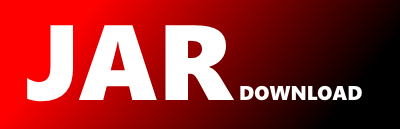
com.foursoft.vecmodel.vec120.AbstractVecTubeSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecTubeSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecTubeSpecificationAssert, A extends VecTubeSpecification> extends AbstractVecWireProtectionSpecificationAssert {
/**
* Creates a new {@link AbstractVecTubeSpecificationAssert}
to make assertions on actual VecTubeSpecification.
* @param actual the VecTubeSpecification we want to make assertions on.
*/
protected AbstractVecTubeSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecTubeSpecification's bendRadius is equal to the given one.
* @param bendRadius the given bendRadius to compare the actual VecTubeSpecification's bendRadius to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's bendRadius is not equal to the given one.
*/
public S hasBendRadius(VecNumericalValue bendRadius) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting bendRadius of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualBendRadius = actual.getBendRadius();
if (!Objects.areEqual(actualBendRadius, bendRadius)) {
failWithMessage(assertjErrorMessage, actual, bendRadius, actualBendRadius);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's height is equal to the given one.
* @param height the given height to compare the actual VecTubeSpecification's height to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's height is not equal to the given one.
*/
public S hasHeight(VecNumericalValue height) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting height of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualHeight = actual.getHeight();
if (!Objects.areEqual(actualHeight, height)) {
failWithMessage(assertjErrorMessage, actual, height, actualHeight);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's innerDiameter is equal to the given one.
* @param innerDiameter the given innerDiameter to compare the actual VecTubeSpecification's innerDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's innerDiameter is not equal to the given one.
*/
public S hasInnerDiameter(VecNumericalValue innerDiameter) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting innerDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualInnerDiameter = actual.getInnerDiameter();
if (!Objects.areEqual(actualInnerDiameter, innerDiameter)) {
failWithMessage(assertjErrorMessage, actual, innerDiameter, actualInnerDiameter);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification is is slit.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification is not is slit.
*/
public S isIsSlit() {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isIsSlit())) {
failWithMessage("\nExpecting that actual VecTubeSpecification is is slit but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification is not is slit.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification is is slit.
*/
public S isNotIsSlit() {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isIsSlit())) {
failWithMessage("\nExpecting that actual VecTubeSpecification is not is slit but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's length is equal to the given one.
* @param length the given length to compare the actual VecTubeSpecification's length to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's length is not equal to the given one.
*/
public S hasLength(VecNumericalValue length) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting length of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualLength = actual.getLength();
if (!Objects.areEqual(actualLength, length)) {
failWithMessage(assertjErrorMessage, actual, length, actualLength);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's nominalSize is equal to the given one.
* @param nominalSize the given nominalSize to compare the actual VecTubeSpecification's nominalSize to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's nominalSize is not equal to the given one.
*/
public S hasNominalSize(String nominalSize) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting nominalSize of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualNominalSize = actual.getNominalSize();
if (!Objects.areEqual(actualNominalSize, nominalSize)) {
failWithMessage(assertjErrorMessage, actual, nominalSize, actualNominalSize);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's outerDiameter is equal to the given one.
* @param outerDiameter the given outerDiameter to compare the actual VecTubeSpecification's outerDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's outerDiameter is not equal to the given one.
*/
public S hasOuterDiameter(VecNumericalValue outerDiameter) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting outerDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualOuterDiameter = actual.getOuterDiameter();
if (!Objects.areEqual(actualOuterDiameter, outerDiameter)) {
failWithMessage(assertjErrorMessage, actual, outerDiameter, actualOuterDiameter);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's secondaryNominalSize is equal to the given one.
* @param secondaryNominalSize the given secondaryNominalSize to compare the actual VecTubeSpecification's secondaryNominalSize to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's secondaryNominalSize is not equal to the given one.
*/
public S hasSecondaryNominalSize(String secondaryNominalSize) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting secondaryNominalSize of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSecondaryNominalSize = actual.getSecondaryNominalSize();
if (!Objects.areEqual(actualSecondaryNominalSize, secondaryNominalSize)) {
failWithMessage(assertjErrorMessage, actual, secondaryNominalSize, actualSecondaryNominalSize);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's shape is equal to the given one.
* @param shape the given shape to compare the actual VecTubeSpecification's shape to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's shape is not equal to the given one.
*/
public S hasShape(String shape) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting shape of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualShape = actual.getShape();
if (!Objects.areEqual(actualShape, shape)) {
failWithMessage(assertjErrorMessage, actual, shape, actualShape);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's slitStyle is equal to the given one.
* @param slitStyle the given slitStyle to compare the actual VecTubeSpecification's slitStyle to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's slitStyle is not equal to the given one.
*/
public S hasSlitStyle(String slitStyle) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting slitStyle of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualSlitStyle = actual.getSlitStyle();
if (!Objects.areEqual(actualSlitStyle, slitStyle)) {
failWithMessage(assertjErrorMessage, actual, slitStyle, actualSlitStyle);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's wallThickness is equal to the given one.
* @param wallThickness the given wallThickness to compare the actual VecTubeSpecification's wallThickness to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's wallThickness is not equal to the given one.
*/
public S hasWallThickness(VecNumericalValue wallThickness) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting wallThickness of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualWallThickness = actual.getWallThickness();
if (!Objects.areEqual(actualWallThickness, wallThickness)) {
failWithMessage(assertjErrorMessage, actual, wallThickness, actualWallThickness);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecTubeSpecification's width is equal to the given one.
* @param width the given width to compare the actual VecTubeSpecification's width to.
* @return this assertion object.
* @throws AssertionError - if the actual VecTubeSpecification's width is not equal to the given one.
*/
public S hasWidth(VecNumericalValue width) {
// check that actual VecTubeSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting width of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualWidth = actual.getWidth();
if (!Objects.areEqual(actualWidth, width)) {
failWithMessage(assertjErrorMessage, actual, width, actualWidth);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy