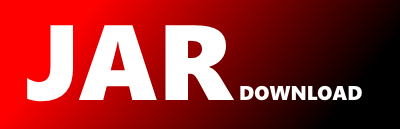
com.foursoft.vecmodel.vec120.AbstractVecSheetOrChapterAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecSheetOrChapter} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecSheetOrChapterAssert, A extends VecSheetOrChapter> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecSheetOrChapterAssert}
to make assertions on actual VecSheetOrChapter.
* @param actual the VecSheetOrChapter we want to make assertions on.
*/
protected AbstractVecSheetOrChapterAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecSheetOrChapter's changeDescriptions contains the given VecChangeDescription elements.
* @param changeDescriptions the given elements that should be contained in actual VecSheetOrChapter's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasChangeDescriptions(VecChangeDescription... changeDescriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription varargs is not null.
if (changeDescriptions == null) failWithMessage("Expecting changeDescriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getChangeDescriptions(), changeDescriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's changeDescriptions contains the given VecChangeDescription elements in Collection.
* @param changeDescriptions the given elements that should be contained in actual VecSheetOrChapter's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasChangeDescriptions(java.util.Collection extends VecChangeDescription> changeDescriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription collection is not null.
if (changeDescriptions == null) {
failWithMessage("Expecting changeDescriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getChangeDescriptions(), changeDescriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's changeDescriptions contains only the given VecChangeDescription elements and nothing else in whatever order.
* @param changeDescriptions the given elements that should be contained in actual VecSheetOrChapter's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasOnlyChangeDescriptions(VecChangeDescription... changeDescriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription varargs is not null.
if (changeDescriptions == null) failWithMessage("Expecting changeDescriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getChangeDescriptions(), changeDescriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's changeDescriptions contains only the given VecChangeDescription elements in Collection and nothing else in whatever order.
* @param changeDescriptions the given elements that should be contained in actual VecSheetOrChapter's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's changeDescriptions does not contain all given VecChangeDescription elements.
*/
public S hasOnlyChangeDescriptions(java.util.Collection extends VecChangeDescription> changeDescriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription collection is not null.
if (changeDescriptions == null) {
failWithMessage("Expecting changeDescriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getChangeDescriptions(), changeDescriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's changeDescriptions does not contain the given VecChangeDescription elements.
*
* @param changeDescriptions the given elements that should not be in actual VecSheetOrChapter's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's changeDescriptions contains any given VecChangeDescription elements.
*/
public S doesNotHaveChangeDescriptions(VecChangeDescription... changeDescriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription varargs is not null.
if (changeDescriptions == null) failWithMessage("Expecting changeDescriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getChangeDescriptions(), changeDescriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's changeDescriptions does not contain the given VecChangeDescription elements in Collection.
*
* @param changeDescriptions the given elements that should not be in actual VecSheetOrChapter's changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's changeDescriptions contains any given VecChangeDescription elements.
*/
public S doesNotHaveChangeDescriptions(java.util.Collection extends VecChangeDescription> changeDescriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecChangeDescription collection is not null.
if (changeDescriptions == null) {
failWithMessage("Expecting changeDescriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getChangeDescriptions(), changeDescriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter has no changeDescriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's changeDescriptions is not empty.
*/
public S hasNoChangeDescriptions() {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have changeDescriptions but had :\n <%s>";
// check
if (actual.getChangeDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getChangeDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's descriptions contains the given VecAbstractLocalizedString elements.
* @param descriptions the given elements that should be contained in actual VecSheetOrChapter's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's descriptions contains the given VecAbstractLocalizedString elements in Collection.
* @param descriptions the given elements that should be contained in actual VecSheetOrChapter's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's descriptions contains only the given VecAbstractLocalizedString elements and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecSheetOrChapter's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's descriptions contains only the given VecAbstractLocalizedString elements in Collection and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecSheetOrChapter's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's descriptions does not contain the given VecAbstractLocalizedString elements.
*
* @param descriptions the given elements that should not be in actual VecSheetOrChapter's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's descriptions does not contain the given VecAbstractLocalizedString elements in Collection.
*
* @param descriptions the given elements that should not be in actual VecSheetOrChapter's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter has no descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's descriptions is not empty.
*/
public S hasNoDescriptions() {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have descriptions but had :\n <%s>";
// check
if (actual.getDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's identification is equal to the given one.
* @param identification the given identification to compare the actual VecSheetOrChapter's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSheetOrChapter's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's parentDocumentVersion is equal to the given one.
* @param parentDocumentVersion the given parentDocumentVersion to compare the actual VecSheetOrChapter's parentDocumentVersion to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSheetOrChapter's parentDocumentVersion is not equal to the given one.
*/
public S hasParentDocumentVersion(VecDocumentVersion parentDocumentVersion) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentDocumentVersion of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecDocumentVersion actualParentDocumentVersion = actual.getParentDocumentVersion();
if (!Objects.areEqual(actualParentDocumentVersion, parentDocumentVersion)) {
failWithMessage(assertjErrorMessage, actual, parentDocumentVersion, actualParentDocumentVersion);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentBasedInstruction contains the given VecDocumentBasedInstruction elements.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecSheetOrChapter's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasRefDocumentBasedInstruction(VecDocumentBasedInstruction... refDocumentBasedInstruction) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction varargs is not null.
if (refDocumentBasedInstruction == null) failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentBasedInstruction contains the given VecDocumentBasedInstruction elements in Collection.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecSheetOrChapter's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasRefDocumentBasedInstruction(java.util.Collection extends VecDocumentBasedInstruction> refDocumentBasedInstruction) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction collection is not null.
if (refDocumentBasedInstruction == null) {
failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentBasedInstruction contains only the given VecDocumentBasedInstruction elements and nothing else in whatever order.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecSheetOrChapter's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasOnlyRefDocumentBasedInstruction(VecDocumentBasedInstruction... refDocumentBasedInstruction) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction varargs is not null.
if (refDocumentBasedInstruction == null) failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentBasedInstruction contains only the given VecDocumentBasedInstruction elements in Collection and nothing else in whatever order.
* @param refDocumentBasedInstruction the given elements that should be contained in actual VecSheetOrChapter's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentBasedInstruction does not contain all given VecDocumentBasedInstruction elements.
*/
public S hasOnlyRefDocumentBasedInstruction(java.util.Collection extends VecDocumentBasedInstruction> refDocumentBasedInstruction) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction collection is not null.
if (refDocumentBasedInstruction == null) {
failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentBasedInstruction does not contain the given VecDocumentBasedInstruction elements.
*
* @param refDocumentBasedInstruction the given elements that should not be in actual VecSheetOrChapter's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentBasedInstruction contains any given VecDocumentBasedInstruction elements.
*/
public S doesNotHaveRefDocumentBasedInstruction(VecDocumentBasedInstruction... refDocumentBasedInstruction) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction varargs is not null.
if (refDocumentBasedInstruction == null) failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentBasedInstruction does not contain the given VecDocumentBasedInstruction elements in Collection.
*
* @param refDocumentBasedInstruction the given elements that should not be in actual VecSheetOrChapter's refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentBasedInstruction contains any given VecDocumentBasedInstruction elements.
*/
public S doesNotHaveRefDocumentBasedInstruction(java.util.Collection extends VecDocumentBasedInstruction> refDocumentBasedInstruction) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentBasedInstruction collection is not null.
if (refDocumentBasedInstruction == null) {
failWithMessage("Expecting refDocumentBasedInstruction parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentBasedInstruction(), refDocumentBasedInstruction.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter has no refDocumentBasedInstruction.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentBasedInstruction is not empty.
*/
public S hasNoRefDocumentBasedInstruction() {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDocumentBasedInstruction but had :\n <%s>";
// check
if (actual.getRefDocumentBasedInstruction().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDocumentBasedInstruction());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup contains the given VecDocumentRelatedAssignmentGroup elements.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasRefDocumentRelatedAssignmentGroup(VecDocumentRelatedAssignmentGroup... refDocumentRelatedAssignmentGroup) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup varargs is not null.
if (refDocumentRelatedAssignmentGroup == null) failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup contains the given VecDocumentRelatedAssignmentGroup elements in Collection.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasRefDocumentRelatedAssignmentGroup(java.util.Collection extends VecDocumentRelatedAssignmentGroup> refDocumentRelatedAssignmentGroup) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup collection is not null.
if (refDocumentRelatedAssignmentGroup == null) {
failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup contains only the given VecDocumentRelatedAssignmentGroup elements and nothing else in whatever order.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasOnlyRefDocumentRelatedAssignmentGroup(VecDocumentRelatedAssignmentGroup... refDocumentRelatedAssignmentGroup) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup varargs is not null.
if (refDocumentRelatedAssignmentGroup == null) failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup contains only the given VecDocumentRelatedAssignmentGroup elements in Collection and nothing else in whatever order.
* @param refDocumentRelatedAssignmentGroup the given elements that should be contained in actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup does not contain all given VecDocumentRelatedAssignmentGroup elements.
*/
public S hasOnlyRefDocumentRelatedAssignmentGroup(java.util.Collection extends VecDocumentRelatedAssignmentGroup> refDocumentRelatedAssignmentGroup) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup collection is not null.
if (refDocumentRelatedAssignmentGroup == null) {
failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup does not contain the given VecDocumentRelatedAssignmentGroup elements.
*
* @param refDocumentRelatedAssignmentGroup the given elements that should not be in actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup contains any given VecDocumentRelatedAssignmentGroup elements.
*/
public S doesNotHaveRefDocumentRelatedAssignmentGroup(VecDocumentRelatedAssignmentGroup... refDocumentRelatedAssignmentGroup) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup varargs is not null.
if (refDocumentRelatedAssignmentGroup == null) failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup does not contain the given VecDocumentRelatedAssignmentGroup elements in Collection.
*
* @param refDocumentRelatedAssignmentGroup the given elements that should not be in actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup contains any given VecDocumentRelatedAssignmentGroup elements.
*/
public S doesNotHaveRefDocumentRelatedAssignmentGroup(java.util.Collection extends VecDocumentRelatedAssignmentGroup> refDocumentRelatedAssignmentGroup) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecDocumentRelatedAssignmentGroup collection is not null.
if (refDocumentRelatedAssignmentGroup == null) {
failWithMessage("Expecting refDocumentRelatedAssignmentGroup parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDocumentRelatedAssignmentGroup(), refDocumentRelatedAssignmentGroup.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter has no refDocumentRelatedAssignmentGroup.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's refDocumentRelatedAssignmentGroup is not empty.
*/
public S hasNoRefDocumentRelatedAssignmentGroup() {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDocumentRelatedAssignmentGroup but had :\n <%s>";
// check
if (actual.getRefDocumentRelatedAssignmentGroup().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDocumentRelatedAssignmentGroup());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's referencedPart contains the given VecPartVersion elements.
* @param referencedPart the given elements that should be contained in actual VecSheetOrChapter's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasReferencedPart(VecPartVersion... referencedPart) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion varargs is not null.
if (referencedPart == null) failWithMessage("Expecting referencedPart parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getReferencedPart(), referencedPart);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's referencedPart contains the given VecPartVersion elements in Collection.
* @param referencedPart the given elements that should be contained in actual VecSheetOrChapter's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasReferencedPart(java.util.Collection extends VecPartVersion> referencedPart) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion collection is not null.
if (referencedPart == null) {
failWithMessage("Expecting referencedPart parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getReferencedPart(), referencedPart.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's referencedPart contains only the given VecPartVersion elements and nothing else in whatever order.
* @param referencedPart the given elements that should be contained in actual VecSheetOrChapter's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasOnlyReferencedPart(VecPartVersion... referencedPart) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion varargs is not null.
if (referencedPart == null) failWithMessage("Expecting referencedPart parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getReferencedPart(), referencedPart);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's referencedPart contains only the given VecPartVersion elements in Collection and nothing else in whatever order.
* @param referencedPart the given elements that should be contained in actual VecSheetOrChapter's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's referencedPart does not contain all given VecPartVersion elements.
*/
public S hasOnlyReferencedPart(java.util.Collection extends VecPartVersion> referencedPart) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion collection is not null.
if (referencedPart == null) {
failWithMessage("Expecting referencedPart parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getReferencedPart(), referencedPart.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's referencedPart does not contain the given VecPartVersion elements.
*
* @param referencedPart the given elements that should not be in actual VecSheetOrChapter's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's referencedPart contains any given VecPartVersion elements.
*/
public S doesNotHaveReferencedPart(VecPartVersion... referencedPart) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion varargs is not null.
if (referencedPart == null) failWithMessage("Expecting referencedPart parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getReferencedPart(), referencedPart);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's referencedPart does not contain the given VecPartVersion elements in Collection.
*
* @param referencedPart the given elements that should not be in actual VecSheetOrChapter's referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's referencedPart contains any given VecPartVersion elements.
*/
public S doesNotHaveReferencedPart(java.util.Collection extends VecPartVersion> referencedPart) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecPartVersion collection is not null.
if (referencedPart == null) {
failWithMessage("Expecting referencedPart parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getReferencedPart(), referencedPart.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter has no referencedPart.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's referencedPart is not empty.
*/
public S hasNoReferencedPart() {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have referencedPart but had :\n <%s>";
// check
if (actual.getReferencedPart().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getReferencedPart());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's sheetFormat is equal to the given one.
* @param sheetFormat the given sheetFormat to compare the actual VecSheetOrChapter's sheetFormat to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSheetOrChapter's sheetFormat is not equal to the given one.
*/
public S hasSheetFormat(String sheetFormat) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting sheetFormat of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSheetFormat = actual.getSheetFormat();
if (!Objects.areEqual(actualSheetFormat, sheetFormat)) {
failWithMessage(assertjErrorMessage, actual, sheetFormat, actualSheetFormat);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's sheetNumber is equal to the given one.
* @param sheetNumber the given sheetNumber to compare the actual VecSheetOrChapter's sheetNumber to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSheetOrChapter's sheetNumber is not equal to the given one.
*/
public S hasSheetNumber(String sheetNumber) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting sheetNumber of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSheetNumber = actual.getSheetNumber();
if (!Objects.areEqual(actualSheetNumber, sheetNumber)) {
failWithMessage(assertjErrorMessage, actual, sheetNumber, actualSheetNumber);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's sheetVersion is equal to the given one.
* @param sheetVersion the given sheetVersion to compare the actual VecSheetOrChapter's sheetVersion to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSheetOrChapter's sheetVersion is not equal to the given one.
*/
public S hasSheetVersion(String sheetVersion) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting sheetVersion of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSheetVersion = actual.getSheetVersion();
if (!Objects.areEqual(actualSheetVersion, sheetVersion)) {
failWithMessage(assertjErrorMessage, actual, sheetVersion, actualSheetVersion);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's specifications contains the given VecSpecification elements.
* @param specifications the given elements that should be contained in actual VecSheetOrChapter's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's specifications does not contain all given VecSpecification elements.
*/
public S hasSpecifications(VecSpecification... specifications) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification varargs is not null.
if (specifications == null) failWithMessage("Expecting specifications parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSpecifications(), specifications);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's specifications contains the given VecSpecification elements in Collection.
* @param specifications the given elements that should be contained in actual VecSheetOrChapter's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's specifications does not contain all given VecSpecification elements.
*/
public S hasSpecifications(java.util.Collection extends VecSpecification> specifications) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification collection is not null.
if (specifications == null) {
failWithMessage("Expecting specifications parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSpecifications(), specifications.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's specifications contains only the given VecSpecification elements and nothing else in whatever order.
* @param specifications the given elements that should be contained in actual VecSheetOrChapter's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's specifications does not contain all given VecSpecification elements.
*/
public S hasOnlySpecifications(VecSpecification... specifications) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification varargs is not null.
if (specifications == null) failWithMessage("Expecting specifications parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSpecifications(), specifications);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's specifications contains only the given VecSpecification elements in Collection and nothing else in whatever order.
* @param specifications the given elements that should be contained in actual VecSheetOrChapter's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's specifications does not contain all given VecSpecification elements.
*/
public S hasOnlySpecifications(java.util.Collection extends VecSpecification> specifications) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification collection is not null.
if (specifications == null) {
failWithMessage("Expecting specifications parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSpecifications(), specifications.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's specifications does not contain the given VecSpecification elements.
*
* @param specifications the given elements that should not be in actual VecSheetOrChapter's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's specifications contains any given VecSpecification elements.
*/
public S doesNotHaveSpecifications(VecSpecification... specifications) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification varargs is not null.
if (specifications == null) failWithMessage("Expecting specifications parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSpecifications(), specifications);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter's specifications does not contain the given VecSpecification elements in Collection.
*
* @param specifications the given elements that should not be in actual VecSheetOrChapter's specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's specifications contains any given VecSpecification elements.
*/
public S doesNotHaveSpecifications(java.util.Collection extends VecSpecification> specifications) {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// check that given VecSpecification collection is not null.
if (specifications == null) {
failWithMessage("Expecting specifications parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSpecifications(), specifications.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSheetOrChapter has no specifications.
* @return this assertion object.
* @throws AssertionError if the actual VecSheetOrChapter's specifications is not empty.
*/
public S hasNoSpecifications() {
// check that actual VecSheetOrChapter we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have specifications but had :\n <%s>";
// check
if (actual.getSpecifications().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getSpecifications());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy