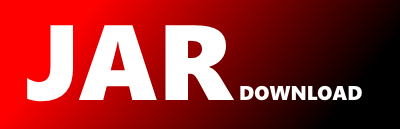
com.foursoft.vecmodel.vec120.AbstractVecTopologySegmentAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTopologySegment} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTopologySegmentAssert, A extends VecTopologySegment> extends AbstractVecConfigurableElementAssert {
/**
* Creates a new {@link AbstractVecTopologySegmentAssert}
to make assertions on actual VecTopologySegment.
* @param actual the VecTopologySegment we want to make assertions on.
*/
protected AbstractVecTopologySegmentAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTopologySegment's aliasIds contains the given VecAliasIdentification elements.
* @param aliasIds the given elements that should be contained in actual VecTopologySegment's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's aliasIds contains the given VecAliasIdentification elements in Collection.
* @param aliasIds the given elements that should be contained in actual VecTopologySegment's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's aliasIds contains only the given VecAliasIdentification elements and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecTopologySegment's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's aliasIds contains only the given VecAliasIdentification elements in Collection and nothing else in whatever order.
* @param aliasIds the given elements that should be contained in actual VecTopologySegment's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's aliasIds does not contain all given VecAliasIdentification elements.
*/
public S hasOnlyAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's aliasIds does not contain the given VecAliasIdentification elements.
*
* @param aliasIds the given elements that should not be in actual VecTopologySegment's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(VecAliasIdentification... aliasIds) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification varargs is not null.
if (aliasIds == null) failWithMessage("Expecting aliasIds parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's aliasIds does not contain the given VecAliasIdentification elements in Collection.
*
* @param aliasIds the given elements that should not be in actual VecTopologySegment's aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's aliasIds contains any given VecAliasIdentification elements.
*/
public S doesNotHaveAliasIds(java.util.Collection extends VecAliasIdentification> aliasIds) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecAliasIdentification collection is not null.
if (aliasIds == null) {
failWithMessage("Expecting aliasIds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAliasIds(), aliasIds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no aliasIds.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's aliasIds is not empty.
*/
public S hasNoAliasIds() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have aliasIds but had :\n <%s>";
// check
if (actual.getAliasIds().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAliasIds());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's crossSectionAreaInformations contains the given VecSegmentCrossSectionArea elements.
* @param crossSectionAreaInformations the given elements that should be contained in actual VecTopologySegment's crossSectionAreaInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's crossSectionAreaInformations does not contain all given VecSegmentCrossSectionArea elements.
*/
public S hasCrossSectionAreaInformations(VecSegmentCrossSectionArea... crossSectionAreaInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentCrossSectionArea varargs is not null.
if (crossSectionAreaInformations == null) failWithMessage("Expecting crossSectionAreaInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCrossSectionAreaInformations(), crossSectionAreaInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's crossSectionAreaInformations contains the given VecSegmentCrossSectionArea elements in Collection.
* @param crossSectionAreaInformations the given elements that should be contained in actual VecTopologySegment's crossSectionAreaInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's crossSectionAreaInformations does not contain all given VecSegmentCrossSectionArea elements.
*/
public S hasCrossSectionAreaInformations(java.util.Collection extends VecSegmentCrossSectionArea> crossSectionAreaInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentCrossSectionArea collection is not null.
if (crossSectionAreaInformations == null) {
failWithMessage("Expecting crossSectionAreaInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCrossSectionAreaInformations(), crossSectionAreaInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's crossSectionAreaInformations contains only the given VecSegmentCrossSectionArea elements and nothing else in whatever order.
* @param crossSectionAreaInformations the given elements that should be contained in actual VecTopologySegment's crossSectionAreaInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's crossSectionAreaInformations does not contain all given VecSegmentCrossSectionArea elements.
*/
public S hasOnlyCrossSectionAreaInformations(VecSegmentCrossSectionArea... crossSectionAreaInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentCrossSectionArea varargs is not null.
if (crossSectionAreaInformations == null) failWithMessage("Expecting crossSectionAreaInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCrossSectionAreaInformations(), crossSectionAreaInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's crossSectionAreaInformations contains only the given VecSegmentCrossSectionArea elements in Collection and nothing else in whatever order.
* @param crossSectionAreaInformations the given elements that should be contained in actual VecTopologySegment's crossSectionAreaInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's crossSectionAreaInformations does not contain all given VecSegmentCrossSectionArea elements.
*/
public S hasOnlyCrossSectionAreaInformations(java.util.Collection extends VecSegmentCrossSectionArea> crossSectionAreaInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentCrossSectionArea collection is not null.
if (crossSectionAreaInformations == null) {
failWithMessage("Expecting crossSectionAreaInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCrossSectionAreaInformations(), crossSectionAreaInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's crossSectionAreaInformations does not contain the given VecSegmentCrossSectionArea elements.
*
* @param crossSectionAreaInformations the given elements that should not be in actual VecTopologySegment's crossSectionAreaInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's crossSectionAreaInformations contains any given VecSegmentCrossSectionArea elements.
*/
public S doesNotHaveCrossSectionAreaInformations(VecSegmentCrossSectionArea... crossSectionAreaInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentCrossSectionArea varargs is not null.
if (crossSectionAreaInformations == null) failWithMessage("Expecting crossSectionAreaInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCrossSectionAreaInformations(), crossSectionAreaInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's crossSectionAreaInformations does not contain the given VecSegmentCrossSectionArea elements in Collection.
*
* @param crossSectionAreaInformations the given elements that should not be in actual VecTopologySegment's crossSectionAreaInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's crossSectionAreaInformations contains any given VecSegmentCrossSectionArea elements.
*/
public S doesNotHaveCrossSectionAreaInformations(java.util.Collection extends VecSegmentCrossSectionArea> crossSectionAreaInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentCrossSectionArea collection is not null.
if (crossSectionAreaInformations == null) {
failWithMessage("Expecting crossSectionAreaInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCrossSectionAreaInformations(), crossSectionAreaInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no crossSectionAreaInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's crossSectionAreaInformations is not empty.
*/
public S hasNoCrossSectionAreaInformations() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have crossSectionAreaInformations but had :\n <%s>";
// check
if (actual.getCrossSectionAreaInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getCrossSectionAreaInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's endNode is equal to the given one.
* @param endNode the given endNode to compare the actual VecTopologySegment's endNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologySegment's endNode is not equal to the given one.
*/
public S hasEndNode(VecTopologyNode endNode) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting endNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTopologyNode actualEndNode = actual.getEndNode();
if (!Objects.areEqual(actualEndNode, endNode)) {
failWithMessage(assertjErrorMessage, actual, endNode, actualEndNode);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's form is equal to the given one.
* @param form the given form to compare the actual VecTopologySegment's form to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologySegment's form is not equal to the given one.
*/
public S hasForm(String form) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting form of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualForm = actual.getForm();
if (!Objects.areEqual(actualForm, form)) {
failWithMessage(assertjErrorMessage, actual, form, actualForm);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's identification is equal to the given one.
* @param identification the given identification to compare the actual VecTopologySegment's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologySegment's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's instantiatedSegment is equal to the given one.
* @param instantiatedSegment the given instantiatedSegment to compare the actual VecTopologySegment's instantiatedSegment to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologySegment's instantiatedSegment is not equal to the given one.
*/
public S hasInstantiatedSegment(VecTopologySegment instantiatedSegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting instantiatedSegment of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTopologySegment actualInstantiatedSegment = actual.getInstantiatedSegment();
if (!Objects.areEqual(actualInstantiatedSegment, instantiatedSegment)) {
failWithMessage(assertjErrorMessage, actual, instantiatedSegment, actualInstantiatedSegment);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's lengthInformations contains the given VecSegmentLength elements.
* @param lengthInformations the given elements that should be contained in actual VecTopologySegment's lengthInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's lengthInformations does not contain all given VecSegmentLength elements.
*/
public S hasLengthInformations(VecSegmentLength... lengthInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLength varargs is not null.
if (lengthInformations == null) failWithMessage("Expecting lengthInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getLengthInformations(), lengthInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's lengthInformations contains the given VecSegmentLength elements in Collection.
* @param lengthInformations the given elements that should be contained in actual VecTopologySegment's lengthInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's lengthInformations does not contain all given VecSegmentLength elements.
*/
public S hasLengthInformations(java.util.Collection extends VecSegmentLength> lengthInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLength collection is not null.
if (lengthInformations == null) {
failWithMessage("Expecting lengthInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getLengthInformations(), lengthInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's lengthInformations contains only the given VecSegmentLength elements and nothing else in whatever order.
* @param lengthInformations the given elements that should be contained in actual VecTopologySegment's lengthInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's lengthInformations does not contain all given VecSegmentLength elements.
*/
public S hasOnlyLengthInformations(VecSegmentLength... lengthInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLength varargs is not null.
if (lengthInformations == null) failWithMessage("Expecting lengthInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getLengthInformations(), lengthInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's lengthInformations contains only the given VecSegmentLength elements in Collection and nothing else in whatever order.
* @param lengthInformations the given elements that should be contained in actual VecTopologySegment's lengthInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's lengthInformations does not contain all given VecSegmentLength elements.
*/
public S hasOnlyLengthInformations(java.util.Collection extends VecSegmentLength> lengthInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLength collection is not null.
if (lengthInformations == null) {
failWithMessage("Expecting lengthInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getLengthInformations(), lengthInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's lengthInformations does not contain the given VecSegmentLength elements.
*
* @param lengthInformations the given elements that should not be in actual VecTopologySegment's lengthInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's lengthInformations contains any given VecSegmentLength elements.
*/
public S doesNotHaveLengthInformations(VecSegmentLength... lengthInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLength varargs is not null.
if (lengthInformations == null) failWithMessage("Expecting lengthInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getLengthInformations(), lengthInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's lengthInformations does not contain the given VecSegmentLength elements in Collection.
*
* @param lengthInformations the given elements that should not be in actual VecTopologySegment's lengthInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's lengthInformations contains any given VecSegmentLength elements.
*/
public S doesNotHaveLengthInformations(java.util.Collection extends VecSegmentLength> lengthInformations) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLength collection is not null.
if (lengthInformations == null) {
failWithMessage("Expecting lengthInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getLengthInformations(), lengthInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no lengthInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's lengthInformations is not empty.
*/
public S hasNoLengthInformations() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have lengthInformations but had :\n <%s>";
// check
if (actual.getLengthInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getLengthInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's parentTopologySpecification is equal to the given one.
* @param parentTopologySpecification the given parentTopologySpecification to compare the actual VecTopologySegment's parentTopologySpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologySegment's parentTopologySpecification is not equal to the given one.
*/
public S hasParentTopologySpecification(VecTopologySpecification parentTopologySpecification) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentTopologySpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTopologySpecification actualParentTopologySpecification = actual.getParentTopologySpecification();
if (!Objects.areEqual(actualParentTopologySpecification, parentTopologySpecification)) {
failWithMessage(assertjErrorMessage, actual, parentTopologySpecification, actualParentTopologySpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's processingInstructions contains the given VecLocalizedString elements.
* @param processingInstructions the given elements that should be contained in actual VecTopologySegment's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasProcessingInstructions(VecLocalizedString... processingInstructions) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's processingInstructions contains the given VecLocalizedString elements in Collection.
* @param processingInstructions the given elements that should be contained in actual VecTopologySegment's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasProcessingInstructions(java.util.Collection extends VecLocalizedString> processingInstructions) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's processingInstructions contains only the given VecLocalizedString elements and nothing else in whatever order.
* @param processingInstructions the given elements that should be contained in actual VecTopologySegment's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasOnlyProcessingInstructions(VecLocalizedString... processingInstructions) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's processingInstructions contains only the given VecLocalizedString elements in Collection and nothing else in whatever order.
* @param processingInstructions the given elements that should be contained in actual VecTopologySegment's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's processingInstructions does not contain all given VecLocalizedString elements.
*/
public S hasOnlyProcessingInstructions(java.util.Collection extends VecLocalizedString> processingInstructions) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's processingInstructions does not contain the given VecLocalizedString elements.
*
* @param processingInstructions the given elements that should not be in actual VecTopologySegment's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's processingInstructions contains any given VecLocalizedString elements.
*/
public S doesNotHaveProcessingInstructions(VecLocalizedString... processingInstructions) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (processingInstructions == null) failWithMessage("Expecting processingInstructions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProcessingInstructions(), processingInstructions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's processingInstructions does not contain the given VecLocalizedString elements in Collection.
*
* @param processingInstructions the given elements that should not be in actual VecTopologySegment's processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's processingInstructions contains any given VecLocalizedString elements.
*/
public S doesNotHaveProcessingInstructions(java.util.Collection extends VecLocalizedString> processingInstructions) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (processingInstructions == null) {
failWithMessage("Expecting processingInstructions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProcessingInstructions(), processingInstructions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no processingInstructions.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's processingInstructions is not empty.
*/
public S hasNoProcessingInstructions() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have processingInstructions but had :\n <%s>";
// check
if (actual.getProcessingInstructions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getProcessingInstructions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refGeometrySegment contains the given VecGeometrySegment elements.
* @param refGeometrySegment the given elements that should be contained in actual VecTopologySegment's refGeometrySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refGeometrySegment does not contain all given VecGeometrySegment elements.
*/
public S hasRefGeometrySegment(VecGeometrySegment... refGeometrySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment varargs is not null.
if (refGeometrySegment == null) failWithMessage("Expecting refGeometrySegment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefGeometrySegment(), refGeometrySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refGeometrySegment contains the given VecGeometrySegment elements in Collection.
* @param refGeometrySegment the given elements that should be contained in actual VecTopologySegment's refGeometrySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refGeometrySegment does not contain all given VecGeometrySegment elements.
*/
public S hasRefGeometrySegment(java.util.Collection extends VecGeometrySegment> refGeometrySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment collection is not null.
if (refGeometrySegment == null) {
failWithMessage("Expecting refGeometrySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefGeometrySegment(), refGeometrySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refGeometrySegment contains only the given VecGeometrySegment elements and nothing else in whatever order.
* @param refGeometrySegment the given elements that should be contained in actual VecTopologySegment's refGeometrySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refGeometrySegment does not contain all given VecGeometrySegment elements.
*/
public S hasOnlyRefGeometrySegment(VecGeometrySegment... refGeometrySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment varargs is not null.
if (refGeometrySegment == null) failWithMessage("Expecting refGeometrySegment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefGeometrySegment(), refGeometrySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refGeometrySegment contains only the given VecGeometrySegment elements in Collection and nothing else in whatever order.
* @param refGeometrySegment the given elements that should be contained in actual VecTopologySegment's refGeometrySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refGeometrySegment does not contain all given VecGeometrySegment elements.
*/
public S hasOnlyRefGeometrySegment(java.util.Collection extends VecGeometrySegment> refGeometrySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment collection is not null.
if (refGeometrySegment == null) {
failWithMessage("Expecting refGeometrySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefGeometrySegment(), refGeometrySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refGeometrySegment does not contain the given VecGeometrySegment elements.
*
* @param refGeometrySegment the given elements that should not be in actual VecTopologySegment's refGeometrySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refGeometrySegment contains any given VecGeometrySegment elements.
*/
public S doesNotHaveRefGeometrySegment(VecGeometrySegment... refGeometrySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment varargs is not null.
if (refGeometrySegment == null) failWithMessage("Expecting refGeometrySegment parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefGeometrySegment(), refGeometrySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refGeometrySegment does not contain the given VecGeometrySegment elements in Collection.
*
* @param refGeometrySegment the given elements that should not be in actual VecTopologySegment's refGeometrySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refGeometrySegment contains any given VecGeometrySegment elements.
*/
public S doesNotHaveRefGeometrySegment(java.util.Collection extends VecGeometrySegment> refGeometrySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment collection is not null.
if (refGeometrySegment == null) {
failWithMessage("Expecting refGeometrySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefGeometrySegment(), refGeometrySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no refGeometrySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refGeometrySegment is not empty.
*/
public S hasNoRefGeometrySegment() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refGeometrySegment but had :\n <%s>";
// check
if (actual.getRefGeometrySegment().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefGeometrySegment());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refPath contains the given VecPath elements.
* @param refPath the given elements that should be contained in actual VecTopologySegment's refPath.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refPath does not contain all given VecPath elements.
*/
public S hasRefPath(VecPath... refPath) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecPath varargs is not null.
if (refPath == null) failWithMessage("Expecting refPath parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPath(), refPath);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refPath contains the given VecPath elements in Collection.
* @param refPath the given elements that should be contained in actual VecTopologySegment's refPath.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refPath does not contain all given VecPath elements.
*/
public S hasRefPath(java.util.Collection extends VecPath> refPath) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecPath collection is not null.
if (refPath == null) {
failWithMessage("Expecting refPath parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPath(), refPath.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refPath contains only the given VecPath elements and nothing else in whatever order.
* @param refPath the given elements that should be contained in actual VecTopologySegment's refPath.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refPath does not contain all given VecPath elements.
*/
public S hasOnlyRefPath(VecPath... refPath) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecPath varargs is not null.
if (refPath == null) failWithMessage("Expecting refPath parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPath(), refPath);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refPath contains only the given VecPath elements in Collection and nothing else in whatever order.
* @param refPath the given elements that should be contained in actual VecTopologySegment's refPath.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refPath does not contain all given VecPath elements.
*/
public S hasOnlyRefPath(java.util.Collection extends VecPath> refPath) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecPath collection is not null.
if (refPath == null) {
failWithMessage("Expecting refPath parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPath(), refPath.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refPath does not contain the given VecPath elements.
*
* @param refPath the given elements that should not be in actual VecTopologySegment's refPath.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refPath contains any given VecPath elements.
*/
public S doesNotHaveRefPath(VecPath... refPath) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecPath varargs is not null.
if (refPath == null) failWithMessage("Expecting refPath parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPath(), refPath);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refPath does not contain the given VecPath elements in Collection.
*
* @param refPath the given elements that should not be in actual VecTopologySegment's refPath.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refPath contains any given VecPath elements.
*/
public S doesNotHaveRefPath(java.util.Collection extends VecPath> refPath) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecPath collection is not null.
if (refPath == null) {
failWithMessage("Expecting refPath parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPath(), refPath.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no refPath.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refPath is not empty.
*/
public S hasNoRefPath() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPath but had :\n <%s>";
// check
if (actual.getRefPath().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPath());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refRouting contains the given VecRouting elements.
* @param refRouting the given elements that should be contained in actual VecTopologySegment's refRouting.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refRouting does not contain all given VecRouting elements.
*/
public S hasRefRouting(VecRouting... refRouting) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecRouting varargs is not null.
if (refRouting == null) failWithMessage("Expecting refRouting parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefRouting(), refRouting);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refRouting contains the given VecRouting elements in Collection.
* @param refRouting the given elements that should be contained in actual VecTopologySegment's refRouting.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refRouting does not contain all given VecRouting elements.
*/
public S hasRefRouting(java.util.Collection extends VecRouting> refRouting) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecRouting collection is not null.
if (refRouting == null) {
failWithMessage("Expecting refRouting parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefRouting(), refRouting.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refRouting contains only the given VecRouting elements and nothing else in whatever order.
* @param refRouting the given elements that should be contained in actual VecTopologySegment's refRouting.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refRouting does not contain all given VecRouting elements.
*/
public S hasOnlyRefRouting(VecRouting... refRouting) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecRouting varargs is not null.
if (refRouting == null) failWithMessage("Expecting refRouting parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefRouting(), refRouting);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refRouting contains only the given VecRouting elements in Collection and nothing else in whatever order.
* @param refRouting the given elements that should be contained in actual VecTopologySegment's refRouting.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refRouting does not contain all given VecRouting elements.
*/
public S hasOnlyRefRouting(java.util.Collection extends VecRouting> refRouting) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecRouting collection is not null.
if (refRouting == null) {
failWithMessage("Expecting refRouting parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefRouting(), refRouting.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refRouting does not contain the given VecRouting elements.
*
* @param refRouting the given elements that should not be in actual VecTopologySegment's refRouting.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refRouting contains any given VecRouting elements.
*/
public S doesNotHaveRefRouting(VecRouting... refRouting) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecRouting varargs is not null.
if (refRouting == null) failWithMessage("Expecting refRouting parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefRouting(), refRouting);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refRouting does not contain the given VecRouting elements in Collection.
*
* @param refRouting the given elements that should not be in actual VecTopologySegment's refRouting.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refRouting contains any given VecRouting elements.
*/
public S doesNotHaveRefRouting(java.util.Collection extends VecRouting> refRouting) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecRouting collection is not null.
if (refRouting == null) {
failWithMessage("Expecting refRouting parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefRouting(), refRouting.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no refRouting.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refRouting is not empty.
*/
public S hasNoRefRouting() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refRouting but had :\n <%s>";
// check
if (actual.getRefRouting().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefRouting());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentLocation contains the given VecSegmentLocation elements.
* @param refSegmentLocation the given elements that should be contained in actual VecTopologySegment's refSegmentLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentLocation does not contain all given VecSegmentLocation elements.
*/
public S hasRefSegmentLocation(VecSegmentLocation... refSegmentLocation) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLocation varargs is not null.
if (refSegmentLocation == null) failWithMessage("Expecting refSegmentLocation parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentLocation(), refSegmentLocation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentLocation contains the given VecSegmentLocation elements in Collection.
* @param refSegmentLocation the given elements that should be contained in actual VecTopologySegment's refSegmentLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentLocation does not contain all given VecSegmentLocation elements.
*/
public S hasRefSegmentLocation(java.util.Collection extends VecSegmentLocation> refSegmentLocation) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLocation collection is not null.
if (refSegmentLocation == null) {
failWithMessage("Expecting refSegmentLocation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentLocation(), refSegmentLocation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentLocation contains only the given VecSegmentLocation elements and nothing else in whatever order.
* @param refSegmentLocation the given elements that should be contained in actual VecTopologySegment's refSegmentLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentLocation does not contain all given VecSegmentLocation elements.
*/
public S hasOnlyRefSegmentLocation(VecSegmentLocation... refSegmentLocation) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLocation varargs is not null.
if (refSegmentLocation == null) failWithMessage("Expecting refSegmentLocation parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentLocation(), refSegmentLocation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentLocation contains only the given VecSegmentLocation elements in Collection and nothing else in whatever order.
* @param refSegmentLocation the given elements that should be contained in actual VecTopologySegment's refSegmentLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentLocation does not contain all given VecSegmentLocation elements.
*/
public S hasOnlyRefSegmentLocation(java.util.Collection extends VecSegmentLocation> refSegmentLocation) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLocation collection is not null.
if (refSegmentLocation == null) {
failWithMessage("Expecting refSegmentLocation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentLocation(), refSegmentLocation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentLocation does not contain the given VecSegmentLocation elements.
*
* @param refSegmentLocation the given elements that should not be in actual VecTopologySegment's refSegmentLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentLocation contains any given VecSegmentLocation elements.
*/
public S doesNotHaveRefSegmentLocation(VecSegmentLocation... refSegmentLocation) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLocation varargs is not null.
if (refSegmentLocation == null) failWithMessage("Expecting refSegmentLocation parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentLocation(), refSegmentLocation);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentLocation does not contain the given VecSegmentLocation elements in Collection.
*
* @param refSegmentLocation the given elements that should not be in actual VecTopologySegment's refSegmentLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentLocation contains any given VecSegmentLocation elements.
*/
public S doesNotHaveRefSegmentLocation(java.util.Collection extends VecSegmentLocation> refSegmentLocation) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentLocation collection is not null.
if (refSegmentLocation == null) {
failWithMessage("Expecting refSegmentLocation parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentLocation(), refSegmentLocation.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no refSegmentLocation.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentLocation is not empty.
*/
public S hasNoRefSegmentLocation() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refSegmentLocation but had :\n <%s>";
// check
if (actual.getRefSegmentLocation().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefSegmentLocation());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentMapping contains the given VecSegmentMapping elements.
* @param refSegmentMapping the given elements that should be contained in actual VecTopologySegment's refSegmentMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentMapping does not contain all given VecSegmentMapping elements.
*/
public S hasRefSegmentMapping(VecSegmentMapping... refSegmentMapping) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentMapping varargs is not null.
if (refSegmentMapping == null) failWithMessage("Expecting refSegmentMapping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentMapping(), refSegmentMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentMapping contains the given VecSegmentMapping elements in Collection.
* @param refSegmentMapping the given elements that should be contained in actual VecTopologySegment's refSegmentMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentMapping does not contain all given VecSegmentMapping elements.
*/
public S hasRefSegmentMapping(java.util.Collection extends VecSegmentMapping> refSegmentMapping) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentMapping collection is not null.
if (refSegmentMapping == null) {
failWithMessage("Expecting refSegmentMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentMapping(), refSegmentMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentMapping contains only the given VecSegmentMapping elements and nothing else in whatever order.
* @param refSegmentMapping the given elements that should be contained in actual VecTopologySegment's refSegmentMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentMapping does not contain all given VecSegmentMapping elements.
*/
public S hasOnlyRefSegmentMapping(VecSegmentMapping... refSegmentMapping) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentMapping varargs is not null.
if (refSegmentMapping == null) failWithMessage("Expecting refSegmentMapping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentMapping(), refSegmentMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentMapping contains only the given VecSegmentMapping elements in Collection and nothing else in whatever order.
* @param refSegmentMapping the given elements that should be contained in actual VecTopologySegment's refSegmentMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentMapping does not contain all given VecSegmentMapping elements.
*/
public S hasOnlyRefSegmentMapping(java.util.Collection extends VecSegmentMapping> refSegmentMapping) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentMapping collection is not null.
if (refSegmentMapping == null) {
failWithMessage("Expecting refSegmentMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentMapping(), refSegmentMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentMapping does not contain the given VecSegmentMapping elements.
*
* @param refSegmentMapping the given elements that should not be in actual VecTopologySegment's refSegmentMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentMapping contains any given VecSegmentMapping elements.
*/
public S doesNotHaveRefSegmentMapping(VecSegmentMapping... refSegmentMapping) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentMapping varargs is not null.
if (refSegmentMapping == null) failWithMessage("Expecting refSegmentMapping parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentMapping(), refSegmentMapping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refSegmentMapping does not contain the given VecSegmentMapping elements in Collection.
*
* @param refSegmentMapping the given elements that should not be in actual VecTopologySegment's refSegmentMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentMapping contains any given VecSegmentMapping elements.
*/
public S doesNotHaveRefSegmentMapping(java.util.Collection extends VecSegmentMapping> refSegmentMapping) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentMapping collection is not null.
if (refSegmentMapping == null) {
failWithMessage("Expecting refSegmentMapping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentMapping(), refSegmentMapping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no refSegmentMapping.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refSegmentMapping is not empty.
*/
public S hasNoRefSegmentMapping() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refSegmentMapping but had :\n <%s>";
// check
if (actual.getRefSegmentMapping().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefSegmentMapping());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refTopologySegment contains the given VecTopologySegment elements.
* @param refTopologySegment the given elements that should be contained in actual VecTopologySegment's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasRefTopologySegment(VecTopologySegment... refTopologySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment varargs is not null.
if (refTopologySegment == null) failWithMessage("Expecting refTopologySegment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologySegment(), refTopologySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refTopologySegment contains the given VecTopologySegment elements in Collection.
* @param refTopologySegment the given elements that should be contained in actual VecTopologySegment's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasRefTopologySegment(java.util.Collection extends VecTopologySegment> refTopologySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment collection is not null.
if (refTopologySegment == null) {
failWithMessage("Expecting refTopologySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologySegment(), refTopologySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refTopologySegment contains only the given VecTopologySegment elements and nothing else in whatever order.
* @param refTopologySegment the given elements that should be contained in actual VecTopologySegment's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasOnlyRefTopologySegment(VecTopologySegment... refTopologySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment varargs is not null.
if (refTopologySegment == null) failWithMessage("Expecting refTopologySegment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologySegment(), refTopologySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refTopologySegment contains only the given VecTopologySegment elements in Collection and nothing else in whatever order.
* @param refTopologySegment the given elements that should be contained in actual VecTopologySegment's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refTopologySegment does not contain all given VecTopologySegment elements.
*/
public S hasOnlyRefTopologySegment(java.util.Collection extends VecTopologySegment> refTopologySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment collection is not null.
if (refTopologySegment == null) {
failWithMessage("Expecting refTopologySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologySegment(), refTopologySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refTopologySegment does not contain the given VecTopologySegment elements.
*
* @param refTopologySegment the given elements that should not be in actual VecTopologySegment's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refTopologySegment contains any given VecTopologySegment elements.
*/
public S doesNotHaveRefTopologySegment(VecTopologySegment... refTopologySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment varargs is not null.
if (refTopologySegment == null) failWithMessage("Expecting refTopologySegment parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologySegment(), refTopologySegment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refTopologySegment does not contain the given VecTopologySegment elements in Collection.
*
* @param refTopologySegment the given elements that should not be in actual VecTopologySegment's refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refTopologySegment contains any given VecTopologySegment elements.
*/
public S doesNotHaveRefTopologySegment(java.util.Collection extends VecTopologySegment> refTopologySegment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecTopologySegment collection is not null.
if (refTopologySegment == null) {
failWithMessage("Expecting refTopologySegment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologySegment(), refTopologySegment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no refTopologySegment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refTopologySegment is not empty.
*/
public S hasNoRefTopologySegment() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTopologySegment but had :\n <%s>";
// check
if (actual.getRefTopologySegment().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTopologySegment());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refZoneAssignment contains the given VecZoneAssignment elements.
* @param refZoneAssignment the given elements that should be contained in actual VecTopologySegment's refZoneAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refZoneAssignment does not contain all given VecZoneAssignment elements.
*/
public S hasRefZoneAssignment(VecZoneAssignment... refZoneAssignment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecZoneAssignment varargs is not null.
if (refZoneAssignment == null) failWithMessage("Expecting refZoneAssignment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefZoneAssignment(), refZoneAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refZoneAssignment contains the given VecZoneAssignment elements in Collection.
* @param refZoneAssignment the given elements that should be contained in actual VecTopologySegment's refZoneAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refZoneAssignment does not contain all given VecZoneAssignment elements.
*/
public S hasRefZoneAssignment(java.util.Collection extends VecZoneAssignment> refZoneAssignment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecZoneAssignment collection is not null.
if (refZoneAssignment == null) {
failWithMessage("Expecting refZoneAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefZoneAssignment(), refZoneAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refZoneAssignment contains only the given VecZoneAssignment elements and nothing else in whatever order.
* @param refZoneAssignment the given elements that should be contained in actual VecTopologySegment's refZoneAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refZoneAssignment does not contain all given VecZoneAssignment elements.
*/
public S hasOnlyRefZoneAssignment(VecZoneAssignment... refZoneAssignment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecZoneAssignment varargs is not null.
if (refZoneAssignment == null) failWithMessage("Expecting refZoneAssignment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefZoneAssignment(), refZoneAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refZoneAssignment contains only the given VecZoneAssignment elements in Collection and nothing else in whatever order.
* @param refZoneAssignment the given elements that should be contained in actual VecTopologySegment's refZoneAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refZoneAssignment does not contain all given VecZoneAssignment elements.
*/
public S hasOnlyRefZoneAssignment(java.util.Collection extends VecZoneAssignment> refZoneAssignment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecZoneAssignment collection is not null.
if (refZoneAssignment == null) {
failWithMessage("Expecting refZoneAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefZoneAssignment(), refZoneAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refZoneAssignment does not contain the given VecZoneAssignment elements.
*
* @param refZoneAssignment the given elements that should not be in actual VecTopologySegment's refZoneAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refZoneAssignment contains any given VecZoneAssignment elements.
*/
public S doesNotHaveRefZoneAssignment(VecZoneAssignment... refZoneAssignment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecZoneAssignment varargs is not null.
if (refZoneAssignment == null) failWithMessage("Expecting refZoneAssignment parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefZoneAssignment(), refZoneAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's refZoneAssignment does not contain the given VecZoneAssignment elements in Collection.
*
* @param refZoneAssignment the given elements that should not be in actual VecTopologySegment's refZoneAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refZoneAssignment contains any given VecZoneAssignment elements.
*/
public S doesNotHaveRefZoneAssignment(java.util.Collection extends VecZoneAssignment> refZoneAssignment) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// check that given VecZoneAssignment collection is not null.
if (refZoneAssignment == null) {
failWithMessage("Expecting refZoneAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefZoneAssignment(), refZoneAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment has no refZoneAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecTopologySegment's refZoneAssignment is not empty.
*/
public S hasNoRefZoneAssignment() {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refZoneAssignment but had :\n <%s>";
// check
if (actual.getRefZoneAssignment().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefZoneAssignment());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTopologySegment's startNode is equal to the given one.
* @param startNode the given startNode to compare the actual VecTopologySegment's startNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTopologySegment's startNode is not equal to the given one.
*/
public S hasStartNode(VecTopologyNode startNode) {
// check that actual VecTopologySegment we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting startNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTopologyNode actualStartNode = actual.getStartNode();
if (!Objects.areEqual(actualStartNode, startNode)) {
failWithMessage(assertjErrorMessage, actual, startNode, actualStartNode);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy