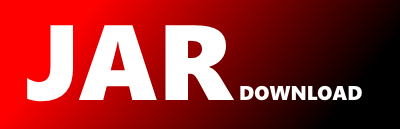
com.franz.agraph.repository.AGFreetextIndexConfig Maven / Gradle / Ivy
/******************************************************************************
** Copyright (c) 2008-2016 Franz Inc.
** All rights reserved. This program and the accompanying materials
** are made available under the terms of the Eclipse Public License v1.0
** which accompanies this distribution, and is available at
** http://www.eclipse.org/legal/epl-v10.html
******************************************************************************/
package com.franz.agraph.repository;
import java.util.ArrayList;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.openrdf.model.URI;
import org.openrdf.model.ValueFactory;
import org.openrdf.model.impl.ValueFactoryImpl;
import org.openrdf.rio.ntriples.NTriplesUtil;
/**
* The class of freetext index configurations.
*
* An index configuration can be customized and then used to create a new freetext index.
*
* See documentation for
* freetext index parameters.
*
* @see #newInstance()
* @see AGRepositoryConnection#createFreetextIndex(String, AGFreetextIndexConfig)
*/
public class AGFreetextIndexConfig {
public static final String PREDICATES = "predicates";
public static final String INDEX_LITERALS = "indexLiterals";
public static final String INDEX_LITERAL_TYPES = "indexLiteralTypes";
public static final String INDEX_RESOURCES = "indexResources";
public static final String INDEX_FIELDS = "indexFields";
public static final String MINIMUM_WORD_SIZE = "minimumWordSize";
public static final String STOP_WORDS = "stopWords";
public static final String WORD_FILTERS = "wordFilters";
public static final String INNER_CHARS = "innerChars";
public static final String BORDER_CHARS = "borderChars";
public static final String TOKENIZER = "tokenizer";
private static final ValueFactory vf = new ValueFactoryImpl();
private List predicates;
private boolean indexLiterals;
private List indexLiteralTypes;
private String indexResources;
private List indexFields;
private int minimumWordSize;
private List stopWords;
private List wordFilters;
private List innerChars;
private List borderChars;
private String tokenizer;
/**
* Returns a new instance having the default index configuration.
*
* The index configuration can be customized and then used
* to create a new freetext index.
*
* See documentation for
* freetext index parameters.
*
* @see AGRepositoryConnection#createFreetextIndex(String, AGFreetextIndexConfig)
*/
public static AGFreetextIndexConfig newInstance() {
return new AGFreetextIndexConfig();
}
private AGFreetextIndexConfig() {
predicates = new ArrayList();
indexLiterals = true;
indexLiteralTypes = new ArrayList();
indexResources = "false";
indexFields = new ArrayList();
indexFields.add("object");
minimumWordSize = 3;
stopWords = new ArrayList();
wordFilters = new ArrayList();
innerChars = new ArrayList();
borderChars = new ArrayList();
tokenizer = "default";
}
AGFreetextIndexConfig(JSONObject config) {
predicates = initPredicates(config);
indexLiterals = initIndexLiterals(config);
indexLiteralTypes = getJSONArrayAsListString(config, INDEX_LITERAL_TYPES);
indexResources = config.optString(INDEX_RESOURCES);
indexFields = getJSONArrayAsListString(config, INDEX_FIELDS);
minimumWordSize = config.optInt(MINIMUM_WORD_SIZE);
stopWords = getJSONArrayAsListString(config, STOP_WORDS);
wordFilters = getJSONArrayAsListString(config, WORD_FILTERS);
innerChars = getJSONArrayAsListString(config, INNER_CHARS);
borderChars = getJSONArrayAsListString(config, BORDER_CHARS);
tokenizer = config.optString(TOKENIZER);
}
private boolean initIndexLiterals(JSONObject config) {
boolean bool;
try {
// Can't use optBoolean here, it defaults to false
bool = config.getBoolean(INDEX_LITERALS);
} catch (JSONException e) {
// TODO: perhaps log this if it happens.
bool = true;
}
return bool;
}
private List initPredicates(JSONObject config) {
List predList = new ArrayList();
JSONArray preds = config.optJSONArray(PREDICATES);
if (preds!=null) {
for (int i = 0; i < preds.length(); i++) {
String uri_nt = preds.optString(i);
URI uri = NTriplesUtil.parseURI(uri_nt, vf);
predList.add(uri);
}
}
return predList;
}
/**
* See documentation for
* freetext index parameters.
*/
public List getPredicates() {
return predicates;
}
/**
* See documentation for
* freetext index parameters.
*/
public boolean getIndexLiterals() {
return indexLiterals;
}
/**
* See documentation for
* freetext index parameters.
*/
public void setIndexLiterals(boolean bool) {
indexLiterals = bool;
}
/**
* See documentation for
* freetext index parameters.
*/
public List getIndexLiteralTypes() {
return indexLiteralTypes;
}
/**
* See documentation for
* freetext index parameters.
*/
public String getIndexResources() {
return indexResources;
}
/**
* See documentation for
* freetext index parameters.
*/
public void setIndexResources(String str) {
//TODO: validity check
indexResources = str;
}
/**
* See documentation for
* freetext index parameters.
*/
public List getIndexFields() {
return indexFields;
}
/**
* See documentation for
* freetext index parameters.
*/
public int getMinimumWordSize() {
return minimumWordSize;
}
/**
* See documentation for
* freetext index parameters.
*/
public void setMinimumWordSize(int size) {
minimumWordSize = size;
}
/**
* See documentation for
* freetext index parameters.
*/
public List getStopWords() {
return stopWords;
}
/**
* See documentation for
* freetext index parameters.
*/
public List getWordFilters() {
return wordFilters;
}
public List getInnerChars() {
return innerChars;
}
/**
* See documentation for
* freetext index parameters.
*/
public List getBorderChars() {
return borderChars;
}
/**
* See documentation for
* freetext index parameters.
*/
public String getTokenizer() {
return tokenizer;
}
/**
* See documentation for
* freetext index parameters.
*/
public void setTokenizer(String tokenizerName) {
tokenizer = tokenizerName;
}
private List getJSONArrayAsListString(JSONObject config, String key) {
List list = new ArrayList();
JSONArray array = config.optJSONArray(key);
if (array!=null) {
for (int i = 0; i < array.length(); i++) {
list.add(array.optString(i));
}
}
return list;
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("");
return sb.toString();
}
}