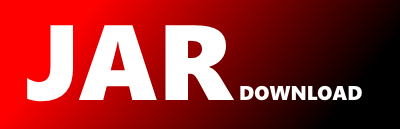
com.foursoft.vecmodel.vec120.AbstractVecWireElementReferenceAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecWireElementReference} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecWireElementReferenceAssert, A extends VecWireElementReference> extends AbstractVecRoutableElementAssert {
/**
* Creates a new {@link AbstractVecWireElementReferenceAssert}
to make assertions on actual VecWireElementReference.
* @param actual the VecWireElementReference we want to make assertions on.
*/
protected AbstractVecWireElementReferenceAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecWireElementReference's connection is equal to the given one.
* @param connection the given connection to compare the actual VecWireElementReference's connection to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference's connection is not equal to the given one.
*/
public S hasConnection(VecConnection connection) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting connection of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecConnection actualConnection = actual.getConnection();
if (!Objects.areEqual(actualConnection, connection)) {
failWithMessage(assertjErrorMessage, actual, connection, actualConnection);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's connectionGroup is equal to the given one.
* @param connectionGroup the given connectionGroup to compare the actual VecWireElementReference's connectionGroup to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference's connectionGroup is not equal to the given one.
*/
public S hasConnectionGroup(VecConnectionGroup connectionGroup) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting connectionGroup of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecConnectionGroup actualConnectionGroup = actual.getConnectionGroup();
if (!Objects.areEqual(actualConnectionGroup, connectionGroup)) {
failWithMessage(assertjErrorMessage, actual, connectionGroup, actualConnectionGroup);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's identification is equal to the given one.
* @param identification the given identification to compare the actual VecWireElementReference's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's parentWireRole is equal to the given one.
* @param parentWireRole the given parentWireRole to compare the actual VecWireElementReference's parentWireRole to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference's parentWireRole is not equal to the given one.
*/
public S hasParentWireRole(VecWireRole parentWireRole) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentWireRole of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecWireRole actualParentWireRole = actual.getParentWireRole();
if (!Objects.areEqual(actualParentWireRole, parentWireRole)) {
failWithMessage(assertjErrorMessage, actual, parentWireRole, actualParentWireRole);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's refWireGrouping contains the given VecWireGrouping elements.
* @param refWireGrouping the given elements that should be contained in actual VecWireElementReference's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasRefWireGrouping(VecWireGrouping... refWireGrouping) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping varargs is not null.
if (refWireGrouping == null) failWithMessage("Expecting refWireGrouping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireGrouping(), refWireGrouping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's refWireGrouping contains the given VecWireGrouping elements in Collection.
* @param refWireGrouping the given elements that should be contained in actual VecWireElementReference's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasRefWireGrouping(java.util.Collection extends VecWireGrouping> refWireGrouping) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping collection is not null.
if (refWireGrouping == null) {
failWithMessage("Expecting refWireGrouping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireGrouping(), refWireGrouping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's refWireGrouping contains only the given VecWireGrouping elements and nothing else in whatever order.
* @param refWireGrouping the given elements that should be contained in actual VecWireElementReference's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasOnlyRefWireGrouping(VecWireGrouping... refWireGrouping) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping varargs is not null.
if (refWireGrouping == null) failWithMessage("Expecting refWireGrouping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireGrouping(), refWireGrouping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's refWireGrouping contains only the given VecWireGrouping elements in Collection and nothing else in whatever order.
* @param refWireGrouping the given elements that should be contained in actual VecWireElementReference's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasOnlyRefWireGrouping(java.util.Collection extends VecWireGrouping> refWireGrouping) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping collection is not null.
if (refWireGrouping == null) {
failWithMessage("Expecting refWireGrouping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireGrouping(), refWireGrouping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's refWireGrouping does not contain the given VecWireGrouping elements.
*
* @param refWireGrouping the given elements that should not be in actual VecWireElementReference's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's refWireGrouping contains any given VecWireGrouping elements.
*/
public S doesNotHaveRefWireGrouping(VecWireGrouping... refWireGrouping) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping varargs is not null.
if (refWireGrouping == null) failWithMessage("Expecting refWireGrouping parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireGrouping(), refWireGrouping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's refWireGrouping does not contain the given VecWireGrouping elements in Collection.
*
* @param refWireGrouping the given elements that should not be in actual VecWireElementReference's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's refWireGrouping contains any given VecWireGrouping elements.
*/
public S doesNotHaveRefWireGrouping(java.util.Collection extends VecWireGrouping> refWireGrouping) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping collection is not null.
if (refWireGrouping == null) {
failWithMessage("Expecting refWireGrouping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireGrouping(), refWireGrouping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference has no refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's refWireGrouping is not empty.
*/
public S hasNoRefWireGrouping() {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireGrouping but had :\n <%s>";
// check
if (actual.getRefWireGrouping().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireGrouping());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's referencedWireElement is equal to the given one.
* @param referencedWireElement the given referencedWireElement to compare the actual VecWireElementReference's referencedWireElement to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference's referencedWireElement is not equal to the given one.
*/
public S hasReferencedWireElement(VecWireElement referencedWireElement) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting referencedWireElement of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecWireElement actualReferencedWireElement = actual.getReferencedWireElement();
if (!Objects.areEqual(actualReferencedWireElement, referencedWireElement)) {
failWithMessage(assertjErrorMessage, actual, referencedWireElement, actualReferencedWireElement);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's signal is equal to the given one.
* @param signal the given signal to compare the actual VecWireElementReference's signal to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference's signal is not equal to the given one.
*/
public S hasSignal(VecSignal signal) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signal of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSignal actualSignal = actual.getSignal();
if (!Objects.areEqual(actualSignal, signal)) {
failWithMessage(assertjErrorMessage, actual, signal, actualSignal);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference is unconnected.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference is not unconnected.
*/
public S isUnconnected() {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isUnconnected())) {
failWithMessage("\nExpecting that actual VecWireElementReference is unconnected but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference is not unconnected.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementReference is unconnected.
*/
public S isNotUnconnected() {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isUnconnected())) {
failWithMessage("\nExpecting that actual VecWireElementReference is not unconnected but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireEnds contains the given VecWireEnd elements.
* @param wireEnds the given elements that should be contained in actual VecWireElementReference's wireEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireEnds does not contain all given VecWireEnd elements.
*/
public S hasWireEnds(VecWireEnd... wireEnds) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd varargs is not null.
if (wireEnds == null) failWithMessage("Expecting wireEnds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireEnds(), wireEnds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireEnds contains the given VecWireEnd elements in Collection.
* @param wireEnds the given elements that should be contained in actual VecWireElementReference's wireEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireEnds does not contain all given VecWireEnd elements.
*/
public S hasWireEnds(java.util.Collection extends VecWireEnd> wireEnds) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd collection is not null.
if (wireEnds == null) {
failWithMessage("Expecting wireEnds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireEnds(), wireEnds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireEnds contains only the given VecWireEnd elements and nothing else in whatever order.
* @param wireEnds the given elements that should be contained in actual VecWireElementReference's wireEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireEnds does not contain all given VecWireEnd elements.
*/
public S hasOnlyWireEnds(VecWireEnd... wireEnds) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd varargs is not null.
if (wireEnds == null) failWithMessage("Expecting wireEnds parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireEnds(), wireEnds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireEnds contains only the given VecWireEnd elements in Collection and nothing else in whatever order.
* @param wireEnds the given elements that should be contained in actual VecWireElementReference's wireEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireEnds does not contain all given VecWireEnd elements.
*/
public S hasOnlyWireEnds(java.util.Collection extends VecWireEnd> wireEnds) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd collection is not null.
if (wireEnds == null) {
failWithMessage("Expecting wireEnds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireEnds(), wireEnds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireEnds does not contain the given VecWireEnd elements.
*
* @param wireEnds the given elements that should not be in actual VecWireElementReference's wireEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireEnds contains any given VecWireEnd elements.
*/
public S doesNotHaveWireEnds(VecWireEnd... wireEnds) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd varargs is not null.
if (wireEnds == null) failWithMessage("Expecting wireEnds parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireEnds(), wireEnds);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireEnds does not contain the given VecWireEnd elements in Collection.
*
* @param wireEnds the given elements that should not be in actual VecWireElementReference's wireEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireEnds contains any given VecWireEnd elements.
*/
public S doesNotHaveWireEnds(java.util.Collection extends VecWireEnd> wireEnds) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd collection is not null.
if (wireEnds == null) {
failWithMessage("Expecting wireEnds parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireEnds(), wireEnds.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference has no wireEnds.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireEnds is not empty.
*/
public S hasNoWireEnds() {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have wireEnds but had :\n <%s>";
// check
if (actual.getWireEnds().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getWireEnds());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireLengths contains the given VecWireLength elements.
* @param wireLengths the given elements that should be contained in actual VecWireElementReference's wireLengths.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireLengths does not contain all given VecWireLength elements.
*/
public S hasWireLengths(VecWireLength... wireLengths) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireLength varargs is not null.
if (wireLengths == null) failWithMessage("Expecting wireLengths parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireLengths(), wireLengths);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireLengths contains the given VecWireLength elements in Collection.
* @param wireLengths the given elements that should be contained in actual VecWireElementReference's wireLengths.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireLengths does not contain all given VecWireLength elements.
*/
public S hasWireLengths(java.util.Collection extends VecWireLength> wireLengths) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireLength collection is not null.
if (wireLengths == null) {
failWithMessage("Expecting wireLengths parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireLengths(), wireLengths.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireLengths contains only the given VecWireLength elements and nothing else in whatever order.
* @param wireLengths the given elements that should be contained in actual VecWireElementReference's wireLengths.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireLengths does not contain all given VecWireLength elements.
*/
public S hasOnlyWireLengths(VecWireLength... wireLengths) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireLength varargs is not null.
if (wireLengths == null) failWithMessage("Expecting wireLengths parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireLengths(), wireLengths);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireLengths contains only the given VecWireLength elements in Collection and nothing else in whatever order.
* @param wireLengths the given elements that should be contained in actual VecWireElementReference's wireLengths.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireLengths does not contain all given VecWireLength elements.
*/
public S hasOnlyWireLengths(java.util.Collection extends VecWireLength> wireLengths) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireLength collection is not null.
if (wireLengths == null) {
failWithMessage("Expecting wireLengths parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireLengths(), wireLengths.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireLengths does not contain the given VecWireLength elements.
*
* @param wireLengths the given elements that should not be in actual VecWireElementReference's wireLengths.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireLengths contains any given VecWireLength elements.
*/
public S doesNotHaveWireLengths(VecWireLength... wireLengths) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireLength varargs is not null.
if (wireLengths == null) failWithMessage("Expecting wireLengths parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireLengths(), wireLengths);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference's wireLengths does not contain the given VecWireLength elements in Collection.
*
* @param wireLengths the given elements that should not be in actual VecWireElementReference's wireLengths.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireLengths contains any given VecWireLength elements.
*/
public S doesNotHaveWireLengths(java.util.Collection extends VecWireLength> wireLengths) {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// check that given VecWireLength collection is not null.
if (wireLengths == null) {
failWithMessage("Expecting wireLengths parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireLengths(), wireLengths.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementReference has no wireLengths.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementReference's wireLengths is not empty.
*/
public S hasNoWireLengths() {
// check that actual VecWireElementReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have wireLengths but had :\n <%s>";
// check
if (actual.getWireLengths().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getWireLengths());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy