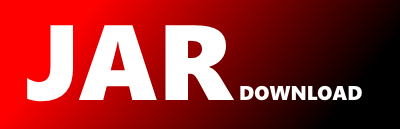
com.foursoft.vecmodel.vec120.AbstractVecTerminalSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTerminalSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTerminalSpecificationAssert, A extends VecTerminalSpecification> extends AbstractVecPartOrUsageRelatedSpecificationAssert {
/**
* Creates a new {@link AbstractVecTerminalSpecificationAssert}
to make assertions on actual VecTerminalSpecification.
* @param actual the VecTerminalSpecification we want to make assertions on.
*/
protected AbstractVecTerminalSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTerminalSpecification's currentInformations contains the given VecTerminalCurrentInformation elements.
* @param currentInformations the given elements that should be contained in actual VecTerminalSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's currentInformations does not contain all given VecTerminalCurrentInformation elements.
*/
public S hasCurrentInformations(VecTerminalCurrentInformation... currentInformations) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalCurrentInformation varargs is not null.
if (currentInformations == null) failWithMessage("Expecting currentInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCurrentInformations(), currentInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's currentInformations contains the given VecTerminalCurrentInformation elements in Collection.
* @param currentInformations the given elements that should be contained in actual VecTerminalSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's currentInformations does not contain all given VecTerminalCurrentInformation elements.
*/
public S hasCurrentInformations(java.util.Collection extends VecTerminalCurrentInformation> currentInformations) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalCurrentInformation collection is not null.
if (currentInformations == null) {
failWithMessage("Expecting currentInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCurrentInformations(), currentInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's currentInformations contains only the given VecTerminalCurrentInformation elements and nothing else in whatever order.
* @param currentInformations the given elements that should be contained in actual VecTerminalSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's currentInformations does not contain all given VecTerminalCurrentInformation elements.
*/
public S hasOnlyCurrentInformations(VecTerminalCurrentInformation... currentInformations) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalCurrentInformation varargs is not null.
if (currentInformations == null) failWithMessage("Expecting currentInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCurrentInformations(), currentInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's currentInformations contains only the given VecTerminalCurrentInformation elements in Collection and nothing else in whatever order.
* @param currentInformations the given elements that should be contained in actual VecTerminalSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's currentInformations does not contain all given VecTerminalCurrentInformation elements.
*/
public S hasOnlyCurrentInformations(java.util.Collection extends VecTerminalCurrentInformation> currentInformations) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalCurrentInformation collection is not null.
if (currentInformations == null) {
failWithMessage("Expecting currentInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCurrentInformations(), currentInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's currentInformations does not contain the given VecTerminalCurrentInformation elements.
*
* @param currentInformations the given elements that should not be in actual VecTerminalSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's currentInformations contains any given VecTerminalCurrentInformation elements.
*/
public S doesNotHaveCurrentInformations(VecTerminalCurrentInformation... currentInformations) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalCurrentInformation varargs is not null.
if (currentInformations == null) failWithMessage("Expecting currentInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCurrentInformations(), currentInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's currentInformations does not contain the given VecTerminalCurrentInformation elements in Collection.
*
* @param currentInformations the given elements that should not be in actual VecTerminalSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's currentInformations contains any given VecTerminalCurrentInformation elements.
*/
public S doesNotHaveCurrentInformations(java.util.Collection extends VecTerminalCurrentInformation> currentInformations) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalCurrentInformation collection is not null.
if (currentInformations == null) {
failWithMessage("Expecting currentInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCurrentInformations(), currentInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification has no currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's currentInformations is not empty.
*/
public S hasNoCurrentInformations() {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have currentInformations but had :\n <%s>";
// check
if (actual.getCurrentInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getCurrentInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's internalTerminalConnections contains the given VecInternalTerminalConnection elements.
* @param internalTerminalConnections the given elements that should be contained in actual VecTerminalSpecification's internalTerminalConnections.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's internalTerminalConnections does not contain all given VecInternalTerminalConnection elements.
*/
public S hasInternalTerminalConnections(VecInternalTerminalConnection... internalTerminalConnections) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalTerminalConnection varargs is not null.
if (internalTerminalConnections == null) failWithMessage("Expecting internalTerminalConnections parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInternalTerminalConnections(), internalTerminalConnections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's internalTerminalConnections contains the given VecInternalTerminalConnection elements in Collection.
* @param internalTerminalConnections the given elements that should be contained in actual VecTerminalSpecification's internalTerminalConnections.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's internalTerminalConnections does not contain all given VecInternalTerminalConnection elements.
*/
public S hasInternalTerminalConnections(java.util.Collection extends VecInternalTerminalConnection> internalTerminalConnections) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalTerminalConnection collection is not null.
if (internalTerminalConnections == null) {
failWithMessage("Expecting internalTerminalConnections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getInternalTerminalConnections(), internalTerminalConnections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's internalTerminalConnections contains only the given VecInternalTerminalConnection elements and nothing else in whatever order.
* @param internalTerminalConnections the given elements that should be contained in actual VecTerminalSpecification's internalTerminalConnections.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's internalTerminalConnections does not contain all given VecInternalTerminalConnection elements.
*/
public S hasOnlyInternalTerminalConnections(VecInternalTerminalConnection... internalTerminalConnections) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalTerminalConnection varargs is not null.
if (internalTerminalConnections == null) failWithMessage("Expecting internalTerminalConnections parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInternalTerminalConnections(), internalTerminalConnections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's internalTerminalConnections contains only the given VecInternalTerminalConnection elements in Collection and nothing else in whatever order.
* @param internalTerminalConnections the given elements that should be contained in actual VecTerminalSpecification's internalTerminalConnections.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's internalTerminalConnections does not contain all given VecInternalTerminalConnection elements.
*/
public S hasOnlyInternalTerminalConnections(java.util.Collection extends VecInternalTerminalConnection> internalTerminalConnections) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalTerminalConnection collection is not null.
if (internalTerminalConnections == null) {
failWithMessage("Expecting internalTerminalConnections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getInternalTerminalConnections(), internalTerminalConnections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's internalTerminalConnections does not contain the given VecInternalTerminalConnection elements.
*
* @param internalTerminalConnections the given elements that should not be in actual VecTerminalSpecification's internalTerminalConnections.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's internalTerminalConnections contains any given VecInternalTerminalConnection elements.
*/
public S doesNotHaveInternalTerminalConnections(VecInternalTerminalConnection... internalTerminalConnections) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalTerminalConnection varargs is not null.
if (internalTerminalConnections == null) failWithMessage("Expecting internalTerminalConnections parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInternalTerminalConnections(), internalTerminalConnections);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's internalTerminalConnections does not contain the given VecInternalTerminalConnection elements in Collection.
*
* @param internalTerminalConnections the given elements that should not be in actual VecTerminalSpecification's internalTerminalConnections.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's internalTerminalConnections contains any given VecInternalTerminalConnection elements.
*/
public S doesNotHaveInternalTerminalConnections(java.util.Collection extends VecInternalTerminalConnection> internalTerminalConnections) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalTerminalConnection collection is not null.
if (internalTerminalConnections == null) {
failWithMessage("Expecting internalTerminalConnections parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getInternalTerminalConnections(), internalTerminalConnections.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification has no internalTerminalConnections.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's internalTerminalConnections is not empty.
*/
public S hasNoInternalTerminalConnections() {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have internalTerminalConnections but had :\n <%s>";
// check
if (actual.getInternalTerminalConnections().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getInternalTerminalConnections());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refCavity contains the given VecCavity elements.
* @param refCavity the given elements that should be contained in actual VecTerminalSpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasRefCavity(VecCavity... refCavity) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity varargs is not null.
if (refCavity == null) failWithMessage("Expecting refCavity parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavity(), refCavity);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refCavity contains the given VecCavity elements in Collection.
* @param refCavity the given elements that should be contained in actual VecTerminalSpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasRefCavity(java.util.Collection extends VecCavity> refCavity) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity collection is not null.
if (refCavity == null) {
failWithMessage("Expecting refCavity parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavity(), refCavity.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refCavity contains only the given VecCavity elements and nothing else in whatever order.
* @param refCavity the given elements that should be contained in actual VecTerminalSpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasOnlyRefCavity(VecCavity... refCavity) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity varargs is not null.
if (refCavity == null) failWithMessage("Expecting refCavity parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavity(), refCavity);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refCavity contains only the given VecCavity elements in Collection and nothing else in whatever order.
* @param refCavity the given elements that should be contained in actual VecTerminalSpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasOnlyRefCavity(java.util.Collection extends VecCavity> refCavity) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity collection is not null.
if (refCavity == null) {
failWithMessage("Expecting refCavity parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavity(), refCavity.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refCavity does not contain the given VecCavity elements.
*
* @param refCavity the given elements that should not be in actual VecTerminalSpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refCavity contains any given VecCavity elements.
*/
public S doesNotHaveRefCavity(VecCavity... refCavity) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity varargs is not null.
if (refCavity == null) failWithMessage("Expecting refCavity parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavity(), refCavity);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refCavity does not contain the given VecCavity elements in Collection.
*
* @param refCavity the given elements that should not be in actual VecTerminalSpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refCavity contains any given VecCavity elements.
*/
public S doesNotHaveRefCavity(java.util.Collection extends VecCavity> refCavity) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity collection is not null.
if (refCavity == null) {
failWithMessage("Expecting refCavity parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavity(), refCavity.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification has no refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refCavity is not empty.
*/
public S hasNoRefCavity() {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavity but had :\n <%s>";
// check
if (actual.getRefCavity().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavity());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refPinComponent contains the given VecPinComponent elements.
* @param refPinComponent the given elements that should be contained in actual VecTerminalSpecification's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasRefPinComponent(VecPinComponent... refPinComponent) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent varargs is not null.
if (refPinComponent == null) failWithMessage("Expecting refPinComponent parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponent(), refPinComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refPinComponent contains the given VecPinComponent elements in Collection.
* @param refPinComponent the given elements that should be contained in actual VecTerminalSpecification's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasRefPinComponent(java.util.Collection extends VecPinComponent> refPinComponent) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent collection is not null.
if (refPinComponent == null) {
failWithMessage("Expecting refPinComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponent(), refPinComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refPinComponent contains only the given VecPinComponent elements and nothing else in whatever order.
* @param refPinComponent the given elements that should be contained in actual VecTerminalSpecification's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasOnlyRefPinComponent(VecPinComponent... refPinComponent) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent varargs is not null.
if (refPinComponent == null) failWithMessage("Expecting refPinComponent parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponent(), refPinComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refPinComponent contains only the given VecPinComponent elements in Collection and nothing else in whatever order.
* @param refPinComponent the given elements that should be contained in actual VecTerminalSpecification's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasOnlyRefPinComponent(java.util.Collection extends VecPinComponent> refPinComponent) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent collection is not null.
if (refPinComponent == null) {
failWithMessage("Expecting refPinComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponent(), refPinComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refPinComponent does not contain the given VecPinComponent elements.
*
* @param refPinComponent the given elements that should not be in actual VecTerminalSpecification's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refPinComponent contains any given VecPinComponent elements.
*/
public S doesNotHaveRefPinComponent(VecPinComponent... refPinComponent) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent varargs is not null.
if (refPinComponent == null) failWithMessage("Expecting refPinComponent parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponent(), refPinComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refPinComponent does not contain the given VecPinComponent elements in Collection.
*
* @param refPinComponent the given elements that should not be in actual VecTerminalSpecification's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refPinComponent contains any given VecPinComponent elements.
*/
public S doesNotHaveRefPinComponent(java.util.Collection extends VecPinComponent> refPinComponent) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent collection is not null.
if (refPinComponent == null) {
failWithMessage("Expecting refPinComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponent(), refPinComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification has no refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refPinComponent is not empty.
*/
public S hasNoRefPinComponent() {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPinComponent but had :\n <%s>";
// check
if (actual.getRefPinComponent().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPinComponent());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refTerminalRole contains the given VecTerminalRole elements.
* @param refTerminalRole the given elements that should be contained in actual VecTerminalSpecification's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasRefTerminalRole(VecTerminalRole... refTerminalRole) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole varargs is not null.
if (refTerminalRole == null) failWithMessage("Expecting refTerminalRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalRole(), refTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refTerminalRole contains the given VecTerminalRole elements in Collection.
* @param refTerminalRole the given elements that should be contained in actual VecTerminalSpecification's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasRefTerminalRole(java.util.Collection extends VecTerminalRole> refTerminalRole) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole collection is not null.
if (refTerminalRole == null) {
failWithMessage("Expecting refTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalRole(), refTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refTerminalRole contains only the given VecTerminalRole elements and nothing else in whatever order.
* @param refTerminalRole the given elements that should be contained in actual VecTerminalSpecification's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasOnlyRefTerminalRole(VecTerminalRole... refTerminalRole) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole varargs is not null.
if (refTerminalRole == null) failWithMessage("Expecting refTerminalRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalRole(), refTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refTerminalRole contains only the given VecTerminalRole elements in Collection and nothing else in whatever order.
* @param refTerminalRole the given elements that should be contained in actual VecTerminalSpecification's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasOnlyRefTerminalRole(java.util.Collection extends VecTerminalRole> refTerminalRole) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole collection is not null.
if (refTerminalRole == null) {
failWithMessage("Expecting refTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalRole(), refTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refTerminalRole does not contain the given VecTerminalRole elements.
*
* @param refTerminalRole the given elements that should not be in actual VecTerminalSpecification's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refTerminalRole contains any given VecTerminalRole elements.
*/
public S doesNotHaveRefTerminalRole(VecTerminalRole... refTerminalRole) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole varargs is not null.
if (refTerminalRole == null) failWithMessage("Expecting refTerminalRole parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalRole(), refTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's refTerminalRole does not contain the given VecTerminalRole elements in Collection.
*
* @param refTerminalRole the given elements that should not be in actual VecTerminalSpecification's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refTerminalRole contains any given VecTerminalRole elements.
*/
public S doesNotHaveRefTerminalRole(java.util.Collection extends VecTerminalRole> refTerminalRole) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole collection is not null.
if (refTerminalRole == null) {
failWithMessage("Expecting refTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalRole(), refTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification has no refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's refTerminalRole is not empty.
*/
public S hasNoRefTerminalRole() {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTerminalRole but had :\n <%s>";
// check
if (actual.getRefTerminalRole().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTerminalRole());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's sealingType is equal to the given one.
* @param sealingType the given sealingType to compare the actual VecTerminalSpecification's sealingType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalSpecification's sealingType is not equal to the given one.
*/
public S hasSealingType(String sealingType) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting sealingType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSealingType = actual.getSealingType();
if (!Objects.areEqual(actualSealingType, sealingType)) {
failWithMessage(assertjErrorMessage, actual, sealingType, actualSealingType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's terminalReceptions contains the given VecTerminalReception elements.
* @param terminalReceptions the given elements that should be contained in actual VecTerminalSpecification's terminalReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's terminalReceptions does not contain all given VecTerminalReception elements.
*/
public S hasTerminalReceptions(VecTerminalReception... terminalReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception varargs is not null.
if (terminalReceptions == null) failWithMessage("Expecting terminalReceptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTerminalReceptions(), terminalReceptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's terminalReceptions contains the given VecTerminalReception elements in Collection.
* @param terminalReceptions the given elements that should be contained in actual VecTerminalSpecification's terminalReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's terminalReceptions does not contain all given VecTerminalReception elements.
*/
public S hasTerminalReceptions(java.util.Collection extends VecTerminalReception> terminalReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception collection is not null.
if (terminalReceptions == null) {
failWithMessage("Expecting terminalReceptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTerminalReceptions(), terminalReceptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's terminalReceptions contains only the given VecTerminalReception elements and nothing else in whatever order.
* @param terminalReceptions the given elements that should be contained in actual VecTerminalSpecification's terminalReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's terminalReceptions does not contain all given VecTerminalReception elements.
*/
public S hasOnlyTerminalReceptions(VecTerminalReception... terminalReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception varargs is not null.
if (terminalReceptions == null) failWithMessage("Expecting terminalReceptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTerminalReceptions(), terminalReceptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's terminalReceptions contains only the given VecTerminalReception elements in Collection and nothing else in whatever order.
* @param terminalReceptions the given elements that should be contained in actual VecTerminalSpecification's terminalReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's terminalReceptions does not contain all given VecTerminalReception elements.
*/
public S hasOnlyTerminalReceptions(java.util.Collection extends VecTerminalReception> terminalReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception collection is not null.
if (terminalReceptions == null) {
failWithMessage("Expecting terminalReceptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTerminalReceptions(), terminalReceptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's terminalReceptions does not contain the given VecTerminalReception elements.
*
* @param terminalReceptions the given elements that should not be in actual VecTerminalSpecification's terminalReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's terminalReceptions contains any given VecTerminalReception elements.
*/
public S doesNotHaveTerminalReceptions(VecTerminalReception... terminalReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception varargs is not null.
if (terminalReceptions == null) failWithMessage("Expecting terminalReceptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTerminalReceptions(), terminalReceptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's terminalReceptions does not contain the given VecTerminalReception elements in Collection.
*
* @param terminalReceptions the given elements that should not be in actual VecTerminalSpecification's terminalReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's terminalReceptions contains any given VecTerminalReception elements.
*/
public S doesNotHaveTerminalReceptions(java.util.Collection extends VecTerminalReception> terminalReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalReception collection is not null.
if (terminalReceptions == null) {
failWithMessage("Expecting terminalReceptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTerminalReceptions(), terminalReceptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification has no terminalReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's terminalReceptions is not empty.
*/
public S hasNoTerminalReceptions() {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have terminalReceptions but had :\n <%s>";
// check
if (actual.getTerminalReceptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getTerminalReceptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's voltageRange is equal to the given one.
* @param voltageRange the given voltageRange to compare the actual VecTerminalSpecification's voltageRange to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTerminalSpecification's voltageRange is not equal to the given one.
*/
public S hasVoltageRange(VecValueRange voltageRange) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting voltageRange of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecValueRange actualVoltageRange = actual.getVoltageRange();
if (!Objects.areEqual(actualVoltageRange, voltageRange)) {
failWithMessage(assertjErrorMessage, actual, voltageRange, actualVoltageRange);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's wireReceptions contains the given VecWireReception elements.
* @param wireReceptions the given elements that should be contained in actual VecTerminalSpecification's wireReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's wireReceptions does not contain all given VecWireReception elements.
*/
public S hasWireReceptions(VecWireReception... wireReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (wireReceptions == null) failWithMessage("Expecting wireReceptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireReceptions(), wireReceptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's wireReceptions contains the given VecWireReception elements in Collection.
* @param wireReceptions the given elements that should be contained in actual VecTerminalSpecification's wireReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's wireReceptions does not contain all given VecWireReception elements.
*/
public S hasWireReceptions(java.util.Collection extends VecWireReception> wireReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (wireReceptions == null) {
failWithMessage("Expecting wireReceptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireReceptions(), wireReceptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's wireReceptions contains only the given VecWireReception elements and nothing else in whatever order.
* @param wireReceptions the given elements that should be contained in actual VecTerminalSpecification's wireReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's wireReceptions does not contain all given VecWireReception elements.
*/
public S hasOnlyWireReceptions(VecWireReception... wireReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (wireReceptions == null) failWithMessage("Expecting wireReceptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireReceptions(), wireReceptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's wireReceptions contains only the given VecWireReception elements in Collection and nothing else in whatever order.
* @param wireReceptions the given elements that should be contained in actual VecTerminalSpecification's wireReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's wireReceptions does not contain all given VecWireReception elements.
*/
public S hasOnlyWireReceptions(java.util.Collection extends VecWireReception> wireReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (wireReceptions == null) {
failWithMessage("Expecting wireReceptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireReceptions(), wireReceptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's wireReceptions does not contain the given VecWireReception elements.
*
* @param wireReceptions the given elements that should not be in actual VecTerminalSpecification's wireReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's wireReceptions contains any given VecWireReception elements.
*/
public S doesNotHaveWireReceptions(VecWireReception... wireReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (wireReceptions == null) failWithMessage("Expecting wireReceptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireReceptions(), wireReceptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification's wireReceptions does not contain the given VecWireReception elements in Collection.
*
* @param wireReceptions the given elements that should not be in actual VecTerminalSpecification's wireReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's wireReceptions contains any given VecWireReception elements.
*/
public S doesNotHaveWireReceptions(java.util.Collection extends VecWireReception> wireReceptions) {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (wireReceptions == null) {
failWithMessage("Expecting wireReceptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireReceptions(), wireReceptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTerminalSpecification has no wireReceptions.
* @return this assertion object.
* @throws AssertionError if the actual VecTerminalSpecification's wireReceptions is not empty.
*/
public S hasNoWireReceptions() {
// check that actual VecTerminalSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have wireReceptions but had :\n <%s>";
// check
if (actual.getWireReceptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getWireReceptions());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy