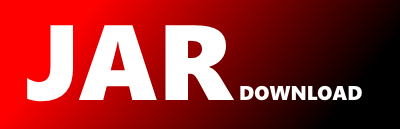
com.foursoft.vecmodel.vec120.AbstractVecUsageNodeAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecUsageNode} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecUsageNodeAssert, A extends VecUsageNode> extends AbstractVecConfigurableElementAssert {
/**
* Creates a new {@link AbstractVecUsageNodeAssert}
to make assertions on actual VecUsageNode.
* @param actual the VecUsageNode we want to make assertions on.
*/
protected AbstractVecUsageNodeAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecUsageNode's abbreviations contains the given VecLocalizedString elements.
* @param abbreviations the given elements that should be contained in actual VecUsageNode's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasAbbreviations(VecLocalizedString... abbreviations) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (abbreviations == null) failWithMessage("Expecting abbreviations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAbbreviations(), abbreviations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's abbreviations contains the given VecLocalizedString elements in Collection.
* @param abbreviations the given elements that should be contained in actual VecUsageNode's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasAbbreviations(java.util.Collection extends VecLocalizedString> abbreviations) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (abbreviations == null) {
failWithMessage("Expecting abbreviations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAbbreviations(), abbreviations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's abbreviations contains only the given VecLocalizedString elements and nothing else in whatever order.
* @param abbreviations the given elements that should be contained in actual VecUsageNode's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasOnlyAbbreviations(VecLocalizedString... abbreviations) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (abbreviations == null) failWithMessage("Expecting abbreviations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAbbreviations(), abbreviations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's abbreviations contains only the given VecLocalizedString elements in Collection and nothing else in whatever order.
* @param abbreviations the given elements that should be contained in actual VecUsageNode's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's abbreviations does not contain all given VecLocalizedString elements.
*/
public S hasOnlyAbbreviations(java.util.Collection extends VecLocalizedString> abbreviations) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (abbreviations == null) {
failWithMessage("Expecting abbreviations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAbbreviations(), abbreviations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's abbreviations does not contain the given VecLocalizedString elements.
*
* @param abbreviations the given elements that should not be in actual VecUsageNode's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's abbreviations contains any given VecLocalizedString elements.
*/
public S doesNotHaveAbbreviations(VecLocalizedString... abbreviations) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString varargs is not null.
if (abbreviations == null) failWithMessage("Expecting abbreviations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAbbreviations(), abbreviations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's abbreviations does not contain the given VecLocalizedString elements in Collection.
*
* @param abbreviations the given elements that should not be in actual VecUsageNode's abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's abbreviations contains any given VecLocalizedString elements.
*/
public S doesNotHaveAbbreviations(java.util.Collection extends VecLocalizedString> abbreviations) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecLocalizedString collection is not null.
if (abbreviations == null) {
failWithMessage("Expecting abbreviations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAbbreviations(), abbreviations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no abbreviations.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's abbreviations is not empty.
*/
public S hasNoAbbreviations() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have abbreviations but had :\n <%s>";
// check
if (actual.getAbbreviations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAbbreviations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's descriptions contains the given VecAbstractLocalizedString elements.
* @param descriptions the given elements that should be contained in actual VecUsageNode's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's descriptions contains the given VecAbstractLocalizedString elements in Collection.
* @param descriptions the given elements that should be contained in actual VecUsageNode's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's descriptions contains only the given VecAbstractLocalizedString elements and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecUsageNode's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's descriptions contains only the given VecAbstractLocalizedString elements in Collection and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecUsageNode's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's descriptions does not contain the given VecAbstractLocalizedString elements.
*
* @param descriptions the given elements that should not be in actual VecUsageNode's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's descriptions does not contain the given VecAbstractLocalizedString elements in Collection.
*
* @param descriptions the given elements that should not be in actual VecUsageNode's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's descriptions is not empty.
*/
public S hasNoDescriptions() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have descriptions but had :\n <%s>";
// check
if (actual.getDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's identification is equal to the given one.
* @param identification the given identification to compare the actual VecUsageNode's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecUsageNode's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's parentUsageNode is equal to the given one.
* @param parentUsageNode the given parentUsageNode to compare the actual VecUsageNode's parentUsageNode to.
* @return this assertion object.
* @throws AssertionError - if the actual VecUsageNode's parentUsageNode is not equal to the given one.
*/
public S hasParentUsageNode(VecUsageNode parentUsageNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentUsageNode of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecUsageNode actualParentUsageNode = actual.getParentUsageNode();
if (!Objects.areEqual(actualParentUsageNode, parentUsageNode)) {
failWithMessage(assertjErrorMessage, actual, parentUsageNode, actualParentUsageNode);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's parentUsageNodeSpecification is equal to the given one.
* @param parentUsageNodeSpecification the given parentUsageNodeSpecification to compare the actual VecUsageNode's parentUsageNodeSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecUsageNode's parentUsageNodeSpecification is not equal to the given one.
*/
public S hasParentUsageNodeSpecification(VecUsageNodeSpecification parentUsageNodeSpecification) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentUsageNodeSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecUsageNodeSpecification actualParentUsageNodeSpecification = actual.getParentUsageNodeSpecification();
if (!Objects.areEqual(actualParentUsageNodeSpecification, parentUsageNodeSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentUsageNodeSpecification, actualParentUsageNodeSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refComponentNode contains the given VecComponentNode elements.
* @param refComponentNode the given elements that should be contained in actual VecUsageNode's refComponentNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refComponentNode does not contain all given VecComponentNode elements.
*/
public S hasRefComponentNode(VecComponentNode... refComponentNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode varargs is not null.
if (refComponentNode == null) failWithMessage("Expecting refComponentNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefComponentNode(), refComponentNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refComponentNode contains the given VecComponentNode elements in Collection.
* @param refComponentNode the given elements that should be contained in actual VecUsageNode's refComponentNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refComponentNode does not contain all given VecComponentNode elements.
*/
public S hasRefComponentNode(java.util.Collection extends VecComponentNode> refComponentNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode collection is not null.
if (refComponentNode == null) {
failWithMessage("Expecting refComponentNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefComponentNode(), refComponentNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refComponentNode contains only the given VecComponentNode elements and nothing else in whatever order.
* @param refComponentNode the given elements that should be contained in actual VecUsageNode's refComponentNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refComponentNode does not contain all given VecComponentNode elements.
*/
public S hasOnlyRefComponentNode(VecComponentNode... refComponentNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode varargs is not null.
if (refComponentNode == null) failWithMessage("Expecting refComponentNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefComponentNode(), refComponentNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refComponentNode contains only the given VecComponentNode elements in Collection and nothing else in whatever order.
* @param refComponentNode the given elements that should be contained in actual VecUsageNode's refComponentNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refComponentNode does not contain all given VecComponentNode elements.
*/
public S hasOnlyRefComponentNode(java.util.Collection extends VecComponentNode> refComponentNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode collection is not null.
if (refComponentNode == null) {
failWithMessage("Expecting refComponentNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefComponentNode(), refComponentNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refComponentNode does not contain the given VecComponentNode elements.
*
* @param refComponentNode the given elements that should not be in actual VecUsageNode's refComponentNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refComponentNode contains any given VecComponentNode elements.
*/
public S doesNotHaveRefComponentNode(VecComponentNode... refComponentNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode varargs is not null.
if (refComponentNode == null) failWithMessage("Expecting refComponentNode parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefComponentNode(), refComponentNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refComponentNode does not contain the given VecComponentNode elements in Collection.
*
* @param refComponentNode the given elements that should not be in actual VecUsageNode's refComponentNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refComponentNode contains any given VecComponentNode elements.
*/
public S doesNotHaveRefComponentNode(java.util.Collection extends VecComponentNode> refComponentNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecComponentNode collection is not null.
if (refComponentNode == null) {
failWithMessage("Expecting refComponentNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefComponentNode(), refComponentNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no refComponentNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refComponentNode is not empty.
*/
public S hasNoRefComponentNode() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refComponentNode but had :\n <%s>";
// check
if (actual.getRefComponentNode().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefComponentNode());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refNetworkNode contains the given VecNetworkNode elements.
* @param refNetworkNode the given elements that should be contained in actual VecUsageNode's refNetworkNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refNetworkNode does not contain all given VecNetworkNode elements.
*/
public S hasRefNetworkNode(VecNetworkNode... refNetworkNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode varargs is not null.
if (refNetworkNode == null) failWithMessage("Expecting refNetworkNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefNetworkNode(), refNetworkNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refNetworkNode contains the given VecNetworkNode elements in Collection.
* @param refNetworkNode the given elements that should be contained in actual VecUsageNode's refNetworkNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refNetworkNode does not contain all given VecNetworkNode elements.
*/
public S hasRefNetworkNode(java.util.Collection extends VecNetworkNode> refNetworkNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode collection is not null.
if (refNetworkNode == null) {
failWithMessage("Expecting refNetworkNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefNetworkNode(), refNetworkNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refNetworkNode contains only the given VecNetworkNode elements and nothing else in whatever order.
* @param refNetworkNode the given elements that should be contained in actual VecUsageNode's refNetworkNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refNetworkNode does not contain all given VecNetworkNode elements.
*/
public S hasOnlyRefNetworkNode(VecNetworkNode... refNetworkNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode varargs is not null.
if (refNetworkNode == null) failWithMessage("Expecting refNetworkNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefNetworkNode(), refNetworkNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refNetworkNode contains only the given VecNetworkNode elements in Collection and nothing else in whatever order.
* @param refNetworkNode the given elements that should be contained in actual VecUsageNode's refNetworkNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refNetworkNode does not contain all given VecNetworkNode elements.
*/
public S hasOnlyRefNetworkNode(java.util.Collection extends VecNetworkNode> refNetworkNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode collection is not null.
if (refNetworkNode == null) {
failWithMessage("Expecting refNetworkNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefNetworkNode(), refNetworkNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refNetworkNode does not contain the given VecNetworkNode elements.
*
* @param refNetworkNode the given elements that should not be in actual VecUsageNode's refNetworkNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refNetworkNode contains any given VecNetworkNode elements.
*/
public S doesNotHaveRefNetworkNode(VecNetworkNode... refNetworkNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode varargs is not null.
if (refNetworkNode == null) failWithMessage("Expecting refNetworkNode parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefNetworkNode(), refNetworkNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refNetworkNode does not contain the given VecNetworkNode elements in Collection.
*
* @param refNetworkNode the given elements that should not be in actual VecUsageNode's refNetworkNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refNetworkNode contains any given VecNetworkNode elements.
*/
public S doesNotHaveRefNetworkNode(java.util.Collection extends VecNetworkNode> refNetworkNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecNetworkNode collection is not null.
if (refNetworkNode == null) {
failWithMessage("Expecting refNetworkNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefNetworkNode(), refNetworkNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no refNetworkNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refNetworkNode is not empty.
*/
public S hasNoRefNetworkNode() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refNetworkNode but had :\n <%s>";
// check
if (actual.getRefNetworkNode().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefNetworkNode());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refOccurrenceOrUsage contains the given VecOccurrenceOrUsage elements.
* @param refOccurrenceOrUsage the given elements that should be contained in actual VecUsageNode's refOccurrenceOrUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refOccurrenceOrUsage does not contain all given VecOccurrenceOrUsage elements.
*/
public S hasRefOccurrenceOrUsage(VecOccurrenceOrUsage... refOccurrenceOrUsage) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsage varargs is not null.
if (refOccurrenceOrUsage == null) failWithMessage("Expecting refOccurrenceOrUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefOccurrenceOrUsage(), refOccurrenceOrUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refOccurrenceOrUsage contains the given VecOccurrenceOrUsage elements in Collection.
* @param refOccurrenceOrUsage the given elements that should be contained in actual VecUsageNode's refOccurrenceOrUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refOccurrenceOrUsage does not contain all given VecOccurrenceOrUsage elements.
*/
public S hasRefOccurrenceOrUsage(java.util.Collection extends VecOccurrenceOrUsage> refOccurrenceOrUsage) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsage collection is not null.
if (refOccurrenceOrUsage == null) {
failWithMessage("Expecting refOccurrenceOrUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefOccurrenceOrUsage(), refOccurrenceOrUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refOccurrenceOrUsage contains only the given VecOccurrenceOrUsage elements and nothing else in whatever order.
* @param refOccurrenceOrUsage the given elements that should be contained in actual VecUsageNode's refOccurrenceOrUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refOccurrenceOrUsage does not contain all given VecOccurrenceOrUsage elements.
*/
public S hasOnlyRefOccurrenceOrUsage(VecOccurrenceOrUsage... refOccurrenceOrUsage) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsage varargs is not null.
if (refOccurrenceOrUsage == null) failWithMessage("Expecting refOccurrenceOrUsage parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefOccurrenceOrUsage(), refOccurrenceOrUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refOccurrenceOrUsage contains only the given VecOccurrenceOrUsage elements in Collection and nothing else in whatever order.
* @param refOccurrenceOrUsage the given elements that should be contained in actual VecUsageNode's refOccurrenceOrUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refOccurrenceOrUsage does not contain all given VecOccurrenceOrUsage elements.
*/
public S hasOnlyRefOccurrenceOrUsage(java.util.Collection extends VecOccurrenceOrUsage> refOccurrenceOrUsage) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsage collection is not null.
if (refOccurrenceOrUsage == null) {
failWithMessage("Expecting refOccurrenceOrUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefOccurrenceOrUsage(), refOccurrenceOrUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refOccurrenceOrUsage does not contain the given VecOccurrenceOrUsage elements.
*
* @param refOccurrenceOrUsage the given elements that should not be in actual VecUsageNode's refOccurrenceOrUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refOccurrenceOrUsage contains any given VecOccurrenceOrUsage elements.
*/
public S doesNotHaveRefOccurrenceOrUsage(VecOccurrenceOrUsage... refOccurrenceOrUsage) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsage varargs is not null.
if (refOccurrenceOrUsage == null) failWithMessage("Expecting refOccurrenceOrUsage parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefOccurrenceOrUsage(), refOccurrenceOrUsage);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refOccurrenceOrUsage does not contain the given VecOccurrenceOrUsage elements in Collection.
*
* @param refOccurrenceOrUsage the given elements that should not be in actual VecUsageNode's refOccurrenceOrUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refOccurrenceOrUsage contains any given VecOccurrenceOrUsage elements.
*/
public S doesNotHaveRefOccurrenceOrUsage(java.util.Collection extends VecOccurrenceOrUsage> refOccurrenceOrUsage) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsage collection is not null.
if (refOccurrenceOrUsage == null) {
failWithMessage("Expecting refOccurrenceOrUsage parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefOccurrenceOrUsage(), refOccurrenceOrUsage.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no refOccurrenceOrUsage.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refOccurrenceOrUsage is not empty.
*/
public S hasNoRefOccurrenceOrUsage() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refOccurrenceOrUsage but had :\n <%s>";
// check
if (actual.getRefOccurrenceOrUsage().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefOccurrenceOrUsage());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refTopologyNode contains the given VecTopologyNode elements.
* @param refTopologyNode the given elements that should be contained in actual VecUsageNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasRefTopologyNode(VecTopologyNode... refTopologyNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode varargs is not null.
if (refTopologyNode == null) failWithMessage("Expecting refTopologyNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologyNode(), refTopologyNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refTopologyNode contains the given VecTopologyNode elements in Collection.
* @param refTopologyNode the given elements that should be contained in actual VecUsageNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasRefTopologyNode(java.util.Collection extends VecTopologyNode> refTopologyNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode collection is not null.
if (refTopologyNode == null) {
failWithMessage("Expecting refTopologyNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTopologyNode(), refTopologyNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refTopologyNode contains only the given VecTopologyNode elements and nothing else in whatever order.
* @param refTopologyNode the given elements that should be contained in actual VecUsageNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasOnlyRefTopologyNode(VecTopologyNode... refTopologyNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode varargs is not null.
if (refTopologyNode == null) failWithMessage("Expecting refTopologyNode parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologyNode(), refTopologyNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refTopologyNode contains only the given VecTopologyNode elements in Collection and nothing else in whatever order.
* @param refTopologyNode the given elements that should be contained in actual VecUsageNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refTopologyNode does not contain all given VecTopologyNode elements.
*/
public S hasOnlyRefTopologyNode(java.util.Collection extends VecTopologyNode> refTopologyNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode collection is not null.
if (refTopologyNode == null) {
failWithMessage("Expecting refTopologyNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTopologyNode(), refTopologyNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refTopologyNode does not contain the given VecTopologyNode elements.
*
* @param refTopologyNode the given elements that should not be in actual VecUsageNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refTopologyNode contains any given VecTopologyNode elements.
*/
public S doesNotHaveRefTopologyNode(VecTopologyNode... refTopologyNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode varargs is not null.
if (refTopologyNode == null) failWithMessage("Expecting refTopologyNode parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologyNode(), refTopologyNode);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refTopologyNode does not contain the given VecTopologyNode elements in Collection.
*
* @param refTopologyNode the given elements that should not be in actual VecUsageNode's refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refTopologyNode contains any given VecTopologyNode elements.
*/
public S doesNotHaveRefTopologyNode(java.util.Collection extends VecTopologyNode> refTopologyNode) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecTopologyNode collection is not null.
if (refTopologyNode == null) {
failWithMessage("Expecting refTopologyNode parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTopologyNode(), refTopologyNode.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no refTopologyNode.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refTopologyNode is not empty.
*/
public S hasNoRefTopologyNode() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTopologyNode but had :\n <%s>";
// check
if (actual.getRefTopologyNode().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTopologyNode());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refUsageConstraint contains the given VecUsageConstraint elements.
* @param refUsageConstraint the given elements that should be contained in actual VecUsageNode's refUsageConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refUsageConstraint does not contain all given VecUsageConstraint elements.
*/
public S hasRefUsageConstraint(VecUsageConstraint... refUsageConstraint) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraint varargs is not null.
if (refUsageConstraint == null) failWithMessage("Expecting refUsageConstraint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefUsageConstraint(), refUsageConstraint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refUsageConstraint contains the given VecUsageConstraint elements in Collection.
* @param refUsageConstraint the given elements that should be contained in actual VecUsageNode's refUsageConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refUsageConstraint does not contain all given VecUsageConstraint elements.
*/
public S hasRefUsageConstraint(java.util.Collection extends VecUsageConstraint> refUsageConstraint) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraint collection is not null.
if (refUsageConstraint == null) {
failWithMessage("Expecting refUsageConstraint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefUsageConstraint(), refUsageConstraint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refUsageConstraint contains only the given VecUsageConstraint elements and nothing else in whatever order.
* @param refUsageConstraint the given elements that should be contained in actual VecUsageNode's refUsageConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refUsageConstraint does not contain all given VecUsageConstraint elements.
*/
public S hasOnlyRefUsageConstraint(VecUsageConstraint... refUsageConstraint) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraint varargs is not null.
if (refUsageConstraint == null) failWithMessage("Expecting refUsageConstraint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefUsageConstraint(), refUsageConstraint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refUsageConstraint contains only the given VecUsageConstraint elements in Collection and nothing else in whatever order.
* @param refUsageConstraint the given elements that should be contained in actual VecUsageNode's refUsageConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refUsageConstraint does not contain all given VecUsageConstraint elements.
*/
public S hasOnlyRefUsageConstraint(java.util.Collection extends VecUsageConstraint> refUsageConstraint) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraint collection is not null.
if (refUsageConstraint == null) {
failWithMessage("Expecting refUsageConstraint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefUsageConstraint(), refUsageConstraint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refUsageConstraint does not contain the given VecUsageConstraint elements.
*
* @param refUsageConstraint the given elements that should not be in actual VecUsageNode's refUsageConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refUsageConstraint contains any given VecUsageConstraint elements.
*/
public S doesNotHaveRefUsageConstraint(VecUsageConstraint... refUsageConstraint) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraint varargs is not null.
if (refUsageConstraint == null) failWithMessage("Expecting refUsageConstraint parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefUsageConstraint(), refUsageConstraint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's refUsageConstraint does not contain the given VecUsageConstraint elements in Collection.
*
* @param refUsageConstraint the given elements that should not be in actual VecUsageNode's refUsageConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refUsageConstraint contains any given VecUsageConstraint elements.
*/
public S doesNotHaveRefUsageConstraint(java.util.Collection extends VecUsageConstraint> refUsageConstraint) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageConstraint collection is not null.
if (refUsageConstraint == null) {
failWithMessage("Expecting refUsageConstraint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefUsageConstraint(), refUsageConstraint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no refUsageConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's refUsageConstraint is not empty.
*/
public S hasNoRefUsageConstraint() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refUsageConstraint but had :\n <%s>";
// check
if (actual.getRefUsageConstraint().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefUsageConstraint());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's subUsageNodes contains the given VecUsageNode elements.
* @param subUsageNodes the given elements that should be contained in actual VecUsageNode's subUsageNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's subUsageNodes does not contain all given VecUsageNode elements.
*/
public S hasSubUsageNodes(VecUsageNode... subUsageNodes) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageNode varargs is not null.
if (subUsageNodes == null) failWithMessage("Expecting subUsageNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSubUsageNodes(), subUsageNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's subUsageNodes contains the given VecUsageNode elements in Collection.
* @param subUsageNodes the given elements that should be contained in actual VecUsageNode's subUsageNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's subUsageNodes does not contain all given VecUsageNode elements.
*/
public S hasSubUsageNodes(java.util.Collection extends VecUsageNode> subUsageNodes) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageNode collection is not null.
if (subUsageNodes == null) {
failWithMessage("Expecting subUsageNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSubUsageNodes(), subUsageNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's subUsageNodes contains only the given VecUsageNode elements and nothing else in whatever order.
* @param subUsageNodes the given elements that should be contained in actual VecUsageNode's subUsageNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's subUsageNodes does not contain all given VecUsageNode elements.
*/
public S hasOnlySubUsageNodes(VecUsageNode... subUsageNodes) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageNode varargs is not null.
if (subUsageNodes == null) failWithMessage("Expecting subUsageNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSubUsageNodes(), subUsageNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's subUsageNodes contains only the given VecUsageNode elements in Collection and nothing else in whatever order.
* @param subUsageNodes the given elements that should be contained in actual VecUsageNode's subUsageNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's subUsageNodes does not contain all given VecUsageNode elements.
*/
public S hasOnlySubUsageNodes(java.util.Collection extends VecUsageNode> subUsageNodes) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageNode collection is not null.
if (subUsageNodes == null) {
failWithMessage("Expecting subUsageNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSubUsageNodes(), subUsageNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's subUsageNodes does not contain the given VecUsageNode elements.
*
* @param subUsageNodes the given elements that should not be in actual VecUsageNode's subUsageNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's subUsageNodes contains any given VecUsageNode elements.
*/
public S doesNotHaveSubUsageNodes(VecUsageNode... subUsageNodes) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageNode varargs is not null.
if (subUsageNodes == null) failWithMessage("Expecting subUsageNodes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSubUsageNodes(), subUsageNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's subUsageNodes does not contain the given VecUsageNode elements in Collection.
*
* @param subUsageNodes the given elements that should not be in actual VecUsageNode's subUsageNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's subUsageNodes contains any given VecUsageNode elements.
*/
public S doesNotHaveSubUsageNodes(java.util.Collection extends VecUsageNode> subUsageNodes) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecUsageNode collection is not null.
if (subUsageNodes == null) {
failWithMessage("Expecting subUsageNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSubUsageNodes(), subUsageNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no subUsageNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's subUsageNodes is not empty.
*/
public S hasNoSubUsageNodes() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have subUsageNodes but had :\n <%s>";
// check
if (actual.getSubUsageNodes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getSubUsageNodes());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's usageNodeType is equal to the given one.
* @param usageNodeType the given usageNodeType to compare the actual VecUsageNode's usageNodeType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecUsageNode's usageNodeType is not equal to the given one.
*/
public S hasUsageNodeType(String usageNodeType) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting usageNodeType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualUsageNodeType = actual.getUsageNodeType();
if (!Objects.areEqual(actualUsageNodeType, usageNodeType)) {
failWithMessage(assertjErrorMessage, actual, usageNodeType, actualUsageNodeType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's usedInProject contains the given VecProject elements.
* @param usedInProject the given elements that should be contained in actual VecUsageNode's usedInProject.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's usedInProject does not contain all given VecProject elements.
*/
public S hasUsedInProject(VecProject... usedInProject) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecProject varargs is not null.
if (usedInProject == null) failWithMessage("Expecting usedInProject parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedInProject(), usedInProject);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's usedInProject contains the given VecProject elements in Collection.
* @param usedInProject the given elements that should be contained in actual VecUsageNode's usedInProject.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's usedInProject does not contain all given VecProject elements.
*/
public S hasUsedInProject(java.util.Collection extends VecProject> usedInProject) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecProject collection is not null.
if (usedInProject == null) {
failWithMessage("Expecting usedInProject parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedInProject(), usedInProject.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's usedInProject contains only the given VecProject elements and nothing else in whatever order.
* @param usedInProject the given elements that should be contained in actual VecUsageNode's usedInProject.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's usedInProject does not contain all given VecProject elements.
*/
public S hasOnlyUsedInProject(VecProject... usedInProject) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecProject varargs is not null.
if (usedInProject == null) failWithMessage("Expecting usedInProject parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedInProject(), usedInProject);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's usedInProject contains only the given VecProject elements in Collection and nothing else in whatever order.
* @param usedInProject the given elements that should be contained in actual VecUsageNode's usedInProject.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's usedInProject does not contain all given VecProject elements.
*/
public S hasOnlyUsedInProject(java.util.Collection extends VecProject> usedInProject) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecProject collection is not null.
if (usedInProject == null) {
failWithMessage("Expecting usedInProject parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedInProject(), usedInProject.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's usedInProject does not contain the given VecProject elements.
*
* @param usedInProject the given elements that should not be in actual VecUsageNode's usedInProject.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's usedInProject contains any given VecProject elements.
*/
public S doesNotHaveUsedInProject(VecProject... usedInProject) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecProject varargs is not null.
if (usedInProject == null) failWithMessage("Expecting usedInProject parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedInProject(), usedInProject);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode's usedInProject does not contain the given VecProject elements in Collection.
*
* @param usedInProject the given elements that should not be in actual VecUsageNode's usedInProject.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's usedInProject contains any given VecProject elements.
*/
public S doesNotHaveUsedInProject(java.util.Collection extends VecProject> usedInProject) {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// check that given VecProject collection is not null.
if (usedInProject == null) {
failWithMessage("Expecting usedInProject parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedInProject(), usedInProject.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecUsageNode has no usedInProject.
* @return this assertion object.
* @throws AssertionError if the actual VecUsageNode's usedInProject is not empty.
*/
public S hasNoUsedInProject() {
// check that actual VecUsageNode we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have usedInProject but had :\n <%s>";
// check
if (actual.getUsedInProject().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getUsedInProject());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy