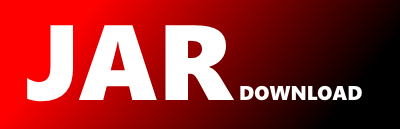
com.foursoft.vecmodel.vec120.AbstractVecUnitAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.AbstractObjectAssert;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecUnit} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecUnitAssert, A extends VecUnit> extends AbstractObjectAssert {
/**
* Creates a new {@link AbstractVecUnitAssert}
to make assertions on actual VecUnit.
* @param actual the VecUnit we want to make assertions on.
*/
protected AbstractVecUnitAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecUnit's exponent is equal to the given one.
* @param exponent the given exponent to compare the actual VecUnit's exponent to.
* @return this assertion object.
* @throws AssertionError - if the actual VecUnit's exponent is not equal to the given one.
*/
public S hasExponent(java.math.BigInteger exponent) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting exponent of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
java.math.BigInteger actualExponent = actual.getExponent();
if (!Objects.areEqual(actualExponent, exponent)) {
failWithMessage(assertjErrorMessage, actual, exponent, actualExponent);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's parentVecContent is equal to the given one.
* @param parentVecContent the given parentVecContent to compare the actual VecUnit's parentVecContent to.
* @return this assertion object.
* @throws AssertionError - if the actual VecUnit's parentVecContent is not equal to the given one.
*/
public S hasParentVecContent(VecContent parentVecContent) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentVecContent of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecContent actualParentVecContent = actual.getParentVecContent();
if (!Objects.areEqual(actualParentVecContent, parentVecContent)) {
failWithMessage(assertjErrorMessage, actual, parentVecContent, actualParentVecContent);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification2D contains the given VecBuildingBlockSpecification2D elements.
* @param refBuildingBlockSpecification2D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification2D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification2D does not contain all given VecBuildingBlockSpecification2D elements.
*/
public S hasRefBuildingBlockSpecification2D(VecBuildingBlockSpecification2D... refBuildingBlockSpecification2D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification2D varargs is not null.
if (refBuildingBlockSpecification2D == null) failWithMessage("Expecting refBuildingBlockSpecification2D parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBuildingBlockSpecification2D(), refBuildingBlockSpecification2D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification2D contains the given VecBuildingBlockSpecification2D elements in Collection.
* @param refBuildingBlockSpecification2D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification2D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification2D does not contain all given VecBuildingBlockSpecification2D elements.
*/
public S hasRefBuildingBlockSpecification2D(java.util.Collection extends VecBuildingBlockSpecification2D> refBuildingBlockSpecification2D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification2D collection is not null.
if (refBuildingBlockSpecification2D == null) {
failWithMessage("Expecting refBuildingBlockSpecification2D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBuildingBlockSpecification2D(), refBuildingBlockSpecification2D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification2D contains only the given VecBuildingBlockSpecification2D elements and nothing else in whatever order.
* @param refBuildingBlockSpecification2D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification2D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification2D does not contain all given VecBuildingBlockSpecification2D elements.
*/
public S hasOnlyRefBuildingBlockSpecification2D(VecBuildingBlockSpecification2D... refBuildingBlockSpecification2D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification2D varargs is not null.
if (refBuildingBlockSpecification2D == null) failWithMessage("Expecting refBuildingBlockSpecification2D parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBuildingBlockSpecification2D(), refBuildingBlockSpecification2D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification2D contains only the given VecBuildingBlockSpecification2D elements in Collection and nothing else in whatever order.
* @param refBuildingBlockSpecification2D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification2D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification2D does not contain all given VecBuildingBlockSpecification2D elements.
*/
public S hasOnlyRefBuildingBlockSpecification2D(java.util.Collection extends VecBuildingBlockSpecification2D> refBuildingBlockSpecification2D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification2D collection is not null.
if (refBuildingBlockSpecification2D == null) {
failWithMessage("Expecting refBuildingBlockSpecification2D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBuildingBlockSpecification2D(), refBuildingBlockSpecification2D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification2D does not contain the given VecBuildingBlockSpecification2D elements.
*
* @param refBuildingBlockSpecification2D the given elements that should not be in actual VecUnit's refBuildingBlockSpecification2D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification2D contains any given VecBuildingBlockSpecification2D elements.
*/
public S doesNotHaveRefBuildingBlockSpecification2D(VecBuildingBlockSpecification2D... refBuildingBlockSpecification2D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification2D varargs is not null.
if (refBuildingBlockSpecification2D == null) failWithMessage("Expecting refBuildingBlockSpecification2D parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBuildingBlockSpecification2D(), refBuildingBlockSpecification2D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification2D does not contain the given VecBuildingBlockSpecification2D elements in Collection.
*
* @param refBuildingBlockSpecification2D the given elements that should not be in actual VecUnit's refBuildingBlockSpecification2D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification2D contains any given VecBuildingBlockSpecification2D elements.
*/
public S doesNotHaveRefBuildingBlockSpecification2D(java.util.Collection extends VecBuildingBlockSpecification2D> refBuildingBlockSpecification2D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification2D collection is not null.
if (refBuildingBlockSpecification2D == null) {
failWithMessage("Expecting refBuildingBlockSpecification2D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBuildingBlockSpecification2D(), refBuildingBlockSpecification2D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit has no refBuildingBlockSpecification2D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification2D is not empty.
*/
public S hasNoRefBuildingBlockSpecification2D() {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refBuildingBlockSpecification2D but had :\n <%s>";
// check
if (actual.getRefBuildingBlockSpecification2D().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefBuildingBlockSpecification2D());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification3D contains the given VecBuildingBlockSpecification3D elements.
* @param refBuildingBlockSpecification3D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification3D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification3D does not contain all given VecBuildingBlockSpecification3D elements.
*/
public S hasRefBuildingBlockSpecification3D(VecBuildingBlockSpecification3D... refBuildingBlockSpecification3D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification3D varargs is not null.
if (refBuildingBlockSpecification3D == null) failWithMessage("Expecting refBuildingBlockSpecification3D parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBuildingBlockSpecification3D(), refBuildingBlockSpecification3D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification3D contains the given VecBuildingBlockSpecification3D elements in Collection.
* @param refBuildingBlockSpecification3D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification3D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification3D does not contain all given VecBuildingBlockSpecification3D elements.
*/
public S hasRefBuildingBlockSpecification3D(java.util.Collection extends VecBuildingBlockSpecification3D> refBuildingBlockSpecification3D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification3D collection is not null.
if (refBuildingBlockSpecification3D == null) {
failWithMessage("Expecting refBuildingBlockSpecification3D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBuildingBlockSpecification3D(), refBuildingBlockSpecification3D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification3D contains only the given VecBuildingBlockSpecification3D elements and nothing else in whatever order.
* @param refBuildingBlockSpecification3D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification3D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification3D does not contain all given VecBuildingBlockSpecification3D elements.
*/
public S hasOnlyRefBuildingBlockSpecification3D(VecBuildingBlockSpecification3D... refBuildingBlockSpecification3D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification3D varargs is not null.
if (refBuildingBlockSpecification3D == null) failWithMessage("Expecting refBuildingBlockSpecification3D parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBuildingBlockSpecification3D(), refBuildingBlockSpecification3D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification3D contains only the given VecBuildingBlockSpecification3D elements in Collection and nothing else in whatever order.
* @param refBuildingBlockSpecification3D the given elements that should be contained in actual VecUnit's refBuildingBlockSpecification3D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification3D does not contain all given VecBuildingBlockSpecification3D elements.
*/
public S hasOnlyRefBuildingBlockSpecification3D(java.util.Collection extends VecBuildingBlockSpecification3D> refBuildingBlockSpecification3D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification3D collection is not null.
if (refBuildingBlockSpecification3D == null) {
failWithMessage("Expecting refBuildingBlockSpecification3D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBuildingBlockSpecification3D(), refBuildingBlockSpecification3D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification3D does not contain the given VecBuildingBlockSpecification3D elements.
*
* @param refBuildingBlockSpecification3D the given elements that should not be in actual VecUnit's refBuildingBlockSpecification3D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification3D contains any given VecBuildingBlockSpecification3D elements.
*/
public S doesNotHaveRefBuildingBlockSpecification3D(VecBuildingBlockSpecification3D... refBuildingBlockSpecification3D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification3D varargs is not null.
if (refBuildingBlockSpecification3D == null) failWithMessage("Expecting refBuildingBlockSpecification3D parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBuildingBlockSpecification3D(), refBuildingBlockSpecification3D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refBuildingBlockSpecification3D does not contain the given VecBuildingBlockSpecification3D elements in Collection.
*
* @param refBuildingBlockSpecification3D the given elements that should not be in actual VecUnit's refBuildingBlockSpecification3D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification3D contains any given VecBuildingBlockSpecification3D elements.
*/
public S doesNotHaveRefBuildingBlockSpecification3D(java.util.Collection extends VecBuildingBlockSpecification3D> refBuildingBlockSpecification3D) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockSpecification3D collection is not null.
if (refBuildingBlockSpecification3D == null) {
failWithMessage("Expecting refBuildingBlockSpecification3D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBuildingBlockSpecification3D(), refBuildingBlockSpecification3D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit has no refBuildingBlockSpecification3D.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refBuildingBlockSpecification3D is not empty.
*/
public S hasNoRefBuildingBlockSpecification3D() {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refBuildingBlockSpecification3D but had :\n <%s>";
// check
if (actual.getRefBuildingBlockSpecification3D().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefBuildingBlockSpecification3D());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refCompositeUnit contains the given VecCompositeUnit elements.
* @param refCompositeUnit the given elements that should be contained in actual VecUnit's refCompositeUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refCompositeUnit does not contain all given VecCompositeUnit elements.
*/
public S hasRefCompositeUnit(VecCompositeUnit... refCompositeUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecCompositeUnit varargs is not null.
if (refCompositeUnit == null) failWithMessage("Expecting refCompositeUnit parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCompositeUnit(), refCompositeUnit);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refCompositeUnit contains the given VecCompositeUnit elements in Collection.
* @param refCompositeUnit the given elements that should be contained in actual VecUnit's refCompositeUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refCompositeUnit does not contain all given VecCompositeUnit elements.
*/
public S hasRefCompositeUnit(java.util.Collection extends VecCompositeUnit> refCompositeUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecCompositeUnit collection is not null.
if (refCompositeUnit == null) {
failWithMessage("Expecting refCompositeUnit parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCompositeUnit(), refCompositeUnit.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refCompositeUnit contains only the given VecCompositeUnit elements and nothing else in whatever order.
* @param refCompositeUnit the given elements that should be contained in actual VecUnit's refCompositeUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refCompositeUnit does not contain all given VecCompositeUnit elements.
*/
public S hasOnlyRefCompositeUnit(VecCompositeUnit... refCompositeUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecCompositeUnit varargs is not null.
if (refCompositeUnit == null) failWithMessage("Expecting refCompositeUnit parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCompositeUnit(), refCompositeUnit);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refCompositeUnit contains only the given VecCompositeUnit elements in Collection and nothing else in whatever order.
* @param refCompositeUnit the given elements that should be contained in actual VecUnit's refCompositeUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refCompositeUnit does not contain all given VecCompositeUnit elements.
*/
public S hasOnlyRefCompositeUnit(java.util.Collection extends VecCompositeUnit> refCompositeUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecCompositeUnit collection is not null.
if (refCompositeUnit == null) {
failWithMessage("Expecting refCompositeUnit parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCompositeUnit(), refCompositeUnit.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refCompositeUnit does not contain the given VecCompositeUnit elements.
*
* @param refCompositeUnit the given elements that should not be in actual VecUnit's refCompositeUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refCompositeUnit contains any given VecCompositeUnit elements.
*/
public S doesNotHaveRefCompositeUnit(VecCompositeUnit... refCompositeUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecCompositeUnit varargs is not null.
if (refCompositeUnit == null) failWithMessage("Expecting refCompositeUnit parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCompositeUnit(), refCompositeUnit);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refCompositeUnit does not contain the given VecCompositeUnit elements in Collection.
*
* @param refCompositeUnit the given elements that should not be in actual VecUnit's refCompositeUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refCompositeUnit contains any given VecCompositeUnit elements.
*/
public S doesNotHaveRefCompositeUnit(java.util.Collection extends VecCompositeUnit> refCompositeUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecCompositeUnit collection is not null.
if (refCompositeUnit == null) {
failWithMessage("Expecting refCompositeUnit parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCompositeUnit(), refCompositeUnit.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit has no refCompositeUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refCompositeUnit is not empty.
*/
public S hasNoRefCompositeUnit() {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCompositeUnit but had :\n <%s>";
// check
if (actual.getRefCompositeUnit().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCompositeUnit());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refDimension contains the given VecDimension elements.
* @param refDimension the given elements that should be contained in actual VecUnit's refDimension.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refDimension does not contain all given VecDimension elements.
*/
public S hasRefDimension(VecDimension... refDimension) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecDimension varargs is not null.
if (refDimension == null) failWithMessage("Expecting refDimension parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDimension(), refDimension);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refDimension contains the given VecDimension elements in Collection.
* @param refDimension the given elements that should be contained in actual VecUnit's refDimension.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refDimension does not contain all given VecDimension elements.
*/
public S hasRefDimension(java.util.Collection extends VecDimension> refDimension) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecDimension collection is not null.
if (refDimension == null) {
failWithMessage("Expecting refDimension parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefDimension(), refDimension.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refDimension contains only the given VecDimension elements and nothing else in whatever order.
* @param refDimension the given elements that should be contained in actual VecUnit's refDimension.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refDimension does not contain all given VecDimension elements.
*/
public S hasOnlyRefDimension(VecDimension... refDimension) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecDimension varargs is not null.
if (refDimension == null) failWithMessage("Expecting refDimension parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDimension(), refDimension);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refDimension contains only the given VecDimension elements in Collection and nothing else in whatever order.
* @param refDimension the given elements that should be contained in actual VecUnit's refDimension.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refDimension does not contain all given VecDimension elements.
*/
public S hasOnlyRefDimension(java.util.Collection extends VecDimension> refDimension) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecDimension collection is not null.
if (refDimension == null) {
failWithMessage("Expecting refDimension parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefDimension(), refDimension.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refDimension does not contain the given VecDimension elements.
*
* @param refDimension the given elements that should not be in actual VecUnit's refDimension.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refDimension contains any given VecDimension elements.
*/
public S doesNotHaveRefDimension(VecDimension... refDimension) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecDimension varargs is not null.
if (refDimension == null) failWithMessage("Expecting refDimension parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDimension(), refDimension);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refDimension does not contain the given VecDimension elements in Collection.
*
* @param refDimension the given elements that should not be in actual VecUnit's refDimension.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refDimension contains any given VecDimension elements.
*/
public S doesNotHaveRefDimension(java.util.Collection extends VecDimension> refDimension) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecDimension collection is not null.
if (refDimension == null) {
failWithMessage("Expecting refDimension parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefDimension(), refDimension.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit has no refDimension.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refDimension is not empty.
*/
public S hasNoRefDimension() {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refDimension but had :\n <%s>";
// check
if (actual.getRefDimension().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefDimension());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refLocalGeometrySpecification contains the given VecLocalGeometrySpecification elements.
* @param refLocalGeometrySpecification the given elements that should be contained in actual VecUnit's refLocalGeometrySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refLocalGeometrySpecification does not contain all given VecLocalGeometrySpecification elements.
*/
public S hasRefLocalGeometrySpecification(VecLocalGeometrySpecification... refLocalGeometrySpecification) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecLocalGeometrySpecification varargs is not null.
if (refLocalGeometrySpecification == null) failWithMessage("Expecting refLocalGeometrySpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefLocalGeometrySpecification(), refLocalGeometrySpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refLocalGeometrySpecification contains the given VecLocalGeometrySpecification elements in Collection.
* @param refLocalGeometrySpecification the given elements that should be contained in actual VecUnit's refLocalGeometrySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refLocalGeometrySpecification does not contain all given VecLocalGeometrySpecification elements.
*/
public S hasRefLocalGeometrySpecification(java.util.Collection extends VecLocalGeometrySpecification> refLocalGeometrySpecification) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecLocalGeometrySpecification collection is not null.
if (refLocalGeometrySpecification == null) {
failWithMessage("Expecting refLocalGeometrySpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefLocalGeometrySpecification(), refLocalGeometrySpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refLocalGeometrySpecification contains only the given VecLocalGeometrySpecification elements and nothing else in whatever order.
* @param refLocalGeometrySpecification the given elements that should be contained in actual VecUnit's refLocalGeometrySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refLocalGeometrySpecification does not contain all given VecLocalGeometrySpecification elements.
*/
public S hasOnlyRefLocalGeometrySpecification(VecLocalGeometrySpecification... refLocalGeometrySpecification) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecLocalGeometrySpecification varargs is not null.
if (refLocalGeometrySpecification == null) failWithMessage("Expecting refLocalGeometrySpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefLocalGeometrySpecification(), refLocalGeometrySpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refLocalGeometrySpecification contains only the given VecLocalGeometrySpecification elements in Collection and nothing else in whatever order.
* @param refLocalGeometrySpecification the given elements that should be contained in actual VecUnit's refLocalGeometrySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refLocalGeometrySpecification does not contain all given VecLocalGeometrySpecification elements.
*/
public S hasOnlyRefLocalGeometrySpecification(java.util.Collection extends VecLocalGeometrySpecification> refLocalGeometrySpecification) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecLocalGeometrySpecification collection is not null.
if (refLocalGeometrySpecification == null) {
failWithMessage("Expecting refLocalGeometrySpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefLocalGeometrySpecification(), refLocalGeometrySpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refLocalGeometrySpecification does not contain the given VecLocalGeometrySpecification elements.
*
* @param refLocalGeometrySpecification the given elements that should not be in actual VecUnit's refLocalGeometrySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refLocalGeometrySpecification contains any given VecLocalGeometrySpecification elements.
*/
public S doesNotHaveRefLocalGeometrySpecification(VecLocalGeometrySpecification... refLocalGeometrySpecification) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecLocalGeometrySpecification varargs is not null.
if (refLocalGeometrySpecification == null) failWithMessage("Expecting refLocalGeometrySpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefLocalGeometrySpecification(), refLocalGeometrySpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refLocalGeometrySpecification does not contain the given VecLocalGeometrySpecification elements in Collection.
*
* @param refLocalGeometrySpecification the given elements that should not be in actual VecUnit's refLocalGeometrySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refLocalGeometrySpecification contains any given VecLocalGeometrySpecification elements.
*/
public S doesNotHaveRefLocalGeometrySpecification(java.util.Collection extends VecLocalGeometrySpecification> refLocalGeometrySpecification) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecLocalGeometrySpecification collection is not null.
if (refLocalGeometrySpecification == null) {
failWithMessage("Expecting refLocalGeometrySpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefLocalGeometrySpecification(), refLocalGeometrySpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit has no refLocalGeometrySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refLocalGeometrySpecification is not empty.
*/
public S hasNoRefLocalGeometrySpecification() {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refLocalGeometrySpecification but had :\n <%s>";
// check
if (actual.getRefLocalGeometrySpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefLocalGeometrySpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refValueWithUnit contains the given VecValueWithUnit elements.
* @param refValueWithUnit the given elements that should be contained in actual VecUnit's refValueWithUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refValueWithUnit does not contain all given VecValueWithUnit elements.
*/
public S hasRefValueWithUnit(VecValueWithUnit... refValueWithUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecValueWithUnit varargs is not null.
if (refValueWithUnit == null) failWithMessage("Expecting refValueWithUnit parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefValueWithUnit(), refValueWithUnit);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refValueWithUnit contains the given VecValueWithUnit elements in Collection.
* @param refValueWithUnit the given elements that should be contained in actual VecUnit's refValueWithUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refValueWithUnit does not contain all given VecValueWithUnit elements.
*/
public S hasRefValueWithUnit(java.util.Collection extends VecValueWithUnit> refValueWithUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecValueWithUnit collection is not null.
if (refValueWithUnit == null) {
failWithMessage("Expecting refValueWithUnit parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefValueWithUnit(), refValueWithUnit.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refValueWithUnit contains only the given VecValueWithUnit elements and nothing else in whatever order.
* @param refValueWithUnit the given elements that should be contained in actual VecUnit's refValueWithUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refValueWithUnit does not contain all given VecValueWithUnit elements.
*/
public S hasOnlyRefValueWithUnit(VecValueWithUnit... refValueWithUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecValueWithUnit varargs is not null.
if (refValueWithUnit == null) failWithMessage("Expecting refValueWithUnit parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefValueWithUnit(), refValueWithUnit);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refValueWithUnit contains only the given VecValueWithUnit elements in Collection and nothing else in whatever order.
* @param refValueWithUnit the given elements that should be contained in actual VecUnit's refValueWithUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refValueWithUnit does not contain all given VecValueWithUnit elements.
*/
public S hasOnlyRefValueWithUnit(java.util.Collection extends VecValueWithUnit> refValueWithUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecValueWithUnit collection is not null.
if (refValueWithUnit == null) {
failWithMessage("Expecting refValueWithUnit parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefValueWithUnit(), refValueWithUnit.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refValueWithUnit does not contain the given VecValueWithUnit elements.
*
* @param refValueWithUnit the given elements that should not be in actual VecUnit's refValueWithUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refValueWithUnit contains any given VecValueWithUnit elements.
*/
public S doesNotHaveRefValueWithUnit(VecValueWithUnit... refValueWithUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecValueWithUnit varargs is not null.
if (refValueWithUnit == null) failWithMessage("Expecting refValueWithUnit parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefValueWithUnit(), refValueWithUnit);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's refValueWithUnit does not contain the given VecValueWithUnit elements in Collection.
*
* @param refValueWithUnit the given elements that should not be in actual VecUnit's refValueWithUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refValueWithUnit contains any given VecValueWithUnit elements.
*/
public S doesNotHaveRefValueWithUnit(java.util.Collection extends VecValueWithUnit> refValueWithUnit) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// check that given VecValueWithUnit collection is not null.
if (refValueWithUnit == null) {
failWithMessage("Expecting refValueWithUnit parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefValueWithUnit(), refValueWithUnit.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit has no refValueWithUnit.
* @return this assertion object.
* @throws AssertionError if the actual VecUnit's refValueWithUnit is not empty.
*/
public S hasNoRefValueWithUnit() {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refValueWithUnit but had :\n <%s>";
// check
if (actual.getRefValueWithUnit().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefValueWithUnit());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUnit's xmlId is equal to the given one.
* @param xmlId the given xmlId to compare the actual VecUnit's xmlId to.
* @return this assertion object.
* @throws AssertionError - if the actual VecUnit's xmlId is not equal to the given one.
*/
public S hasXmlId(String xmlId) {
// check that actual VecUnit we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting xmlId of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualXmlId = actual.getXmlId();
if (!Objects.areEqual(actualXmlId, xmlId)) {
failWithMessage(assertjErrorMessage, actual, xmlId, actualXmlId);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy