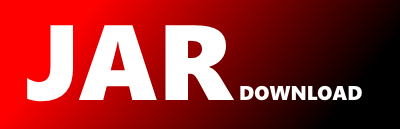
com.foursoft.vecmodel.vec120.AbstractVecTerminalRoleAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTerminalRole} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTerminalRoleAssert, A extends VecTerminalRole> extends AbstractVecRoleAssert {
/**
* Creates a new {@link AbstractVecTerminalRoleAssert}
to make assertions on actual VecTerminalRole.
* @param actual the VecTerminalRole we want to make assertions on.
*/
protected AbstractVecTerminalRoleAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTerminalRole's componentPort is equal to the given one.
* @param componentPort the given componentPort to compare the actual VecTerminalRole's componentPort to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalRole's componentPort is not equal to the given one.
*/
public S hasComponentPort(VecComponentPort componentPort) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting componentPort of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecComponentPort actualComponentPort = actual.getComponentPort();
if (!Objects.areEqual(actualComponentPort, componentPort)) {
failWithMessage(assertjErrorMessage, actual, componentPort, actualComponentPort);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's parentCavityReference is equal to the given one.
* @param parentCavityReference the given parentCavityReference to compare the actual VecTerminalRole's parentCavityReference to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalRole's parentCavityReference is not equal to the given one.
*/
public S hasParentCavityReference(VecCavityReference parentCavityReference) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentCavityReference of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCavityReference actualParentCavityReference = actual.getParentCavityReference();
if (!Objects.areEqual(actualParentCavityReference, parentCavityReference)) {
failWithMessage(assertjErrorMessage, actual, parentCavityReference, actualParentCavityReference);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's parentPinComponentReference is equal to the given one.
* @param parentPinComponentReference the given parentPinComponentReference to compare the actual VecTerminalRole's parentPinComponentReference to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalRole's parentPinComponentReference is not equal to the given one.
*/
public S hasParentPinComponentReference(VecPinComponentReference parentPinComponentReference) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentPinComponentReference of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecPinComponentReference actualParentPinComponentReference = actual.getParentPinComponentReference();
if (!Objects.areEqual(actualParentPinComponentReference, parentPinComponentReference)) {
failWithMessage(assertjErrorMessage, actual, parentPinComponentReference, actualParentPinComponentReference);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refContactPoint contains the given VecContactPoint elements.
* @param refContactPoint the given elements that should be contained in actual VecTerminalRole's refContactPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refContactPoint does not contain all given VecContactPoint elements.
*/
public S hasRefContactPoint(VecContactPoint... refContactPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint varargs is not null.
if (refContactPoint == null) failWithMessage("Expecting refContactPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefContactPoint(), refContactPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refContactPoint contains the given VecContactPoint elements in Collection.
* @param refContactPoint the given elements that should be contained in actual VecTerminalRole's refContactPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refContactPoint does not contain all given VecContactPoint elements.
*/
public S hasRefContactPoint(java.util.Collection extends VecContactPoint> refContactPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint collection is not null.
if (refContactPoint == null) {
failWithMessage("Expecting refContactPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefContactPoint(), refContactPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refContactPoint contains only the given VecContactPoint elements and nothing else in whatever order.
* @param refContactPoint the given elements that should be contained in actual VecTerminalRole's refContactPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refContactPoint does not contain all given VecContactPoint elements.
*/
public S hasOnlyRefContactPoint(VecContactPoint... refContactPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint varargs is not null.
if (refContactPoint == null) failWithMessage("Expecting refContactPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefContactPoint(), refContactPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refContactPoint contains only the given VecContactPoint elements in Collection and nothing else in whatever order.
* @param refContactPoint the given elements that should be contained in actual VecTerminalRole's refContactPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refContactPoint does not contain all given VecContactPoint elements.
*/
public S hasOnlyRefContactPoint(java.util.Collection extends VecContactPoint> refContactPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint collection is not null.
if (refContactPoint == null) {
failWithMessage("Expecting refContactPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefContactPoint(), refContactPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refContactPoint does not contain the given VecContactPoint elements.
*
* @param refContactPoint the given elements that should not be in actual VecTerminalRole's refContactPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refContactPoint contains any given VecContactPoint elements.
*/
public S doesNotHaveRefContactPoint(VecContactPoint... refContactPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint varargs is not null.
if (refContactPoint == null) failWithMessage("Expecting refContactPoint parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefContactPoint(), refContactPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refContactPoint does not contain the given VecContactPoint elements in Collection.
*
* @param refContactPoint the given elements that should not be in actual VecTerminalRole's refContactPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refContactPoint contains any given VecContactPoint elements.
*/
public S doesNotHaveRefContactPoint(java.util.Collection extends VecContactPoint> refContactPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecContactPoint collection is not null.
if (refContactPoint == null) {
failWithMessage("Expecting refContactPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefContactPoint(), refContactPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole has no refContactPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refContactPoint is not empty.
*/
public S hasNoRefContactPoint() {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refContactPoint but had :\n <%s>";
// check
if (actual.getRefContactPoint().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefContactPoint());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refMatingPoint contains the given VecMatingPoint elements.
* @param refMatingPoint the given elements that should be contained in actual VecTerminalRole's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasRefMatingPoint(VecMatingPoint... refMatingPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint varargs is not null.
if (refMatingPoint == null) failWithMessage("Expecting refMatingPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMatingPoint(), refMatingPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refMatingPoint contains the given VecMatingPoint elements in Collection.
* @param refMatingPoint the given elements that should be contained in actual VecTerminalRole's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasRefMatingPoint(java.util.Collection extends VecMatingPoint> refMatingPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint collection is not null.
if (refMatingPoint == null) {
failWithMessage("Expecting refMatingPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefMatingPoint(), refMatingPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refMatingPoint contains only the given VecMatingPoint elements and nothing else in whatever order.
* @param refMatingPoint the given elements that should be contained in actual VecTerminalRole's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasOnlyRefMatingPoint(VecMatingPoint... refMatingPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint varargs is not null.
if (refMatingPoint == null) failWithMessage("Expecting refMatingPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMatingPoint(), refMatingPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refMatingPoint contains only the given VecMatingPoint elements in Collection and nothing else in whatever order.
* @param refMatingPoint the given elements that should be contained in actual VecTerminalRole's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refMatingPoint does not contain all given VecMatingPoint elements.
*/
public S hasOnlyRefMatingPoint(java.util.Collection extends VecMatingPoint> refMatingPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint collection is not null.
if (refMatingPoint == null) {
failWithMessage("Expecting refMatingPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefMatingPoint(), refMatingPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refMatingPoint does not contain the given VecMatingPoint elements.
*
* @param refMatingPoint the given elements that should not be in actual VecTerminalRole's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refMatingPoint contains any given VecMatingPoint elements.
*/
public S doesNotHaveRefMatingPoint(VecMatingPoint... refMatingPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint varargs is not null.
if (refMatingPoint == null) failWithMessage("Expecting refMatingPoint parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMatingPoint(), refMatingPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's refMatingPoint does not contain the given VecMatingPoint elements in Collection.
*
* @param refMatingPoint the given elements that should not be in actual VecTerminalRole's refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refMatingPoint contains any given VecMatingPoint elements.
*/
public S doesNotHaveRefMatingPoint(java.util.Collection extends VecMatingPoint> refMatingPoint) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecMatingPoint collection is not null.
if (refMatingPoint == null) {
failWithMessage("Expecting refMatingPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefMatingPoint(), refMatingPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole has no refMatingPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's refMatingPoint is not empty.
*/
public S hasNoRefMatingPoint() {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refMatingPoint but had :\n <%s>";
// check
if (actual.getRefMatingPoint().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefMatingPoint());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's sealState is equal to the given one.
* @param sealState the given sealState to compare the actual VecTerminalRole's sealState to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalRole's sealState is not equal to the given one.
*/
public S hasSealState(String sealState) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting sealState of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSealState = actual.getSealState();
if (!Objects.areEqual(actualSealState, sealState)) {
failWithMessage(assertjErrorMessage, actual, sealState, actualSealState);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's terminalReceptionReferences contains the given VecTerminalReceptionReference elements.
* @param terminalReceptionReferences the given elements that should be contained in actual VecTerminalRole's terminalReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's terminalReceptionReferences does not contain all given VecTerminalReceptionReference elements.
*/
public S hasTerminalReceptionReferences(VecTerminalReceptionReference... terminalReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReceptionReference varargs is not null.
if (terminalReceptionReferences == null) failWithMessage("Expecting terminalReceptionReferences parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTerminalReceptionReferences(), terminalReceptionReferences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's terminalReceptionReferences contains the given VecTerminalReceptionReference elements in Collection.
* @param terminalReceptionReferences the given elements that should be contained in actual VecTerminalRole's terminalReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's terminalReceptionReferences does not contain all given VecTerminalReceptionReference elements.
*/
public S hasTerminalReceptionReferences(java.util.Collection extends VecTerminalReceptionReference> terminalReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReceptionReference collection is not null.
if (terminalReceptionReferences == null) {
failWithMessage("Expecting terminalReceptionReferences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTerminalReceptionReferences(), terminalReceptionReferences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's terminalReceptionReferences contains only the given VecTerminalReceptionReference elements and nothing else in whatever order.
* @param terminalReceptionReferences the given elements that should be contained in actual VecTerminalRole's terminalReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's terminalReceptionReferences does not contain all given VecTerminalReceptionReference elements.
*/
public S hasOnlyTerminalReceptionReferences(VecTerminalReceptionReference... terminalReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReceptionReference varargs is not null.
if (terminalReceptionReferences == null) failWithMessage("Expecting terminalReceptionReferences parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTerminalReceptionReferences(), terminalReceptionReferences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's terminalReceptionReferences contains only the given VecTerminalReceptionReference elements in Collection and nothing else in whatever order.
* @param terminalReceptionReferences the given elements that should be contained in actual VecTerminalRole's terminalReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's terminalReceptionReferences does not contain all given VecTerminalReceptionReference elements.
*/
public S hasOnlyTerminalReceptionReferences(java.util.Collection extends VecTerminalReceptionReference> terminalReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReceptionReference collection is not null.
if (terminalReceptionReferences == null) {
failWithMessage("Expecting terminalReceptionReferences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTerminalReceptionReferences(), terminalReceptionReferences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's terminalReceptionReferences does not contain the given VecTerminalReceptionReference elements.
*
* @param terminalReceptionReferences the given elements that should not be in actual VecTerminalRole's terminalReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's terminalReceptionReferences contains any given VecTerminalReceptionReference elements.
*/
public S doesNotHaveTerminalReceptionReferences(VecTerminalReceptionReference... terminalReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReceptionReference varargs is not null.
if (terminalReceptionReferences == null) failWithMessage("Expecting terminalReceptionReferences parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTerminalReceptionReferences(), terminalReceptionReferences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's terminalReceptionReferences does not contain the given VecTerminalReceptionReference elements in Collection.
*
* @param terminalReceptionReferences the given elements that should not be in actual VecTerminalRole's terminalReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's terminalReceptionReferences contains any given VecTerminalReceptionReference elements.
*/
public S doesNotHaveTerminalReceptionReferences(java.util.Collection extends VecTerminalReceptionReference> terminalReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReceptionReference collection is not null.
if (terminalReceptionReferences == null) {
failWithMessage("Expecting terminalReceptionReferences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTerminalReceptionReferences(), terminalReceptionReferences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole has no terminalReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's terminalReceptionReferences is not empty.
*/
public S hasNoTerminalReceptionReferences() {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have terminalReceptionReferences but had :\n <%s>";
// check
if (actual.getTerminalReceptionReferences().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getTerminalReceptionReferences());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's terminalSpecification is equal to the given one.
* @param terminalSpecification the given terminalSpecification to compare the actual VecTerminalRole's terminalSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalRole's terminalSpecification is not equal to the given one.
*/
public S hasTerminalSpecification(VecTerminalSpecification terminalSpecification) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting terminalSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTerminalSpecification actualTerminalSpecification = actual.getTerminalSpecification();
if (!Objects.areEqual(actualTerminalSpecification, terminalSpecification)) {
failWithMessage(assertjErrorMessage, actual, terminalSpecification, actualTerminalSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's wireReceptionReferences contains the given VecWireReceptionReference elements.
* @param wireReceptionReferences the given elements that should be contained in actual VecTerminalRole's wireReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's wireReceptionReferences does not contain all given VecWireReceptionReference elements.
*/
public S hasWireReceptionReferences(VecWireReceptionReference... wireReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionReference varargs is not null.
if (wireReceptionReferences == null) failWithMessage("Expecting wireReceptionReferences parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireReceptionReferences(), wireReceptionReferences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's wireReceptionReferences contains the given VecWireReceptionReference elements in Collection.
* @param wireReceptionReferences the given elements that should be contained in actual VecTerminalRole's wireReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's wireReceptionReferences does not contain all given VecWireReceptionReference elements.
*/
public S hasWireReceptionReferences(java.util.Collection extends VecWireReceptionReference> wireReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionReference collection is not null.
if (wireReceptionReferences == null) {
failWithMessage("Expecting wireReceptionReferences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireReceptionReferences(), wireReceptionReferences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's wireReceptionReferences contains only the given VecWireReceptionReference elements and nothing else in whatever order.
* @param wireReceptionReferences the given elements that should be contained in actual VecTerminalRole's wireReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's wireReceptionReferences does not contain all given VecWireReceptionReference elements.
*/
public S hasOnlyWireReceptionReferences(VecWireReceptionReference... wireReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionReference varargs is not null.
if (wireReceptionReferences == null) failWithMessage("Expecting wireReceptionReferences parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireReceptionReferences(), wireReceptionReferences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's wireReceptionReferences contains only the given VecWireReceptionReference elements in Collection and nothing else in whatever order.
* @param wireReceptionReferences the given elements that should be contained in actual VecTerminalRole's wireReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's wireReceptionReferences does not contain all given VecWireReceptionReference elements.
*/
public S hasOnlyWireReceptionReferences(java.util.Collection extends VecWireReceptionReference> wireReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionReference collection is not null.
if (wireReceptionReferences == null) {
failWithMessage("Expecting wireReceptionReferences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireReceptionReferences(), wireReceptionReferences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's wireReceptionReferences does not contain the given VecWireReceptionReference elements.
*
* @param wireReceptionReferences the given elements that should not be in actual VecTerminalRole's wireReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's wireReceptionReferences contains any given VecWireReceptionReference elements.
*/
public S doesNotHaveWireReceptionReferences(VecWireReceptionReference... wireReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionReference varargs is not null.
if (wireReceptionReferences == null) failWithMessage("Expecting wireReceptionReferences parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireReceptionReferences(), wireReceptionReferences);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole's wireReceptionReferences does not contain the given VecWireReceptionReference elements in Collection.
*
* @param wireReceptionReferences the given elements that should not be in actual VecTerminalRole's wireReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's wireReceptionReferences contains any given VecWireReceptionReference elements.
*/
public S doesNotHaveWireReceptionReferences(java.util.Collection extends VecWireReceptionReference> wireReceptionReferences) {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionReference collection is not null.
if (wireReceptionReferences == null) {
failWithMessage("Expecting wireReceptionReferences parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireReceptionReferences(), wireReceptionReferences.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalRole has no wireReceptionReferences.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalRole's wireReceptionReferences is not empty.
*/
public S hasNoWireReceptionReferences() {
// check that actual VecTerminalRole we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have wireReceptionReferences but had :\n <%s>";
// check
if (actual.getWireReceptionReferences().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getWireReceptionReferences());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy