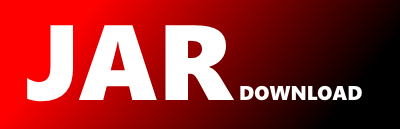
com.foursoft.vecmodel.vec120.AbstractVecWireReceptionSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecWireReceptionSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecWireReceptionSpecificationAssert, A extends VecWireReceptionSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecWireReceptionSpecificationAssert}
to make assertions on actual VecWireReceptionSpecification.
* @param actual the VecWireReceptionSpecification we want to make assertions on.
*/
protected AbstractVecWireReceptionSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecWireReceptionSpecification's addOns contains the given VecWireReceptionAddOn elements.
* @param addOns the given elements that should be contained in actual VecWireReceptionSpecification's addOns.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's addOns does not contain all given VecWireReceptionAddOn elements.
*/
public S hasAddOns(VecWireReceptionAddOn... addOns) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionAddOn varargs is not null.
if (addOns == null) failWithMessage("Expecting addOns parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAddOns(), addOns);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's addOns contains the given VecWireReceptionAddOn elements in Collection.
* @param addOns the given elements that should be contained in actual VecWireReceptionSpecification's addOns.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's addOns does not contain all given VecWireReceptionAddOn elements.
*/
public S hasAddOns(java.util.Collection extends VecWireReceptionAddOn> addOns) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionAddOn collection is not null.
if (addOns == null) {
failWithMessage("Expecting addOns parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAddOns(), addOns.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's addOns contains only the given VecWireReceptionAddOn elements and nothing else in whatever order.
* @param addOns the given elements that should be contained in actual VecWireReceptionSpecification's addOns.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's addOns does not contain all given VecWireReceptionAddOn elements.
*/
public S hasOnlyAddOns(VecWireReceptionAddOn... addOns) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionAddOn varargs is not null.
if (addOns == null) failWithMessage("Expecting addOns parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAddOns(), addOns);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's addOns contains only the given VecWireReceptionAddOn elements in Collection and nothing else in whatever order.
* @param addOns the given elements that should be contained in actual VecWireReceptionSpecification's addOns.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's addOns does not contain all given VecWireReceptionAddOn elements.
*/
public S hasOnlyAddOns(java.util.Collection extends VecWireReceptionAddOn> addOns) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionAddOn collection is not null.
if (addOns == null) {
failWithMessage("Expecting addOns parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAddOns(), addOns.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's addOns does not contain the given VecWireReceptionAddOn elements.
*
* @param addOns the given elements that should not be in actual VecWireReceptionSpecification's addOns.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's addOns contains any given VecWireReceptionAddOn elements.
*/
public S doesNotHaveAddOns(VecWireReceptionAddOn... addOns) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionAddOn varargs is not null.
if (addOns == null) failWithMessage("Expecting addOns parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAddOns(), addOns);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's addOns does not contain the given VecWireReceptionAddOn elements in Collection.
*
* @param addOns the given elements that should not be in actual VecWireReceptionSpecification's addOns.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's addOns contains any given VecWireReceptionAddOn elements.
*/
public S doesNotHaveAddOns(java.util.Collection extends VecWireReceptionAddOn> addOns) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReceptionAddOn collection is not null.
if (addOns == null) {
failWithMessage("Expecting addOns parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAddOns(), addOns.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification has no addOns.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's addOns is not empty.
*/
public S hasNoAddOns() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have addOns but had :\n <%s>";
// check
if (actual.getAddOns().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAddOns());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's coreCrossSectionArea is equal to the given one.
* @param coreCrossSectionArea the given coreCrossSectionArea to compare the actual VecWireReceptionSpecification's coreCrossSectionArea to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification's coreCrossSectionArea is not equal to the given one.
*/
public S hasCoreCrossSectionArea(VecValueRange coreCrossSectionArea) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting coreCrossSectionArea of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecValueRange actualCoreCrossSectionArea = actual.getCoreCrossSectionArea();
if (!Objects.areEqual(actualCoreCrossSectionArea, coreCrossSectionArea)) {
failWithMessage(assertjErrorMessage, actual, coreCrossSectionArea, actualCoreCrossSectionArea);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's insulationDisplacementLength is equal to the given one.
* @param insulationDisplacementLength the given insulationDisplacementLength to compare the actual VecWireReceptionSpecification's insulationDisplacementLength to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification's insulationDisplacementLength is not equal to the given one.
*/
public S hasInsulationDisplacementLength(VecNumericalValue insulationDisplacementLength) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting insulationDisplacementLength of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualInsulationDisplacementLength = actual.getInsulationDisplacementLength();
if (!Objects.areEqual(actualInsulationDisplacementLength, insulationDisplacementLength)) {
failWithMessage(assertjErrorMessage, actual, insulationDisplacementLength, actualInsulationDisplacementLength);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification is multi contact.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification is not multi contact.
*/
public S isMultiContact() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isMultiContact())) {
failWithMessage("\nExpecting that actual VecWireReceptionSpecification is multi contact but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification is not multi contact.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification is multi contact.
*/
public S isNotMultiContact() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isMultiContact())) {
failWithMessage("\nExpecting that actual VecWireReceptionSpecification is not multi contact but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's platingMaterials contains the given VecMaterial elements.
* @param platingMaterials the given elements that should be contained in actual VecWireReceptionSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasPlatingMaterials(VecMaterial... platingMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (platingMaterials == null) failWithMessage("Expecting platingMaterials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlatingMaterials(), platingMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's platingMaterials contains the given VecMaterial elements in Collection.
* @param platingMaterials the given elements that should be contained in actual VecWireReceptionSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasPlatingMaterials(java.util.Collection extends VecMaterial> platingMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (platingMaterials == null) {
failWithMessage("Expecting platingMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlatingMaterials(), platingMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's platingMaterials contains only the given VecMaterial elements and nothing else in whatever order.
* @param platingMaterials the given elements that should be contained in actual VecWireReceptionSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasOnlyPlatingMaterials(VecMaterial... platingMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (platingMaterials == null) failWithMessage("Expecting platingMaterials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlatingMaterials(), platingMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's platingMaterials contains only the given VecMaterial elements in Collection and nothing else in whatever order.
* @param platingMaterials the given elements that should be contained in actual VecWireReceptionSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasOnlyPlatingMaterials(java.util.Collection extends VecMaterial> platingMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (platingMaterials == null) {
failWithMessage("Expecting platingMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlatingMaterials(), platingMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's platingMaterials does not contain the given VecMaterial elements.
*
* @param platingMaterials the given elements that should not be in actual VecWireReceptionSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's platingMaterials contains any given VecMaterial elements.
*/
public S doesNotHavePlatingMaterials(VecMaterial... platingMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (platingMaterials == null) failWithMessage("Expecting platingMaterials parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlatingMaterials(), platingMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's platingMaterials does not contain the given VecMaterial elements in Collection.
*
* @param platingMaterials the given elements that should not be in actual VecWireReceptionSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's platingMaterials contains any given VecMaterial elements.
*/
public S doesNotHavePlatingMaterials(java.util.Collection extends VecMaterial> platingMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (platingMaterials == null) {
failWithMessage("Expecting platingMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlatingMaterials(), platingMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification has no platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's platingMaterials is not empty.
*/
public S hasNoPlatingMaterials() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have platingMaterials but had :\n <%s>";
// check
if (actual.getPlatingMaterials().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPlatingMaterials());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireEndAccessorySpecification contains the given VecWireEndAccessorySpecification elements.
* @param refWireEndAccessorySpecification the given elements that should be contained in actual VecWireReceptionSpecification's refWireEndAccessorySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireEndAccessorySpecification does not contain all given VecWireEndAccessorySpecification elements.
*/
public S hasRefWireEndAccessorySpecification(VecWireEndAccessorySpecification... refWireEndAccessorySpecification) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessorySpecification varargs is not null.
if (refWireEndAccessorySpecification == null) failWithMessage("Expecting refWireEndAccessorySpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireEndAccessorySpecification(), refWireEndAccessorySpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireEndAccessorySpecification contains the given VecWireEndAccessorySpecification elements in Collection.
* @param refWireEndAccessorySpecification the given elements that should be contained in actual VecWireReceptionSpecification's refWireEndAccessorySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireEndAccessorySpecification does not contain all given VecWireEndAccessorySpecification elements.
*/
public S hasRefWireEndAccessorySpecification(java.util.Collection extends VecWireEndAccessorySpecification> refWireEndAccessorySpecification) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessorySpecification collection is not null.
if (refWireEndAccessorySpecification == null) {
failWithMessage("Expecting refWireEndAccessorySpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireEndAccessorySpecification(), refWireEndAccessorySpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireEndAccessorySpecification contains only the given VecWireEndAccessorySpecification elements and nothing else in whatever order.
* @param refWireEndAccessorySpecification the given elements that should be contained in actual VecWireReceptionSpecification's refWireEndAccessorySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireEndAccessorySpecification does not contain all given VecWireEndAccessorySpecification elements.
*/
public S hasOnlyRefWireEndAccessorySpecification(VecWireEndAccessorySpecification... refWireEndAccessorySpecification) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessorySpecification varargs is not null.
if (refWireEndAccessorySpecification == null) failWithMessage("Expecting refWireEndAccessorySpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireEndAccessorySpecification(), refWireEndAccessorySpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireEndAccessorySpecification contains only the given VecWireEndAccessorySpecification elements in Collection and nothing else in whatever order.
* @param refWireEndAccessorySpecification the given elements that should be contained in actual VecWireReceptionSpecification's refWireEndAccessorySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireEndAccessorySpecification does not contain all given VecWireEndAccessorySpecification elements.
*/
public S hasOnlyRefWireEndAccessorySpecification(java.util.Collection extends VecWireEndAccessorySpecification> refWireEndAccessorySpecification) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessorySpecification collection is not null.
if (refWireEndAccessorySpecification == null) {
failWithMessage("Expecting refWireEndAccessorySpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireEndAccessorySpecification(), refWireEndAccessorySpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireEndAccessorySpecification does not contain the given VecWireEndAccessorySpecification elements.
*
* @param refWireEndAccessorySpecification the given elements that should not be in actual VecWireReceptionSpecification's refWireEndAccessorySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireEndAccessorySpecification contains any given VecWireEndAccessorySpecification elements.
*/
public S doesNotHaveRefWireEndAccessorySpecification(VecWireEndAccessorySpecification... refWireEndAccessorySpecification) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessorySpecification varargs is not null.
if (refWireEndAccessorySpecification == null) failWithMessage("Expecting refWireEndAccessorySpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireEndAccessorySpecification(), refWireEndAccessorySpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireEndAccessorySpecification does not contain the given VecWireEndAccessorySpecification elements in Collection.
*
* @param refWireEndAccessorySpecification the given elements that should not be in actual VecWireReceptionSpecification's refWireEndAccessorySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireEndAccessorySpecification contains any given VecWireEndAccessorySpecification elements.
*/
public S doesNotHaveRefWireEndAccessorySpecification(java.util.Collection extends VecWireEndAccessorySpecification> refWireEndAccessorySpecification) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireEndAccessorySpecification collection is not null.
if (refWireEndAccessorySpecification == null) {
failWithMessage("Expecting refWireEndAccessorySpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireEndAccessorySpecification(), refWireEndAccessorySpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification has no refWireEndAccessorySpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireEndAccessorySpecification is not empty.
*/
public S hasNoRefWireEndAccessorySpecification() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireEndAccessorySpecification but had :\n <%s>";
// check
if (actual.getRefWireEndAccessorySpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireEndAccessorySpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireReception contains the given VecWireReception elements.
* @param refWireReception the given elements that should be contained in actual VecWireReceptionSpecification's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireReception does not contain all given VecWireReception elements.
*/
public S hasRefWireReception(VecWireReception... refWireReception) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (refWireReception == null) failWithMessage("Expecting refWireReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireReception(), refWireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireReception contains the given VecWireReception elements in Collection.
* @param refWireReception the given elements that should be contained in actual VecWireReceptionSpecification's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireReception does not contain all given VecWireReception elements.
*/
public S hasRefWireReception(java.util.Collection extends VecWireReception> refWireReception) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (refWireReception == null) {
failWithMessage("Expecting refWireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireReception(), refWireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireReception contains only the given VecWireReception elements and nothing else in whatever order.
* @param refWireReception the given elements that should be contained in actual VecWireReceptionSpecification's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireReception does not contain all given VecWireReception elements.
*/
public S hasOnlyRefWireReception(VecWireReception... refWireReception) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (refWireReception == null) failWithMessage("Expecting refWireReception parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireReception(), refWireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireReception contains only the given VecWireReception elements in Collection and nothing else in whatever order.
* @param refWireReception the given elements that should be contained in actual VecWireReceptionSpecification's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireReception does not contain all given VecWireReception elements.
*/
public S hasOnlyRefWireReception(java.util.Collection extends VecWireReception> refWireReception) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (refWireReception == null) {
failWithMessage("Expecting refWireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireReception(), refWireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireReception does not contain the given VecWireReception elements.
*
* @param refWireReception the given elements that should not be in actual VecWireReceptionSpecification's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireReception contains any given VecWireReception elements.
*/
public S doesNotHaveRefWireReception(VecWireReception... refWireReception) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception varargs is not null.
if (refWireReception == null) failWithMessage("Expecting refWireReception parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireReception(), refWireReception);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's refWireReception does not contain the given VecWireReception elements in Collection.
*
* @param refWireReception the given elements that should not be in actual VecWireReceptionSpecification's refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireReception contains any given VecWireReception elements.
*/
public S doesNotHaveRefWireReception(java.util.Collection extends VecWireReception> refWireReception) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireReception collection is not null.
if (refWireReception == null) {
failWithMessage("Expecting refWireReception parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireReception(), refWireReception.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification has no refWireReception.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's refWireReception is not empty.
*/
public S hasNoRefWireReception() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireReception but had :\n <%s>";
// check
if (actual.getRefWireReception().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireReception());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification is sealable.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification is not sealable.
*/
public S isSealable() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isSealable())) {
failWithMessage("\nExpecting that actual VecWireReceptionSpecification is sealable but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification is not sealable.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification is sealable.
*/
public S isNotSealable() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isSealable())) {
failWithMessage("\nExpecting that actual VecWireReceptionSpecification is not sealable but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's validConductorMaterials contains the given VecConductorMaterial elements.
* @param validConductorMaterials the given elements that should be contained in actual VecWireReceptionSpecification's validConductorMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's validConductorMaterials does not contain all given VecConductorMaterial elements.
*/
public S hasValidConductorMaterials(VecConductorMaterial... validConductorMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorMaterial varargs is not null.
if (validConductorMaterials == null) failWithMessage("Expecting validConductorMaterials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getValidConductorMaterials(), validConductorMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's validConductorMaterials contains the given VecConductorMaterial elements in Collection.
* @param validConductorMaterials the given elements that should be contained in actual VecWireReceptionSpecification's validConductorMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's validConductorMaterials does not contain all given VecConductorMaterial elements.
*/
public S hasValidConductorMaterials(java.util.Collection extends VecConductorMaterial> validConductorMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorMaterial collection is not null.
if (validConductorMaterials == null) {
failWithMessage("Expecting validConductorMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getValidConductorMaterials(), validConductorMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's validConductorMaterials contains only the given VecConductorMaterial elements and nothing else in whatever order.
* @param validConductorMaterials the given elements that should be contained in actual VecWireReceptionSpecification's validConductorMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's validConductorMaterials does not contain all given VecConductorMaterial elements.
*/
public S hasOnlyValidConductorMaterials(VecConductorMaterial... validConductorMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorMaterial varargs is not null.
if (validConductorMaterials == null) failWithMessage("Expecting validConductorMaterials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getValidConductorMaterials(), validConductorMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's validConductorMaterials contains only the given VecConductorMaterial elements in Collection and nothing else in whatever order.
* @param validConductorMaterials the given elements that should be contained in actual VecWireReceptionSpecification's validConductorMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's validConductorMaterials does not contain all given VecConductorMaterial elements.
*/
public S hasOnlyValidConductorMaterials(java.util.Collection extends VecConductorMaterial> validConductorMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorMaterial collection is not null.
if (validConductorMaterials == null) {
failWithMessage("Expecting validConductorMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getValidConductorMaterials(), validConductorMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's validConductorMaterials does not contain the given VecConductorMaterial elements.
*
* @param validConductorMaterials the given elements that should not be in actual VecWireReceptionSpecification's validConductorMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's validConductorMaterials contains any given VecConductorMaterial elements.
*/
public S doesNotHaveValidConductorMaterials(VecConductorMaterial... validConductorMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorMaterial varargs is not null.
if (validConductorMaterials == null) failWithMessage("Expecting validConductorMaterials parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getValidConductorMaterials(), validConductorMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's validConductorMaterials does not contain the given VecConductorMaterial elements in Collection.
*
* @param validConductorMaterials the given elements that should not be in actual VecWireReceptionSpecification's validConductorMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's validConductorMaterials contains any given VecConductorMaterial elements.
*/
public S doesNotHaveValidConductorMaterials(java.util.Collection extends VecConductorMaterial> validConductorMaterials) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorMaterial collection is not null.
if (validConductorMaterials == null) {
failWithMessage("Expecting validConductorMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getValidConductorMaterials(), validConductorMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification has no validConductorMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecWireReceptionSpecification's validConductorMaterials is not empty.
*/
public S hasNoValidConductorMaterials() {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have validConductorMaterials but had :\n <%s>";
// check
if (actual.getValidConductorMaterials().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getValidConductorMaterials());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's wireElementOutsideDiameter is equal to the given one.
* @param wireElementOutsideDiameter the given wireElementOutsideDiameter to compare the actual VecWireReceptionSpecification's wireElementOutsideDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification's wireElementOutsideDiameter is not equal to the given one.
*/
public S hasWireElementOutsideDiameter(VecValueRange wireElementOutsideDiameter) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting wireElementOutsideDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecValueRange actualWireElementOutsideDiameter = actual.getWireElementOutsideDiameter();
if (!Objects.areEqual(actualWireElementOutsideDiameter, wireElementOutsideDiameter)) {
failWithMessage(assertjErrorMessage, actual, wireElementOutsideDiameter, actualWireElementOutsideDiameter);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecWireReceptionSpecification's wireReceptionType is equal to the given one.
* @param wireReceptionType the given wireReceptionType to compare the actual VecWireReceptionSpecification's wireReceptionType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecWireReceptionSpecification's wireReceptionType is not equal to the given one.
*/
public S hasWireReceptionType(String wireReceptionType) {
// check that actual VecWireReceptionSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting wireReceptionType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualWireReceptionType = actual.getWireReceptionType();
if (!Objects.areEqual(actualWireReceptionType, wireReceptionType)) {
failWithMessage(assertjErrorMessage, actual, wireReceptionType, actualWireReceptionType);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy