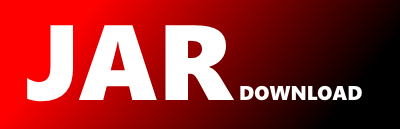
com.foursoft.vecmodel.vec120.AbstractVecSignalAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecSignal} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecSignalAssert, A extends VecSignal> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecSignalAssert}
to make assertions on actual VecSignal.
* @param actual the VecSignal we want to make assertions on.
*/
protected AbstractVecSignalAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecSignal's aliasIds contains the given VecAliasIdentification elements.
* @param aliasIds the given elements that should be contained in actual VecSignal's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's aliasIds contains the given VecAliasIdentification elements in Collection.
* @param aliasIds the given elements that should be contained in actual VecSignal's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's aliasIds contains only the given VecAliasIdentification elements and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecSignal's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's aliasIds contains only the given VecAliasIdentification elements in Collection and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecSignal's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's aliasIds does not contain the given VecAliasIdentification elements.
*
* @param aliasIds the given elements that should not be in actual VecSignal's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's aliasIds does not contain the given VecAliasIdentification elements in Collection.
*
* @param aliasIds the given elements that should not be in actual VecSignal's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal has no aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's aliasIds is not empty.
*/
public S hasNoAliasIds() {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have aliasIds but had :\n <%s>";
// check
if (actual.getAliasIds().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAliasIds());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's clampName is equal to the given one.
* @param clampName the given clampName to compare the actual VecSignal's clampName to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's clampName is not equal to the given one.
*/
public S hasClampName(String clampName) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting clampName of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualClampName = actual.getClampName();
if (!Objects.areEqual(actualClampName, clampName)) {
failWithMessage(assertjErrorMessage, actual, clampName, actualClampName);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's currentType is equal to the given one.
* @param currentType the given currentType to compare the actual VecSignal's currentType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's currentType is not equal to the given one.
*/
public S hasCurrentType(String currentType) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting currentType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualCurrentType = actual.getCurrentType();
if (!Objects.areEqual(actualCurrentType, currentType)) {
failWithMessage(assertjErrorMessage, actual, currentType, actualCurrentType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's dataRate is equal to the given one.
* @param dataRate the given dataRate to compare the actual VecSignal's dataRate to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's dataRate is not equal to the given one.
*/
public S hasDataRate(VecNumericalValue dataRate) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting dataRate of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualDataRate = actual.getDataRate();
if (!Objects.areEqual(actualDataRate, dataRate)) {
failWithMessage(assertjErrorMessage, actual, dataRate, actualDataRate);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's descriptions contains the given VecAbstractLocalizedString elements.
* @param descriptions the given elements that should be contained in actual VecSignal's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's descriptions contains the given VecAbstractLocalizedString elements in Collection.
* @param descriptions the given elements that should be contained in actual VecSignal's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's descriptions contains only the given VecAbstractLocalizedString elements and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecSignal's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's descriptions contains only the given VecAbstractLocalizedString elements in Collection and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecSignal's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's descriptions does not contain the given VecAbstractLocalizedString elements.
*
* @param descriptions the given elements that should not be in actual VecSignal's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's descriptions does not contain the given VecAbstractLocalizedString elements in Collection.
*
* @param descriptions the given elements that should not be in actual VecSignal's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal has no descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's descriptions is not empty.
*/
public S hasNoDescriptions() {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have descriptions but had :\n <%s>";
// check
if (actual.getDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's identification is equal to the given one.
* @param identification the given identification to compare the actual VecSignal's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's netType is equal to the given one.
* @param netType the given netType to compare the actual VecSignal's netType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's netType is not equal to the given one.
*/
public S hasNetType(VecNetType netType) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting netType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNetType actualNetType = actual.getNetType();
if (!Objects.areEqual(actualNetType, netType)) {
failWithMessage(assertjErrorMessage, actual, netType, actualNetType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's nominalVoltage is equal to the given one.
* @param nominalVoltage the given nominalVoltage to compare the actual VecSignal's nominalVoltage to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's nominalVoltage is not equal to the given one.
*/
public S hasNominalVoltage(String nominalVoltage) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting nominalVoltage of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualNominalVoltage = actual.getNominalVoltage();
if (!Objects.areEqual(actualNominalVoltage, nominalVoltage)) {
failWithMessage(assertjErrorMessage, actual, nominalVoltage, actualNominalVoltage);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's parentSignalSpecification is equal to the given one.
* @param parentSignalSpecification the given parentSignalSpecification to compare the actual VecSignal's parentSignalSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's parentSignalSpecification is not equal to the given one.
*/
public S hasParentSignalSpecification(VecSignalSpecification parentSignalSpecification) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentSignalSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSignalSpecification actualParentSignalSpecification = actual.getParentSignalSpecification();
if (!Objects.areEqual(actualParentSignalSpecification, parentSignalSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentSignalSpecification, actualParentSignalSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's recommendedConductorSpecification is equal to the given one.
* @param recommendedConductorSpecification the given recommendedConductorSpecification to compare the actual VecSignal's recommendedConductorSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's recommendedConductorSpecification is not equal to the given one.
*/
public S hasRecommendedConductorSpecification(VecConductorSpecification recommendedConductorSpecification) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting recommendedConductorSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecConductorSpecification actualRecommendedConductorSpecification = actual.getRecommendedConductorSpecification();
if (!Objects.areEqual(actualRecommendedConductorSpecification, recommendedConductorSpecification)) {
failWithMessage(assertjErrorMessage, actual, recommendedConductorSpecification, actualRecommendedConductorSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's recommendedInsulationSpecification is equal to the given one.
* @param recommendedInsulationSpecification the given recommendedInsulationSpecification to compare the actual VecSignal's recommendedInsulationSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's recommendedInsulationSpecification is not equal to the given one.
*/
public S hasRecommendedInsulationSpecification(VecInsulationSpecification recommendedInsulationSpecification) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting recommendedInsulationSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecInsulationSpecification actualRecommendedInsulationSpecification = actual.getRecommendedInsulationSpecification();
if (!Objects.areEqual(actualRecommendedInsulationSpecification, recommendedInsulationSpecification)) {
failWithMessage(assertjErrorMessage, actual, recommendedInsulationSpecification, actualRecommendedInsulationSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refComponentPort contains the given VecComponentPort elements.
* @param refComponentPort the given elements that should be contained in actual VecSignal's refComponentPort.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refComponentPort does not contain all given VecComponentPort elements.
*/
public S hasRefComponentPort(VecComponentPort... refComponentPort) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecComponentPort varargs is not null.
if (refComponentPort == null) failWithMessage("Expecting refComponentPort parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefComponentPort(), refComponentPort);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refComponentPort contains the given VecComponentPort elements in Collection.
* @param refComponentPort the given elements that should be contained in actual VecSignal's refComponentPort.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refComponentPort does not contain all given VecComponentPort elements.
*/
public S hasRefComponentPort(java.util.Collection extends VecComponentPort> refComponentPort) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecComponentPort collection is not null.
if (refComponentPort == null) {
failWithMessage("Expecting refComponentPort parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefComponentPort(), refComponentPort.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refComponentPort contains only the given VecComponentPort elements and nothing else in whatever order.
* @param refComponentPort the given elements that should be contained in actual VecSignal's refComponentPort.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refComponentPort does not contain all given VecComponentPort elements.
*/
public S hasOnlyRefComponentPort(VecComponentPort... refComponentPort) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecComponentPort varargs is not null.
if (refComponentPort == null) failWithMessage("Expecting refComponentPort parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefComponentPort(), refComponentPort);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refComponentPort contains only the given VecComponentPort elements in Collection and nothing else in whatever order.
* @param refComponentPort the given elements that should be contained in actual VecSignal's refComponentPort.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refComponentPort does not contain all given VecComponentPort elements.
*/
public S hasOnlyRefComponentPort(java.util.Collection extends VecComponentPort> refComponentPort) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecComponentPort collection is not null.
if (refComponentPort == null) {
failWithMessage("Expecting refComponentPort parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefComponentPort(), refComponentPort.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refComponentPort does not contain the given VecComponentPort elements.
*
* @param refComponentPort the given elements that should not be in actual VecSignal's refComponentPort.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refComponentPort contains any given VecComponentPort elements.
*/
public S doesNotHaveRefComponentPort(VecComponentPort... refComponentPort) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecComponentPort varargs is not null.
if (refComponentPort == null) failWithMessage("Expecting refComponentPort parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefComponentPort(), refComponentPort);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refComponentPort does not contain the given VecComponentPort elements in Collection.
*
* @param refComponentPort the given elements that should not be in actual VecSignal's refComponentPort.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refComponentPort contains any given VecComponentPort elements.
*/
public S doesNotHaveRefComponentPort(java.util.Collection extends VecComponentPort> refComponentPort) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecComponentPort collection is not null.
if (refComponentPort == null) {
failWithMessage("Expecting refComponentPort parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefComponentPort(), refComponentPort.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal has no refComponentPort.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refComponentPort is not empty.
*/
public S hasNoRefComponentPort() {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refComponentPort but had :\n <%s>";
// check
if (actual.getRefComponentPort().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefComponentPort());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refConnection contains the given VecConnection elements.
* @param refConnection the given elements that should be contained in actual VecSignal's refConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refConnection does not contain all given VecConnection elements.
*/
public S hasRefConnection(VecConnection... refConnection) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecConnection varargs is not null.
if (refConnection == null) failWithMessage("Expecting refConnection parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConnection(), refConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refConnection contains the given VecConnection elements in Collection.
* @param refConnection the given elements that should be contained in actual VecSignal's refConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refConnection does not contain all given VecConnection elements.
*/
public S hasRefConnection(java.util.Collection extends VecConnection> refConnection) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecConnection collection is not null.
if (refConnection == null) {
failWithMessage("Expecting refConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConnection(), refConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refConnection contains only the given VecConnection elements and nothing else in whatever order.
* @param refConnection the given elements that should be contained in actual VecSignal's refConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refConnection does not contain all given VecConnection elements.
*/
public S hasOnlyRefConnection(VecConnection... refConnection) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecConnection varargs is not null.
if (refConnection == null) failWithMessage("Expecting refConnection parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConnection(), refConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refConnection contains only the given VecConnection elements in Collection and nothing else in whatever order.
* @param refConnection the given elements that should be contained in actual VecSignal's refConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refConnection does not contain all given VecConnection elements.
*/
public S hasOnlyRefConnection(java.util.Collection extends VecConnection> refConnection) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecConnection collection is not null.
if (refConnection == null) {
failWithMessage("Expecting refConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConnection(), refConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refConnection does not contain the given VecConnection elements.
*
* @param refConnection the given elements that should not be in actual VecSignal's refConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refConnection contains any given VecConnection elements.
*/
public S doesNotHaveRefConnection(VecConnection... refConnection) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecConnection varargs is not null.
if (refConnection == null) failWithMessage("Expecting refConnection parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConnection(), refConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refConnection does not contain the given VecConnection elements in Collection.
*
* @param refConnection the given elements that should not be in actual VecSignal's refConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refConnection contains any given VecConnection elements.
*/
public S doesNotHaveRefConnection(java.util.Collection extends VecConnection> refConnection) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecConnection collection is not null.
if (refConnection == null) {
failWithMessage("Expecting refConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConnection(), refConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal has no refConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refConnection is not empty.
*/
public S hasNoRefConnection() {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refConnection but had :\n <%s>";
// check
if (actual.getRefConnection().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefConnection());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refPinComponentBehavior contains the given VecPinComponentBehavior elements.
* @param refPinComponentBehavior the given elements that should be contained in actual VecSignal's refPinComponentBehavior.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refPinComponentBehavior does not contain all given VecPinComponentBehavior elements.
*/
public S hasRefPinComponentBehavior(VecPinComponentBehavior... refPinComponentBehavior) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior varargs is not null.
if (refPinComponentBehavior == null) failWithMessage("Expecting refPinComponentBehavior parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponentBehavior(), refPinComponentBehavior);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refPinComponentBehavior contains the given VecPinComponentBehavior elements in Collection.
* @param refPinComponentBehavior the given elements that should be contained in actual VecSignal's refPinComponentBehavior.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refPinComponentBehavior does not contain all given VecPinComponentBehavior elements.
*/
public S hasRefPinComponentBehavior(java.util.Collection extends VecPinComponentBehavior> refPinComponentBehavior) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior collection is not null.
if (refPinComponentBehavior == null) {
failWithMessage("Expecting refPinComponentBehavior parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponentBehavior(), refPinComponentBehavior.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refPinComponentBehavior contains only the given VecPinComponentBehavior elements and nothing else in whatever order.
* @param refPinComponentBehavior the given elements that should be contained in actual VecSignal's refPinComponentBehavior.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refPinComponentBehavior does not contain all given VecPinComponentBehavior elements.
*/
public S hasOnlyRefPinComponentBehavior(VecPinComponentBehavior... refPinComponentBehavior) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior varargs is not null.
if (refPinComponentBehavior == null) failWithMessage("Expecting refPinComponentBehavior parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponentBehavior(), refPinComponentBehavior);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refPinComponentBehavior contains only the given VecPinComponentBehavior elements in Collection and nothing else in whatever order.
* @param refPinComponentBehavior the given elements that should be contained in actual VecSignal's refPinComponentBehavior.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refPinComponentBehavior does not contain all given VecPinComponentBehavior elements.
*/
public S hasOnlyRefPinComponentBehavior(java.util.Collection extends VecPinComponentBehavior> refPinComponentBehavior) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior collection is not null.
if (refPinComponentBehavior == null) {
failWithMessage("Expecting refPinComponentBehavior parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponentBehavior(), refPinComponentBehavior.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refPinComponentBehavior does not contain the given VecPinComponentBehavior elements.
*
* @param refPinComponentBehavior the given elements that should not be in actual VecSignal's refPinComponentBehavior.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refPinComponentBehavior contains any given VecPinComponentBehavior elements.
*/
public S doesNotHaveRefPinComponentBehavior(VecPinComponentBehavior... refPinComponentBehavior) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior varargs is not null.
if (refPinComponentBehavior == null) failWithMessage("Expecting refPinComponentBehavior parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponentBehavior(), refPinComponentBehavior);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refPinComponentBehavior does not contain the given VecPinComponentBehavior elements in Collection.
*
* @param refPinComponentBehavior the given elements that should not be in actual VecSignal's refPinComponentBehavior.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refPinComponentBehavior contains any given VecPinComponentBehavior elements.
*/
public S doesNotHaveRefPinComponentBehavior(java.util.Collection extends VecPinComponentBehavior> refPinComponentBehavior) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponentBehavior collection is not null.
if (refPinComponentBehavior == null) {
failWithMessage("Expecting refPinComponentBehavior parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponentBehavior(), refPinComponentBehavior.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal has no refPinComponentBehavior.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refPinComponentBehavior is not empty.
*/
public S hasNoRefPinComponentBehavior() {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPinComponentBehavior but had :\n <%s>";
// check
if (actual.getRefPinComponentBehavior().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPinComponentBehavior());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refWireElementReference contains the given VecWireElementReference elements.
* @param refWireElementReference the given elements that should be contained in actual VecSignal's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasRefWireElementReference(VecWireElementReference... refWireElementReference) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference varargs is not null.
if (refWireElementReference == null) failWithMessage("Expecting refWireElementReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementReference(), refWireElementReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refWireElementReference contains the given VecWireElementReference elements in Collection.
* @param refWireElementReference the given elements that should be contained in actual VecSignal's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasRefWireElementReference(java.util.Collection extends VecWireElementReference> refWireElementReference) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference collection is not null.
if (refWireElementReference == null) {
failWithMessage("Expecting refWireElementReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementReference(), refWireElementReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refWireElementReference contains only the given VecWireElementReference elements and nothing else in whatever order.
* @param refWireElementReference the given elements that should be contained in actual VecSignal's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasOnlyRefWireElementReference(VecWireElementReference... refWireElementReference) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference varargs is not null.
if (refWireElementReference == null) failWithMessage("Expecting refWireElementReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementReference(), refWireElementReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refWireElementReference contains only the given VecWireElementReference elements in Collection and nothing else in whatever order.
* @param refWireElementReference the given elements that should be contained in actual VecSignal's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refWireElementReference does not contain all given VecWireElementReference elements.
*/
public S hasOnlyRefWireElementReference(java.util.Collection extends VecWireElementReference> refWireElementReference) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference collection is not null.
if (refWireElementReference == null) {
failWithMessage("Expecting refWireElementReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementReference(), refWireElementReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refWireElementReference does not contain the given VecWireElementReference elements.
*
* @param refWireElementReference the given elements that should not be in actual VecSignal's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refWireElementReference contains any given VecWireElementReference elements.
*/
public S doesNotHaveRefWireElementReference(VecWireElementReference... refWireElementReference) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference varargs is not null.
if (refWireElementReference == null) failWithMessage("Expecting refWireElementReference parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementReference(), refWireElementReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's refWireElementReference does not contain the given VecWireElementReference elements in Collection.
*
* @param refWireElementReference the given elements that should not be in actual VecSignal's refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refWireElementReference contains any given VecWireElementReference elements.
*/
public S doesNotHaveRefWireElementReference(java.util.Collection extends VecWireElementReference> refWireElementReference) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementReference collection is not null.
if (refWireElementReference == null) {
failWithMessage("Expecting refWireElementReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementReference(), refWireElementReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal has no refWireElementReference.
* @return this assertion object.
* @throws AssertionError if the actual VecSignal's refWireElementReference is not empty.
*/
public S hasNoRefWireElementReference() {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireElementReference but had :\n <%s>";
// check
if (actual.getRefWireElementReference().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireElementReference());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's signalCurve is equal to the given one.
* @param signalCurve the given signalCurve to compare the actual VecSignal's signalCurve to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's signalCurve is not equal to the given one.
*/
public S hasSignalCurve(String signalCurve) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalCurve of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSignalCurve = actual.getSignalCurve();
if (!Objects.areEqual(actualSignalCurve, signalCurve)) {
failWithMessage(assertjErrorMessage, actual, signalCurve, actualSignalCurve);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's signalForm is equal to the given one.
* @param signalForm the given signalForm to compare the actual VecSignal's signalForm to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's signalForm is not equal to the given one.
*/
public S hasSignalForm(String signalForm) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalForm of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSignalForm = actual.getSignalForm();
if (!Objects.areEqual(actualSignalForm, signalForm)) {
failWithMessage(assertjErrorMessage, actual, signalForm, actualSignalForm);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's signalInformationType is equal to the given one.
* @param signalInformationType the given signalInformationType to compare the actual VecSignal's signalInformationType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's signalInformationType is not equal to the given one.
*/
public S hasSignalInformationType(String signalInformationType) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalInformationType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSignalInformationType = actual.getSignalInformationType();
if (!Objects.areEqual(actualSignalInformationType, signalInformationType)) {
failWithMessage(assertjErrorMessage, actual, signalInformationType, actualSignalInformationType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's signalName is equal to the given one.
* @param signalName the given signalName to compare the actual VecSignal's signalName to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's signalName is not equal to the given one.
*/
public S hasSignalName(String signalName) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalName of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSignalName = actual.getSignalName();
if (!Objects.areEqual(actualSignalName, signalName)) {
failWithMessage(assertjErrorMessage, actual, signalName, actualSignalName);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's signalSubType is equal to the given one.
* @param signalSubType the given signalSubType to compare the actual VecSignal's signalSubType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's signalSubType is not equal to the given one.
*/
public S hasSignalSubType(String signalSubType) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalSubType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSignalSubType = actual.getSignalSubType();
if (!Objects.areEqual(actualSignalSubType, signalSubType)) {
failWithMessage(assertjErrorMessage, actual, signalSubType, actualSignalSubType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's signalTransmissionMediumType is equal to the given one.
* @param signalTransmissionMediumType the given signalTransmissionMediumType to compare the actual VecSignal's signalTransmissionMediumType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's signalTransmissionMediumType is not equal to the given one.
*/
public S hasSignalTransmissionMediumType(String signalTransmissionMediumType) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalTransmissionMediumType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSignalTransmissionMediumType = actual.getSignalTransmissionMediumType();
if (!Objects.areEqual(actualSignalTransmissionMediumType, signalTransmissionMediumType)) {
failWithMessage(assertjErrorMessage, actual, signalTransmissionMediumType, actualSignalTransmissionMediumType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecSignal's signalType is equal to the given one.
* @param signalType the given signalType to compare the actual VecSignal's signalType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecSignal's signalType is not equal to the given one.
*/
public S hasSignalType(String signalType) {
// check that actual VecSignal we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSignalType = actual.getSignalType();
if (!Objects.areEqual(actualSignalType, signalType)) {
failWithMessage(assertjErrorMessage, actual, signalType, actualSignalType);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy