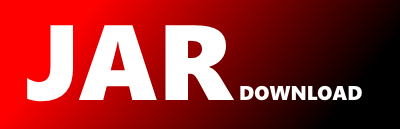
com.foursoft.vecmodel.vec120.AbstractVecValueRangeAssert Maven / Gradle / Ivy
Show all versions of vec120-assertions Show documentation
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.Assertions;
/**
* Abstract base class for {@link VecValueRange} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecValueRangeAssert, A extends VecValueRange> extends AbstractVecValueWithUnitAssert {
/**
* Creates a new {@link AbstractVecValueRangeAssert}
to make assertions on actual VecValueRange.
* @param actual the VecValueRange we want to make assertions on.
*/
protected AbstractVecValueRangeAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecValueRange's maximum is equal to the given one.
* @param maximum the given maximum to compare the actual VecValueRange's maximum to.
* @return this assertion object.
* @throws AssertionError - if the actual VecValueRange's maximum is not equal to the given one.
*/
public S hasMaximum(double maximum) {
// check that actual VecValueRange we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting maximum of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for maximum
double actualMaximum = actual.getMaximum();
if (actualMaximum != maximum) {
failWithMessage(assertjErrorMessage, actual, maximum, actualMaximum);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecValueRange's maximum is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param maximum the value to compare the actual VecValueRange's maximum to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecValueRange's maximum is not close enough to the given value.
*/
public S hasMaximumCloseTo(double maximum, double assertjOffset) {
// check that actual VecValueRange we want to make assertions on is not null.
isNotNull();
double actualMaximum = actual.getMaximum();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting maximum:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualMaximum, maximum, assertjOffset, Math.abs(maximum - actualMaximum));
// check
Assertions.assertThat(actualMaximum).overridingErrorMessage(assertjErrorMessage).isCloseTo(maximum, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecValueRange's minimum is equal to the given one.
* @param minimum the given minimum to compare the actual VecValueRange's minimum to.
* @return this assertion object.
* @throws AssertionError - if the actual VecValueRange's minimum is not equal to the given one.
*/
public S hasMinimum(double minimum) {
// check that actual VecValueRange we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minimum of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for minimum
double actualMinimum = actual.getMinimum();
if (actualMinimum != minimum) {
failWithMessage(assertjErrorMessage, actual, minimum, actualMinimum);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecValueRange's minimum is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param minimum the value to compare the actual VecValueRange's minimum to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecValueRange's minimum is not close enough to the given value.
*/
public S hasMinimumCloseTo(double minimum, double assertjOffset) {
// check that actual VecValueRange we want to make assertions on is not null.
isNotNull();
double actualMinimum = actual.getMinimum();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting minimum:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualMinimum, minimum, assertjOffset, Math.abs(minimum - actualMinimum));
// check
Assertions.assertThat(actualMinimum).overridingErrorMessage(assertjErrorMessage).isCloseTo(minimum, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
}