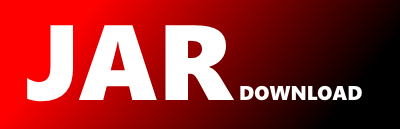
com.foursoft.vecmodel.vec120.assertions.Assertions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120.assertions;
/**
* Entry point for assertions of different data types. Each method in this class is a static factory for the
* type-specific assertion objects.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public class Assertions {
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAbstractLocalizedStringAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAbstractLocalizedStringAssert assertThat(com.foursoft.vecmodel.vec120.VecAbstractLocalizedString actual) {
return new com.foursoft.vecmodel.vec120.VecAbstractLocalizedStringAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAbstractSlotAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAbstractSlotAssert assertThat(com.foursoft.vecmodel.vec120.VecAbstractSlot actual) {
return new com.foursoft.vecmodel.vec120.VecAbstractSlotAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAbstractSlotReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAbstractSlotReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecAbstractSlotReference actual) {
return new com.foursoft.vecmodel.vec120.VecAbstractSlotReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAliasIdentificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAliasIdentificationAssert assertThat(com.foursoft.vecmodel.vec120.VecAliasIdentification actual) {
return new com.foursoft.vecmodel.vec120.VecAliasIdentificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAnchorTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAnchorTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecAnchorType actual) {
return new com.foursoft.vecmodel.vec120.VecAnchorTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAntennaSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAntennaSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecAntennaSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecAntennaSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecApplicationConstraintAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecApplicationConstraintAssert assertThat(com.foursoft.vecmodel.vec120.VecApplicationConstraint actual) {
return new com.foursoft.vecmodel.vec120.VecApplicationConstraintAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecApplicationConstraintSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecApplicationConstraintSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecApplicationConstraintSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecApplicationConstraintSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecApplicationConstraintTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecApplicationConstraintTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecApplicationConstraintType actual) {
return new com.foursoft.vecmodel.vec120.VecApplicationConstraintTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecApprovalAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecApprovalAssert assertThat(com.foursoft.vecmodel.vec120.VecApproval actual) {
return new com.foursoft.vecmodel.vec120.VecApprovalAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAssignmentGroupAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAssignmentGroupAssert assertThat(com.foursoft.vecmodel.vec120.VecAssignmentGroup actual) {
return new com.foursoft.vecmodel.vec120.VecAssignmentGroupAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecAssignmentGroupSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecAssignmentGroupSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecAssignmentGroupSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecAssignmentGroupSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBaselineSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBaselineSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecBaselineSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecBaselineSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBatterySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBatterySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecBatterySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecBatterySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBoltMountedFixingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBoltMountedFixingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecBoltMountedFixingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecBoltMountedFixingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBoltTerminalRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBoltTerminalRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecBoltTerminalRole actual) {
return new com.foursoft.vecmodel.vec120.VecBoltTerminalRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBoltTerminalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBoltTerminalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecBoltTerminalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecBoltTerminalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBooleanValuePropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBooleanValuePropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecBooleanValueProperty actual) {
return new com.foursoft.vecmodel.vec120.VecBooleanValuePropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBoundingBoxAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBoundingBoxAssert assertThat(com.foursoft.vecmodel.vec120.VecBoundingBox actual) {
return new com.foursoft.vecmodel.vec120.VecBoundingBoxAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBridgeTerminalRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBridgeTerminalRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecBridgeTerminalRole actual) {
return new com.foursoft.vecmodel.vec120.VecBridgeTerminalRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBridgeTerminalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBridgeTerminalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecBridgeTerminalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecBridgeTerminalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning2DAssert assertThat(com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning2D actual) {
return new com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning3DAssert assertThat(com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning3D actual) {
return new com.foursoft.vecmodel.vec120.VecBuildingBlockPositioning3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification2DAssert assertThat(com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification2D actual) {
return new com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification3DAssert assertThat(com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification3D actual) {
return new com.foursoft.vecmodel.vec120.VecBuildingBlockSpecification3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableDuctOutletAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableDuctOutletAssert assertThat(com.foursoft.vecmodel.vec120.VecCableDuctOutlet actual) {
return new com.foursoft.vecmodel.vec120.VecCableDuctOutletAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableDuctRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableDuctRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecCableDuctRole actual) {
return new com.foursoft.vecmodel.vec120.VecCableDuctRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableDuctSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableDuctSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCableDuctSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCableDuctSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableLeadThroughAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableLeadThroughAssert assertThat(com.foursoft.vecmodel.vec120.VecCableLeadThrough actual) {
return new com.foursoft.vecmodel.vec120.VecCableLeadThroughAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableLeadThroughReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableLeadThroughReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecCableLeadThroughReference actual) {
return new com.foursoft.vecmodel.vec120.VecCableLeadThroughReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableLeadThroughSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableLeadThroughSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCableLeadThroughSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCableLeadThroughSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableTieRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableTieRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecCableTieRole actual) {
return new com.foursoft.vecmodel.vec120.VecCableTieRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCableTieSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCableTieSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCableTieSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCableTieSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCapacitorSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCapacitorSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCapacitorSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCapacitorSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCartesianDimensionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCartesianDimensionAssert assertThat(com.foursoft.vecmodel.vec120.VecCartesianDimension actual) {
return new com.foursoft.vecmodel.vec120.VecCartesianDimensionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCartesianPoint2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCartesianPoint2DAssert assertThat(com.foursoft.vecmodel.vec120.VecCartesianPoint2D actual) {
return new com.foursoft.vecmodel.vec120.VecCartesianPoint2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCartesianPoint3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCartesianPoint3DAssert assertThat(com.foursoft.vecmodel.vec120.VecCartesianPoint3D actual) {
return new com.foursoft.vecmodel.vec120.VecCartesianPoint3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCartesianVectorAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCartesianVectorAssert assertThat(com.foursoft.vecmodel.vec120.VecCartesianVector actual) {
return new com.foursoft.vecmodel.vec120.VecCartesianVectorAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCartesianVector2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCartesianVector2DAssert assertThat(com.foursoft.vecmodel.vec120.VecCartesianVector2D actual) {
return new com.foursoft.vecmodel.vec120.VecCartesianVector2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCartesianVector3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCartesianVector3DAssert assertThat(com.foursoft.vecmodel.vec120.VecCartesianVector3D actual) {
return new com.foursoft.vecmodel.vec120.VecCartesianVector3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityAssert assertThat(com.foursoft.vecmodel.vec120.VecCavity actual) {
return new com.foursoft.vecmodel.vec120.VecCavityAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityAccessoryRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityAccessoryRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityAccessoryRole actual) {
return new com.foursoft.vecmodel.vec120.VecCavityAccessoryRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityAccessorySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityAccessorySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityAccessorySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCavityAccessorySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityAddOnAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityAddOnAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityAddOn actual) {
return new com.foursoft.vecmodel.vec120.VecCavityAddOnAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityCouplingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityCouplingAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityCoupling actual) {
return new com.foursoft.vecmodel.vec120.VecCavityCouplingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityMappingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityMappingAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityMapping actual) {
return new com.foursoft.vecmodel.vec120.VecCavityMappingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityMountingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityMountingAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityMounting actual) {
return new com.foursoft.vecmodel.vec120.VecCavityMountingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityMountingDetailAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityMountingDetailAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityMountingDetail actual) {
return new com.foursoft.vecmodel.vec120.VecCavityMountingDetailAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityPartSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityPartSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityPartSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCavityPartSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityPlugRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityPlugRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityPlugRole actual) {
return new com.foursoft.vecmodel.vec120.VecCavityPlugRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityPlugSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityPlugSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityPlugSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCavityPlugSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavityReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavityReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecCavityReference actual) {
return new com.foursoft.vecmodel.vec120.VecCavityReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavitySealRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavitySealRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecCavitySealRole actual) {
return new com.foursoft.vecmodel.vec120.VecCavitySealRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavitySealSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavitySealSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCavitySealSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCavitySealSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCavitySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCavitySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCavitySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCavitySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecChangeDescriptionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecChangeDescriptionAssert assertThat(com.foursoft.vecmodel.vec120.VecChangeDescription actual) {
return new com.foursoft.vecmodel.vec120.VecChangeDescriptionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCodingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCodingAssert assertThat(com.foursoft.vecmodel.vec120.VecCoding actual) {
return new com.foursoft.vecmodel.vec120.VecCodingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecColorAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecColorAssert assertThat(com.foursoft.vecmodel.vec120.VecColor actual) {
return new com.foursoft.vecmodel.vec120.VecColorAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecComplexPropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecComplexPropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecComplexProperty actual) {
return new com.foursoft.vecmodel.vec120.VecComplexPropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecComponentConnectorAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecComponentConnectorAssert assertThat(com.foursoft.vecmodel.vec120.VecComponentConnector actual) {
return new com.foursoft.vecmodel.vec120.VecComponentConnectorAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecComponentNodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecComponentNodeAssert assertThat(com.foursoft.vecmodel.vec120.VecComponentNode actual) {
return new com.foursoft.vecmodel.vec120.VecComponentNodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecComponentPortAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecComponentPortAssert assertThat(com.foursoft.vecmodel.vec120.VecComponentPort actual) {
return new com.foursoft.vecmodel.vec120.VecComponentPortAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCompositeUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCompositeUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecCompositeUnit actual) {
return new com.foursoft.vecmodel.vec120.VecCompositeUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCompositionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCompositionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCompositionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCompositionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConductorCurrentInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConductorCurrentInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecConductorCurrentInformation actual) {
return new com.foursoft.vecmodel.vec120.VecConductorCurrentInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConductorMaterialAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConductorMaterialAssert assertThat(com.foursoft.vecmodel.vec120.VecConductorMaterial actual) {
return new com.foursoft.vecmodel.vec120.VecConductorMaterialAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConductorSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConductorSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecConductorSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecConductorSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConfigurableElementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConfigurableElementAssert assertThat(com.foursoft.vecmodel.vec120.VecConfigurableElement actual) {
return new com.foursoft.vecmodel.vec120.VecConfigurableElementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectionAssert assertThat(com.foursoft.vecmodel.vec120.VecConnection actual) {
return new com.foursoft.vecmodel.vec120.VecConnectionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectionEndAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectionEndAssert assertThat(com.foursoft.vecmodel.vec120.VecConnectionEnd actual) {
return new com.foursoft.vecmodel.vec120.VecConnectionEndAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectionGroupAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectionGroupAssert assertThat(com.foursoft.vecmodel.vec120.VecConnectionGroup actual) {
return new com.foursoft.vecmodel.vec120.VecConnectionGroupAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecConnectionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecConnectionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectorHousingCapRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectorHousingCapRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecConnectorHousingCapRole actual) {
return new com.foursoft.vecmodel.vec120.VecConnectorHousingCapRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectorHousingCapSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectorHousingCapSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecConnectorHousingCapSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecConnectorHousingCapSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectorHousingRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectorHousingRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecConnectorHousingRole actual) {
return new com.foursoft.vecmodel.vec120.VecConnectorHousingRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecConnectorHousingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecConnectorHousingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecConnectorHousingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecConnectorHousingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecContactPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecContactPointAssert assertThat(com.foursoft.vecmodel.vec120.VecContactPoint actual) {
return new com.foursoft.vecmodel.vec120.VecContactPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecContactingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecContactingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecContactingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecContactingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecContentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecContentAssert assertThat(com.foursoft.vecmodel.vec120.VecContent actual) {
return new com.foursoft.vecmodel.vec120.VecContentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecContractAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecContractAssert assertThat(com.foursoft.vecmodel.vec120.VecContract actual) {
return new com.foursoft.vecmodel.vec120.VecContractAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCopyrightInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCopyrightInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecCopyrightInformation actual) {
return new com.foursoft.vecmodel.vec120.VecCopyrightInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCoreSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCoreSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCoreSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCoreSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCorrugatedPipeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCorrugatedPipeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCorrugatedPipeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCorrugatedPipeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCouplingPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCouplingPointAssert assertThat(com.foursoft.vecmodel.vec120.VecCouplingPoint actual) {
return new com.foursoft.vecmodel.vec120.VecCouplingPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCouplingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCouplingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecCouplingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecCouplingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCreationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCreationAssert assertThat(com.foursoft.vecmodel.vec120.VecCreation actual) {
return new com.foursoft.vecmodel.vec120.VecCreationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCurve3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCurve3DAssert assertThat(com.foursoft.vecmodel.vec120.VecCurve3D actual) {
return new com.foursoft.vecmodel.vec120.VecCurve3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCustomPropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCustomPropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecCustomProperty actual) {
return new com.foursoft.vecmodel.vec120.VecCustomPropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecCustomUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecCustomUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecCustomUnit actual) {
return new com.foursoft.vecmodel.vec120.VecCustomUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDateValuePropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDateValuePropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecDateValueProperty actual) {
return new com.foursoft.vecmodel.vec120.VecDateValuePropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDefaultDimensionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDefaultDimensionAssert assertThat(com.foursoft.vecmodel.vec120.VecDefaultDimension actual) {
return new com.foursoft.vecmodel.vec120.VecDefaultDimensionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDefaultDimensionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDefaultDimensionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecDefaultDimensionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecDefaultDimensionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDimensionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDimensionAssert assertThat(com.foursoft.vecmodel.vec120.VecDimension actual) {
return new com.foursoft.vecmodel.vec120.VecDimensionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDimensionAnchorAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDimensionAnchorAssert assertThat(com.foursoft.vecmodel.vec120.VecDimensionAnchor actual) {
return new com.foursoft.vecmodel.vec120.VecDimensionAnchorAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDiodeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDiodeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecDiodeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecDiodeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDocumentBasedInstructionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDocumentBasedInstructionAssert assertThat(com.foursoft.vecmodel.vec120.VecDocumentBasedInstruction actual) {
return new com.foursoft.vecmodel.vec120.VecDocumentBasedInstructionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDocumentRelatedAssignmentGroupAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDocumentRelatedAssignmentGroupAssert assertThat(com.foursoft.vecmodel.vec120.VecDocumentRelatedAssignmentGroup actual) {
return new com.foursoft.vecmodel.vec120.VecDocumentRelatedAssignmentGroupAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDocumentVersionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDocumentVersionAssert assertThat(com.foursoft.vecmodel.vec120.VecDocumentVersion actual) {
return new com.foursoft.vecmodel.vec120.VecDocumentVersionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecDoubleValuePropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecDoubleValuePropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecDoubleValueProperty actual) {
return new com.foursoft.vecmodel.vec120.VecDoubleValuePropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecEEComponentRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecEEComponentRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecEEComponentRole actual) {
return new com.foursoft.vecmodel.vec120.VecEEComponentRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecEEComponentSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecEEComponentSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecEEComponentSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecEEComponentSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecEdgeMountedFixingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecEdgeMountedFixingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecEdgeMountedFixingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecEdgeMountedFixingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecExtendableElementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecExtendableElementAssert assertThat(com.foursoft.vecmodel.vec120.VecExtendableElement actual) {
return new com.foursoft.vecmodel.vec120.VecExtendableElementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecExtensionSlotAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecExtensionSlotAssert assertThat(com.foursoft.vecmodel.vec120.VecExtensionSlot actual) {
return new com.foursoft.vecmodel.vec120.VecExtensionSlotAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecExtensionSlotReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecExtensionSlotReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecExtensionSlotReference actual) {
return new com.foursoft.vecmodel.vec120.VecExtensionSlotReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecExternalMappingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecExternalMappingAssert assertThat(com.foursoft.vecmodel.vec120.VecExternalMapping actual) {
return new com.foursoft.vecmodel.vec120.VecExternalMappingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecExternalMappingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecExternalMappingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecExternalMappingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecExternalMappingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFerriteRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFerriteRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecFerriteRole actual) {
return new com.foursoft.vecmodel.vec120.VecFerriteRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFerriteSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFerriteSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecFerriteSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecFerriteSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFileBasedInstructionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFileBasedInstructionAssert assertThat(com.foursoft.vecmodel.vec120.VecFileBasedInstruction actual) {
return new com.foursoft.vecmodel.vec120.VecFileBasedInstructionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFillerSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFillerSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecFillerSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecFillerSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFittingOutletAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFittingOutletAssert assertThat(com.foursoft.vecmodel.vec120.VecFittingOutlet actual) {
return new com.foursoft.vecmodel.vec120.VecFittingOutletAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFittingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFittingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecFittingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecFittingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFixingRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFixingRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecFixingRole actual) {
return new com.foursoft.vecmodel.vec120.VecFixingRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFixingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFixingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecFixingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecFixingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFlatCoreSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFlatCoreSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecFlatCoreSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecFlatCoreSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFunctionalAssignmentGroupAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFunctionalAssignmentGroupAssert assertThat(com.foursoft.vecmodel.vec120.VecFunctionalAssignmentGroup actual) {
return new com.foursoft.vecmodel.vec120.VecFunctionalAssignmentGroupAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFunctionalRequirementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFunctionalRequirementAssert assertThat(com.foursoft.vecmodel.vec120.VecFunctionalRequirement actual) {
return new com.foursoft.vecmodel.vec120.VecFunctionalRequirementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFuseComponentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFuseComponentAssert assertThat(com.foursoft.vecmodel.vec120.VecFuseComponent actual) {
return new com.foursoft.vecmodel.vec120.VecFuseComponentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecFuseSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecFuseSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecFuseSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecFuseSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGeneralTechnicalPartSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGeneralTechnicalPartSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecGeneralTechnicalPartSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecGeneralTechnicalPartSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGeometryNodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGeometryNodeAssert assertThat(com.foursoft.vecmodel.vec120.VecGeometryNode actual) {
return new com.foursoft.vecmodel.vec120.VecGeometryNodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGeometryNode2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGeometryNode2DAssert assertThat(com.foursoft.vecmodel.vec120.VecGeometryNode2D actual) {
return new com.foursoft.vecmodel.vec120.VecGeometryNode2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGeometryNode3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGeometryNode3DAssert assertThat(com.foursoft.vecmodel.vec120.VecGeometryNode3D actual) {
return new com.foursoft.vecmodel.vec120.VecGeometryNode3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGeometrySegmentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGeometrySegmentAssert assertThat(com.foursoft.vecmodel.vec120.VecGeometrySegment actual) {
return new com.foursoft.vecmodel.vec120.VecGeometrySegmentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGeometrySegment2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGeometrySegment2DAssert assertThat(com.foursoft.vecmodel.vec120.VecGeometrySegment2D actual) {
return new com.foursoft.vecmodel.vec120.VecGeometrySegment2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGeometrySegment3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGeometrySegment3DAssert assertThat(com.foursoft.vecmodel.vec120.VecGeometrySegment3D actual) {
return new com.foursoft.vecmodel.vec120.VecGeometrySegment3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGrommetRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGrommetRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecGrommetRole actual) {
return new com.foursoft.vecmodel.vec120.VecGrommetRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecGrommetSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecGrommetSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecGrommetSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecGrommetSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecHarnessDrawingSpecification2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecHarnessDrawingSpecification2DAssert assertThat(com.foursoft.vecmodel.vec120.VecHarnessDrawingSpecification2D actual) {
return new com.foursoft.vecmodel.vec120.VecHarnessDrawingSpecification2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecHarnessGeometrySpecification3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecHarnessGeometrySpecification3DAssert assertThat(com.foursoft.vecmodel.vec120.VecHarnessGeometrySpecification3D actual) {
return new com.foursoft.vecmodel.vec120.VecHarnessGeometrySpecification3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecHistoryEntryTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecHistoryEntryTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecHistoryEntryType actual) {
return new com.foursoft.vecmodel.vec120.VecHistoryEntryTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecHoleMountedFixingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecHoleMountedFixingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecHoleMountedFixingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecHoleMountedFixingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecHousingComponentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecHousingComponentAssert assertThat(com.foursoft.vecmodel.vec120.VecHousingComponent actual) {
return new com.foursoft.vecmodel.vec120.VecHousingComponentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecHousingComponentReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecHousingComponentReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecHousingComponentReference actual) {
return new com.foursoft.vecmodel.vec120.VecHousingComponentReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecIECPrefixAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecIECPrefixAssert assertThat(com.foursoft.vecmodel.vec120.VecIECPrefix actual) {
return new com.foursoft.vecmodel.vec120.VecIECPrefixAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecIECUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecIECUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecIECUnit actual) {
return new com.foursoft.vecmodel.vec120.VecIECUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecIECUnitNameAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecIECUnitNameAssert assertThat(com.foursoft.vecmodel.vec120.VecIECUnitName actual) {
return new com.foursoft.vecmodel.vec120.VecIECUnitNameAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecImperialUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecImperialUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecImperialUnit actual) {
return new com.foursoft.vecmodel.vec120.VecImperialUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecImperialUnitNameAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecImperialUnitNameAssert assertThat(com.foursoft.vecmodel.vec120.VecImperialUnitName actual) {
return new com.foursoft.vecmodel.vec120.VecImperialUnitNameAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecInstructionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecInstructionAssert assertThat(com.foursoft.vecmodel.vec120.VecInstruction actual) {
return new com.foursoft.vecmodel.vec120.VecInstructionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecInsulationSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecInsulationSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecInsulationSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecInsulationSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecIntegerValuePropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecIntegerValuePropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecIntegerValueProperty actual) {
return new com.foursoft.vecmodel.vec120.VecIntegerValuePropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecInternalComponentConnectionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecInternalComponentConnectionAssert assertThat(com.foursoft.vecmodel.vec120.VecInternalComponentConnection actual) {
return new com.foursoft.vecmodel.vec120.VecInternalComponentConnectionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecInternalTerminalConnectionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecInternalTerminalConnectionAssert assertThat(com.foursoft.vecmodel.vec120.VecInternalTerminalConnection actual) {
return new com.foursoft.vecmodel.vec120.VecInternalTerminalConnectionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecItemEquivalenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecItemEquivalenceAssert assertThat(com.foursoft.vecmodel.vec120.VecItemEquivalence actual) {
return new com.foursoft.vecmodel.vec120.VecItemEquivalenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecItemHistoryEntryAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecItemHistoryEntryAssert assertThat(com.foursoft.vecmodel.vec120.VecItemHistoryEntry actual) {
return new com.foursoft.vecmodel.vec120.VecItemHistoryEntryAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecItemVersionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecItemVersionAssert assertThat(com.foursoft.vecmodel.vec120.VecItemVersion actual) {
return new com.foursoft.vecmodel.vec120.VecItemVersionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecLanguageCodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecLanguageCodeAssert assertThat(com.foursoft.vecmodel.vec120.VecLanguageCode actual) {
return new com.foursoft.vecmodel.vec120.VecLanguageCodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecLocalGeometrySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecLocalGeometrySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecLocalGeometrySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecLocalGeometrySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecLocalPositionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecLocalPositionAssert assertThat(com.foursoft.vecmodel.vec120.VecLocalPosition actual) {
return new com.foursoft.vecmodel.vec120.VecLocalPositionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecLocalizedStringAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecLocalizedStringAssert assertThat(com.foursoft.vecmodel.vec120.VecLocalizedString actual) {
return new com.foursoft.vecmodel.vec120.VecLocalizedStringAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecLocalizedStringPropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecLocalizedStringPropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecLocalizedStringProperty actual) {
return new com.foursoft.vecmodel.vec120.VecLocalizedStringPropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecLocalizedTypedStringAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecLocalizedTypedStringAssert assertThat(com.foursoft.vecmodel.vec120.VecLocalizedTypedString actual) {
return new com.foursoft.vecmodel.vec120.VecLocalizedTypedStringAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecLocationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecLocationAssert assertThat(com.foursoft.vecmodel.vec120.VecLocation actual) {
return new com.foursoft.vecmodel.vec120.VecLocationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMappingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMappingAssert assertThat(com.foursoft.vecmodel.vec120.VecMapping actual) {
return new com.foursoft.vecmodel.vec120.VecMappingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMappingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMappingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecMappingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecMappingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMassInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMassInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecMassInformation actual) {
return new com.foursoft.vecmodel.vec120.VecMassInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMaterialAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMaterialAssert assertThat(com.foursoft.vecmodel.vec120.VecMaterial actual) {
return new com.foursoft.vecmodel.vec120.VecMaterialAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMatingDetailAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMatingDetailAssert assertThat(com.foursoft.vecmodel.vec120.VecMatingDetail actual) {
return new com.foursoft.vecmodel.vec120.VecMatingDetailAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMatingPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMatingPointAssert assertThat(com.foursoft.vecmodel.vec120.VecMatingPoint actual) {
return new com.foursoft.vecmodel.vec120.VecMatingPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMeasurePointPositionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMeasurePointPositionAssert assertThat(com.foursoft.vecmodel.vec120.VecMeasurePointPosition actual) {
return new com.foursoft.vecmodel.vec120.VecMeasurePointPositionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMeasurementPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMeasurementPointAssert assertThat(com.foursoft.vecmodel.vec120.VecMeasurementPoint actual) {
return new com.foursoft.vecmodel.vec120.VecMeasurementPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMeasurementPointReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMeasurementPointReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecMeasurementPointReference actual) {
return new com.foursoft.vecmodel.vec120.VecMeasurementPointReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecModularSlotAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecModularSlotAssert assertThat(com.foursoft.vecmodel.vec120.VecModularSlot actual) {
return new com.foursoft.vecmodel.vec120.VecModularSlotAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecModularSlotAddOnAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecModularSlotAddOnAssert assertThat(com.foursoft.vecmodel.vec120.VecModularSlotAddOn actual) {
return new com.foursoft.vecmodel.vec120.VecModularSlotAddOnAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecModularSlotReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecModularSlotReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecModularSlotReference actual) {
return new com.foursoft.vecmodel.vec120.VecModularSlotReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecModuleFamilyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecModuleFamilyAssert assertThat(com.foursoft.vecmodel.vec120.VecModuleFamily actual) {
return new com.foursoft.vecmodel.vec120.VecModuleFamilyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecModuleFamilySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecModuleFamilySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecModuleFamilySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecModuleFamilySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecModuleListAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecModuleListAssert assertThat(com.foursoft.vecmodel.vec120.VecModuleList actual) {
return new com.foursoft.vecmodel.vec120.VecModuleListAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecModuleListSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecModuleListSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecModuleListSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecModuleListSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMultiCavityPlugSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMultiCavityPlugSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecMultiCavityPlugSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecMultiCavityPlugSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMultiCavitySealSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMultiCavitySealSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecMultiCavitySealSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecMultiCavitySealSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecMultiFuseSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecMultiFuseSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecMultiFuseSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecMultiFuseSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNURBSControlPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNURBSControlPointAssert assertThat(com.foursoft.vecmodel.vec120.VecNURBSControlPoint actual) {
return new com.foursoft.vecmodel.vec120.VecNURBSControlPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNURBSCurveAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNURBSCurveAssert assertThat(com.foursoft.vecmodel.vec120.VecNURBSCurve actual) {
return new com.foursoft.vecmodel.vec120.VecNURBSCurveAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNetAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNetAssert assertThat(com.foursoft.vecmodel.vec120.VecNet actual) {
return new com.foursoft.vecmodel.vec120.VecNetAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNetGroupAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNetGroupAssert assertThat(com.foursoft.vecmodel.vec120.VecNetGroup actual) {
return new com.foursoft.vecmodel.vec120.VecNetGroupAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNetSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNetSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecNetSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecNetSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNetTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNetTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecNetType actual) {
return new com.foursoft.vecmodel.vec120.VecNetTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNetworkNodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNetworkNodeAssert assertThat(com.foursoft.vecmodel.vec120.VecNetworkNode actual) {
return new com.foursoft.vecmodel.vec120.VecNetworkNodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNetworkPortAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNetworkPortAssert assertThat(com.foursoft.vecmodel.vec120.VecNetworkPort actual) {
return new com.foursoft.vecmodel.vec120.VecNetworkPortAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNodeLocationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNodeLocationAssert assertThat(com.foursoft.vecmodel.vec120.VecNodeLocation actual) {
return new com.foursoft.vecmodel.vec120.VecNodeLocationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNodeMappingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNodeMappingAssert assertThat(com.foursoft.vecmodel.vec120.VecNodeMapping actual) {
return new com.foursoft.vecmodel.vec120.VecNodeMappingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNumericalValueAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNumericalValueAssert assertThat(com.foursoft.vecmodel.vec120.VecNumericalValue actual) {
return new com.foursoft.vecmodel.vec120.VecNumericalValueAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecNumericalValuePropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecNumericalValuePropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecNumericalValueProperty actual) {
return new com.foursoft.vecmodel.vec120.VecNumericalValuePropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageAssert assertThat(com.foursoft.vecmodel.vec120.VecOccurrenceOrUsage actual) {
return new com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem2DAssert assertThat(com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem2D actual) {
return new com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem3DAssert assertThat(com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem3D actual) {
return new com.foursoft.vecmodel.vec120.VecOccurrenceOrUsageViewItem3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOnPointPlacementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOnPointPlacementAssert assertThat(com.foursoft.vecmodel.vec120.VecOnPointPlacement actual) {
return new com.foursoft.vecmodel.vec120.VecOnPointPlacementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOnWayPlacementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOnWayPlacementAssert assertThat(com.foursoft.vecmodel.vec120.VecOnWayPlacement actual) {
return new com.foursoft.vecmodel.vec120.VecOnWayPlacementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOpenCavitiesAssignmentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOpenCavitiesAssignmentAssert assertThat(com.foursoft.vecmodel.vec120.VecOpenCavitiesAssignment actual) {
return new com.foursoft.vecmodel.vec120.VecOpenCavitiesAssignmentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalRole actual) {
return new com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecOpenWireEndTerminalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOtherUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOtherUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecOtherUnit actual) {
return new com.foursoft.vecmodel.vec120.VecOtherUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecOtherUnitNameAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecOtherUnitNameAssert assertThat(com.foursoft.vecmodel.vec120.VecOtherUnitName actual) {
return new com.foursoft.vecmodel.vec120.VecOtherUnitNameAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartOccurrenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartOccurrenceAssert assertThat(com.foursoft.vecmodel.vec120.VecPartOccurrence actual) {
return new com.foursoft.vecmodel.vec120.VecPartOccurrenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartOrUsageRelatedSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartOrUsageRelatedSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPartOrUsageRelatedSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPartOrUsageRelatedSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartRelationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartRelationAssert assertThat(com.foursoft.vecmodel.vec120.VecPartRelation actual) {
return new com.foursoft.vecmodel.vec120.VecPartRelationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartRelationTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartRelationTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecPartRelationType actual) {
return new com.foursoft.vecmodel.vec120.VecPartRelationTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartStructureSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartStructureSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPartStructureSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPartStructureSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartSubstitutionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartSubstitutionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPartSubstitutionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPartSubstitutionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartUsageAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartUsageAssert assertThat(com.foursoft.vecmodel.vec120.VecPartUsage actual) {
return new com.foursoft.vecmodel.vec120.VecPartUsageAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartUsageSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartUsageSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPartUsageSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPartUsageSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartVersionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartVersionAssert assertThat(com.foursoft.vecmodel.vec120.VecPartVersion actual) {
return new com.foursoft.vecmodel.vec120.VecPartVersionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPartWithSubComponentsRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPartWithSubComponentsRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecPartWithSubComponentsRole actual) {
return new com.foursoft.vecmodel.vec120.VecPartWithSubComponentsRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPathAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPathAssert assertThat(com.foursoft.vecmodel.vec120.VecPath actual) {
return new com.foursoft.vecmodel.vec120.VecPathAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPathSegmentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPathSegmentAssert assertThat(com.foursoft.vecmodel.vec120.VecPathSegment actual) {
return new com.foursoft.vecmodel.vec120.VecPathSegmentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPermissionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPermissionAssert assertThat(com.foursoft.vecmodel.vec120.VecPermission actual) {
return new com.foursoft.vecmodel.vec120.VecPermissionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPersonAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPersonAssert assertThat(com.foursoft.vecmodel.vec120.VecPerson actual) {
return new com.foursoft.vecmodel.vec120.VecPersonAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinComponentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinComponentAssert assertThat(com.foursoft.vecmodel.vec120.VecPinComponent actual) {
return new com.foursoft.vecmodel.vec120.VecPinComponentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinComponentBehaviorAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinComponentBehaviorAssert assertThat(com.foursoft.vecmodel.vec120.VecPinComponentBehavior actual) {
return new com.foursoft.vecmodel.vec120.VecPinComponentBehaviorAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinComponentReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinComponentReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecPinComponentReference actual) {
return new com.foursoft.vecmodel.vec120.VecPinComponentReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinCurrentInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinCurrentInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecPinCurrentInformation actual) {
return new com.foursoft.vecmodel.vec120.VecPinCurrentInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinOpticalInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinOpticalInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecPinOpticalInformation actual) {
return new com.foursoft.vecmodel.vec120.VecPinOpticalInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinTimingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinTimingAssert assertThat(com.foursoft.vecmodel.vec120.VecPinTiming actual) {
return new com.foursoft.vecmodel.vec120.VecPinTimingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinVoltageInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinVoltageInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecPinVoltageInformation actual) {
return new com.foursoft.vecmodel.vec120.VecPinVoltageInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinWireMappingPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinWireMappingPointAssert assertThat(com.foursoft.vecmodel.vec120.VecPinWireMappingPoint actual) {
return new com.foursoft.vecmodel.vec120.VecPinWireMappingPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPinWireMappingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPinWireMappingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPinWireMappingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPinWireMappingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlaceableElementRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlaceableElementRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecPlaceableElementRole actual) {
return new com.foursoft.vecmodel.vec120.VecPlaceableElementRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlaceableElementSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlaceableElementSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPlaceableElementSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPlaceableElementSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlacementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlacementAssert assertThat(com.foursoft.vecmodel.vec120.VecPlacement actual) {
return new com.foursoft.vecmodel.vec120.VecPlacementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlacementPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlacementPointAssert assertThat(com.foursoft.vecmodel.vec120.VecPlacementPoint actual) {
return new com.foursoft.vecmodel.vec120.VecPlacementPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlacementPointPositionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlacementPointPositionAssert assertThat(com.foursoft.vecmodel.vec120.VecPlacementPointPosition actual) {
return new com.foursoft.vecmodel.vec120.VecPlacementPointPositionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlacementPointReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlacementPointReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecPlacementPointReference actual) {
return new com.foursoft.vecmodel.vec120.VecPlacementPointReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlacementSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlacementSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPlacementSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPlacementSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPlacementTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPlacementTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecPlacementType actual) {
return new com.foursoft.vecmodel.vec120.VecPlacementTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPluggableTerminalRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPluggableTerminalRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecPluggableTerminalRole actual) {
return new com.foursoft.vecmodel.vec120.VecPluggableTerminalRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPluggableTerminalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPluggableTerminalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPluggableTerminalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPluggableTerminalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPotentialDistributorSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPotentialDistributorSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecPotentialDistributorSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecPotentialDistributorSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPowerConsumptionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPowerConsumptionAssert assertThat(com.foursoft.vecmodel.vec120.VecPowerConsumption actual) {
return new com.foursoft.vecmodel.vec120.VecPowerConsumptionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecPrimaryPartTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecPrimaryPartTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecPrimaryPartType actual) {
return new com.foursoft.vecmodel.vec120.VecPrimaryPartTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecProjectAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecProjectAssert assertThat(com.foursoft.vecmodel.vec120.VecProject actual) {
return new com.foursoft.vecmodel.vec120.VecProjectAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecReaderAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecReaderAssert assertThat(com.foursoft.vecmodel.vec120.VecReader actual) {
return new com.foursoft.vecmodel.vec120.VecReaderAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRelaySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRelaySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecRelaySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecRelaySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRequirementsConformanceSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRequirementsConformanceSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecRequirementsConformanceSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecRequirementsConformanceSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRequirementsConformanceStatementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRequirementsConformanceStatementAssert assertThat(com.foursoft.vecmodel.vec120.VecRequirementsConformanceStatement actual) {
return new com.foursoft.vecmodel.vec120.VecRequirementsConformanceStatementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRingTerminalRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRingTerminalRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecRingTerminalRole actual) {
return new com.foursoft.vecmodel.vec120.VecRingTerminalRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRingTerminalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRingTerminalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecRingTerminalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecRingTerminalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRobustnessPropertiesAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRobustnessPropertiesAssert assertThat(com.foursoft.vecmodel.vec120.VecRobustnessProperties actual) {
return new com.foursoft.vecmodel.vec120.VecRobustnessPropertiesAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecRole actual) {
return new com.foursoft.vecmodel.vec120.VecRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRoutableElementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRoutableElementAssert assertThat(com.foursoft.vecmodel.vec120.VecRoutableElement actual) {
return new com.foursoft.vecmodel.vec120.VecRoutableElementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRoutingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRoutingAssert assertThat(com.foursoft.vecmodel.vec120.VecRouting actual) {
return new com.foursoft.vecmodel.vec120.VecRoutingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecRoutingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecRoutingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecRoutingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecRoutingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSIUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSIUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecSIUnit actual) {
return new com.foursoft.vecmodel.vec120.VecSIUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSealedCavitiesAssignmentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSealedCavitiesAssignmentAssert assertThat(com.foursoft.vecmodel.vec120.VecSealedCavitiesAssignment actual) {
return new com.foursoft.vecmodel.vec120.VecSealedCavitiesAssignmentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSegmentConnectionPointAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSegmentConnectionPointAssert assertThat(com.foursoft.vecmodel.vec120.VecSegmentConnectionPoint actual) {
return new com.foursoft.vecmodel.vec120.VecSegmentConnectionPointAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSegmentCrossSectionAreaAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSegmentCrossSectionAreaAssert assertThat(com.foursoft.vecmodel.vec120.VecSegmentCrossSectionArea actual) {
return new com.foursoft.vecmodel.vec120.VecSegmentCrossSectionAreaAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSegmentLengthAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSegmentLengthAssert assertThat(com.foursoft.vecmodel.vec120.VecSegmentLength actual) {
return new com.foursoft.vecmodel.vec120.VecSegmentLengthAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSegmentLocationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSegmentLocationAssert assertThat(com.foursoft.vecmodel.vec120.VecSegmentLocation actual) {
return new com.foursoft.vecmodel.vec120.VecSegmentLocationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSegmentMappingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSegmentMappingAssert assertThat(com.foursoft.vecmodel.vec120.VecSegmentMapping actual) {
return new com.foursoft.vecmodel.vec120.VecSegmentMappingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSheetOrChapterAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSheetOrChapterAssert assertThat(com.foursoft.vecmodel.vec120.VecSheetOrChapter actual) {
return new com.foursoft.vecmodel.vec120.VecSheetOrChapterAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecShieldSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecShieldSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecShieldSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecShieldSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecShrinkableTubeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecShrinkableTubeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecShrinkableTubeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecShrinkableTubeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSiPrefixAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSiPrefixAssert assertThat(com.foursoft.vecmodel.vec120.VecSiPrefix actual) {
return new com.foursoft.vecmodel.vec120.VecSiPrefixAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSiUnitNameAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSiUnitNameAssert assertThat(com.foursoft.vecmodel.vec120.VecSiUnitName actual) {
return new com.foursoft.vecmodel.vec120.VecSiUnitNameAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSignalAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSignalAssert assertThat(com.foursoft.vecmodel.vec120.VecSignal actual) {
return new com.foursoft.vecmodel.vec120.VecSignalAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSignalDirectionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSignalDirectionAssert assertThat(com.foursoft.vecmodel.vec120.VecSignalDirection actual) {
return new com.foursoft.vecmodel.vec120.VecSignalDirectionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSignalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSignalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecSignalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecSignalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSimpleValuePropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSimpleValuePropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecSimpleValueProperty actual) {
return new com.foursoft.vecmodel.vec120.VecSimpleValuePropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSizeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSizeAssert assertThat(com.foursoft.vecmodel.vec120.VecSize actual) {
return new com.foursoft.vecmodel.vec120.VecSizeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSlotAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSlotAssert assertThat(com.foursoft.vecmodel.vec120.VecSlot actual) {
return new com.foursoft.vecmodel.vec120.VecSlotAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSlotCouplingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSlotCouplingAssert assertThat(com.foursoft.vecmodel.vec120.VecSlotCoupling actual) {
return new com.foursoft.vecmodel.vec120.VecSlotCouplingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSlotLayoutAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSlotLayoutAssert assertThat(com.foursoft.vecmodel.vec120.VecSlotLayout actual) {
return new com.foursoft.vecmodel.vec120.VecSlotLayoutAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSlotMappingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSlotMappingAssert assertThat(com.foursoft.vecmodel.vec120.VecSlotMapping actual) {
return new com.foursoft.vecmodel.vec120.VecSlotMappingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSlotReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSlotReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecSlotReference actual) {
return new com.foursoft.vecmodel.vec120.VecSlotReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSlotSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSlotSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecSlotSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecSlotSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSoundDampingClassAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSoundDampingClassAssert assertThat(com.foursoft.vecmodel.vec120.VecSoundDampingClass actual) {
return new com.foursoft.vecmodel.vec120.VecSoundDampingClassAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSpecificRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSpecificRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecSpecificRole actual) {
return new com.foursoft.vecmodel.vec120.VecSpecificRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSpliceTerminalRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSpliceTerminalRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecSpliceTerminalRole actual) {
return new com.foursoft.vecmodel.vec120.VecSpliceTerminalRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSpliceTerminalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSpliceTerminalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecSpliceTerminalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecSpliceTerminalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecStripeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecStripeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecStripeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecStripeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecSwitchingStateAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecSwitchingStateAssert assertThat(com.foursoft.vecmodel.vec120.VecSwitchingState actual) {
return new com.foursoft.vecmodel.vec120.VecSwitchingStateAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTapeRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTapeRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecTapeRole actual) {
return new com.foursoft.vecmodel.vec120.VecTapeRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTapeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTapeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTapeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTapeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTapingDirectionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTapingDirectionAssert assertThat(com.foursoft.vecmodel.vec120.VecTapingDirection actual) {
return new com.foursoft.vecmodel.vec120.VecTapingDirectionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTemperatureInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTemperatureInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecTemperatureInformation actual) {
return new com.foursoft.vecmodel.vec120.VecTemperatureInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalCurrentInformationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalCurrentInformationAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalCurrentInformation actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalCurrentInformationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalPairingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalPairingAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalPairing actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalPairingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalPairingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalPairingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalPairingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalPairingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalReceptionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalReceptionAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalReception actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalReceptionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalReceptionReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalReceptionReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalReceptionReference actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalReceptionReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalReceptionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalReceptionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalReceptionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalReceptionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalRole actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTerminalTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTerminalTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecTerminalType actual) {
return new com.foursoft.vecmodel.vec120.VecTerminalTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTextBasedInstructionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTextBasedInstructionAssert assertThat(com.foursoft.vecmodel.vec120.VecTextBasedInstruction actual) {
return new com.foursoft.vecmodel.vec120.VecTextBasedInstructionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecToleranceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecToleranceAssert assertThat(com.foursoft.vecmodel.vec120.VecTolerance actual) {
return new com.foursoft.vecmodel.vec120.VecToleranceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologyBendingRestrictionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologyBendingRestrictionAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologyBendingRestriction actual) {
return new com.foursoft.vecmodel.vec120.VecTopologyBendingRestrictionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologyBendingRestrictionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologyBendingRestrictionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologyBendingRestrictionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTopologyBendingRestrictionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologyGroupSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologyGroupSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologyGroupSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTopologyGroupSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologyMappingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologyMappingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologyMappingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTopologyMappingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologyNodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologyNodeAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologyNode actual) {
return new com.foursoft.vecmodel.vec120.VecTopologyNodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologySegmentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologySegmentAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologySegment actual) {
return new com.foursoft.vecmodel.vec120.VecTopologySegmentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTopologySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologyZoneAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologyZoneAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologyZone actual) {
return new com.foursoft.vecmodel.vec120.VecTopologyZoneAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTopologyZoneSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTopologyZoneSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTopologyZoneSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTopologyZoneSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTransformation2DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTransformation2DAssert assertThat(com.foursoft.vecmodel.vec120.VecTransformation2D actual) {
return new com.foursoft.vecmodel.vec120.VecTransformation2DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTransformation3DAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTransformation3DAssert assertThat(com.foursoft.vecmodel.vec120.VecTransformation3D actual) {
return new com.foursoft.vecmodel.vec120.VecTransformation3DAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecTubeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecTubeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecTubeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecTubeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUSUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUSUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecUSUnit actual) {
return new com.foursoft.vecmodel.vec120.VecUSUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUSUnitNameAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUSUnitNameAssert assertThat(com.foursoft.vecmodel.vec120.VecUSUnitName actual) {
return new com.foursoft.vecmodel.vec120.VecUSUnitNameAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecUnit actual) {
return new com.foursoft.vecmodel.vec120.VecUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUsageConstraintAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUsageConstraintAssert assertThat(com.foursoft.vecmodel.vec120.VecUsageConstraint actual) {
return new com.foursoft.vecmodel.vec120.VecUsageConstraintAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUsageConstraintSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUsageConstraintSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecUsageConstraintSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecUsageConstraintSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUsageConstraintTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUsageConstraintTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecUsageConstraintType actual) {
return new com.foursoft.vecmodel.vec120.VecUsageConstraintTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUsageNodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUsageNodeAssert assertThat(com.foursoft.vecmodel.vec120.VecUsageNode actual) {
return new com.foursoft.vecmodel.vec120.VecUsageNodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecUsageNodeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecUsageNodeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecUsageNodeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecUsageNodeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecValueDeterminationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecValueDeterminationAssert assertThat(com.foursoft.vecmodel.vec120.VecValueDetermination actual) {
return new com.foursoft.vecmodel.vec120.VecValueDeterminationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecValueRangeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecValueRangeAssert assertThat(com.foursoft.vecmodel.vec120.VecValueRange actual) {
return new com.foursoft.vecmodel.vec120.VecValueRangeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecValueRangePropertyAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecValueRangePropertyAssert assertThat(com.foursoft.vecmodel.vec120.VecValueRangeProperty actual) {
return new com.foursoft.vecmodel.vec120.VecValueRangePropertyAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecValueWithUnitAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecValueWithUnitAssert assertThat(com.foursoft.vecmodel.vec120.VecValueWithUnit actual) {
return new com.foursoft.vecmodel.vec120.VecValueWithUnitAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantCodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantCodeAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantCode actual) {
return new com.foursoft.vecmodel.vec120.VecVariantCodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantCodeSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantCodeSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantCodeSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecVariantCodeSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantConfigurationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantConfigurationAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantConfiguration actual) {
return new com.foursoft.vecmodel.vec120.VecVariantConfigurationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantConfigurationSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantConfigurationSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantConfigurationSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecVariantConfigurationSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantGroupAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantGroupAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantGroup actual) {
return new com.foursoft.vecmodel.vec120.VecVariantGroupAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantGroupSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantGroupSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantGroupSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecVariantGroupSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantStructureNodeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantStructureNodeAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantStructureNode actual) {
return new com.foursoft.vecmodel.vec120.VecVariantStructureNodeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecVariantStructureSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecVariantStructureSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecVariantStructureSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecVariantStructureSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireElementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireElementAssert assertThat(com.foursoft.vecmodel.vec120.VecWireElement actual) {
return new com.foursoft.vecmodel.vec120.VecWireElementAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireElementReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireElementReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecWireElementReference actual) {
return new com.foursoft.vecmodel.vec120.VecWireElementReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireElementSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireElementSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecWireElementSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecWireElementSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireEndAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireEndAssert assertThat(com.foursoft.vecmodel.vec120.VecWireEnd actual) {
return new com.foursoft.vecmodel.vec120.VecWireEndAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireEndAccessoryRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireEndAccessoryRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecWireEndAccessoryRole actual) {
return new com.foursoft.vecmodel.vec120.VecWireEndAccessoryRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireEndAccessorySpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireEndAccessorySpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecWireEndAccessorySpecification actual) {
return new com.foursoft.vecmodel.vec120.VecWireEndAccessorySpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireGroupSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireGroupSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecWireGroupSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecWireGroupSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireGroupingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireGroupingAssert assertThat(com.foursoft.vecmodel.vec120.VecWireGrouping actual) {
return new com.foursoft.vecmodel.vec120.VecWireGroupingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireGroupingSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireGroupingSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecWireGroupingSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecWireGroupingSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireLengthAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireLengthAssert assertThat(com.foursoft.vecmodel.vec120.VecWireLength actual) {
return new com.foursoft.vecmodel.vec120.VecWireLengthAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireMountingAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireMountingAssert assertThat(com.foursoft.vecmodel.vec120.VecWireMounting actual) {
return new com.foursoft.vecmodel.vec120.VecWireMountingAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireMountingDetailAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireMountingDetailAssert assertThat(com.foursoft.vecmodel.vec120.VecWireMountingDetail actual) {
return new com.foursoft.vecmodel.vec120.VecWireMountingDetailAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireProtectionRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireProtectionRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecWireProtectionRole actual) {
return new com.foursoft.vecmodel.vec120.VecWireProtectionRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireProtectionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireProtectionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecWireProtectionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecWireProtectionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireReceptionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireReceptionAssert assertThat(com.foursoft.vecmodel.vec120.VecWireReception actual) {
return new com.foursoft.vecmodel.vec120.VecWireReceptionAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireReceptionAddOnAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireReceptionAddOnAssert assertThat(com.foursoft.vecmodel.vec120.VecWireReceptionAddOn actual) {
return new com.foursoft.vecmodel.vec120.VecWireReceptionAddOnAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireReceptionReferenceAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireReceptionReferenceAssert assertThat(com.foursoft.vecmodel.vec120.VecWireReceptionReference actual) {
return new com.foursoft.vecmodel.vec120.VecWireReceptionReferenceAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireReceptionSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireReceptionSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecWireReceptionSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecWireReceptionSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireRoleAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireRoleAssert assertThat(com.foursoft.vecmodel.vec120.VecWireRole actual) {
return new com.foursoft.vecmodel.vec120.VecWireRoleAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireSpecificationAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireSpecificationAssert assertThat(com.foursoft.vecmodel.vec120.VecWireSpecification actual) {
return new com.foursoft.vecmodel.vec120.VecWireSpecificationAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWireTypeAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWireTypeAssert assertThat(com.foursoft.vecmodel.vec120.VecWireType actual) {
return new com.foursoft.vecmodel.vec120.VecWireTypeAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecWriterAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecWriterAssert assertThat(com.foursoft.vecmodel.vec120.VecWriter actual) {
return new com.foursoft.vecmodel.vec120.VecWriterAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecZoneAssignmentAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecZoneAssignmentAssert assertThat(com.foursoft.vecmodel.vec120.VecZoneAssignment actual) {
return new com.foursoft.vecmodel.vec120.VecZoneAssignmentAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VecZoneCoverageAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VecZoneCoverageAssert assertThat(com.foursoft.vecmodel.vec120.VecZoneCoverage actual) {
return new com.foursoft.vecmodel.vec120.VecZoneCoverageAssert(actual);
}
/**
* Creates a new instance of {@link com.foursoft.vecmodel.vec120.VersionAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
@org.assertj.core.util.CheckReturnValue
public static com.foursoft.vecmodel.vec120.VersionAssert assertThat(com.foursoft.vecmodel.vec120.Version actual) {
return new com.foursoft.vecmodel.vec120.VersionAssert(actual);
}
/**
* Creates a new {@link Assertions}
.
*/
protected Assertions() {
// empty
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy