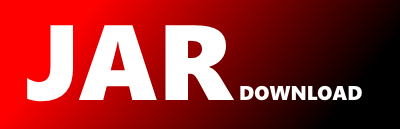
com.foursoft.vecmodel.vec120.AbstractVecPlaceableElementSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
/**
* Abstract base class for {@link VecPlaceableElementSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPlaceableElementSpecificationAssert, A extends VecPlaceableElementSpecification> extends AbstractVecPartOrUsageRelatedSpecificationAssert {
/**
* Creates a new {@link AbstractVecPlaceableElementSpecificationAssert}
to make assertions on actual VecPlaceableElementSpecification.
* @param actual the VecPlaceableElementSpecification we want to make assertions on.
*/
protected AbstractVecPlaceableElementSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPlaceableElementSpecification's measurementPoints contains the given VecMeasurementPoint elements.
* @param measurementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's measurementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's measurementPoints does not contain all given VecMeasurementPoint elements.
*/
public S hasMeasurementPoints(VecMeasurementPoint... measurementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMeasurementPoint varargs is not null.
if (measurementPoints == null) failWithMessage("Expecting measurementPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMeasurementPoints(), measurementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's measurementPoints contains the given VecMeasurementPoint elements in Collection.
* @param measurementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's measurementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's measurementPoints does not contain all given VecMeasurementPoint elements.
*/
public S hasMeasurementPoints(java.util.Collection extends VecMeasurementPoint> measurementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMeasurementPoint collection is not null.
if (measurementPoints == null) {
failWithMessage("Expecting measurementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMeasurementPoints(), measurementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's measurementPoints contains only the given VecMeasurementPoint elements and nothing else in whatever order.
* @param measurementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's measurementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's measurementPoints does not contain all given VecMeasurementPoint elements.
*/
public S hasOnlyMeasurementPoints(VecMeasurementPoint... measurementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMeasurementPoint varargs is not null.
if (measurementPoints == null) failWithMessage("Expecting measurementPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMeasurementPoints(), measurementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's measurementPoints contains only the given VecMeasurementPoint elements in Collection and nothing else in whatever order.
* @param measurementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's measurementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's measurementPoints does not contain all given VecMeasurementPoint elements.
*/
public S hasOnlyMeasurementPoints(java.util.Collection extends VecMeasurementPoint> measurementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMeasurementPoint collection is not null.
if (measurementPoints == null) {
failWithMessage("Expecting measurementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMeasurementPoints(), measurementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's measurementPoints does not contain the given VecMeasurementPoint elements.
*
* @param measurementPoints the given elements that should not be in actual VecPlaceableElementSpecification's measurementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's measurementPoints contains any given VecMeasurementPoint elements.
*/
public S doesNotHaveMeasurementPoints(VecMeasurementPoint... measurementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMeasurementPoint varargs is not null.
if (measurementPoints == null) failWithMessage("Expecting measurementPoints parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMeasurementPoints(), measurementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's measurementPoints does not contain the given VecMeasurementPoint elements in Collection.
*
* @param measurementPoints the given elements that should not be in actual VecPlaceableElementSpecification's measurementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's measurementPoints contains any given VecMeasurementPoint elements.
*/
public S doesNotHaveMeasurementPoints(java.util.Collection extends VecMeasurementPoint> measurementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMeasurementPoint collection is not null.
if (measurementPoints == null) {
failWithMessage("Expecting measurementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMeasurementPoints(), measurementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification has no measurementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's measurementPoints is not empty.
*/
public S hasNoMeasurementPoints() {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have measurementPoints but had :\n <%s>";
// check
if (actual.getMeasurementPoints().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getMeasurementPoints());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's placementPoints contains the given VecPlacementPoint elements.
* @param placementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's placementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's placementPoints does not contain all given VecPlacementPoint elements.
*/
public S hasPlacementPoints(VecPlacementPoint... placementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPoint varargs is not null.
if (placementPoints == null) failWithMessage("Expecting placementPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlacementPoints(), placementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's placementPoints contains the given VecPlacementPoint elements in Collection.
* @param placementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's placementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's placementPoints does not contain all given VecPlacementPoint elements.
*/
public S hasPlacementPoints(java.util.Collection extends VecPlacementPoint> placementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPoint collection is not null.
if (placementPoints == null) {
failWithMessage("Expecting placementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlacementPoints(), placementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's placementPoints contains only the given VecPlacementPoint elements and nothing else in whatever order.
* @param placementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's placementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's placementPoints does not contain all given VecPlacementPoint elements.
*/
public S hasOnlyPlacementPoints(VecPlacementPoint... placementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPoint varargs is not null.
if (placementPoints == null) failWithMessage("Expecting placementPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlacementPoints(), placementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's placementPoints contains only the given VecPlacementPoint elements in Collection and nothing else in whatever order.
* @param placementPoints the given elements that should be contained in actual VecPlaceableElementSpecification's placementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's placementPoints does not contain all given VecPlacementPoint elements.
*/
public S hasOnlyPlacementPoints(java.util.Collection extends VecPlacementPoint> placementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPoint collection is not null.
if (placementPoints == null) {
failWithMessage("Expecting placementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlacementPoints(), placementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's placementPoints does not contain the given VecPlacementPoint elements.
*
* @param placementPoints the given elements that should not be in actual VecPlaceableElementSpecification's placementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's placementPoints contains any given VecPlacementPoint elements.
*/
public S doesNotHavePlacementPoints(VecPlacementPoint... placementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPoint varargs is not null.
if (placementPoints == null) failWithMessage("Expecting placementPoints parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlacementPoints(), placementPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's placementPoints does not contain the given VecPlacementPoint elements in Collection.
*
* @param placementPoints the given elements that should not be in actual VecPlaceableElementSpecification's placementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's placementPoints contains any given VecPlacementPoint elements.
*/
public S doesNotHavePlacementPoints(java.util.Collection extends VecPlacementPoint> placementPoints) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPoint collection is not null.
if (placementPoints == null) {
failWithMessage("Expecting placementPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlacementPoints(), placementPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification has no placementPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's placementPoints is not empty.
*/
public S hasNoPlacementPoints() {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have placementPoints but had :\n <%s>";
// check
if (actual.getPlacementPoints().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPlacementPoints());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's refPlaceableElementRole contains the given VecPlaceableElementRole elements.
* @param refPlaceableElementRole the given elements that should be contained in actual VecPlaceableElementSpecification's refPlaceableElementRole.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's refPlaceableElementRole does not contain all given VecPlaceableElementRole elements.
*/
public S hasRefPlaceableElementRole(VecPlaceableElementRole... refPlaceableElementRole) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlaceableElementRole varargs is not null.
if (refPlaceableElementRole == null) failWithMessage("Expecting refPlaceableElementRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPlaceableElementRole(), refPlaceableElementRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's refPlaceableElementRole contains the given VecPlaceableElementRole elements in Collection.
* @param refPlaceableElementRole the given elements that should be contained in actual VecPlaceableElementSpecification's refPlaceableElementRole.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's refPlaceableElementRole does not contain all given VecPlaceableElementRole elements.
*/
public S hasRefPlaceableElementRole(java.util.Collection extends VecPlaceableElementRole> refPlaceableElementRole) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlaceableElementRole collection is not null.
if (refPlaceableElementRole == null) {
failWithMessage("Expecting refPlaceableElementRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPlaceableElementRole(), refPlaceableElementRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's refPlaceableElementRole contains only the given VecPlaceableElementRole elements and nothing else in whatever order.
* @param refPlaceableElementRole the given elements that should be contained in actual VecPlaceableElementSpecification's refPlaceableElementRole.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's refPlaceableElementRole does not contain all given VecPlaceableElementRole elements.
*/
public S hasOnlyRefPlaceableElementRole(VecPlaceableElementRole... refPlaceableElementRole) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlaceableElementRole varargs is not null.
if (refPlaceableElementRole == null) failWithMessage("Expecting refPlaceableElementRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPlaceableElementRole(), refPlaceableElementRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's refPlaceableElementRole contains only the given VecPlaceableElementRole elements in Collection and nothing else in whatever order.
* @param refPlaceableElementRole the given elements that should be contained in actual VecPlaceableElementSpecification's refPlaceableElementRole.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's refPlaceableElementRole does not contain all given VecPlaceableElementRole elements.
*/
public S hasOnlyRefPlaceableElementRole(java.util.Collection extends VecPlaceableElementRole> refPlaceableElementRole) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlaceableElementRole collection is not null.
if (refPlaceableElementRole == null) {
failWithMessage("Expecting refPlaceableElementRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPlaceableElementRole(), refPlaceableElementRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's refPlaceableElementRole does not contain the given VecPlaceableElementRole elements.
*
* @param refPlaceableElementRole the given elements that should not be in actual VecPlaceableElementSpecification's refPlaceableElementRole.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's refPlaceableElementRole contains any given VecPlaceableElementRole elements.
*/
public S doesNotHaveRefPlaceableElementRole(VecPlaceableElementRole... refPlaceableElementRole) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlaceableElementRole varargs is not null.
if (refPlaceableElementRole == null) failWithMessage("Expecting refPlaceableElementRole parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPlaceableElementRole(), refPlaceableElementRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's refPlaceableElementRole does not contain the given VecPlaceableElementRole elements in Collection.
*
* @param refPlaceableElementRole the given elements that should not be in actual VecPlaceableElementSpecification's refPlaceableElementRole.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's refPlaceableElementRole contains any given VecPlaceableElementRole elements.
*/
public S doesNotHaveRefPlaceableElementRole(java.util.Collection extends VecPlaceableElementRole> refPlaceableElementRole) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlaceableElementRole collection is not null.
if (refPlaceableElementRole == null) {
failWithMessage("Expecting refPlaceableElementRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPlaceableElementRole(), refPlaceableElementRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification has no refPlaceableElementRole.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's refPlaceableElementRole is not empty.
*/
public S hasNoRefPlaceableElementRole() {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPlaceableElementRole but had :\n <%s>";
// check
if (actual.getRefPlaceableElementRole().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPlaceableElementRole());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's validPlacementTypes contains the given VecPlacementType elements.
* @param validPlacementTypes the given elements that should be contained in actual VecPlaceableElementSpecification's validPlacementTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's validPlacementTypes does not contain all given VecPlacementType elements.
*/
public S hasValidPlacementTypes(VecPlacementType... validPlacementTypes) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementType varargs is not null.
if (validPlacementTypes == null) failWithMessage("Expecting validPlacementTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getValidPlacementTypes(), validPlacementTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's validPlacementTypes contains the given VecPlacementType elements in Collection.
* @param validPlacementTypes the given elements that should be contained in actual VecPlaceableElementSpecification's validPlacementTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's validPlacementTypes does not contain all given VecPlacementType elements.
*/
public S hasValidPlacementTypes(java.util.Collection extends VecPlacementType> validPlacementTypes) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementType collection is not null.
if (validPlacementTypes == null) {
failWithMessage("Expecting validPlacementTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getValidPlacementTypes(), validPlacementTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's validPlacementTypes contains only the given VecPlacementType elements and nothing else in whatever order.
* @param validPlacementTypes the given elements that should be contained in actual VecPlaceableElementSpecification's validPlacementTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's validPlacementTypes does not contain all given VecPlacementType elements.
*/
public S hasOnlyValidPlacementTypes(VecPlacementType... validPlacementTypes) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementType varargs is not null.
if (validPlacementTypes == null) failWithMessage("Expecting validPlacementTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getValidPlacementTypes(), validPlacementTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's validPlacementTypes contains only the given VecPlacementType elements in Collection and nothing else in whatever order.
* @param validPlacementTypes the given elements that should be contained in actual VecPlaceableElementSpecification's validPlacementTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's validPlacementTypes does not contain all given VecPlacementType elements.
*/
public S hasOnlyValidPlacementTypes(java.util.Collection extends VecPlacementType> validPlacementTypes) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementType collection is not null.
if (validPlacementTypes == null) {
failWithMessage("Expecting validPlacementTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getValidPlacementTypes(), validPlacementTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's validPlacementTypes does not contain the given VecPlacementType elements.
*
* @param validPlacementTypes the given elements that should not be in actual VecPlaceableElementSpecification's validPlacementTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's validPlacementTypes contains any given VecPlacementType elements.
*/
public S doesNotHaveValidPlacementTypes(VecPlacementType... validPlacementTypes) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementType varargs is not null.
if (validPlacementTypes == null) failWithMessage("Expecting validPlacementTypes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getValidPlacementTypes(), validPlacementTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification's validPlacementTypes does not contain the given VecPlacementType elements in Collection.
*
* @param validPlacementTypes the given elements that should not be in actual VecPlaceableElementSpecification's validPlacementTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's validPlacementTypes contains any given VecPlacementType elements.
*/
public S doesNotHaveValidPlacementTypes(java.util.Collection extends VecPlacementType> validPlacementTypes) {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementType collection is not null.
if (validPlacementTypes == null) {
failWithMessage("Expecting validPlacementTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getValidPlacementTypes(), validPlacementTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlaceableElementSpecification has no validPlacementTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecPlaceableElementSpecification's validPlacementTypes is not empty.
*/
public S hasNoValidPlacementTypes() {
// check that actual VecPlaceableElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have validPlacementTypes but had :\n <%s>";
// check
if (actual.getValidPlacementTypes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getValidPlacementTypes());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy