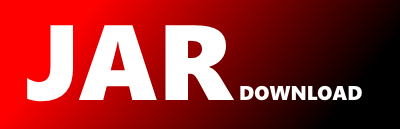
com.franz.agraph.pool.AGConnPoolJndiFactory Maven / Gradle / Ivy
Show all versions of agraph-java-client Show documentation
/******************************************************************************
** Copyright (c) 2008-2016 Franz Inc.
** All rights reserved. This program and the accompanying materials
** are made available under the terms of the Eclipse Public License v1.0
** which accompanies this distribution, and is available at
** http://www.eclipse.org/legal/epl-v10.html
******************************************************************************/
package com.franz.agraph.pool;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
import javax.naming.Context;
import javax.naming.Name;
import javax.naming.RefAddr;
import javax.naming.Reference;
import javax.naming.spi.ObjectFactory;
import javax.servlet.ServletContextListener;
import org.apache.commons.pool.impl.GenericObjectPool;
import com.franz.agraph.repository.AGRepository;
import com.franz.agraph.repository.AGRepositoryConnection;
import com.franz.agraph.repository.AGServer;
import com.franz.util.Closeable;
/**
* JNDI factory for {@link AGConnPool}.
*
* The Commons-Pool library is required to use this package:
* Apache Commons Pool, commons-pool-1.5.6.jar.
* Note, this jar along with the agraph-java-client jar and all of its dependencies
* must be in the webserver's library for it to be able to load.
*
*
* The properties supported for the connections are specified
* by {@link AGConnProp}.
*
*
* The properties supported for the pooling are specified
* by {@link AGPoolProp}.
*
*
* Note, when {@link Closeable#close()} is called
* on an {@link AGConnPool},
* connections will be closed whether they are
* idle (have been returned) or not.
* This is different from {@link GenericObjectPool#close()}.
* Also note, when a {@link AGRepositoryConnection} from the pool is closed,
* the {@link AGRepository} and {@link AGServer} will also be closed
* since these are not shared with other {@link AGRepositoryConnection}s.
*
*
*
* Example Tomcat JNDI configuration, based on
* Tomcat HOW-TO create custom resource factories:
* In /WEB-INF/web.xml:
*
{@code
*
* AllegroGraph connection pool
* connection-pool/agraph
* com.franz.agraph.pool.AGConnPool
*
* }
* Your code:
* {@code
* Context initCtx = new InitialContext();
* Context envCtx = (Context) initCtx.lookup("java:comp/env");
* AGConnPool pool = (AGConnPool) envCtx.lookup("connection-pool/agraph");
* AGRepositoryConnection conn = pool.borrowConnection();
* try {
* ...
* conn.commit();
* } finally {
* conn.close();
* // or equivalently
* pool.returnObject(conn);
* }
* }
* Tomcat's resource factory:
* {@code
*
* ...
*
* ...
*
* }
*
* Closing the connection pool is important because server sessions will
* stay active until {@link AGConnProp#sessionLifetime}.
* The option to use a Runtime shutdownHook is built-in with {@link AGPoolProp#shutdownHook}.
* Another option is to use {@link ServletContextListener} - this is appropriate if the
* agraph jar is deployed within your webapp and not with the webserver.
* With tomcat, a Lifecycle Listener can be configured, but the implementation to do this
* is not included in this library.
*
*
* @since v4.3.3
*/
public class AGConnPoolJndiFactory implements ObjectFactory {
/**
* From the obj {@link Reference}, gets the {@link RefAddr}
* names and values, converts to Maps and
* returns {@link AGConnPool#create(Object...)}.
*/
@Override
public Object getObjectInstance(Object obj,
Name name,
Context nameCtx,
Hashtable,?> environment)
throws Exception {
if (!(obj instanceof Reference)) {
return null;
}
Reference ref = (Reference) obj;
if (! AGConnPool.class.getName().equals(ref.getClassName())) {
return null;
}
Map connProps = (Map) refToMap(ref, AGConnProp.values());
Map poolProps = (Map) refToMap(ref, AGPoolProp.values());
return AGConnPool.create(connProps, poolProps);
}
/**
* @param values enum values
* @return map suitable for {@link AGConnPool#create(Map, Map)}
*/
private static Map extends Enum, String> refToMap(Reference ref, Enum[] values) {
Map props = new HashMap();
for (Enum prop : values) {
RefAddr ra = ref.get(prop.name());
if (ra == null) {
ra = ref.get(prop.name().toLowerCase());
}
if (ra != null) {
String propertyValue = ra.getContent().toString();
props.put(prop, propertyValue);
}
}
return props;
}
}