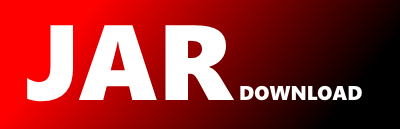
com.foursoft.vecmodel.vec120.AbstractVecPermissionAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecPermission} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPermissionAssert, A extends VecPermission> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecPermissionAssert}
to make assertions on actual VecPermission.
* @param actual the VecPermission we want to make assertions on.
*/
protected AbstractVecPermissionAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPermission's parentApproval is equal to the given one.
* @param parentApproval the given parentApproval to compare the actual VecPermission's parentApproval to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPermission's parentApproval is not equal to the given one.
*/
public S hasParentApproval(VecApproval parentApproval) {
// check that actual VecPermission we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentApproval of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecApproval actualParentApproval = actual.getParentApproval();
if (!Objects.areEqual(actualParentApproval, parentApproval)) {
failWithMessage(assertjErrorMessage, actual, parentApproval, actualParentApproval);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPermission's permission is equal to the given one.
* @param permission the given permission to compare the actual VecPermission's permission to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPermission's permission is not equal to the given one.
*/
public S hasPermission(String permission) {
// check that actual VecPermission we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting permission of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualPermission = actual.getPermission();
if (!Objects.areEqual(actualPermission, permission)) {
failWithMessage(assertjErrorMessage, actual, permission, actualPermission);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPermission's permissionDate is equal to the given one.
* @param permissionDate the given permissionDate to compare the actual VecPermission's permissionDate to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPermission's permissionDate is not equal to the given one.
*/
public S hasPermissionDate(javax.xml.datatype.XMLGregorianCalendar permissionDate) {
// check that actual VecPermission we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting permissionDate of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
javax.xml.datatype.XMLGregorianCalendar actualPermissionDate = actual.getPermissionDate();
if (!Objects.areEqual(actualPermissionDate, permissionDate)) {
failWithMessage(assertjErrorMessage, actual, permissionDate, actualPermissionDate);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPermission's permitter is equal to the given one.
* @param permitter the given permitter to compare the actual VecPermission's permitter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPermission's permitter is not equal to the given one.
*/
public S hasPermitter(VecPerson permitter) {
// check that actual VecPermission we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting permitter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPerson actualPermitter = actual.getPermitter();
if (!Objects.areEqual(actualPermitter, permitter)) {
failWithMessage(assertjErrorMessage, actual, permitter, actualPermitter);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy