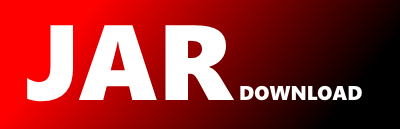
com.foursoft.vecmodel.vec120.AbstractVecTransformation2DAssert Maven / Gradle / Ivy
Show all versions of vec120-assertions Show documentation
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.Assertions;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTransformation2D} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTransformation2DAssert, A extends VecTransformation2D> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecTransformation2DAssert}
to make assertions on actual VecTransformation2D.
* @param actual the VecTransformation2D we want to make assertions on.
*/
protected AbstractVecTransformation2DAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTransformation2D's a11 is equal to the given one.
* @param a11 the given a11 to compare the actual VecTransformation2D's a11 to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a11 is not equal to the given one.
*/
public S hasA11(double a11) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting a11 of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for a11
double actualA11 = actual.getA11();
if (actualA11 != a11) {
failWithMessage(assertjErrorMessage, actual, a11, actualA11);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's a11 is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param a11 the value to compare the actual VecTransformation2D's a11 to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a11 is not close enough to the given value.
*/
public S hasA11CloseTo(double a11, double assertjOffset) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
double actualA11 = actual.getA11();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting a11:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualA11, a11, assertjOffset, Math.abs(a11 - actualA11));
// check
Assertions.assertThat(actualA11).overridingErrorMessage(assertjErrorMessage).isCloseTo(a11, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's a12 is equal to the given one.
* @param a12 the given a12 to compare the actual VecTransformation2D's a12 to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a12 is not equal to the given one.
*/
public S hasA12(double a12) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting a12 of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for a12
double actualA12 = actual.getA12();
if (actualA12 != a12) {
failWithMessage(assertjErrorMessage, actual, a12, actualA12);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's a12 is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param a12 the value to compare the actual VecTransformation2D's a12 to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a12 is not close enough to the given value.
*/
public S hasA12CloseTo(double a12, double assertjOffset) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
double actualA12 = actual.getA12();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting a12:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualA12, a12, assertjOffset, Math.abs(a12 - actualA12));
// check
Assertions.assertThat(actualA12).overridingErrorMessage(assertjErrorMessage).isCloseTo(a12, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's a21 is equal to the given one.
* @param a21 the given a21 to compare the actual VecTransformation2D's a21 to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a21 is not equal to the given one.
*/
public S hasA21(double a21) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting a21 of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for a21
double actualA21 = actual.getA21();
if (actualA21 != a21) {
failWithMessage(assertjErrorMessage, actual, a21, actualA21);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's a21 is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param a21 the value to compare the actual VecTransformation2D's a21 to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a21 is not close enough to the given value.
*/
public S hasA21CloseTo(double a21, double assertjOffset) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
double actualA21 = actual.getA21();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting a21:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualA21, a21, assertjOffset, Math.abs(a21 - actualA21));
// check
Assertions.assertThat(actualA21).overridingErrorMessage(assertjErrorMessage).isCloseTo(a21, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's a22 is equal to the given one.
* @param a22 the given a22 to compare the actual VecTransformation2D's a22 to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a22 is not equal to the given one.
*/
public S hasA22(double a22) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting a22 of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for a22
double actualA22 = actual.getA22();
if (actualA22 != a22) {
failWithMessage(assertjErrorMessage, actual, a22, actualA22);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's a22 is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param a22 the value to compare the actual VecTransformation2D's a22 to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's a22 is not close enough to the given value.
*/
public S hasA22CloseTo(double a22, double assertjOffset) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
double actualA22 = actual.getA22();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting a22:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualA22, a22, assertjOffset, Math.abs(a22 - actualA22));
// check
Assertions.assertThat(actualA22).overridingErrorMessage(assertjErrorMessage).isCloseTo(a22, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's origin is equal to the given one.
* @param origin the given origin to compare the actual VecTransformation2D's origin to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's origin is not equal to the given one.
*/
public S hasOrigin(VecCartesianPoint2D origin) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting origin of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCartesianPoint2D actualOrigin = actual.getOrigin();
if (!Objects.areEqual(actualOrigin, origin)) {
failWithMessage(assertjErrorMessage, actual, origin, actualOrigin);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTransformation2D's parentOccurrenceOrUsageViewItem2D is equal to the given one.
* @param parentOccurrenceOrUsageViewItem2D the given parentOccurrenceOrUsageViewItem2D to compare the actual VecTransformation2D's parentOccurrenceOrUsageViewItem2D to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTransformation2D's parentOccurrenceOrUsageViewItem2D is not equal to the given one.
*/
public S hasParentOccurrenceOrUsageViewItem2D(VecOccurrenceOrUsageViewItem2D parentOccurrenceOrUsageViewItem2D) {
// check that actual VecTransformation2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentOccurrenceOrUsageViewItem2D of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecOccurrenceOrUsageViewItem2D actualParentOccurrenceOrUsageViewItem2D = actual.getParentOccurrenceOrUsageViewItem2D();
if (!Objects.areEqual(actualParentOccurrenceOrUsageViewItem2D, parentOccurrenceOrUsageViewItem2D)) {
failWithMessage(assertjErrorMessage, actual, parentOccurrenceOrUsageViewItem2D, actualParentOccurrenceOrUsageViewItem2D);
}
// return the current assertion for method chaining
return myself;
}
}