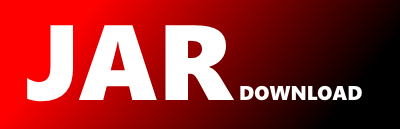
com.foursoft.vecmodel.vec120.AbstractVecPinTimingAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.AbstractObjectAssert;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecPinTiming} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPinTimingAssert, A extends VecPinTiming> extends AbstractObjectAssert {
/**
* Creates a new {@link AbstractVecPinTimingAssert}
to make assertions on actual VecPinTiming.
* @param actual the VecPinTiming we want to make assertions on.
*/
protected AbstractVecPinTimingAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPinTiming's parentPinCurrentInformation is equal to the given one.
* @param parentPinCurrentInformation the given parentPinCurrentInformation to compare the actual VecPinTiming's parentPinCurrentInformation to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinTiming's parentPinCurrentInformation is not equal to the given one.
*/
public S hasParentPinCurrentInformation(VecPinCurrentInformation parentPinCurrentInformation) {
// check that actual VecPinTiming we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentPinCurrentInformation of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPinCurrentInformation actualParentPinCurrentInformation = actual.getParentPinCurrentInformation();
if (!Objects.areEqual(actualParentPinCurrentInformation, parentPinCurrentInformation)) {
failWithMessage(assertjErrorMessage, actual, parentPinCurrentInformation, actualParentPinCurrentInformation);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinTiming's parentPinVoltageInformation is equal to the given one.
* @param parentPinVoltageInformation the given parentPinVoltageInformation to compare the actual VecPinTiming's parentPinVoltageInformation to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinTiming's parentPinVoltageInformation is not equal to the given one.
*/
public S hasParentPinVoltageInformation(VecPinVoltageInformation parentPinVoltageInformation) {
// check that actual VecPinTiming we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentPinVoltageInformation of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPinVoltageInformation actualParentPinVoltageInformation = actual.getParentPinVoltageInformation();
if (!Objects.areEqual(actualParentPinVoltageInformation, parentPinVoltageInformation)) {
failWithMessage(assertjErrorMessage, actual, parentPinVoltageInformation, actualParentPinVoltageInformation);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinTiming's time is equal to the given one.
* @param time the given time to compare the actual VecPinTiming's time to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinTiming's time is not equal to the given one.
*/
public S hasTime(VecNumericalValue time) {
// check that actual VecPinTiming we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting time of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualTime = actual.getTime();
if (!Objects.areEqual(actualTime, time)) {
failWithMessage(assertjErrorMessage, actual, time, actualTime);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinTiming's type is equal to the given one.
* @param type the given type to compare the actual VecPinTiming's type to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinTiming's type is not equal to the given one.
*/
public S hasType(String type) {
// check that actual VecPinTiming we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting type of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualType = actual.getType();
if (!Objects.areEqual(actualType, type)) {
failWithMessage(assertjErrorMessage, actual, type, actualType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPinTiming's xmlId is equal to the given one.
* @param xmlId the given xmlId to compare the actual VecPinTiming's xmlId to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPinTiming's xmlId is not equal to the given one.
*/
public S hasXmlId(String xmlId) {
// check that actual VecPinTiming we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting xmlId of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualXmlId = actual.getXmlId();
if (!Objects.areEqual(actualXmlId, xmlId)) {
failWithMessage(assertjErrorMessage, actual, xmlId, actualXmlId);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy