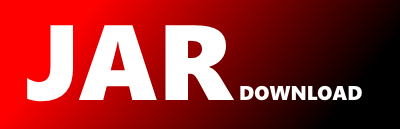
com.foursoft.vecmodel.vec120.AbstractVecPinComponentAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecPinComponent} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPinComponentAssert, A extends VecPinComponent> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecPinComponentAssert}
to make assertions on actual VecPinComponent.
* @param actual the VecPinComponent we want to make assertions on.
*/
protected AbstractVecPinComponentAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPinComponent's behaviours contains the given VecPinComponentBehavior elements.
* @param behaviours the given elements that should be contained in actual VecPinComponent's behaviours.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's behaviours does not contain all given VecPinComponentBehavior elements.
*/
public S hasBehaviours(VecPinComponentBehavior... behaviours) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior varargs is not null.
if (behaviours == null) failWithMessage("Expecting behaviours parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getBehaviours(), behaviours);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's behaviours contains the given VecPinComponentBehavior elements in Collection.
* @param behaviours the given elements that should be contained in actual VecPinComponent's behaviours.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's behaviours does not contain all given VecPinComponentBehavior elements.
*/
public S hasBehaviours(java.util.Collection extends VecPinComponentBehavior> behaviours) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior collection is not null.
if (behaviours == null) {
failWithMessage("Expecting behaviours parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getBehaviours(), behaviours.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's behaviours contains only the given VecPinComponentBehavior elements and nothing else in whatever order.
* @param behaviours the given elements that should be contained in actual VecPinComponent's behaviours.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's behaviours does not contain all given VecPinComponentBehavior elements.
*/
public S hasOnlyBehaviours(VecPinComponentBehavior... behaviours) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior varargs is not null.
if (behaviours == null) failWithMessage("Expecting behaviours parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getBehaviours(), behaviours);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's behaviours contains only the given VecPinComponentBehavior elements in Collection and nothing else in whatever order.
* @param behaviours the given elements that should be contained in actual VecPinComponent's behaviours.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's behaviours does not contain all given VecPinComponentBehavior elements.
*/
public S hasOnlyBehaviours(java.util.Collection extends VecPinComponentBehavior> behaviours) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior collection is not null.
if (behaviours == null) {
failWithMessage("Expecting behaviours parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getBehaviours(), behaviours.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's behaviours does not contain the given VecPinComponentBehavior elements.
*
* @param behaviours the given elements that should not be in actual VecPinComponent's behaviours.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's behaviours contains any given VecPinComponentBehavior elements.
*/
public S doesNotHaveBehaviours(VecPinComponentBehavior... behaviours) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior varargs is not null.
if (behaviours == null) failWithMessage("Expecting behaviours parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getBehaviours(), behaviours);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's behaviours does not contain the given VecPinComponentBehavior elements in Collection.
*
* @param behaviours the given elements that should not be in actual VecPinComponent's behaviours.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's behaviours contains any given VecPinComponentBehavior elements.
*/
public S doesNotHaveBehaviours(java.util.Collection extends VecPinComponentBehavior> behaviours) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior collection is not null.
if (behaviours == null) {
failWithMessage("Expecting behaviours parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getBehaviours(), behaviours.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent has no behaviours.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's behaviours is not empty.
*/
public S hasNoBehaviours() {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have behaviours but had :\n <%s>";
// check
if (actual.getBehaviours().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getBehaviours());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's descriptions contains the given VecAbstractLocalizedString elements.
* @param descriptions the given elements that should be contained in actual VecPinComponent's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's descriptions contains the given VecAbstractLocalizedString elements in Collection.
* @param descriptions the given elements that should be contained in actual VecPinComponent's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's descriptions contains only the given VecAbstractLocalizedString elements and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecPinComponent's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's descriptions contains only the given VecAbstractLocalizedString elements in Collection and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecPinComponent's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's descriptions does not contain the given VecAbstractLocalizedString elements.
*
* @param descriptions the given elements that should not be in actual VecPinComponent's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's descriptions does not contain the given VecAbstractLocalizedString elements in Collection.
*
* @param descriptions the given elements that should not be in actual VecPinComponent's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent has no descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's descriptions is not empty.
*/
public S hasNoDescriptions() {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have descriptions but had :\n <%s>";
// check
if (actual.getDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's identification is equal to the given one.
* @param identification the given identification to compare the actual VecPinComponent's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinComponent's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's parentHousingComponent is equal to the given one.
* @param parentHousingComponent the given parentHousingComponent to compare the actual VecPinComponent's parentHousingComponent to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinComponent's parentHousingComponent is not equal to the given one.
*/
public S hasParentHousingComponent(VecHousingComponent parentHousingComponent) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentHousingComponent of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecHousingComponent actualParentHousingComponent = actual.getParentHousingComponent();
if (!Objects.areEqual(actualParentHousingComponent, parentHousingComponent)) {
failWithMessage(assertjErrorMessage, actual, parentHousingComponent, actualParentHousingComponent);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's pinComponentType is equal to the given one.
* @param pinComponentType the given pinComponentType to compare the actual VecPinComponent's pinComponentType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinComponent's pinComponentType is not equal to the given one.
*/
public S hasPinComponentType(String pinComponentType) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting pinComponentType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualPinComponentType = actual.getPinComponentType();
if (!Objects.areEqual(actualPinComponentType, pinComponentType)) {
failWithMessage(assertjErrorMessage, actual, pinComponentType, actualPinComponentType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's pinSpecification is equal to the given one.
* @param pinSpecification the given pinSpecification to compare the actual VecPinComponent's pinSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinComponent's pinSpecification is not equal to the given one.
*/
public S hasPinSpecification(VecTerminalSpecification pinSpecification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting pinSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTerminalSpecification actualPinSpecification = actual.getPinSpecification();
if (!Objects.areEqual(actualPinSpecification, pinSpecification)) {
failWithMessage(assertjErrorMessage, actual, pinSpecification, actualPinSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refDiodeSpecification contains the given VecDiodeSpecification elements.
* @param refDiodeSpecification the given elements that should be contained in actual VecPinComponent's refDiodeSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refDiodeSpecification does not contain all given VecDiodeSpecification elements.
*/
public S hasRefDiodeSpecification(VecDiodeSpecification... refDiodeSpecification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecDiodeSpecification varargs is not null.
if (refDiodeSpecification == null) failWithMessage("Expecting refDiodeSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDiodeSpecification(), refDiodeSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refDiodeSpecification contains the given VecDiodeSpecification elements in Collection.
* @param refDiodeSpecification the given elements that should be contained in actual VecPinComponent's refDiodeSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refDiodeSpecification does not contain all given VecDiodeSpecification elements.
*/
public S hasRefDiodeSpecification(java.util.Collection extends VecDiodeSpecification> refDiodeSpecification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecDiodeSpecification collection is not null.
if (refDiodeSpecification == null) {
failWithMessage("Expecting refDiodeSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDiodeSpecification(), refDiodeSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refDiodeSpecification contains only the given VecDiodeSpecification elements and nothing else in whatever order.
* @param refDiodeSpecification the given elements that should be contained in actual VecPinComponent's refDiodeSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refDiodeSpecification does not contain all given VecDiodeSpecification elements.
*/
public S hasOnlyRefDiodeSpecification(VecDiodeSpecification... refDiodeSpecification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecDiodeSpecification varargs is not null.
if (refDiodeSpecification == null) failWithMessage("Expecting refDiodeSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDiodeSpecification(), refDiodeSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refDiodeSpecification contains only the given VecDiodeSpecification elements in Collection and nothing else in whatever order.
* @param refDiodeSpecification the given elements that should be contained in actual VecPinComponent's refDiodeSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refDiodeSpecification does not contain all given VecDiodeSpecification elements.
*/
public S hasOnlyRefDiodeSpecification(java.util.Collection extends VecDiodeSpecification> refDiodeSpecification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecDiodeSpecification collection is not null.
if (refDiodeSpecification == null) {
failWithMessage("Expecting refDiodeSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDiodeSpecification(), refDiodeSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refDiodeSpecification does not contain the given VecDiodeSpecification elements.
*
* @param refDiodeSpecification the given elements that should not be in actual VecPinComponent's refDiodeSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refDiodeSpecification contains any given VecDiodeSpecification elements.
*/
public S doesNotHaveRefDiodeSpecification(VecDiodeSpecification... refDiodeSpecification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecDiodeSpecification varargs is not null.
if (refDiodeSpecification == null) failWithMessage("Expecting refDiodeSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDiodeSpecification(), refDiodeSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refDiodeSpecification does not contain the given VecDiodeSpecification elements in Collection.
*
* @param refDiodeSpecification the given elements that should not be in actual VecPinComponent's refDiodeSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refDiodeSpecification contains any given VecDiodeSpecification elements.
*/
public S doesNotHaveRefDiodeSpecification(java.util.Collection extends VecDiodeSpecification> refDiodeSpecification) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecDiodeSpecification collection is not null.
if (refDiodeSpecification == null) {
failWithMessage("Expecting refDiodeSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDiodeSpecification(), refDiodeSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent has no refDiodeSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refDiodeSpecification is not empty.
*/
public S hasNoRefDiodeSpecification() {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDiodeSpecification but had :\n <%s>";
// check
if (actual.getRefDiodeSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDiodeSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refFuseComponent contains the given VecFuseComponent elements.
* @param refFuseComponent the given elements that should be contained in actual VecPinComponent's refFuseComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refFuseComponent does not contain all given VecFuseComponent elements.
*/
public S hasRefFuseComponent(VecFuseComponent... refFuseComponent) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecFuseComponent varargs is not null.
if (refFuseComponent == null) failWithMessage("Expecting refFuseComponent parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefFuseComponent(), refFuseComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refFuseComponent contains the given VecFuseComponent elements in Collection.
* @param refFuseComponent the given elements that should be contained in actual VecPinComponent's refFuseComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refFuseComponent does not contain all given VecFuseComponent elements.
*/
public S hasRefFuseComponent(java.util.Collection extends VecFuseComponent> refFuseComponent) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecFuseComponent collection is not null.
if (refFuseComponent == null) {
failWithMessage("Expecting refFuseComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefFuseComponent(), refFuseComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refFuseComponent contains only the given VecFuseComponent elements and nothing else in whatever order.
* @param refFuseComponent the given elements that should be contained in actual VecPinComponent's refFuseComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refFuseComponent does not contain all given VecFuseComponent elements.
*/
public S hasOnlyRefFuseComponent(VecFuseComponent... refFuseComponent) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecFuseComponent varargs is not null.
if (refFuseComponent == null) failWithMessage("Expecting refFuseComponent parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefFuseComponent(), refFuseComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refFuseComponent contains only the given VecFuseComponent elements in Collection and nothing else in whatever order.
* @param refFuseComponent the given elements that should be contained in actual VecPinComponent's refFuseComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refFuseComponent does not contain all given VecFuseComponent elements.
*/
public S hasOnlyRefFuseComponent(java.util.Collection extends VecFuseComponent> refFuseComponent) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecFuseComponent collection is not null.
if (refFuseComponent == null) {
failWithMessage("Expecting refFuseComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefFuseComponent(), refFuseComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refFuseComponent does not contain the given VecFuseComponent elements.
*
* @param refFuseComponent the given elements that should not be in actual VecPinComponent's refFuseComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refFuseComponent contains any given VecFuseComponent elements.
*/
public S doesNotHaveRefFuseComponent(VecFuseComponent... refFuseComponent) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecFuseComponent varargs is not null.
if (refFuseComponent == null) failWithMessage("Expecting refFuseComponent parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefFuseComponent(), refFuseComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refFuseComponent does not contain the given VecFuseComponent elements in Collection.
*
* @param refFuseComponent the given elements that should not be in actual VecPinComponent's refFuseComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refFuseComponent contains any given VecFuseComponent elements.
*/
public S doesNotHaveRefFuseComponent(java.util.Collection extends VecFuseComponent> refFuseComponent) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecFuseComponent collection is not null.
if (refFuseComponent == null) {
failWithMessage("Expecting refFuseComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefFuseComponent(), refFuseComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent has no refFuseComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refFuseComponent is not empty.
*/
public S hasNoRefFuseComponent() {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refFuseComponent but had :\n <%s>";
// check
if (actual.getRefFuseComponent().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefFuseComponent());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refInternalComponentConnection contains the given VecInternalComponentConnection elements.
* @param refInternalComponentConnection the given elements that should be contained in actual VecPinComponent's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasRefInternalComponentConnection(VecInternalComponentConnection... refInternalComponentConnection) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (refInternalComponentConnection == null) failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refInternalComponentConnection contains the given VecInternalComponentConnection elements in Collection.
* @param refInternalComponentConnection the given elements that should be contained in actual VecPinComponent's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasRefInternalComponentConnection(java.util.Collection extends VecInternalComponentConnection> refInternalComponentConnection) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (refInternalComponentConnection == null) {
failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refInternalComponentConnection contains only the given VecInternalComponentConnection elements and nothing else in whatever order.
* @param refInternalComponentConnection the given elements that should be contained in actual VecPinComponent's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasOnlyRefInternalComponentConnection(VecInternalComponentConnection... refInternalComponentConnection) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (refInternalComponentConnection == null) failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refInternalComponentConnection contains only the given VecInternalComponentConnection elements in Collection and nothing else in whatever order.
* @param refInternalComponentConnection the given elements that should be contained in actual VecPinComponent's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasOnlyRefInternalComponentConnection(java.util.Collection extends VecInternalComponentConnection> refInternalComponentConnection) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (refInternalComponentConnection == null) {
failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refInternalComponentConnection does not contain the given VecInternalComponentConnection elements.
*
* @param refInternalComponentConnection the given elements that should not be in actual VecPinComponent's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refInternalComponentConnection contains any given VecInternalComponentConnection elements.
*/
public S doesNotHaveRefInternalComponentConnection(VecInternalComponentConnection... refInternalComponentConnection) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (refInternalComponentConnection == null) failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refInternalComponentConnection does not contain the given VecInternalComponentConnection elements in Collection.
*
* @param refInternalComponentConnection the given elements that should not be in actual VecPinComponent's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refInternalComponentConnection contains any given VecInternalComponentConnection elements.
*/
public S doesNotHaveRefInternalComponentConnection(java.util.Collection extends VecInternalComponentConnection> refInternalComponentConnection) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (refInternalComponentConnection == null) {
failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent has no refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refInternalComponentConnection is not empty.
*/
public S hasNoRefInternalComponentConnection() {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refInternalComponentConnection but had :\n <%s>";
// check
if (actual.getRefInternalComponentConnection().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefInternalComponentConnection());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refPinComponentReference contains the given VecPinComponentReference elements.
* @param refPinComponentReference the given elements that should be contained in actual VecPinComponent's refPinComponentReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refPinComponentReference does not contain all given VecPinComponentReference elements.
*/
public S hasRefPinComponentReference(VecPinComponentReference... refPinComponentReference) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentReference varargs is not null.
if (refPinComponentReference == null) failWithMessage("Expecting refPinComponentReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponentReference(), refPinComponentReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refPinComponentReference contains the given VecPinComponentReference elements in Collection.
* @param refPinComponentReference the given elements that should be contained in actual VecPinComponent's refPinComponentReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refPinComponentReference does not contain all given VecPinComponentReference elements.
*/
public S hasRefPinComponentReference(java.util.Collection extends VecPinComponentReference> refPinComponentReference) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentReference collection is not null.
if (refPinComponentReference == null) {
failWithMessage("Expecting refPinComponentReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponentReference(), refPinComponentReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refPinComponentReference contains only the given VecPinComponentReference elements and nothing else in whatever order.
* @param refPinComponentReference the given elements that should be contained in actual VecPinComponent's refPinComponentReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refPinComponentReference does not contain all given VecPinComponentReference elements.
*/
public S hasOnlyRefPinComponentReference(VecPinComponentReference... refPinComponentReference) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentReference varargs is not null.
if (refPinComponentReference == null) failWithMessage("Expecting refPinComponentReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponentReference(), refPinComponentReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refPinComponentReference contains only the given VecPinComponentReference elements in Collection and nothing else in whatever order.
* @param refPinComponentReference the given elements that should be contained in actual VecPinComponent's refPinComponentReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refPinComponentReference does not contain all given VecPinComponentReference elements.
*/
public S hasOnlyRefPinComponentReference(java.util.Collection extends VecPinComponentReference> refPinComponentReference) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentReference collection is not null.
if (refPinComponentReference == null) {
failWithMessage("Expecting refPinComponentReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponentReference(), refPinComponentReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refPinComponentReference does not contain the given VecPinComponentReference elements.
*
* @param refPinComponentReference the given elements that should not be in actual VecPinComponent's refPinComponentReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refPinComponentReference contains any given VecPinComponentReference elements.
*/
public S doesNotHaveRefPinComponentReference(VecPinComponentReference... refPinComponentReference) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentReference varargs is not null.
if (refPinComponentReference == null) failWithMessage("Expecting refPinComponentReference parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponentReference(), refPinComponentReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's refPinComponentReference does not contain the given VecPinComponentReference elements in Collection.
*
* @param refPinComponentReference the given elements that should not be in actual VecPinComponent's refPinComponentReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refPinComponentReference contains any given VecPinComponentReference elements.
*/
public S doesNotHaveRefPinComponentReference(java.util.Collection extends VecPinComponentReference> refPinComponentReference) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentReference collection is not null.
if (refPinComponentReference == null) {
failWithMessage("Expecting refPinComponentReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponentReference(), refPinComponentReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent has no refPinComponentReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPinComponent's refPinComponentReference is not empty.
*/
public S hasNoRefPinComponentReference() {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPinComponentReference but had :\n <%s>";
// check
if (actual.getRefPinComponentReference().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPinComponentReference());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinComponent's referencedCavity is equal to the given one.
* @param referencedCavity the given referencedCavity to compare the actual VecPinComponent's referencedCavity to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinComponent's referencedCavity is not equal to the given one.
*/
public S hasReferencedCavity(VecCavity referencedCavity) {
// check that actual VecPinComponent we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting referencedCavity of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCavity actualReferencedCavity = actual.getReferencedCavity();
if (!Objects.areEqual(actualReferencedCavity, referencedCavity)) {
failWithMessage(assertjErrorMessage, actual, referencedCavity, actualReferencedCavity);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy