPartOrUsageRelatedSpecification == null) {
failWithMessage("Expecting refPartOrUsageRelatedSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartOrUsageRelatedSpecification(), refPartOrUsageRelatedSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOrUsageRelatedSpecification does not contain the given VecPartOrUsageRelatedSpecification elements.
*
* @param refPartOrUsageRelatedSpecification the given elements that should not be in actual VecPartVersion's refPartOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOrUsageRelatedSpecification contains any given VecPartOrUsageRelatedSpecification elements.
*/
public S doesNotHaveRefPartOrUsageRelatedSpecification(VecPartOrUsageRelatedSpecification... refPartOrUsageRelatedSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification varargs is not null.
if (refPartOrUsageRelatedSpecification == null) failWithMessage("Expecting refPartOrUsageRelatedSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOrUsageRelatedSpecification(), refPartOrUsageRelatedSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartOrUsageRelatedSpecification does not contain the given VecPartOrUsageRelatedSpecification elements in Collection.
*
* @param refPartOrUsageRelatedSpecification the given elements that should not be in actual VecPartVersion's refPartOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOrUsageRelatedSpecification contains any given VecPartOrUsageRelatedSpecification elements.
*/
public S doesNotHaveRefPartOrUsageRelatedSpecification(java.util.Collection extends VecPartOrUsageRelatedSpecification> refPartOrUsageRelatedSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartOrUsageRelatedSpecification collection is not null.
if (refPartOrUsageRelatedSpecification == null) {
failWithMessage("Expecting refPartOrUsageRelatedSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartOrUsageRelatedSpecification(), refPartOrUsageRelatedSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refPartOrUsageRelatedSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartOrUsageRelatedSpecification is not empty.
*/
public S hasNoRefPartOrUsageRelatedSpecification() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPartOrUsageRelatedSpecification but had :\n <%s>";
// check
if (actual.getRefPartOrUsageRelatedSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPartOrUsageRelatedSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartRelation contains the given VecPartRelation elements.
* @param refPartRelation the given elements that should be contained in actual VecPartVersion's refPartRelation.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartRelation does not contain all given VecPartRelation elements.
*/
public S hasRefPartRelation(VecPartRelation... refPartRelation) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation varargs is not null.
if (refPartRelation == null) failWithMessage("Expecting refPartRelation parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartRelation(), refPartRelation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartRelation contains the given VecPartRelation elements in Collection.
* @param refPartRelation the given elements that should be contained in actual VecPartVersion's refPartRelation.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartRelation does not contain all given VecPartRelation elements.
*/
public S hasRefPartRelation(java.util.Collection extends VecPartRelation> refPartRelation) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation collection is not null.
if (refPartRelation == null) {
failWithMessage("Expecting refPartRelation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartRelation(), refPartRelation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartRelation contains only the given VecPartRelation elements and nothing else in whatever order.
* @param refPartRelation the given elements that should be contained in actual VecPartVersion's refPartRelation.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartRelation does not contain all given VecPartRelation elements.
*/
public S hasOnlyRefPartRelation(VecPartRelation... refPartRelation) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation varargs is not null.
if (refPartRelation == null) failWithMessage("Expecting refPartRelation parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartRelation(), refPartRelation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartRelation contains only the given VecPartRelation elements in Collection and nothing else in whatever order.
* @param refPartRelation the given elements that should be contained in actual VecPartVersion's refPartRelation.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartRelation does not contain all given VecPartRelation elements.
*/
public S hasOnlyRefPartRelation(java.util.Collection extends VecPartRelation> refPartRelation) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation collection is not null.
if (refPartRelation == null) {
failWithMessage("Expecting refPartRelation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartRelation(), refPartRelation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartRelation does not contain the given VecPartRelation elements.
*
* @param refPartRelation the given elements that should not be in actual VecPartVersion's refPartRelation.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartRelation contains any given VecPartRelation elements.
*/
public S doesNotHaveRefPartRelation(VecPartRelation... refPartRelation) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation varargs is not null.
if (refPartRelation == null) failWithMessage("Expecting refPartRelation parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartRelation(), refPartRelation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartRelation does not contain the given VecPartRelation elements in Collection.
*
* @param refPartRelation the given elements that should not be in actual VecPartVersion's refPartRelation.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartRelation contains any given VecPartRelation elements.
*/
public S doesNotHaveRefPartRelation(java.util.Collection extends VecPartRelation> refPartRelation) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartRelation collection is not null.
if (refPartRelation == null) {
failWithMessage("Expecting refPartRelation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartRelation(), refPartRelation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refPartRelation.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartRelation is not empty.
*/
public S hasNoRefPartRelation() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPartRelation but had :\n <%s>";
// check
if (actual.getRefPartRelation().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPartRelation());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartSubstitutionSpecification contains the given VecPartSubstitutionSpecification elements.
* @param refPartSubstitutionSpecification the given elements that should be contained in actual VecPartVersion's refPartSubstitutionSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartSubstitutionSpecification does not contain all given VecPartSubstitutionSpecification elements.
*/
public S hasRefPartSubstitutionSpecification(VecPartSubstitutionSpecification... refPartSubstitutionSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartSubstitutionSpecification varargs is not null.
if (refPartSubstitutionSpecification == null) failWithMessage("Expecting refPartSubstitutionSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartSubstitutionSpecification(), refPartSubstitutionSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartSubstitutionSpecification contains the given VecPartSubstitutionSpecification elements in Collection.
* @param refPartSubstitutionSpecification the given elements that should be contained in actual VecPartVersion's refPartSubstitutionSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartSubstitutionSpecification does not contain all given VecPartSubstitutionSpecification elements.
*/
public S hasRefPartSubstitutionSpecification(java.util.Collection extends VecPartSubstitutionSpecification> refPartSubstitutionSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartSubstitutionSpecification collection is not null.
if (refPartSubstitutionSpecification == null) {
failWithMessage("Expecting refPartSubstitutionSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPartSubstitutionSpecification(), refPartSubstitutionSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartSubstitutionSpecification contains only the given VecPartSubstitutionSpecification elements and nothing else in whatever order.
* @param refPartSubstitutionSpecification the given elements that should be contained in actual VecPartVersion's refPartSubstitutionSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartSubstitutionSpecification does not contain all given VecPartSubstitutionSpecification elements.
*/
public S hasOnlyRefPartSubstitutionSpecification(VecPartSubstitutionSpecification... refPartSubstitutionSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartSubstitutionSpecification varargs is not null.
if (refPartSubstitutionSpecification == null) failWithMessage("Expecting refPartSubstitutionSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartSubstitutionSpecification(), refPartSubstitutionSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartSubstitutionSpecification contains only the given VecPartSubstitutionSpecification elements in Collection and nothing else in whatever order.
* @param refPartSubstitutionSpecification the given elements that should be contained in actual VecPartVersion's refPartSubstitutionSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartSubstitutionSpecification does not contain all given VecPartSubstitutionSpecification elements.
*/
public S hasOnlyRefPartSubstitutionSpecification(java.util.Collection extends VecPartSubstitutionSpecification> refPartSubstitutionSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartSubstitutionSpecification collection is not null.
if (refPartSubstitutionSpecification == null) {
failWithMessage("Expecting refPartSubstitutionSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPartSubstitutionSpecification(), refPartSubstitutionSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartSubstitutionSpecification does not contain the given VecPartSubstitutionSpecification elements.
*
* @param refPartSubstitutionSpecification the given elements that should not be in actual VecPartVersion's refPartSubstitutionSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartSubstitutionSpecification contains any given VecPartSubstitutionSpecification elements.
*/
public S doesNotHaveRefPartSubstitutionSpecification(VecPartSubstitutionSpecification... refPartSubstitutionSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartSubstitutionSpecification varargs is not null.
if (refPartSubstitutionSpecification == null) failWithMessage("Expecting refPartSubstitutionSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartSubstitutionSpecification(), refPartSubstitutionSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refPartSubstitutionSpecification does not contain the given VecPartSubstitutionSpecification elements in Collection.
*
* @param refPartSubstitutionSpecification the given elements that should not be in actual VecPartVersion's refPartSubstitutionSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartSubstitutionSpecification contains any given VecPartSubstitutionSpecification elements.
*/
public S doesNotHaveRefPartSubstitutionSpecification(java.util.Collection extends VecPartSubstitutionSpecification> refPartSubstitutionSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecPartSubstitutionSpecification collection is not null.
if (refPartSubstitutionSpecification == null) {
failWithMessage("Expecting refPartSubstitutionSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPartSubstitutionSpecification(), refPartSubstitutionSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refPartSubstitutionSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refPartSubstitutionSpecification is not empty.
*/
public S hasNoRefPartSubstitutionSpecification() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPartSubstitutionSpecification but had :\n <%s>";
// check
if (actual.getRefPartSubstitutionSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPartSubstitutionSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refSheetOrChapter contains the given VecSheetOrChapter elements.
* @param refSheetOrChapter the given elements that should be contained in actual VecPartVersion's refSheetOrChapter.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refSheetOrChapter does not contain all given VecSheetOrChapter elements.
*/
public S hasRefSheetOrChapter(VecSheetOrChapter... refSheetOrChapter) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter varargs is not null.
if (refSheetOrChapter == null) failWithMessage("Expecting refSheetOrChapter parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSheetOrChapter(), refSheetOrChapter);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refSheetOrChapter contains the given VecSheetOrChapter elements in Collection.
* @param refSheetOrChapter the given elements that should be contained in actual VecPartVersion's refSheetOrChapter.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refSheetOrChapter does not contain all given VecSheetOrChapter elements.
*/
public S hasRefSheetOrChapter(java.util.Collection extends VecSheetOrChapter> refSheetOrChapter) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter collection is not null.
if (refSheetOrChapter == null) {
failWithMessage("Expecting refSheetOrChapter parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSheetOrChapter(), refSheetOrChapter.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refSheetOrChapter contains only the given VecSheetOrChapter elements and nothing else in whatever order.
* @param refSheetOrChapter the given elements that should be contained in actual VecPartVersion's refSheetOrChapter.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refSheetOrChapter does not contain all given VecSheetOrChapter elements.
*/
public S hasOnlyRefSheetOrChapter(VecSheetOrChapter... refSheetOrChapter) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter varargs is not null.
if (refSheetOrChapter == null) failWithMessage("Expecting refSheetOrChapter parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSheetOrChapter(), refSheetOrChapter);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refSheetOrChapter contains only the given VecSheetOrChapter elements in Collection and nothing else in whatever order.
* @param refSheetOrChapter the given elements that should be contained in actual VecPartVersion's refSheetOrChapter.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refSheetOrChapter does not contain all given VecSheetOrChapter elements.
*/
public S hasOnlyRefSheetOrChapter(java.util.Collection extends VecSheetOrChapter> refSheetOrChapter) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter collection is not null.
if (refSheetOrChapter == null) {
failWithMessage("Expecting refSheetOrChapter parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSheetOrChapter(), refSheetOrChapter.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refSheetOrChapter does not contain the given VecSheetOrChapter elements.
*
* @param refSheetOrChapter the given elements that should not be in actual VecPartVersion's refSheetOrChapter.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refSheetOrChapter contains any given VecSheetOrChapter elements.
*/
public S doesNotHaveRefSheetOrChapter(VecSheetOrChapter... refSheetOrChapter) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter varargs is not null.
if (refSheetOrChapter == null) failWithMessage("Expecting refSheetOrChapter parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSheetOrChapter(), refSheetOrChapter);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refSheetOrChapter does not contain the given VecSheetOrChapter elements in Collection.
*
* @param refSheetOrChapter the given elements that should not be in actual VecPartVersion's refSheetOrChapter.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refSheetOrChapter contains any given VecSheetOrChapter elements.
*/
public S doesNotHaveRefSheetOrChapter(java.util.Collection extends VecSheetOrChapter> refSheetOrChapter) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecSheetOrChapter collection is not null.
if (refSheetOrChapter == null) {
failWithMessage("Expecting refSheetOrChapter parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSheetOrChapter(), refSheetOrChapter.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refSheetOrChapter.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refSheetOrChapter is not empty.
*/
public S hasNoRefSheetOrChapter() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refSheetOrChapter but had :\n <%s>";
// check
if (actual.getRefSheetOrChapter().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefSheetOrChapter());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refTerminalPairing contains the given VecTerminalPairing elements.
* @param refTerminalPairing the given elements that should be contained in actual VecPartVersion's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasRefTerminalPairing(VecTerminalPairing... refTerminalPairing) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing varargs is not null.
if (refTerminalPairing == null) failWithMessage("Expecting refTerminalPairing parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalPairing(), refTerminalPairing);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refTerminalPairing contains the given VecTerminalPairing elements in Collection.
* @param refTerminalPairing the given elements that should be contained in actual VecPartVersion's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasRefTerminalPairing(java.util.Collection extends VecTerminalPairing> refTerminalPairing) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing collection is not null.
if (refTerminalPairing == null) {
failWithMessage("Expecting refTerminalPairing parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalPairing(), refTerminalPairing.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refTerminalPairing contains only the given VecTerminalPairing elements and nothing else in whatever order.
* @param refTerminalPairing the given elements that should be contained in actual VecPartVersion's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasOnlyRefTerminalPairing(VecTerminalPairing... refTerminalPairing) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing varargs is not null.
if (refTerminalPairing == null) failWithMessage("Expecting refTerminalPairing parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalPairing(), refTerminalPairing);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refTerminalPairing contains only the given VecTerminalPairing elements in Collection and nothing else in whatever order.
* @param refTerminalPairing the given elements that should be contained in actual VecPartVersion's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasOnlyRefTerminalPairing(java.util.Collection extends VecTerminalPairing> refTerminalPairing) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing collection is not null.
if (refTerminalPairing == null) {
failWithMessage("Expecting refTerminalPairing parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalPairing(), refTerminalPairing.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refTerminalPairing does not contain the given VecTerminalPairing elements.
*
* @param refTerminalPairing the given elements that should not be in actual VecPartVersion's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refTerminalPairing contains any given VecTerminalPairing elements.
*/
public S doesNotHaveRefTerminalPairing(VecTerminalPairing... refTerminalPairing) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing varargs is not null.
if (refTerminalPairing == null) failWithMessage("Expecting refTerminalPairing parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalPairing(), refTerminalPairing);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refTerminalPairing does not contain the given VecTerminalPairing elements in Collection.
*
* @param refTerminalPairing the given elements that should not be in actual VecPartVersion's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refTerminalPairing contains any given VecTerminalPairing elements.
*/
public S doesNotHaveRefTerminalPairing(java.util.Collection extends VecTerminalPairing> refTerminalPairing) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing collection is not null.
if (refTerminalPairing == null) {
failWithMessage("Expecting refTerminalPairing parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalPairing(), refTerminalPairing.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refTerminalPairing is not empty.
*/
public S hasNoRefTerminalPairing() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTerminalPairing but had :\n <%s>";
// check
if (actual.getRefTerminalPairing().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTerminalPairing());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refUsageConstraintSpecification contains the given VecUsageConstraintSpecification elements.
* @param refUsageConstraintSpecification the given elements that should be contained in actual VecPartVersion's refUsageConstraintSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refUsageConstraintSpecification does not contain all given VecUsageConstraintSpecification elements.
*/
public S hasRefUsageConstraintSpecification(VecUsageConstraintSpecification... refUsageConstraintSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraintSpecification varargs is not null.
if (refUsageConstraintSpecification == null) failWithMessage("Expecting refUsageConstraintSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefUsageConstraintSpecification(), refUsageConstraintSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refUsageConstraintSpecification contains the given VecUsageConstraintSpecification elements in Collection.
* @param refUsageConstraintSpecification the given elements that should be contained in actual VecPartVersion's refUsageConstraintSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refUsageConstraintSpecification does not contain all given VecUsageConstraintSpecification elements.
*/
public S hasRefUsageConstraintSpecification(java.util.Collection extends VecUsageConstraintSpecification> refUsageConstraintSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraintSpecification collection is not null.
if (refUsageConstraintSpecification == null) {
failWithMessage("Expecting refUsageConstraintSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefUsageConstraintSpecification(), refUsageConstraintSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refUsageConstraintSpecification contains only the given VecUsageConstraintSpecification elements and nothing else in whatever order.
* @param refUsageConstraintSpecification the given elements that should be contained in actual VecPartVersion's refUsageConstraintSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refUsageConstraintSpecification does not contain all given VecUsageConstraintSpecification elements.
*/
public S hasOnlyRefUsageConstraintSpecification(VecUsageConstraintSpecification... refUsageConstraintSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraintSpecification varargs is not null.
if (refUsageConstraintSpecification == null) failWithMessage("Expecting refUsageConstraintSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefUsageConstraintSpecification(), refUsageConstraintSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refUsageConstraintSpecification contains only the given VecUsageConstraintSpecification elements in Collection and nothing else in whatever order.
* @param refUsageConstraintSpecification the given elements that should be contained in actual VecPartVersion's refUsageConstraintSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refUsageConstraintSpecification does not contain all given VecUsageConstraintSpecification elements.
*/
public S hasOnlyRefUsageConstraintSpecification(java.util.Collection extends VecUsageConstraintSpecification> refUsageConstraintSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraintSpecification collection is not null.
if (refUsageConstraintSpecification == null) {
failWithMessage("Expecting refUsageConstraintSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefUsageConstraintSpecification(), refUsageConstraintSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refUsageConstraintSpecification does not contain the given VecUsageConstraintSpecification elements.
*
* @param refUsageConstraintSpecification the given elements that should not be in actual VecPartVersion's refUsageConstraintSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refUsageConstraintSpecification contains any given VecUsageConstraintSpecification elements.
*/
public S doesNotHaveRefUsageConstraintSpecification(VecUsageConstraintSpecification... refUsageConstraintSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraintSpecification varargs is not null.
if (refUsageConstraintSpecification == null) failWithMessage("Expecting refUsageConstraintSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefUsageConstraintSpecification(), refUsageConstraintSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion's refUsageConstraintSpecification does not contain the given VecUsageConstraintSpecification elements in Collection.
*
* @param refUsageConstraintSpecification the given elements that should not be in actual VecPartVersion's refUsageConstraintSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refUsageConstraintSpecification contains any given VecUsageConstraintSpecification elements.
*/
public S doesNotHaveRefUsageConstraintSpecification(java.util.Collection extends VecUsageConstraintSpecification> refUsageConstraintSpecification) {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraintSpecification collection is not null.
if (refUsageConstraintSpecification == null) {
failWithMessage("Expecting refUsageConstraintSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefUsageConstraintSpecification(), refUsageConstraintSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPartVersion has no refUsageConstraintSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPartVersion's refUsageConstraintSpecification is not empty.
*/
public S hasNoRefUsageConstraintSpecification() {
// check that actual VecPartVersion we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refUsageConstraintSpecification but had :\n <%s>";
// check
if (actual.getRefUsageConstraintSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefUsageConstraintSpecification());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy