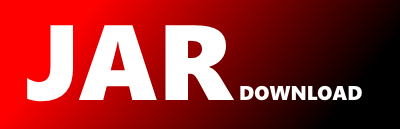
com.foursoft.vecmodel.vec120.AbstractVecTopologyNodeAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTopologyNode} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTopologyNodeAssert, A extends VecTopologyNode> extends AbstractVecConfigurableElementAssert {
/**
* Creates a new {@link AbstractVecTopologyNodeAssert}
to make assertions on actual VecTopologyNode.
* @param actual the VecTopologyNode we want to make assertions on.
*/
protected AbstractVecTopologyNodeAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTopologyNode's aliasIds contains the given VecAliasIdentification elements.
* @param aliasIds the given elements that should be contained in actual VecTopologyNode's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's aliasIds contains the given VecAliasIdentification elements in Collection.
* @param aliasIds the given elements that should be contained in actual VecTopologyNode's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's aliasIds contains only the given VecAliasIdentification elements and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecTopologyNode's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's aliasIds contains only the given VecAliasIdentification elements in Collection and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecTopologyNode's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's aliasIds does not contain the given VecAliasIdentification elements.
*
* @param aliasIds the given elements that should not be in actual VecTopologyNode's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's aliasIds does not contain the given VecAliasIdentification elements in Collection.
*
* @param aliasIds the given elements that should not be in actual VecTopologyNode's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode has no aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's aliasIds is not empty.
*/
public S hasNoAliasIds() {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have aliasIds but had :\n <%s>";
// check
if (actual.getAliasIds().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAliasIds());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's identification is equal to the given one.
* @param identification the given identification to compare the actual VecTopologyNode's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologyNode's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's instantiatedNode is equal to the given one.
* @param instantiatedNode the given instantiatedNode to compare the actual VecTopologyNode's instantiatedNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologyNode's instantiatedNode is not equal to the given one.
*/
public S hasInstantiatedNode(VecTopologyNode instantiatedNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting instantiatedNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTopologyNode actualInstantiatedNode = actual.getInstantiatedNode();
if (!Objects.areEqual(actualInstantiatedNode, instantiatedNode)) {
failWithMessage(assertjErrorMessage, actual, instantiatedNode, actualInstantiatedNode);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's matchingPointId is equal to the given one.
* @param matchingPointId the given matchingPointId to compare the actual VecTopologyNode's matchingPointId to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologyNode's matchingPointId is not equal to the given one.
*/
public S hasMatchingPointId(String matchingPointId) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting matchingPointId of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualMatchingPointId = actual.getMatchingPointId();
if (!Objects.areEqual(actualMatchingPointId, matchingPointId)) {
failWithMessage(assertjErrorMessage, actual, matchingPointId, actualMatchingPointId);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's nodeType is equal to the given one.
* @param nodeType the given nodeType to compare the actual VecTopologyNode's nodeType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologyNode's nodeType is not equal to the given one.
*/
public S hasNodeType(String nodeType) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting nodeType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualNodeType = actual.getNodeType();
if (!Objects.areEqual(actualNodeType, nodeType)) {
failWithMessage(assertjErrorMessage, actual, nodeType, actualNodeType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's parentTopologySpecification is equal to the given one.
* @param parentTopologySpecification the given parentTopologySpecification to compare the actual VecTopologyNode's parentTopologySpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologyNode's parentTopologySpecification is not equal to the given one.
*/
public S hasParentTopologySpecification(VecTopologySpecification parentTopologySpecification) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentTopologySpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTopologySpecification actualParentTopologySpecification = actual.getParentTopologySpecification();
if (!Objects.areEqual(actualParentTopologySpecification, parentTopologySpecification)) {
failWithMessage(assertjErrorMessage, actual, parentTopologySpecification, actualParentTopologySpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's processingInstructions contains the given VecLocalizedString elements.
* @param processingInstructions the given elements that should be contained in actual VecTopologyNode's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasProcessingInstructions(VecLocalizedString... processingInstructions) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's processingInstructions contains the given VecLocalizedString elements in Collection.
* @param processingInstructions the given elements that should be contained in actual VecTopologyNode's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasProcessingInstructions(java.util.Collection extends VecLocalizedString> processingInstructions) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's processingInstructions contains only the given VecLocalizedString elements and nothing else in whatever order.
* @param processingInstructions the given elements that should be contained in actual VecTopologyNode's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasOnlyProcessingInstructions(VecLocalizedString... processingInstructions) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's processingInstructions contains only the given VecLocalizedString elements in Collection and nothing else in whatever order.
* @param processingInstructions the given elements that should be contained in actual VecTopologyNode's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasOnlyProcessingInstructions(java.util.Collection extends VecLocalizedString> processingInstructions) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's processingInstructions does not contain the given VecLocalizedString elements.
*
* @param processingInstructions the given elements that should not be in actual VecTopologyNode's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's processingInstructions contains any given VecLocalizedString elements.
*/
public S doesNotHaveProcessingInstructions(VecLocalizedString... processingInstructions) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's processingInstructions does not contain the given VecLocalizedString elements in Collection.
*
* @param processingInstructions the given elements that should not be in actual VecTopologyNode's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's processingInstructions contains any given VecLocalizedString elements.
*/
public S doesNotHaveProcessingInstructions(java.util.Collection extends VecLocalizedString> processingInstructions) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode has no processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's processingInstructions is not empty.
*/
public S hasNoProcessingInstructions() {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have processingInstructions but had :\n <%s>";
// check
if (actual.getProcessingInstructions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getProcessingInstructions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's realizedUsageNode is equal to the given one.
* @param realizedUsageNode the given realizedUsageNode to compare the actual VecTopologyNode's realizedUsageNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologyNode's realizedUsageNode is not equal to the given one.
*/
public S hasRealizedUsageNode(VecUsageNode realizedUsageNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting realizedUsageNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecUsageNode actualRealizedUsageNode = actual.getRealizedUsageNode();
if (!Objects.areEqual(actualRealizedUsageNode, realizedUsageNode)) {
failWithMessage(assertjErrorMessage, actual, realizedUsageNode, actualRealizedUsageNode);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refGeometryNode contains the given VecGeometryNode elements.
* @param refGeometryNode the given elements that should be contained in actual VecTopologyNode's refGeometryNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refGeometryNode does not contain all given VecGeometryNode elements.
*/
public S hasRefGeometryNode(VecGeometryNode... refGeometryNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode varargs is not null.
if (refGeometryNode == null) failWithMessage("Expecting refGeometryNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefGeometryNode(), refGeometryNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refGeometryNode contains the given VecGeometryNode elements in Collection.
* @param refGeometryNode the given elements that should be contained in actual VecTopologyNode's refGeometryNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refGeometryNode does not contain all given VecGeometryNode elements.
*/
public S hasRefGeometryNode(java.util.Collection extends VecGeometryNode> refGeometryNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode collection is not null.
if (refGeometryNode == null) {
failWithMessage("Expecting refGeometryNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefGeometryNode(), refGeometryNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refGeometryNode contains only the given VecGeometryNode elements and nothing else in whatever order.
* @param refGeometryNode the given elements that should be contained in actual VecTopologyNode's refGeometryNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refGeometryNode does not contain all given VecGeometryNode elements.
*/
public S hasOnlyRefGeometryNode(VecGeometryNode... refGeometryNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode varargs is not null.
if (refGeometryNode == null) failWithMessage("Expecting refGeometryNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefGeometryNode(), refGeometryNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refGeometryNode contains only the given VecGeometryNode elements in Collection and nothing else in whatever order.
* @param refGeometryNode the given elements that should be contained in actual VecTopologyNode's refGeometryNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refGeometryNode does not contain all given VecGeometryNode elements.
*/
public S hasOnlyRefGeometryNode(java.util.Collection extends VecGeometryNode> refGeometryNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode collection is not null.
if (refGeometryNode == null) {
failWithMessage("Expecting refGeometryNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefGeometryNode(), refGeometryNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refGeometryNode does not contain the given VecGeometryNode elements.
*
* @param refGeometryNode the given elements that should not be in actual VecTopologyNode's refGeometryNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refGeometryNode contains any given VecGeometryNode elements.
*/
public S doesNotHaveRefGeometryNode(VecGeometryNode... refGeometryNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode varargs is not null.
if (refGeometryNode == null) failWithMessage("Expecting refGeometryNode parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefGeometryNode(), refGeometryNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refGeometryNode does not contain the given VecGeometryNode elements in Collection.
*
* @param refGeometryNode the given elements that should not be in actual VecTopologyNode's refGeometryNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refGeometryNode contains any given VecGeometryNode elements.
*/
public S doesNotHaveRefGeometryNode(java.util.Collection extends VecGeometryNode> refGeometryNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode collection is not null.
if (refGeometryNode == null) {
failWithMessage("Expecting refGeometryNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefGeometryNode(), refGeometryNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode has no refGeometryNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refGeometryNode is not empty.
*/
public S hasNoRefGeometryNode() {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refGeometryNode but had :\n <%s>";
// check
if (actual.getRefGeometryNode().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefGeometryNode());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeLocation contains the given VecNodeLocation elements.
* @param refNodeLocation the given elements that should be contained in actual VecTopologyNode's refNodeLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeLocation does not contain all given VecNodeLocation elements.
*/
public S hasRefNodeLocation(VecNodeLocation... refNodeLocation) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeLocation varargs is not null.
if (refNodeLocation == null) failWithMessage("Expecting refNodeLocation parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefNodeLocation(), refNodeLocation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeLocation contains the given VecNodeLocation elements in Collection.
* @param refNodeLocation the given elements that should be contained in actual VecTopologyNode's refNodeLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeLocation does not contain all given VecNodeLocation elements.
*/
public S hasRefNodeLocation(java.util.Collection extends VecNodeLocation> refNodeLocation) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeLocation collection is not null.
if (refNodeLocation == null) {
failWithMessage("Expecting refNodeLocation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefNodeLocation(), refNodeLocation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeLocation contains only the given VecNodeLocation elements and nothing else in whatever order.
* @param refNodeLocation the given elements that should be contained in actual VecTopologyNode's refNodeLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeLocation does not contain all given VecNodeLocation elements.
*/
public S hasOnlyRefNodeLocation(VecNodeLocation... refNodeLocation) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeLocation varargs is not null.
if (refNodeLocation == null) failWithMessage("Expecting refNodeLocation parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefNodeLocation(), refNodeLocation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeLocation contains only the given VecNodeLocation elements in Collection and nothing else in whatever order.
* @param refNodeLocation the given elements that should be contained in actual VecTopologyNode's refNodeLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeLocation does not contain all given VecNodeLocation elements.
*/
public S hasOnlyRefNodeLocation(java.util.Collection extends VecNodeLocation> refNodeLocation) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeLocation collection is not null.
if (refNodeLocation == null) {
failWithMessage("Expecting refNodeLocation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefNodeLocation(), refNodeLocation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeLocation does not contain the given VecNodeLocation elements.
*
* @param refNodeLocation the given elements that should not be in actual VecTopologyNode's refNodeLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeLocation contains any given VecNodeLocation elements.
*/
public S doesNotHaveRefNodeLocation(VecNodeLocation... refNodeLocation) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeLocation varargs is not null.
if (refNodeLocation == null) failWithMessage("Expecting refNodeLocation parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefNodeLocation(), refNodeLocation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeLocation does not contain the given VecNodeLocation elements in Collection.
*
* @param refNodeLocation the given elements that should not be in actual VecTopologyNode's refNodeLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeLocation contains any given VecNodeLocation elements.
*/
public S doesNotHaveRefNodeLocation(java.util.Collection extends VecNodeLocation> refNodeLocation) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeLocation collection is not null.
if (refNodeLocation == null) {
failWithMessage("Expecting refNodeLocation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefNodeLocation(), refNodeLocation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode has no refNodeLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeLocation is not empty.
*/
public S hasNoRefNodeLocation() {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refNodeLocation but had :\n <%s>";
// check
if (actual.getRefNodeLocation().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefNodeLocation());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeMapping contains the given VecNodeMapping elements.
* @param refNodeMapping the given elements that should be contained in actual VecTopologyNode's refNodeMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeMapping does not contain all given VecNodeMapping elements.
*/
public S hasRefNodeMapping(VecNodeMapping... refNodeMapping) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeMapping varargs is not null.
if (refNodeMapping == null) failWithMessage("Expecting refNodeMapping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefNodeMapping(), refNodeMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeMapping contains the given VecNodeMapping elements in Collection.
* @param refNodeMapping the given elements that should be contained in actual VecTopologyNode's refNodeMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeMapping does not contain all given VecNodeMapping elements.
*/
public S hasRefNodeMapping(java.util.Collection extends VecNodeMapping> refNodeMapping) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeMapping collection is not null.
if (refNodeMapping == null) {
failWithMessage("Expecting refNodeMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefNodeMapping(), refNodeMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeMapping contains only the given VecNodeMapping elements and nothing else in whatever order.
* @param refNodeMapping the given elements that should be contained in actual VecTopologyNode's refNodeMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeMapping does not contain all given VecNodeMapping elements.
*/
public S hasOnlyRefNodeMapping(VecNodeMapping... refNodeMapping) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeMapping varargs is not null.
if (refNodeMapping == null) failWithMessage("Expecting refNodeMapping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefNodeMapping(), refNodeMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeMapping contains only the given VecNodeMapping elements in Collection and nothing else in whatever order.
* @param refNodeMapping the given elements that should be contained in actual VecTopologyNode's refNodeMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeMapping does not contain all given VecNodeMapping elements.
*/
public S hasOnlyRefNodeMapping(java.util.Collection extends VecNodeMapping> refNodeMapping) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeMapping collection is not null.
if (refNodeMapping == null) {
failWithMessage("Expecting refNodeMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefNodeMapping(), refNodeMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeMapping does not contain the given VecNodeMapping elements.
*
* @param refNodeMapping the given elements that should not be in actual VecTopologyNode's refNodeMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeMapping contains any given VecNodeMapping elements.
*/
public S doesNotHaveRefNodeMapping(VecNodeMapping... refNodeMapping) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeMapping varargs is not null.
if (refNodeMapping == null) failWithMessage("Expecting refNodeMapping parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefNodeMapping(), refNodeMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refNodeMapping does not contain the given VecNodeMapping elements in Collection.
*
* @param refNodeMapping the given elements that should not be in actual VecTopologyNode's refNodeMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeMapping contains any given VecNodeMapping elements.
*/
public S doesNotHaveRefNodeMapping(java.util.Collection extends VecNodeMapping> refNodeMapping) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecNodeMapping collection is not null.
if (refNodeMapping == null) {
failWithMessage("Expecting refNodeMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefNodeMapping(), refNodeMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode has no refNodeMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refNodeMapping is not empty.
*/
public S hasNoRefNodeMapping() {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refNodeMapping but had :\n <%s>";
// check
if (actual.getRefNodeMapping().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefNodeMapping());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologyNode contains the given VecTopologyNode elements.
* @param refTopologyNode the given elements that should be contained in actual VecTopologyNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasRefTopologyNode(VecTopologyNode... refTopologyNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode varargs is not null.
if (refTopologyNode == null) failWithMessage("Expecting refTopologyNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologyNode(), refTopologyNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologyNode contains the given VecTopologyNode elements in Collection.
* @param refTopologyNode the given elements that should be contained in actual VecTopologyNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasRefTopologyNode(java.util.Collection extends VecTopologyNode> refTopologyNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode collection is not null.
if (refTopologyNode == null) {
failWithMessage("Expecting refTopologyNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologyNode(), refTopologyNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologyNode contains only the given VecTopologyNode elements and nothing else in whatever order.
* @param refTopologyNode the given elements that should be contained in actual VecTopologyNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasOnlyRefTopologyNode(VecTopologyNode... refTopologyNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode varargs is not null.
if (refTopologyNode == null) failWithMessage("Expecting refTopologyNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologyNode(), refTopologyNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologyNode contains only the given VecTopologyNode elements in Collection and nothing else in whatever order.
* @param refTopologyNode the given elements that should be contained in actual VecTopologyNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasOnlyRefTopologyNode(java.util.Collection extends VecTopologyNode> refTopologyNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode collection is not null.
if (refTopologyNode == null) {
failWithMessage("Expecting refTopologyNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologyNode(), refTopologyNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologyNode does not contain the given VecTopologyNode elements.
*
* @param refTopologyNode the given elements that should not be in actual VecTopologyNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologyNode contains any given VecTopologyNode elements.
*/
public S doesNotHaveRefTopologyNode(VecTopologyNode... refTopologyNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode varargs is not null.
if (refTopologyNode == null) failWithMessage("Expecting refTopologyNode parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologyNode(), refTopologyNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologyNode does not contain the given VecTopologyNode elements in Collection.
*
* @param refTopologyNode the given elements that should not be in actual VecTopologyNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologyNode contains any given VecTopologyNode elements.
*/
public S doesNotHaveRefTopologyNode(java.util.Collection extends VecTopologyNode> refTopologyNode) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode collection is not null.
if (refTopologyNode == null) {
failWithMessage("Expecting refTopologyNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologyNode(), refTopologyNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode has no refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologyNode is not empty.
*/
public S hasNoRefTopologyNode() {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTopologyNode but had :\n <%s>";
// check
if (actual.getRefTopologyNode().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTopologyNode());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologySegment contains the given VecTopologySegment elements.
* @param refTopologySegment the given elements that should be contained in actual VecTopologyNode's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasRefTopologySegment(VecTopologySegment... refTopologySegment) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment varargs is not null.
if (refTopologySegment == null) failWithMessage("Expecting refTopologySegment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologySegment(), refTopologySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologySegment contains the given VecTopologySegment elements in Collection.
* @param refTopologySegment the given elements that should be contained in actual VecTopologyNode's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasRefTopologySegment(java.util.Collection extends VecTopologySegment> refTopologySegment) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment collection is not null.
if (refTopologySegment == null) {
failWithMessage("Expecting refTopologySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologySegment(), refTopologySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologySegment contains only the given VecTopologySegment elements and nothing else in whatever order.
* @param refTopologySegment the given elements that should be contained in actual VecTopologyNode's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasOnlyRefTopologySegment(VecTopologySegment... refTopologySegment) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment varargs is not null.
if (refTopologySegment == null) failWithMessage("Expecting refTopologySegment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologySegment(), refTopologySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologySegment contains only the given VecTopologySegment elements in Collection and nothing else in whatever order.
* @param refTopologySegment the given elements that should be contained in actual VecTopologyNode's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasOnlyRefTopologySegment(java.util.Collection extends VecTopologySegment> refTopologySegment) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment collection is not null.
if (refTopologySegment == null) {
failWithMessage("Expecting refTopologySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologySegment(), refTopologySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologySegment does not contain the given VecTopologySegment elements.
*
* @param refTopologySegment the given elements that should not be in actual VecTopologyNode's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologySegment contains any given VecTopologySegment elements.
*/
public S doesNotHaveRefTopologySegment(VecTopologySegment... refTopologySegment) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment varargs is not null.
if (refTopologySegment == null) failWithMessage("Expecting refTopologySegment parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologySegment(), refTopologySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode's refTopologySegment does not contain the given VecTopologySegment elements in Collection.
*
* @param refTopologySegment the given elements that should not be in actual VecTopologyNode's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologySegment contains any given VecTopologySegment elements.
*/
public S doesNotHaveRefTopologySegment(java.util.Collection extends VecTopologySegment> refTopologySegment) {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment collection is not null.
if (refTopologySegment == null) {
failWithMessage("Expecting refTopologySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologySegment(), refTopologySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologyNode has no refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologyNode's refTopologySegment is not empty.
*/
public S hasNoRefTopologySegment() {
// check that actual VecTopologyNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTopologySegment but had :\n <%s>";
// check
if (actual.getRefTopologySegment().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTopologySegment());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy