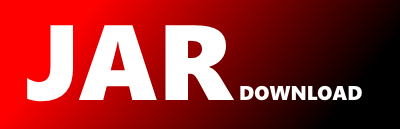
com.foursoft.vecmodel.vec120.AbstractVecTerminalPairingAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTerminalPairing} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTerminalPairingAssert, A extends VecTerminalPairing> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecTerminalPairingAssert}
to make assertions on actual VecTerminalPairing.
* @param actual the VecTerminalPairing we want to make assertions on.
*/
protected AbstractVecTerminalPairingAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTerminalPairing's contactResistance is equal to the given one.
* @param contactResistance the given contactResistance to compare the actual VecTerminalPairing's contactResistance to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalPairing's contactResistance is not equal to the given one.
*/
public S hasContactResistance(VecNumericalValue contactResistance) {
// check that actual VecTerminalPairing we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting contactResistance of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualContactResistance = actual.getContactResistance();
if (!Objects.areEqual(actualContactResistance, contactResistance)) {
failWithMessage(assertjErrorMessage, actual, contactResistance, actualContactResistance);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalPairing's firstTerminal is equal to the given one.
* @param firstTerminal the given firstTerminal to compare the actual VecTerminalPairing's firstTerminal to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalPairing's firstTerminal is not equal to the given one.
*/
public S hasFirstTerminal(VecPartVersion firstTerminal) {
// check that actual VecTerminalPairing we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting firstTerminal of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPartVersion actualFirstTerminal = actual.getFirstTerminal();
if (!Objects.areEqual(actualFirstTerminal, firstTerminal)) {
failWithMessage(assertjErrorMessage, actual, firstTerminal, actualFirstTerminal);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalPairing's matingForce is equal to the given one.
* @param matingForce the given matingForce to compare the actual VecTerminalPairing's matingForce to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalPairing's matingForce is not equal to the given one.
*/
public S hasMatingForce(VecNumericalValue matingForce) {
// check that actual VecTerminalPairing we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting matingForce of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualMatingForce = actual.getMatingForce();
if (!Objects.areEqual(actualMatingForce, matingForce)) {
failWithMessage(assertjErrorMessage, actual, matingForce, actualMatingForce);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalPairing's parentTerminalPairingSpecification is equal to the given one.
* @param parentTerminalPairingSpecification the given parentTerminalPairingSpecification to compare the actual VecTerminalPairing's parentTerminalPairingSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalPairing's parentTerminalPairingSpecification is not equal to the given one.
*/
public S hasParentTerminalPairingSpecification(VecTerminalPairingSpecification parentTerminalPairingSpecification) {
// check that actual VecTerminalPairing we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentTerminalPairingSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTerminalPairingSpecification actualParentTerminalPairingSpecification = actual.getParentTerminalPairingSpecification();
if (!Objects.areEqual(actualParentTerminalPairingSpecification, parentTerminalPairingSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentTerminalPairingSpecification, actualParentTerminalPairingSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalPairing's referencedCoreSpecification is equal to the given one.
* @param referencedCoreSpecification the given referencedCoreSpecification to compare the actual VecTerminalPairing's referencedCoreSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalPairing's referencedCoreSpecification is not equal to the given one.
*/
public S hasReferencedCoreSpecification(VecConductorSpecification referencedCoreSpecification) {
// check that actual VecTerminalPairing we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting referencedCoreSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecConductorSpecification actualReferencedCoreSpecification = actual.getReferencedCoreSpecification();
if (!Objects.areEqual(actualReferencedCoreSpecification, referencedCoreSpecification)) {
failWithMessage(assertjErrorMessage, actual, referencedCoreSpecification, actualReferencedCoreSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalPairing's secondTerminal is equal to the given one.
* @param secondTerminal the given secondTerminal to compare the actual VecTerminalPairing's secondTerminal to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalPairing's secondTerminal is not equal to the given one.
*/
public S hasSecondTerminal(VecPartVersion secondTerminal) {
// check that actual VecTerminalPairing we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting secondTerminal of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPartVersion actualSecondTerminal = actual.getSecondTerminal();
if (!Objects.areEqual(actualSecondTerminal, secondTerminal)) {
failWithMessage(assertjErrorMessage, actual, secondTerminal, actualSecondTerminal);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalPairing's unmatingForce is equal to the given one.
* @param unmatingForce the given unmatingForce to compare the actual VecTerminalPairing's unmatingForce to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalPairing's unmatingForce is not equal to the given one.
*/
public S hasUnmatingForce(VecNumericalValue unmatingForce) {
// check that actual VecTerminalPairing we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting unmatingForce of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualUnmatingForce = actual.getUnmatingForce();
if (!Objects.areEqual(actualUnmatingForce, unmatingForce)) {
failWithMessage(assertjErrorMessage, actual, unmatingForce, actualUnmatingForce);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy