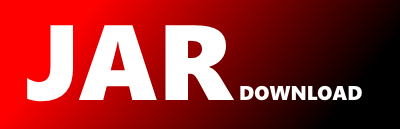
com.foursoft.vecmodel.vec120.AbstractVecWireGroupSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecWireGroupSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecWireGroupSpecificationAssert, A extends VecWireGroupSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecWireGroupSpecificationAssert}
to make assertions on actual VecWireGroupSpecification.
* @param actual the VecWireGroupSpecification we want to make assertions on.
*/
protected AbstractVecWireGroupSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecWireGroupSpecification's groupType is equal to the given one.
* @param groupType the given groupType to compare the actual VecWireGroupSpecification's groupType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireGroupSpecification's groupType is not equal to the given one.
*/
public S hasGroupType(String groupType) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting groupType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualGroupType = actual.getGroupType();
if (!Objects.areEqual(actualGroupType, groupType)) {
failWithMessage(assertjErrorMessage, actual, groupType, actualGroupType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's lengthOfTwist is equal to the given one.
* @param lengthOfTwist the given lengthOfTwist to compare the actual VecWireGroupSpecification's lengthOfTwist to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireGroupSpecification's lengthOfTwist is not equal to the given one.
*/
public S hasLengthOfTwist(VecNumericalValue lengthOfTwist) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting lengthOfTwist of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualLengthOfTwist = actual.getLengthOfTwist();
if (!Objects.areEqual(actualLengthOfTwist, lengthOfTwist)) {
failWithMessage(assertjErrorMessage, actual, lengthOfTwist, actualLengthOfTwist);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireElementSpecification contains the given VecWireElementSpecification elements.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireGroupSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireElementSpecification contains the given VecWireElementSpecification elements in Collection.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireGroupSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireElementSpecification contains only the given VecWireElementSpecification elements and nothing else in whatever order.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireGroupSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlyRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireElementSpecification contains only the given VecWireElementSpecification elements in Collection and nothing else in whatever order.
* @param refWireElementSpecification the given elements that should be contained in actual VecWireGroupSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlyRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireElementSpecification does not contain the given VecWireElementSpecification elements.
*
* @param refWireElementSpecification the given elements that should not be in actual VecWireGroupSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireElementSpecification does not contain the given VecWireElementSpecification elements in Collection.
*
* @param refWireElementSpecification the given elements that should not be in actual VecWireGroupSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification has no refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireElementSpecification is not empty.
*/
public S hasNoRefWireElementSpecification() {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireElementSpecification but had :\n <%s>";
// check
if (actual.getRefWireElementSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireElementSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireGrouping contains the given VecWireGrouping elements.
* @param refWireGrouping the given elements that should be contained in actual VecWireGroupSpecification's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasRefWireGrouping(VecWireGrouping... refWireGrouping) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping varargs is not null.
if (refWireGrouping == null) failWithMessage("Expecting refWireGrouping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireGrouping(), refWireGrouping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireGrouping contains the given VecWireGrouping elements in Collection.
* @param refWireGrouping the given elements that should be contained in actual VecWireGroupSpecification's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasRefWireGrouping(java.util.Collection extends VecWireGrouping> refWireGrouping) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping collection is not null.
if (refWireGrouping == null) {
failWithMessage("Expecting refWireGrouping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireGrouping(), refWireGrouping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireGrouping contains only the given VecWireGrouping elements and nothing else in whatever order.
* @param refWireGrouping the given elements that should be contained in actual VecWireGroupSpecification's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasOnlyRefWireGrouping(VecWireGrouping... refWireGrouping) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping varargs is not null.
if (refWireGrouping == null) failWithMessage("Expecting refWireGrouping parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireGrouping(), refWireGrouping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireGrouping contains only the given VecWireGrouping elements in Collection and nothing else in whatever order.
* @param refWireGrouping the given elements that should be contained in actual VecWireGroupSpecification's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireGrouping does not contain all given VecWireGrouping elements.
*/
public S hasOnlyRefWireGrouping(java.util.Collection extends VecWireGrouping> refWireGrouping) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping collection is not null.
if (refWireGrouping == null) {
failWithMessage("Expecting refWireGrouping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireGrouping(), refWireGrouping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireGrouping does not contain the given VecWireGrouping elements.
*
* @param refWireGrouping the given elements that should not be in actual VecWireGroupSpecification's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireGrouping contains any given VecWireGrouping elements.
*/
public S doesNotHaveRefWireGrouping(VecWireGrouping... refWireGrouping) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping varargs is not null.
if (refWireGrouping == null) failWithMessage("Expecting refWireGrouping parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireGrouping(), refWireGrouping);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification's refWireGrouping does not contain the given VecWireGrouping elements in Collection.
*
* @param refWireGrouping the given elements that should not be in actual VecWireGroupSpecification's refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireGrouping contains any given VecWireGrouping elements.
*/
public S doesNotHaveRefWireGrouping(java.util.Collection extends VecWireGrouping> refWireGrouping) {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireGrouping collection is not null.
if (refWireGrouping == null) {
failWithMessage("Expecting refWireGrouping parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireGrouping(), refWireGrouping.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireGroupSpecification has no refWireGrouping.
* @return this assertion object.
* @throws AssertionError if the actual VecWireGroupSpecification's refWireGrouping is not empty.
*/
public S hasNoRefWireGrouping() {
// check that actual VecWireGroupSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireGrouping but had :\n <%s>";
// check
if (actual.getRefWireGrouping().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireGrouping());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy