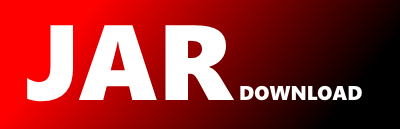
com.foursoft.vecmodel.vec120.AbstractVecWireMountingAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecWireMounting} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecWireMountingAssert, A extends VecWireMounting> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecWireMountingAssert}
to make assertions on actual VecWireMounting.
* @param actual the VecWireMounting we want to make assertions on.
*/
protected AbstractVecWireMountingAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecWireMounting's mountedCavitySeal is equal to the given one.
* @param mountedCavitySeal the given mountedCavitySeal to compare the actual VecWireMounting's mountedCavitySeal to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireMounting's mountedCavitySeal is not equal to the given one.
*/
public S hasMountedCavitySeal(VecCavitySealRole mountedCavitySeal) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting mountedCavitySeal of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCavitySealRole actualMountedCavitySeal = actual.getMountedCavitySeal();
if (!Objects.areEqual(actualMountedCavitySeal, mountedCavitySeal)) {
failWithMessage(assertjErrorMessage, actual, mountedCavitySeal, actualMountedCavitySeal);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's parentContactPoint is equal to the given one.
* @param parentContactPoint the given parentContactPoint to compare the actual VecWireMounting's parentContactPoint to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireMounting's parentContactPoint is not equal to the given one.
*/
public S hasParentContactPoint(VecContactPoint parentContactPoint) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentContactPoint of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecContactPoint actualParentContactPoint = actual.getParentContactPoint();
if (!Objects.areEqual(actualParentContactPoint, parentContactPoint)) {
failWithMessage(assertjErrorMessage, actual, parentContactPoint, actualParentContactPoint);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's referencedWireEnd contains the given VecWireEnd elements.
* @param referencedWireEnd the given elements that should be contained in actual VecWireMounting's referencedWireEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's referencedWireEnd does not contain all given VecWireEnd elements.
*/
public S hasReferencedWireEnd(VecWireEnd... referencedWireEnd) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd varargs is not null.
if (referencedWireEnd == null) failWithMessage("Expecting referencedWireEnd parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getReferencedWireEnd(), referencedWireEnd);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's referencedWireEnd contains the given VecWireEnd elements in Collection.
* @param referencedWireEnd the given elements that should be contained in actual VecWireMounting's referencedWireEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's referencedWireEnd does not contain all given VecWireEnd elements.
*/
public S hasReferencedWireEnd(java.util.Collection extends VecWireEnd> referencedWireEnd) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd collection is not null.
if (referencedWireEnd == null) {
failWithMessage("Expecting referencedWireEnd parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getReferencedWireEnd(), referencedWireEnd.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's referencedWireEnd contains only the given VecWireEnd elements and nothing else in whatever order.
* @param referencedWireEnd the given elements that should be contained in actual VecWireMounting's referencedWireEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's referencedWireEnd does not contain all given VecWireEnd elements.
*/
public S hasOnlyReferencedWireEnd(VecWireEnd... referencedWireEnd) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd varargs is not null.
if (referencedWireEnd == null) failWithMessage("Expecting referencedWireEnd parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getReferencedWireEnd(), referencedWireEnd);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's referencedWireEnd contains only the given VecWireEnd elements in Collection and nothing else in whatever order.
* @param referencedWireEnd the given elements that should be contained in actual VecWireMounting's referencedWireEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's referencedWireEnd does not contain all given VecWireEnd elements.
*/
public S hasOnlyReferencedWireEnd(java.util.Collection extends VecWireEnd> referencedWireEnd) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd collection is not null.
if (referencedWireEnd == null) {
failWithMessage("Expecting referencedWireEnd parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getReferencedWireEnd(), referencedWireEnd.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's referencedWireEnd does not contain the given VecWireEnd elements.
*
* @param referencedWireEnd the given elements that should not be in actual VecWireMounting's referencedWireEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's referencedWireEnd contains any given VecWireEnd elements.
*/
public S doesNotHaveReferencedWireEnd(VecWireEnd... referencedWireEnd) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd varargs is not null.
if (referencedWireEnd == null) failWithMessage("Expecting referencedWireEnd parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getReferencedWireEnd(), referencedWireEnd);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's referencedWireEnd does not contain the given VecWireEnd elements in Collection.
*
* @param referencedWireEnd the given elements that should not be in actual VecWireMounting's referencedWireEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's referencedWireEnd contains any given VecWireEnd elements.
*/
public S doesNotHaveReferencedWireEnd(java.util.Collection extends VecWireEnd> referencedWireEnd) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEnd collection is not null.
if (referencedWireEnd == null) {
failWithMessage("Expecting referencedWireEnd parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getReferencedWireEnd(), referencedWireEnd.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting has no referencedWireEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's referencedWireEnd is not empty.
*/
public S hasNoReferencedWireEnd() {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have referencedWireEnd but had :\n <%s>";
// check
if (actual.getReferencedWireEnd().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getReferencedWireEnd());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireEndAccessory contains the given VecWireEndAccessoryRole elements.
* @param wireEndAccessory the given elements that should be contained in actual VecWireMounting's wireEndAccessory.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireEndAccessory does not contain all given VecWireEndAccessoryRole elements.
*/
public S hasWireEndAccessory(VecWireEndAccessoryRole... wireEndAccessory) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessoryRole varargs is not null.
if (wireEndAccessory == null) failWithMessage("Expecting wireEndAccessory parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireEndAccessory(), wireEndAccessory);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireEndAccessory contains the given VecWireEndAccessoryRole elements in Collection.
* @param wireEndAccessory the given elements that should be contained in actual VecWireMounting's wireEndAccessory.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireEndAccessory does not contain all given VecWireEndAccessoryRole elements.
*/
public S hasWireEndAccessory(java.util.Collection extends VecWireEndAccessoryRole> wireEndAccessory) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessoryRole collection is not null.
if (wireEndAccessory == null) {
failWithMessage("Expecting wireEndAccessory parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireEndAccessory(), wireEndAccessory.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireEndAccessory contains only the given VecWireEndAccessoryRole elements and nothing else in whatever order.
* @param wireEndAccessory the given elements that should be contained in actual VecWireMounting's wireEndAccessory.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireEndAccessory does not contain all given VecWireEndAccessoryRole elements.
*/
public S hasOnlyWireEndAccessory(VecWireEndAccessoryRole... wireEndAccessory) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessoryRole varargs is not null.
if (wireEndAccessory == null) failWithMessage("Expecting wireEndAccessory parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireEndAccessory(), wireEndAccessory);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireEndAccessory contains only the given VecWireEndAccessoryRole elements in Collection and nothing else in whatever order.
* @param wireEndAccessory the given elements that should be contained in actual VecWireMounting's wireEndAccessory.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireEndAccessory does not contain all given VecWireEndAccessoryRole elements.
*/
public S hasOnlyWireEndAccessory(java.util.Collection extends VecWireEndAccessoryRole> wireEndAccessory) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessoryRole collection is not null.
if (wireEndAccessory == null) {
failWithMessage("Expecting wireEndAccessory parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireEndAccessory(), wireEndAccessory.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireEndAccessory does not contain the given VecWireEndAccessoryRole elements.
*
* @param wireEndAccessory the given elements that should not be in actual VecWireMounting's wireEndAccessory.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireEndAccessory contains any given VecWireEndAccessoryRole elements.
*/
public S doesNotHaveWireEndAccessory(VecWireEndAccessoryRole... wireEndAccessory) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessoryRole varargs is not null.
if (wireEndAccessory == null) failWithMessage("Expecting wireEndAccessory parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireEndAccessory(), wireEndAccessory);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireEndAccessory does not contain the given VecWireEndAccessoryRole elements in Collection.
*
* @param wireEndAccessory the given elements that should not be in actual VecWireMounting's wireEndAccessory.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireEndAccessory contains any given VecWireEndAccessoryRole elements.
*/
public S doesNotHaveWireEndAccessory(java.util.Collection extends VecWireEndAccessoryRole> wireEndAccessory) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessoryRole collection is not null.
if (wireEndAccessory == null) {
failWithMessage("Expecting wireEndAccessory parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireEndAccessory(), wireEndAccessory.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting has no wireEndAccessory.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireEndAccessory is not empty.
*/
public S hasNoWireEndAccessory() {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have wireEndAccessory but had :\n <%s>";
// check
if (actual.getWireEndAccessory().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getWireEndAccessory());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireMountingDetails contains the given VecWireMountingDetail elements.
* @param wireMountingDetails the given elements that should be contained in actual VecWireMounting's wireMountingDetails.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireMountingDetails does not contain all given VecWireMountingDetail elements.
*/
public S hasWireMountingDetails(VecWireMountingDetail... wireMountingDetails) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireMountingDetail varargs is not null.
if (wireMountingDetails == null) failWithMessage("Expecting wireMountingDetails parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireMountingDetails(), wireMountingDetails);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireMountingDetails contains the given VecWireMountingDetail elements in Collection.
* @param wireMountingDetails the given elements that should be contained in actual VecWireMounting's wireMountingDetails.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireMountingDetails does not contain all given VecWireMountingDetail elements.
*/
public S hasWireMountingDetails(java.util.Collection extends VecWireMountingDetail> wireMountingDetails) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireMountingDetail collection is not null.
if (wireMountingDetails == null) {
failWithMessage("Expecting wireMountingDetails parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getWireMountingDetails(), wireMountingDetails.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireMountingDetails contains only the given VecWireMountingDetail elements and nothing else in whatever order.
* @param wireMountingDetails the given elements that should be contained in actual VecWireMounting's wireMountingDetails.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireMountingDetails does not contain all given VecWireMountingDetail elements.
*/
public S hasOnlyWireMountingDetails(VecWireMountingDetail... wireMountingDetails) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireMountingDetail varargs is not null.
if (wireMountingDetails == null) failWithMessage("Expecting wireMountingDetails parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireMountingDetails(), wireMountingDetails);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireMountingDetails contains only the given VecWireMountingDetail elements in Collection and nothing else in whatever order.
* @param wireMountingDetails the given elements that should be contained in actual VecWireMounting's wireMountingDetails.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireMountingDetails does not contain all given VecWireMountingDetail elements.
*/
public S hasOnlyWireMountingDetails(java.util.Collection extends VecWireMountingDetail> wireMountingDetails) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireMountingDetail collection is not null.
if (wireMountingDetails == null) {
failWithMessage("Expecting wireMountingDetails parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getWireMountingDetails(), wireMountingDetails.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireMountingDetails does not contain the given VecWireMountingDetail elements.
*
* @param wireMountingDetails the given elements that should not be in actual VecWireMounting's wireMountingDetails.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireMountingDetails contains any given VecWireMountingDetail elements.
*/
public S doesNotHaveWireMountingDetails(VecWireMountingDetail... wireMountingDetails) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireMountingDetail varargs is not null.
if (wireMountingDetails == null) failWithMessage("Expecting wireMountingDetails parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireMountingDetails(), wireMountingDetails);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting's wireMountingDetails does not contain the given VecWireMountingDetail elements in Collection.
*
* @param wireMountingDetails the given elements that should not be in actual VecWireMounting's wireMountingDetails.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireMountingDetails contains any given VecWireMountingDetail elements.
*/
public S doesNotHaveWireMountingDetails(java.util.Collection extends VecWireMountingDetail> wireMountingDetails) {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// check that given VecWireMountingDetail collection is not null.
if (wireMountingDetails == null) {
failWithMessage("Expecting wireMountingDetails parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getWireMountingDetails(), wireMountingDetails.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireMounting has no wireMountingDetails.
* @return this assertion object.
* @throws AssertionError if the actual VecWireMounting's wireMountingDetails is not empty.
*/
public S hasNoWireMountingDetails() {
// check that actual VecWireMounting we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have wireMountingDetails but had :\n <%s>";
// check
if (actual.getWireMountingDetails().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getWireMountingDetails());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy