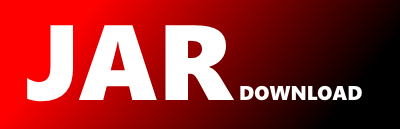
com.foursoft.vecmodel.vec120.AbstractVecTapeRoleAssert Maven / Gradle / Ivy
Show all versions of vec120-assertions Show documentation
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.Assertions;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTapeRole} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTapeRoleAssert, A extends VecTapeRole> extends AbstractVecWireProtectionRoleAssert {
/**
* Creates a new {@link AbstractVecTapeRoleAssert}
to make assertions on actual VecTapeRole.
* @param actual the VecTapeRole we want to make assertions on.
*/
protected AbstractVecTapeRoleAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTapeRole's gradient is equal to the given one.
* @param gradient the given gradient to compare the actual VecTapeRole's gradient to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTapeRole's gradient is not equal to the given one.
*/
public S hasGradient(VecValueWithUnit gradient) {
// check that actual VecTapeRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting gradient of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecValueWithUnit actualGradient = actual.getGradient();
if (!Objects.areEqual(actualGradient, gradient)) {
failWithMessage(assertjErrorMessage, actual, gradient, actualGradient);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTapeRole's tapeOverlap is equal to the given one.
* @param tapeOverlap the given tapeOverlap to compare the actual VecTapeRole's tapeOverlap to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTapeRole's tapeOverlap is not equal to the given one.
*/
public S hasTapeOverlap(VecNumericalValue tapeOverlap) {
// check that actual VecTapeRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting tapeOverlap of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualTapeOverlap = actual.getTapeOverlap();
if (!Objects.areEqual(actualTapeOverlap, tapeOverlap)) {
failWithMessage(assertjErrorMessage, actual, tapeOverlap, actualTapeOverlap);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTapeRole's tapeOverlapRelative is equal to the given one.
* @param tapeOverlapRelative the given tapeOverlapRelative to compare the actual VecTapeRole's tapeOverlapRelative to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTapeRole's tapeOverlapRelative is not equal to the given one.
*/
public S hasTapeOverlapRelative(Double tapeOverlapRelative) {
// check that actual VecTapeRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting tapeOverlapRelative of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Double actualTapeOverlapRelative = actual.getTapeOverlapRelative();
if (!Objects.areEqual(actualTapeOverlapRelative, tapeOverlapRelative)) {
failWithMessage(assertjErrorMessage, actual, tapeOverlapRelative, actualTapeOverlapRelative);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTapeRole's tapeOverlapRelative is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param tapeOverlapRelative the value to compare the actual VecTapeRole's tapeOverlapRelative to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecTapeRole's tapeOverlapRelative is not close enough to the given value.
*/
public S hasTapeOverlapRelativeCloseTo(Double tapeOverlapRelative, Double assertjOffset) {
// check that actual VecTapeRole we want to make assertions on is not null.
isNotNull();
Double actualTapeOverlapRelative = actual.getTapeOverlapRelative();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting tapeOverlapRelative:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualTapeOverlapRelative, tapeOverlapRelative, assertjOffset, Math.abs(tapeOverlapRelative - actualTapeOverlapRelative));
// check
Assertions.assertThat(actualTapeOverlapRelative).overridingErrorMessage(assertjErrorMessage).isCloseTo(tapeOverlapRelative, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTapeRole's tapingDirection is equal to the given one.
* @param tapingDirection the given tapingDirection to compare the actual VecTapeRole's tapingDirection to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTapeRole's tapingDirection is not equal to the given one.
*/
public S hasTapingDirection(VecTapingDirection tapingDirection) {
// check that actual VecTapeRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting tapingDirection of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTapingDirection actualTapingDirection = actual.getTapingDirection();
if (!Objects.areEqual(actualTapingDirection, tapingDirection)) {
failWithMessage(assertjErrorMessage, actual, tapingDirection, actualTapingDirection);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTapeRole's windingFirmness is equal to the given one.
* @param windingFirmness the given windingFirmness to compare the actual VecTapeRole's windingFirmness to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTapeRole's windingFirmness is not equal to the given one.
*/
public S hasWindingFirmness(String windingFirmness) {
// check that actual VecTapeRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting windingFirmness of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualWindingFirmness = actual.getWindingFirmness();
if (!Objects.areEqual(actualWindingFirmness, windingFirmness)) {
failWithMessage(assertjErrorMessage, actual, windingFirmness, actualWindingFirmness);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTapeRole's windingType is equal to the given one.
* @param windingType the given windingType to compare the actual VecTapeRole's windingType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTapeRole's windingType is not equal to the given one.
*/
public S hasWindingType(String windingType) {
// check that actual VecTapeRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting windingType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualWindingType = actual.getWindingType();
if (!Objects.areEqual(actualWindingType, windingType)) {
failWithMessage(assertjErrorMessage, actual, windingType, actualWindingType);
}
// return the current assertion for method chaining
return myself;
}
}