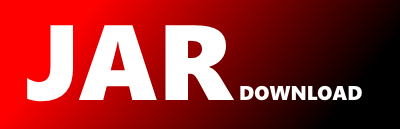
com.foursoft.vecmodel.vec120.AbstractVecWireElementSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecWireElementSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecWireElementSpecificationAssert, A extends VecWireElementSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecWireElementSpecificationAssert}
to make assertions on actual VecWireElementSpecification.
* @param actual the VecWireElementSpecification we want to make assertions on.
*/
protected AbstractVecWireElementSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecWireElementSpecification's conductorSpecification is equal to the given one.
* @param conductorSpecification the given conductorSpecification to compare the actual VecWireElementSpecification's conductorSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's conductorSpecification is not equal to the given one.
*/
public S hasConductorSpecification(VecConductorSpecification conductorSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting conductorSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecConductorSpecification actualConductorSpecification = actual.getConductorSpecification();
if (!Objects.areEqual(actualConductorSpecification, conductorSpecification)) {
failWithMessage(assertjErrorMessage, actual, conductorSpecification, actualConductorSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's fillerSpecification is equal to the given one.
* @param fillerSpecification the given fillerSpecification to compare the actual VecWireElementSpecification's fillerSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's fillerSpecification is not equal to the given one.
*/
public S hasFillerSpecification(VecFillerSpecification fillerSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting fillerSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecFillerSpecification actualFillerSpecification = actual.getFillerSpecification();
if (!Objects.areEqual(actualFillerSpecification, fillerSpecification)) {
failWithMessage(assertjErrorMessage, actual, fillerSpecification, actualFillerSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's gridSpacing is equal to the given one.
* @param gridSpacing the given gridSpacing to compare the actual VecWireElementSpecification's gridSpacing to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's gridSpacing is not equal to the given one.
*/
public S hasGridSpacing(VecNumericalValue gridSpacing) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting gridSpacing of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualGridSpacing = actual.getGridSpacing();
if (!Objects.areEqual(actualGridSpacing, gridSpacing)) {
failWithMessage(assertjErrorMessage, actual, gridSpacing, actualGridSpacing);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's impedance is equal to the given one.
* @param impedance the given impedance to compare the actual VecWireElementSpecification's impedance to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's impedance is not equal to the given one.
*/
public S hasImpedance(VecNumericalValue impedance) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting impedance of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualImpedance = actual.getImpedance();
if (!Objects.areEqual(actualImpedance, impedance)) {
failWithMessage(assertjErrorMessage, actual, impedance, actualImpedance);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's insulationSpecification is equal to the given one.
* @param insulationSpecification the given insulationSpecification to compare the actual VecWireElementSpecification's insulationSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's insulationSpecification is not equal to the given one.
*/
public S hasInsulationSpecification(VecInsulationSpecification insulationSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting insulationSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecInsulationSpecification actualInsulationSpecification = actual.getInsulationSpecification();
if (!Objects.areEqual(actualInsulationSpecification, insulationSpecification)) {
failWithMessage(assertjErrorMessage, actual, insulationSpecification, actualInsulationSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's minBendRadiusDynamic is equal to the given one.
* @param minBendRadiusDynamic the given minBendRadiusDynamic to compare the actual VecWireElementSpecification's minBendRadiusDynamic to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's minBendRadiusDynamic is not equal to the given one.
*/
public S hasMinBendRadiusDynamic(VecNumericalValue minBendRadiusDynamic) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minBendRadiusDynamic of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualMinBendRadiusDynamic = actual.getMinBendRadiusDynamic();
if (!Objects.areEqual(actualMinBendRadiusDynamic, minBendRadiusDynamic)) {
failWithMessage(assertjErrorMessage, actual, minBendRadiusDynamic, actualMinBendRadiusDynamic);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's minBendRadiusStatic is equal to the given one.
* @param minBendRadiusStatic the given minBendRadiusStatic to compare the actual VecWireElementSpecification's minBendRadiusStatic to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's minBendRadiusStatic is not equal to the given one.
*/
public S hasMinBendRadiusStatic(VecNumericalValue minBendRadiusStatic) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minBendRadiusStatic of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualMinBendRadiusStatic = actual.getMinBendRadiusStatic();
if (!Objects.areEqual(actualMinBendRadiusStatic, minBendRadiusStatic)) {
failWithMessage(assertjErrorMessage, actual, minBendRadiusStatic, actualMinBendRadiusStatic);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's outsideDiameter is equal to the given one.
* @param outsideDiameter the given outsideDiameter to compare the actual VecWireElementSpecification's outsideDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's outsideDiameter is not equal to the given one.
*/
public S hasOutsideDiameter(VecNumericalValue outsideDiameter) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting outsideDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualOutsideDiameter = actual.getOutsideDiameter();
if (!Objects.areEqual(actualOutsideDiameter, outsideDiameter)) {
failWithMessage(assertjErrorMessage, actual, outsideDiameter, actualOutsideDiameter);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElement contains the given VecWireElement elements.
* @param refWireElement the given elements that should be contained in actual VecWireElementSpecification's refWireElement.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElement does not contain all given VecWireElement elements.
*/
public S hasRefWireElement(VecWireElement... refWireElement) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElement varargs is not null.
if (refWireElement == null) failWithMessage("Expecting refWireElement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElement(), refWireElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElement contains the given VecWireElement elements in Collection.
* @param refWireElement the given elements that should be contained in actual VecWireElementSpecification's refWireElement.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElement does not contain all given VecWireElement elements.
*/
public S hasRefWireElement(java.util.Collection extends VecWireElement> refWireElement) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElement collection is not null.
if (refWireElement == null) {
failWithMessage("Expecting refWireElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElement(), refWireElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElement contains only the given VecWireElement elements and nothing else in whatever order.
* @param refWireElement the given elements that should be contained in actual VecWireElementSpecification's refWireElement.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElement does not contain all given VecWireElement elements.
*/
public S hasOnlyRefWireElement(VecWireElement... refWireElement) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElement varargs is not null.
if (refWireElement == null) failWithMessage("Expecting refWireElement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElement(), refWireElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElement contains only the given VecWireElement elements in Collection and nothing else in whatever order.
* @param refWireElement the given elements that should be contained in actual VecWireElementSpecification's refWireElement.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElement does not contain all given VecWireElement elements.
*/
public S hasOnlyRefWireElement(java.util.Collection extends VecWireElement> refWireElement) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElement collection is not null.
if (refWireElement == null) {
failWithMessage("Expecting refWireElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElement(), refWireElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElement does not contain the given VecWireElement elements.
*
* @param refWireElement the given elements that should not be in actual VecWireElementSpecification's refWireElement.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElement contains any given VecWireElement elements.
*/
public S doesNotHaveRefWireElement(VecWireElement... refWireElement) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElement varargs is not null.
if (refWireElement == null) failWithMessage("Expecting refWireElement parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElement(), refWireElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElement does not contain the given VecWireElement elements in Collection.
*
* @param refWireElement the given elements that should not be in actual VecWireElementSpecification's refWireElement.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElement contains any given VecWireElement elements.
*/
public S doesNotHaveRefWireElement(java.util.Collection extends VecWireElement> refWireElement) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElement collection is not null.
if (refWireElement == null) {
failWithMessage("Expecting refWireElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElement(), refWireElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification has no refWireElement.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElement is not empty.
*/
public S hasNoRefWireElement() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireElement but had :\n <%s>";
// check
if (actual.getRefWireElement().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireElement());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElementSpecification contains the given VecWireElementSpecification elements.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElementSpecification contains the given VecWireElementSpecification elements in Collection.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElementSpecification contains only the given VecWireElementSpecification elements and nothing else in whatever order.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlyRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElementSpecification contains only the given VecWireElementSpecification elements in Collection and nothing else in whatever order.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlyRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElementSpecification does not contain the given VecWireElementSpecification elements.
*
* @param refWireElementSpecification the given elements that should not be in actual VecWireElementSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireElementSpecification does not contain the given VecWireElementSpecification elements in Collection.
*
* @param refWireElementSpecification the given elements that should not be in actual VecWireElementSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification has no refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireElementSpecification is not empty.
*/
public S hasNoRefWireElementSpecification() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireElementSpecification but had :\n <%s>";
// check
if (actual.getRefWireElementSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireElementSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireSpecification contains the given VecWireSpecification elements.
* @param refWireSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireSpecification does not contain all given VecWireSpecification elements.
*/
public S hasRefWireSpecification(VecWireSpecification... refWireSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireSpecification varargs is not null.
if (refWireSpecification == null) failWithMessage("Expecting refWireSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireSpecification(), refWireSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireSpecification contains the given VecWireSpecification elements in Collection.
* @param refWireSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireSpecification does not contain all given VecWireSpecification elements.
*/
public S hasRefWireSpecification(java.util.Collection extends VecWireSpecification> refWireSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireSpecification collection is not null.
if (refWireSpecification == null) {
failWithMessage("Expecting refWireSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireSpecification(), refWireSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireSpecification contains only the given VecWireSpecification elements and nothing else in whatever order.
* @param refWireSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireSpecification does not contain all given VecWireSpecification elements.
*/
public S hasOnlyRefWireSpecification(VecWireSpecification... refWireSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireSpecification varargs is not null.
if (refWireSpecification == null) failWithMessage("Expecting refWireSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireSpecification(), refWireSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireSpecification contains only the given VecWireSpecification elements in Collection and nothing else in whatever order.
* @param refWireSpecification the given elements that should be contained in actual VecWireElementSpecification's refWireSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireSpecification does not contain all given VecWireSpecification elements.
*/
public S hasOnlyRefWireSpecification(java.util.Collection extends VecWireSpecification> refWireSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireSpecification collection is not null.
if (refWireSpecification == null) {
failWithMessage("Expecting refWireSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireSpecification(), refWireSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireSpecification does not contain the given VecWireSpecification elements.
*
* @param refWireSpecification the given elements that should not be in actual VecWireElementSpecification's refWireSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireSpecification contains any given VecWireSpecification elements.
*/
public S doesNotHaveRefWireSpecification(VecWireSpecification... refWireSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireSpecification varargs is not null.
if (refWireSpecification == null) failWithMessage("Expecting refWireSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireSpecification(), refWireSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's refWireSpecification does not contain the given VecWireSpecification elements in Collection.
*
* @param refWireSpecification the given elements that should not be in actual VecWireElementSpecification's refWireSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireSpecification contains any given VecWireSpecification elements.
*/
public S doesNotHaveRefWireSpecification(java.util.Collection extends VecWireSpecification> refWireSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireSpecification collection is not null.
if (refWireSpecification == null) {
failWithMessage("Expecting refWireSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireSpecification(), refWireSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification has no refWireSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's refWireSpecification is not empty.
*/
public S hasNoRefWireSpecification() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireSpecification but had :\n <%s>";
// check
if (actual.getRefWireSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's shape is equal to the given one.
* @param shape the given shape to compare the actual VecWireElementSpecification's shape to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's shape is not equal to the given one.
*/
public S hasShape(String shape) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting shape of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualShape = actual.getShape();
if (!Objects.areEqual(actualShape, shape)) {
failWithMessage(assertjErrorMessage, actual, shape, actualShape);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's size is equal to the given one.
* @param size the given size to compare the actual VecWireElementSpecification's size to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's size is not equal to the given one.
*/
public S hasSize(VecSize size) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting size of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSize actualSize = actual.getSize();
if (!Objects.areEqual(actualSize, size)) {
failWithMessage(assertjErrorMessage, actual, size, actualSize);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's subWireElementSpecification contains the given VecWireElementSpecification elements.
* @param subWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's subWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's subWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasSubWireElementSpecification(VecWireElementSpecification... subWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (subWireElementSpecification == null) failWithMessage("Expecting subWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSubWireElementSpecification(), subWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's subWireElementSpecification contains the given VecWireElementSpecification elements in Collection.
* @param subWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's subWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's subWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasSubWireElementSpecification(java.util.Collection extends VecWireElementSpecification> subWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (subWireElementSpecification == null) {
failWithMessage("Expecting subWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getSubWireElementSpecification(), subWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's subWireElementSpecification contains only the given VecWireElementSpecification elements and nothing else in whatever order.
* @param subWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's subWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's subWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlySubWireElementSpecification(VecWireElementSpecification... subWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (subWireElementSpecification == null) failWithMessage("Expecting subWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSubWireElementSpecification(), subWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's subWireElementSpecification contains only the given VecWireElementSpecification elements in Collection and nothing else in whatever order.
* @param subWireElementSpecification the given elements that should be contained in actual VecWireElementSpecification's subWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's subWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlySubWireElementSpecification(java.util.Collection extends VecWireElementSpecification> subWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (subWireElementSpecification == null) {
failWithMessage("Expecting subWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getSubWireElementSpecification(), subWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's subWireElementSpecification does not contain the given VecWireElementSpecification elements.
*
* @param subWireElementSpecification the given elements that should not be in actual VecWireElementSpecification's subWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's subWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveSubWireElementSpecification(VecWireElementSpecification... subWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (subWireElementSpecification == null) failWithMessage("Expecting subWireElementSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSubWireElementSpecification(), subWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's subWireElementSpecification does not contain the given VecWireElementSpecification elements in Collection.
*
* @param subWireElementSpecification the given elements that should not be in actual VecWireElementSpecification's subWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's subWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveSubWireElementSpecification(java.util.Collection extends VecWireElementSpecification> subWireElementSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (subWireElementSpecification == null) {
failWithMessage("Expecting subWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getSubWireElementSpecification(), subWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification has no subWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's subWireElementSpecification is not empty.
*/
public S hasNoSubWireElementSpecification() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have subWireElementSpecification but had :\n <%s>";
// check
if (actual.getSubWireElementSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getSubWireElementSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification is suited for dynamic use.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification is not suited for dynamic use.
*/
public S isSuitedForDynamicUse() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isSuitedForDynamicUse())) {
failWithMessage("\nExpecting that actual VecWireElementSpecification is suited for dynamic use but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification is not suited for dynamic use.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification is suited for dynamic use.
*/
public S isNotSuitedForDynamicUse() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isSuitedForDynamicUse())) {
failWithMessage("\nExpecting that actual VecWireElementSpecification is not suited for dynamic use but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's types contains the given VecWireType elements.
* @param types the given elements that should be contained in actual VecWireElementSpecification's types.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's types does not contain all given VecWireType elements.
*/
public S hasTypes(VecWireType... types) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireType varargs is not null.
if (types == null) failWithMessage("Expecting types parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTypes(), types);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's types contains the given VecWireType elements in Collection.
* @param types the given elements that should be contained in actual VecWireElementSpecification's types.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's types does not contain all given VecWireType elements.
*/
public S hasTypes(java.util.Collection extends VecWireType> types) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireType collection is not null.
if (types == null) {
failWithMessage("Expecting types parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getTypes(), types.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's types contains only the given VecWireType elements and nothing else in whatever order.
* @param types the given elements that should be contained in actual VecWireElementSpecification's types.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's types does not contain all given VecWireType elements.
*/
public S hasOnlyTypes(VecWireType... types) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireType varargs is not null.
if (types == null) failWithMessage("Expecting types parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTypes(), types);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's types contains only the given VecWireType elements in Collection and nothing else in whatever order.
* @param types the given elements that should be contained in actual VecWireElementSpecification's types.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's types does not contain all given VecWireType elements.
*/
public S hasOnlyTypes(java.util.Collection extends VecWireType> types) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireType collection is not null.
if (types == null) {
failWithMessage("Expecting types parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getTypes(), types.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's types does not contain the given VecWireType elements.
*
* @param types the given elements that should not be in actual VecWireElementSpecification's types.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's types contains any given VecWireType elements.
*/
public S doesNotHaveTypes(VecWireType... types) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireType varargs is not null.
if (types == null) failWithMessage("Expecting types parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTypes(), types);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's types does not contain the given VecWireType elements in Collection.
*
* @param types the given elements that should not be in actual VecWireElementSpecification's types.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's types contains any given VecWireType elements.
*/
public S doesNotHaveTypes(java.util.Collection extends VecWireType> types) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireType collection is not null.
if (types == null) {
failWithMessage("Expecting types parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getTypes(), types.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification has no types.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's types is not empty.
*/
public S hasNoTypes() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have types but had :\n <%s>";
// check
if (actual.getTypes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getTypes());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's validWireReceptionTypes contains the given String elements.
* @param validWireReceptionTypes the given elements that should be contained in actual VecWireElementSpecification's validWireReceptionTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's validWireReceptionTypes does not contain all given String elements.
*/
public S hasValidWireReceptionTypes(String... validWireReceptionTypes) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given String varargs is not null.
if (validWireReceptionTypes == null) failWithMessage("Expecting validWireReceptionTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getValidWireReceptionTypes(), validWireReceptionTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's validWireReceptionTypes contains the given String elements in Collection.
* @param validWireReceptionTypes the given elements that should be contained in actual VecWireElementSpecification's validWireReceptionTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's validWireReceptionTypes does not contain all given String elements.
*/
public S hasValidWireReceptionTypes(java.util.Collection extends String> validWireReceptionTypes) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given String collection is not null.
if (validWireReceptionTypes == null) {
failWithMessage("Expecting validWireReceptionTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getValidWireReceptionTypes(), validWireReceptionTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's validWireReceptionTypes contains only the given String elements and nothing else in whatever order.
* @param validWireReceptionTypes the given elements that should be contained in actual VecWireElementSpecification's validWireReceptionTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's validWireReceptionTypes does not contain all given String elements.
*/
public S hasOnlyValidWireReceptionTypes(String... validWireReceptionTypes) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given String varargs is not null.
if (validWireReceptionTypes == null) failWithMessage("Expecting validWireReceptionTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getValidWireReceptionTypes(), validWireReceptionTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's validWireReceptionTypes contains only the given String elements in Collection and nothing else in whatever order.
* @param validWireReceptionTypes the given elements that should be contained in actual VecWireElementSpecification's validWireReceptionTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's validWireReceptionTypes does not contain all given String elements.
*/
public S hasOnlyValidWireReceptionTypes(java.util.Collection extends String> validWireReceptionTypes) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given String collection is not null.
if (validWireReceptionTypes == null) {
failWithMessage("Expecting validWireReceptionTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getValidWireReceptionTypes(), validWireReceptionTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's validWireReceptionTypes does not contain the given String elements.
*
* @param validWireReceptionTypes the given elements that should not be in actual VecWireElementSpecification's validWireReceptionTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's validWireReceptionTypes contains any given String elements.
*/
public S doesNotHaveValidWireReceptionTypes(String... validWireReceptionTypes) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given String varargs is not null.
if (validWireReceptionTypes == null) failWithMessage("Expecting validWireReceptionTypes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getValidWireReceptionTypes(), validWireReceptionTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's validWireReceptionTypes does not contain the given String elements in Collection.
*
* @param validWireReceptionTypes the given elements that should not be in actual VecWireElementSpecification's validWireReceptionTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's validWireReceptionTypes contains any given String elements.
*/
public S doesNotHaveValidWireReceptionTypes(java.util.Collection extends String> validWireReceptionTypes) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// check that given String collection is not null.
if (validWireReceptionTypes == null) {
failWithMessage("Expecting validWireReceptionTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getValidWireReceptionTypes(), validWireReceptionTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification has no validWireReceptionTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecWireElementSpecification's validWireReceptionTypes is not empty.
*/
public S hasNoValidWireReceptionTypes() {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have validWireReceptionTypes but had :\n <%s>";
// check
if (actual.getValidWireReceptionTypes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getValidWireReceptionTypes());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireElementSpecification's wireGroupSpecification is equal to the given one.
* @param wireGroupSpecification the given wireGroupSpecification to compare the actual VecWireElementSpecification's wireGroupSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireElementSpecification's wireGroupSpecification is not equal to the given one.
*/
public S hasWireGroupSpecification(VecWireGroupSpecification wireGroupSpecification) {
// check that actual VecWireElementSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting wireGroupSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecWireGroupSpecification actualWireGroupSpecification = actual.getWireGroupSpecification();
if (!Objects.areEqual(actualWireGroupSpecification, wireGroupSpecification)) {
failWithMessage(assertjErrorMessage, actual, wireGroupSpecification, actualWireGroupSpecification);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy