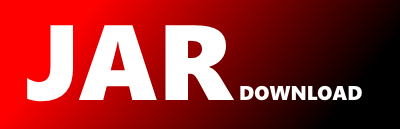
com.foursoft.vecmodel.vec120.AbstractVecPlacementPointAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecPlacementPoint} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecPlacementPointAssert, A extends VecPlacementPoint> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecPlacementPointAssert}
to make assertions on actual VecPlacementPoint.
* @param actual the VecPlacementPoint we want to make assertions on.
*/
protected AbstractVecPlacementPointAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecPlacementPoint's identification is equal to the given one.
* @param identification the given identification to compare the actual VecPlacementPoint's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPlacementPoint's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's parentPlaceableElementSpecification is equal to the given one.
* @param parentPlaceableElementSpecification the given parentPlaceableElementSpecification to compare the actual VecPlacementPoint's parentPlaceableElementSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPlacementPoint's parentPlaceableElementSpecification is not equal to the given one.
*/
public S hasParentPlaceableElementSpecification(VecPlaceableElementSpecification parentPlaceableElementSpecification) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentPlaceableElementSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPlaceableElementSpecification actualParentPlaceableElementSpecification = actual.getParentPlaceableElementSpecification();
if (!Objects.areEqual(actualParentPlaceableElementSpecification, parentPlaceableElementSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentPlaceableElementSpecification, actualParentPlaceableElementSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableDuctOutlet contains the given VecCableDuctOutlet elements.
* @param refCableDuctOutlet the given elements that should be contained in actual VecPlacementPoint's refCableDuctOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableDuctOutlet does not contain all given VecCableDuctOutlet elements.
*/
public S hasRefCableDuctOutlet(VecCableDuctOutlet... refCableDuctOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableDuctOutlet varargs is not null.
if (refCableDuctOutlet == null) failWithMessage("Expecting refCableDuctOutlet parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCableDuctOutlet(), refCableDuctOutlet);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableDuctOutlet contains the given VecCableDuctOutlet elements in Collection.
* @param refCableDuctOutlet the given elements that should be contained in actual VecPlacementPoint's refCableDuctOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableDuctOutlet does not contain all given VecCableDuctOutlet elements.
*/
public S hasRefCableDuctOutlet(java.util.Collection extends VecCableDuctOutlet> refCableDuctOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableDuctOutlet collection is not null.
if (refCableDuctOutlet == null) {
failWithMessage("Expecting refCableDuctOutlet parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCableDuctOutlet(), refCableDuctOutlet.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableDuctOutlet contains only the given VecCableDuctOutlet elements and nothing else in whatever order.
* @param refCableDuctOutlet the given elements that should be contained in actual VecPlacementPoint's refCableDuctOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableDuctOutlet does not contain all given VecCableDuctOutlet elements.
*/
public S hasOnlyRefCableDuctOutlet(VecCableDuctOutlet... refCableDuctOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableDuctOutlet varargs is not null.
if (refCableDuctOutlet == null) failWithMessage("Expecting refCableDuctOutlet parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCableDuctOutlet(), refCableDuctOutlet);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableDuctOutlet contains only the given VecCableDuctOutlet elements in Collection and nothing else in whatever order.
* @param refCableDuctOutlet the given elements that should be contained in actual VecPlacementPoint's refCableDuctOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableDuctOutlet does not contain all given VecCableDuctOutlet elements.
*/
public S hasOnlyRefCableDuctOutlet(java.util.Collection extends VecCableDuctOutlet> refCableDuctOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableDuctOutlet collection is not null.
if (refCableDuctOutlet == null) {
failWithMessage("Expecting refCableDuctOutlet parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCableDuctOutlet(), refCableDuctOutlet.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableDuctOutlet does not contain the given VecCableDuctOutlet elements.
*
* @param refCableDuctOutlet the given elements that should not be in actual VecPlacementPoint's refCableDuctOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableDuctOutlet contains any given VecCableDuctOutlet elements.
*/
public S doesNotHaveRefCableDuctOutlet(VecCableDuctOutlet... refCableDuctOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableDuctOutlet varargs is not null.
if (refCableDuctOutlet == null) failWithMessage("Expecting refCableDuctOutlet parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCableDuctOutlet(), refCableDuctOutlet);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableDuctOutlet does not contain the given VecCableDuctOutlet elements in Collection.
*
* @param refCableDuctOutlet the given elements that should not be in actual VecPlacementPoint's refCableDuctOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableDuctOutlet contains any given VecCableDuctOutlet elements.
*/
public S doesNotHaveRefCableDuctOutlet(java.util.Collection extends VecCableDuctOutlet> refCableDuctOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableDuctOutlet collection is not null.
if (refCableDuctOutlet == null) {
failWithMessage("Expecting refCableDuctOutlet parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCableDuctOutlet(), refCableDuctOutlet.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint has no refCableDuctOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableDuctOutlet is not empty.
*/
public S hasNoRefCableDuctOutlet() {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCableDuctOutlet but had :\n <%s>";
// check
if (actual.getRefCableDuctOutlet().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCableDuctOutlet());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableLeadThrough contains the given VecCableLeadThrough elements.
* @param refCableLeadThrough the given elements that should be contained in actual VecPlacementPoint's refCableLeadThrough.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableLeadThrough does not contain all given VecCableLeadThrough elements.
*/
public S hasRefCableLeadThrough(VecCableLeadThrough... refCableLeadThrough) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableLeadThrough varargs is not null.
if (refCableLeadThrough == null) failWithMessage("Expecting refCableLeadThrough parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCableLeadThrough(), refCableLeadThrough);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableLeadThrough contains the given VecCableLeadThrough elements in Collection.
* @param refCableLeadThrough the given elements that should be contained in actual VecPlacementPoint's refCableLeadThrough.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableLeadThrough does not contain all given VecCableLeadThrough elements.
*/
public S hasRefCableLeadThrough(java.util.Collection extends VecCableLeadThrough> refCableLeadThrough) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableLeadThrough collection is not null.
if (refCableLeadThrough == null) {
failWithMessage("Expecting refCableLeadThrough parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCableLeadThrough(), refCableLeadThrough.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableLeadThrough contains only the given VecCableLeadThrough elements and nothing else in whatever order.
* @param refCableLeadThrough the given elements that should be contained in actual VecPlacementPoint's refCableLeadThrough.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableLeadThrough does not contain all given VecCableLeadThrough elements.
*/
public S hasOnlyRefCableLeadThrough(VecCableLeadThrough... refCableLeadThrough) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableLeadThrough varargs is not null.
if (refCableLeadThrough == null) failWithMessage("Expecting refCableLeadThrough parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCableLeadThrough(), refCableLeadThrough);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableLeadThrough contains only the given VecCableLeadThrough elements in Collection and nothing else in whatever order.
* @param refCableLeadThrough the given elements that should be contained in actual VecPlacementPoint's refCableLeadThrough.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableLeadThrough does not contain all given VecCableLeadThrough elements.
*/
public S hasOnlyRefCableLeadThrough(java.util.Collection extends VecCableLeadThrough> refCableLeadThrough) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableLeadThrough collection is not null.
if (refCableLeadThrough == null) {
failWithMessage("Expecting refCableLeadThrough parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCableLeadThrough(), refCableLeadThrough.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableLeadThrough does not contain the given VecCableLeadThrough elements.
*
* @param refCableLeadThrough the given elements that should not be in actual VecPlacementPoint's refCableLeadThrough.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableLeadThrough contains any given VecCableLeadThrough elements.
*/
public S doesNotHaveRefCableLeadThrough(VecCableLeadThrough... refCableLeadThrough) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableLeadThrough varargs is not null.
if (refCableLeadThrough == null) failWithMessage("Expecting refCableLeadThrough parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCableLeadThrough(), refCableLeadThrough);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refCableLeadThrough does not contain the given VecCableLeadThrough elements in Collection.
*
* @param refCableLeadThrough the given elements that should not be in actual VecPlacementPoint's refCableLeadThrough.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableLeadThrough contains any given VecCableLeadThrough elements.
*/
public S doesNotHaveRefCableLeadThrough(java.util.Collection extends VecCableLeadThrough> refCableLeadThrough) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecCableLeadThrough collection is not null.
if (refCableLeadThrough == null) {
failWithMessage("Expecting refCableLeadThrough parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCableLeadThrough(), refCableLeadThrough.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint has no refCableLeadThrough.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refCableLeadThrough is not empty.
*/
public S hasNoRefCableLeadThrough() {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCableLeadThrough but had :\n <%s>";
// check
if (actual.getRefCableLeadThrough().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCableLeadThrough());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refFittingOutlet contains the given VecFittingOutlet elements.
* @param refFittingOutlet the given elements that should be contained in actual VecPlacementPoint's refFittingOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refFittingOutlet does not contain all given VecFittingOutlet elements.
*/
public S hasRefFittingOutlet(VecFittingOutlet... refFittingOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecFittingOutlet varargs is not null.
if (refFittingOutlet == null) failWithMessage("Expecting refFittingOutlet parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefFittingOutlet(), refFittingOutlet);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refFittingOutlet contains the given VecFittingOutlet elements in Collection.
* @param refFittingOutlet the given elements that should be contained in actual VecPlacementPoint's refFittingOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refFittingOutlet does not contain all given VecFittingOutlet elements.
*/
public S hasRefFittingOutlet(java.util.Collection extends VecFittingOutlet> refFittingOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecFittingOutlet collection is not null.
if (refFittingOutlet == null) {
failWithMessage("Expecting refFittingOutlet parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefFittingOutlet(), refFittingOutlet.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refFittingOutlet contains only the given VecFittingOutlet elements and nothing else in whatever order.
* @param refFittingOutlet the given elements that should be contained in actual VecPlacementPoint's refFittingOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refFittingOutlet does not contain all given VecFittingOutlet elements.
*/
public S hasOnlyRefFittingOutlet(VecFittingOutlet... refFittingOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecFittingOutlet varargs is not null.
if (refFittingOutlet == null) failWithMessage("Expecting refFittingOutlet parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefFittingOutlet(), refFittingOutlet);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refFittingOutlet contains only the given VecFittingOutlet elements in Collection and nothing else in whatever order.
* @param refFittingOutlet the given elements that should be contained in actual VecPlacementPoint's refFittingOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refFittingOutlet does not contain all given VecFittingOutlet elements.
*/
public S hasOnlyRefFittingOutlet(java.util.Collection extends VecFittingOutlet> refFittingOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecFittingOutlet collection is not null.
if (refFittingOutlet == null) {
failWithMessage("Expecting refFittingOutlet parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefFittingOutlet(), refFittingOutlet.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refFittingOutlet does not contain the given VecFittingOutlet elements.
*
* @param refFittingOutlet the given elements that should not be in actual VecPlacementPoint's refFittingOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refFittingOutlet contains any given VecFittingOutlet elements.
*/
public S doesNotHaveRefFittingOutlet(VecFittingOutlet... refFittingOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecFittingOutlet varargs is not null.
if (refFittingOutlet == null) failWithMessage("Expecting refFittingOutlet parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefFittingOutlet(), refFittingOutlet);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refFittingOutlet does not contain the given VecFittingOutlet elements in Collection.
*
* @param refFittingOutlet the given elements that should not be in actual VecPlacementPoint's refFittingOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refFittingOutlet contains any given VecFittingOutlet elements.
*/
public S doesNotHaveRefFittingOutlet(java.util.Collection extends VecFittingOutlet> refFittingOutlet) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecFittingOutlet collection is not null.
if (refFittingOutlet == null) {
failWithMessage("Expecting refFittingOutlet parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefFittingOutlet(), refFittingOutlet.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint has no refFittingOutlet.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refFittingOutlet is not empty.
*/
public S hasNoRefFittingOutlet() {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refFittingOutlet but had :\n <%s>";
// check
if (actual.getRefFittingOutlet().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefFittingOutlet());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointPosition contains the given VecPlacementPointPosition elements.
* @param refPlacementPointPosition the given elements that should be contained in actual VecPlacementPoint's refPlacementPointPosition.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointPosition does not contain all given VecPlacementPointPosition elements.
*/
public S hasRefPlacementPointPosition(VecPlacementPointPosition... refPlacementPointPosition) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointPosition varargs is not null.
if (refPlacementPointPosition == null) failWithMessage("Expecting refPlacementPointPosition parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPlacementPointPosition(), refPlacementPointPosition);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointPosition contains the given VecPlacementPointPosition elements in Collection.
* @param refPlacementPointPosition the given elements that should be contained in actual VecPlacementPoint's refPlacementPointPosition.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointPosition does not contain all given VecPlacementPointPosition elements.
*/
public S hasRefPlacementPointPosition(java.util.Collection extends VecPlacementPointPosition> refPlacementPointPosition) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointPosition collection is not null.
if (refPlacementPointPosition == null) {
failWithMessage("Expecting refPlacementPointPosition parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPlacementPointPosition(), refPlacementPointPosition.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointPosition contains only the given VecPlacementPointPosition elements and nothing else in whatever order.
* @param refPlacementPointPosition the given elements that should be contained in actual VecPlacementPoint's refPlacementPointPosition.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointPosition does not contain all given VecPlacementPointPosition elements.
*/
public S hasOnlyRefPlacementPointPosition(VecPlacementPointPosition... refPlacementPointPosition) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointPosition varargs is not null.
if (refPlacementPointPosition == null) failWithMessage("Expecting refPlacementPointPosition parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPlacementPointPosition(), refPlacementPointPosition);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointPosition contains only the given VecPlacementPointPosition elements in Collection and nothing else in whatever order.
* @param refPlacementPointPosition the given elements that should be contained in actual VecPlacementPoint's refPlacementPointPosition.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointPosition does not contain all given VecPlacementPointPosition elements.
*/
public S hasOnlyRefPlacementPointPosition(java.util.Collection extends VecPlacementPointPosition> refPlacementPointPosition) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointPosition collection is not null.
if (refPlacementPointPosition == null) {
failWithMessage("Expecting refPlacementPointPosition parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPlacementPointPosition(), refPlacementPointPosition.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointPosition does not contain the given VecPlacementPointPosition elements.
*
* @param refPlacementPointPosition the given elements that should not be in actual VecPlacementPoint's refPlacementPointPosition.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointPosition contains any given VecPlacementPointPosition elements.
*/
public S doesNotHaveRefPlacementPointPosition(VecPlacementPointPosition... refPlacementPointPosition) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointPosition varargs is not null.
if (refPlacementPointPosition == null) failWithMessage("Expecting refPlacementPointPosition parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPlacementPointPosition(), refPlacementPointPosition);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointPosition does not contain the given VecPlacementPointPosition elements in Collection.
*
* @param refPlacementPointPosition the given elements that should not be in actual VecPlacementPoint's refPlacementPointPosition.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointPosition contains any given VecPlacementPointPosition elements.
*/
public S doesNotHaveRefPlacementPointPosition(java.util.Collection extends VecPlacementPointPosition> refPlacementPointPosition) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointPosition collection is not null.
if (refPlacementPointPosition == null) {
failWithMessage("Expecting refPlacementPointPosition parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPlacementPointPosition(), refPlacementPointPosition.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint has no refPlacementPointPosition.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointPosition is not empty.
*/
public S hasNoRefPlacementPointPosition() {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPlacementPointPosition but had :\n <%s>";
// check
if (actual.getRefPlacementPointPosition().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPlacementPointPosition());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointReference contains the given VecPlacementPointReference elements.
* @param refPlacementPointReference the given elements that should be contained in actual VecPlacementPoint's refPlacementPointReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointReference does not contain all given VecPlacementPointReference elements.
*/
public S hasRefPlacementPointReference(VecPlacementPointReference... refPlacementPointReference) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference varargs is not null.
if (refPlacementPointReference == null) failWithMessage("Expecting refPlacementPointReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPlacementPointReference(), refPlacementPointReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointReference contains the given VecPlacementPointReference elements in Collection.
* @param refPlacementPointReference the given elements that should be contained in actual VecPlacementPoint's refPlacementPointReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointReference does not contain all given VecPlacementPointReference elements.
*/
public S hasRefPlacementPointReference(java.util.Collection extends VecPlacementPointReference> refPlacementPointReference) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference collection is not null.
if (refPlacementPointReference == null) {
failWithMessage("Expecting refPlacementPointReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPlacementPointReference(), refPlacementPointReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointReference contains only the given VecPlacementPointReference elements and nothing else in whatever order.
* @param refPlacementPointReference the given elements that should be contained in actual VecPlacementPoint's refPlacementPointReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointReference does not contain all given VecPlacementPointReference elements.
*/
public S hasOnlyRefPlacementPointReference(VecPlacementPointReference... refPlacementPointReference) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference varargs is not null.
if (refPlacementPointReference == null) failWithMessage("Expecting refPlacementPointReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPlacementPointReference(), refPlacementPointReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointReference contains only the given VecPlacementPointReference elements in Collection and nothing else in whatever order.
* @param refPlacementPointReference the given elements that should be contained in actual VecPlacementPoint's refPlacementPointReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointReference does not contain all given VecPlacementPointReference elements.
*/
public S hasOnlyRefPlacementPointReference(java.util.Collection extends VecPlacementPointReference> refPlacementPointReference) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference collection is not null.
if (refPlacementPointReference == null) {
failWithMessage("Expecting refPlacementPointReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPlacementPointReference(), refPlacementPointReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointReference does not contain the given VecPlacementPointReference elements.
*
* @param refPlacementPointReference the given elements that should not be in actual VecPlacementPoint's refPlacementPointReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointReference contains any given VecPlacementPointReference elements.
*/
public S doesNotHaveRefPlacementPointReference(VecPlacementPointReference... refPlacementPointReference) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference varargs is not null.
if (refPlacementPointReference == null) failWithMessage("Expecting refPlacementPointReference parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPlacementPointReference(), refPlacementPointReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refPlacementPointReference does not contain the given VecPlacementPointReference elements in Collection.
*
* @param refPlacementPointReference the given elements that should not be in actual VecPlacementPoint's refPlacementPointReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointReference contains any given VecPlacementPointReference elements.
*/
public S doesNotHaveRefPlacementPointReference(java.util.Collection extends VecPlacementPointReference> refPlacementPointReference) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecPlacementPointReference collection is not null.
if (refPlacementPointReference == null) {
failWithMessage("Expecting refPlacementPointReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPlacementPointReference(), refPlacementPointReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint has no refPlacementPointReference.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refPlacementPointReference is not empty.
*/
public S hasNoRefPlacementPointReference() {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPlacementPointReference but had :\n <%s>";
// check
if (actual.getRefPlacementPointReference().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPlacementPointReference());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refSegmentConnectionPoint contains the given VecSegmentConnectionPoint elements.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecPlacementPoint's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasRefSegmentConnectionPoint(VecSegmentConnectionPoint... refSegmentConnectionPoint) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint varargs is not null.
if (refSegmentConnectionPoint == null) failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refSegmentConnectionPoint contains the given VecSegmentConnectionPoint elements in Collection.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecPlacementPoint's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasRefSegmentConnectionPoint(java.util.Collection extends VecSegmentConnectionPoint> refSegmentConnectionPoint) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint collection is not null.
if (refSegmentConnectionPoint == null) {
failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refSegmentConnectionPoint contains only the given VecSegmentConnectionPoint elements and nothing else in whatever order.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecPlacementPoint's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasOnlyRefSegmentConnectionPoint(VecSegmentConnectionPoint... refSegmentConnectionPoint) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint varargs is not null.
if (refSegmentConnectionPoint == null) failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refSegmentConnectionPoint contains only the given VecSegmentConnectionPoint elements in Collection and nothing else in whatever order.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecPlacementPoint's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasOnlyRefSegmentConnectionPoint(java.util.Collection extends VecSegmentConnectionPoint> refSegmentConnectionPoint) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint collection is not null.
if (refSegmentConnectionPoint == null) {
failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refSegmentConnectionPoint does not contain the given VecSegmentConnectionPoint elements.
*
* @param refSegmentConnectionPoint the given elements that should not be in actual VecPlacementPoint's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refSegmentConnectionPoint contains any given VecSegmentConnectionPoint elements.
*/
public S doesNotHaveRefSegmentConnectionPoint(VecSegmentConnectionPoint... refSegmentConnectionPoint) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint varargs is not null.
if (refSegmentConnectionPoint == null) failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refSegmentConnectionPoint does not contain the given VecSegmentConnectionPoint elements in Collection.
*
* @param refSegmentConnectionPoint the given elements that should not be in actual VecPlacementPoint's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refSegmentConnectionPoint contains any given VecSegmentConnectionPoint elements.
*/
public S doesNotHaveRefSegmentConnectionPoint(java.util.Collection extends VecSegmentConnectionPoint> refSegmentConnectionPoint) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint collection is not null.
if (refSegmentConnectionPoint == null) {
failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint has no refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refSegmentConnectionPoint is not empty.
*/
public S hasNoRefSegmentConnectionPoint() {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refSegmentConnectionPoint but had :\n <%s>";
// check
if (actual.getRefSegmentConnectionPoint().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefSegmentConnectionPoint());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refWireReception contains the given VecWireReception elements.
* @param refWireReception the given elements that should be contained in actual VecPlacementPoint's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refWireReception does not contain all given VecWireReception elements.
*/
public S hasRefWireReception(VecWireReception... refWireReception) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (refWireReception == null) failWithMessage("Expecting refWireReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireReception(), refWireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refWireReception contains the given VecWireReception elements in Collection.
* @param refWireReception the given elements that should be contained in actual VecPlacementPoint's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refWireReception does not contain all given VecWireReception elements.
*/
public S hasRefWireReception(java.util.Collection extends VecWireReception> refWireReception) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (refWireReception == null) {
failWithMessage("Expecting refWireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireReception(), refWireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refWireReception contains only the given VecWireReception elements and nothing else in whatever order.
* @param refWireReception the given elements that should be contained in actual VecPlacementPoint's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refWireReception does not contain all given VecWireReception elements.
*/
public S hasOnlyRefWireReception(VecWireReception... refWireReception) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (refWireReception == null) failWithMessage("Expecting refWireReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireReception(), refWireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refWireReception contains only the given VecWireReception elements in Collection and nothing else in whatever order.
* @param refWireReception the given elements that should be contained in actual VecPlacementPoint's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refWireReception does not contain all given VecWireReception elements.
*/
public S hasOnlyRefWireReception(java.util.Collection extends VecWireReception> refWireReception) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (refWireReception == null) {
failWithMessage("Expecting refWireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireReception(), refWireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refWireReception does not contain the given VecWireReception elements.
*
* @param refWireReception the given elements that should not be in actual VecPlacementPoint's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refWireReception contains any given VecWireReception elements.
*/
public S doesNotHaveRefWireReception(VecWireReception... refWireReception) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (refWireReception == null) failWithMessage("Expecting refWireReception parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireReception(), refWireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's refWireReception does not contain the given VecWireReception elements in Collection.
*
* @param refWireReception the given elements that should not be in actual VecPlacementPoint's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refWireReception contains any given VecWireReception elements.
*/
public S doesNotHaveRefWireReception(java.util.Collection extends VecWireReception> refWireReception) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (refWireReception == null) {
failWithMessage("Expecting refWireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireReception(), refWireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint has no refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecPlacementPoint's refWireReception is not empty.
*/
public S hasNoRefWireReception() {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireReception but had :\n <%s>";
// check
if (actual.getRefWireReception().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireReception());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecPlacementPoint's segmentDiameter is equal to the given one.
* @param segmentDiameter the given segmentDiameter to compare the actual VecPlacementPoint's segmentDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecPlacementPoint's segmentDiameter is not equal to the given one.
*/
public S hasSegmentDiameter(VecValueRange segmentDiameter) {
// check that actual VecPlacementPoint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting segmentDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecValueRange actualSegmentDiameter = actual.getSegmentDiameter();
if (!Objects.areEqual(actualSegmentDiameter, segmentDiameter)) {
failWithMessage(assertjErrorMessage, actual, segmentDiameter, actualSegmentDiameter);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy