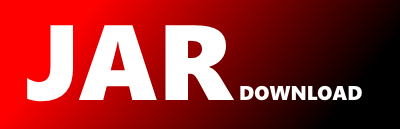
com.fredericboisguerin.excel.reader.ExcelReaderImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of excel-reader-writer Show documentation
Show all versions of excel-reader-writer Show documentation
A library to read/write excel files with simple descriptions of the model
package com.fredericboisguerin.excel.reader;
import com.fredericboisguerin.excel.common.ExcelRow;
import com.fredericboisguerin.excel.common.Information;
import com.fredericboisguerin.excel.common.InformationValue;
import com.fredericboisguerin.excel.converter.ConverterException;
import com.fredericboisguerin.excel.converter.ConverterHelper;
import com.fredericboisguerin.excel.description.SheetDescription;
import com.fredericboisguerin.excel.description.WorkbookDescription;
import org.jxls.reader.*;
import java.io.InputStream;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.*;
import java.util.stream.Collectors;
/**
* Created by fboisguerin on 23/07/2015.
*/
public class ExcelReaderImpl implements ExcelReader {
private final WorkbookDescription wbDescription;
public static final String ITEMS_VARIABLE_NAME = "instruments";
public static final String BEAN_KEY = "instrument";
public ExcelReaderImpl(WorkbookDescription wbDescription) {
this.wbDescription = wbDescription;
}
private static final BeanCellMapping getBeanCellMapping(int colIdx, int rowIdx) {
// fredericboisguerin : I know this is not a very smart solution...
if (colIdx > BufferItemConstants.NB_FIELD - 1) {
throw new IllegalArgumentException(
String.format("The col index %d is too high (max=%d)", colIdx, BufferItemConstants.NB_FIELD - 1));
}
return new BeanCellMapping(rowIdx, (short) colIdx, BEAN_KEY, BufferItemConstants.FIELD_PREFIX + colIdx);
}
private static final List getBeanCellMappings(SheetDescription sheetDescription,
int rowIdx) {
List cellMappings = new ArrayList<>();
cellMappings.add(getBeanCellMapping(sheetDescription.getBreaklLoopOnEmptyColumnIdx(), rowIdx));
// Informations
Set columnIdx = sheetDescription.getColumnIndexInfoMap().keySet();
cellMappings.addAll(
columnIdx.stream().map(col -> getBeanCellMapping(col, rowIdx)).collect(Collectors.toList()));
return cellMappings;
}
private static XLSSheetReader getXlsSheetReader(SheetDescription sheetDescription,
String itemsVariableName) {
XLSSheetReader sheetReader = new XLSSheetReaderImpl();
// First lines block reader
XLSBlockReader firstLineBlockReader = new SimpleBlockReaderImpl(0,
sheetDescription.getStartRowPayloadIdx() - 1);
sheetReader.addBlockReader(firstLineBlockReader);
// For each item
XLSForEachBlockReaderImpl instrumentBlockReader = new XLSForEachBlockReaderImpl(
sheetDescription.getStartRowPayloadIdx(),
sheetDescription.getStartRowPayloadIdx(), itemsVariableName, BEAN_KEY, BufferItem.class);
List beanCellMappings = getBeanCellMappings(sheetDescription,
sheetDescription.getStartRowPayloadIdx());
SimpleBlockReader rowBlockReader = new SimpleBlockReaderImpl(
sheetDescription.getStartRowPayloadIdx(),
sheetDescription.getStartRowPayloadIdx(), beanCellMappings);
instrumentBlockReader.addBlockReader(rowBlockReader);
// Loop break condition
OffsetCellCheck cellCheck = new OffsetCellCheckImpl(
sheetDescription.getBreaklLoopOnEmptyColumnIdx(), "");
OffsetRowCheck rowCheck = new OffsetRowCheckImpl(0);
rowCheck.addCellCheck(cellCheck);
SectionCheck sectionCheck = new SimpleSectionCheck(Arrays.asList(rowCheck));
instrumentBlockReader.setLoopBreakCondition(sectionCheck);
sheetReader.addBlockReader(instrumentBlockReader);
return sheetReader;
}
private static XLSReader getWorkbookReader(
WorkbookDescription description,
Map> beans,
Map jxlsBeans) {
XLSReader mainReader = new XLSReaderImpl();
description.getSheetIndexSheetInfoMap().forEach(
(sheetIdx, sheetInfoDescription) -> {
final String itemsVariableName = String.format("%s%d", ITEMS_VARIABLE_NAME, sheetIdx);
List itemList = new ArrayList<>();
beans.put(sheetIdx, itemList);
jxlsBeans.put(itemsVariableName, itemList);
mainReader.addSheetReader(sheetIdx, getXlsSheetReader(sheetInfoDescription, itemsVariableName));
}
);
return mainReader;
}
private static Method getBufferItemMethod(int columnIdx) {
try {
return BufferItem.class.getMethod(String.format("get%s%s%d",
BufferItemConstants.FIELD_PREFIX.substring(0, 1).toUpperCase(),
BufferItemConstants.FIELD_PREFIX.substring(1),
columnIdx));
} catch (NoSuchMethodException e) {
e.printStackTrace();
}
System.exit(1);
return null;
}
private static Map getInformationValues(BufferItem bufferItem,
SheetDescription description) {
final Map informationValues = new HashMap<>();
description.getColumnIndexInfoMap().forEach((columnIdx, information) -> {
try {
String strValue = (String) getBufferItemMethod(columnIdx).invoke(bufferItem);
Object value = ConverterHelper.getConverter(information.getType()).convert(strValue);
informationValues.put(information, new InformationValue(value));
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
} catch (ConverterException e) {
e.printStackTrace();
}
});
return informationValues;
}
private static List convertSheetToInstrumentList(
List mBeans,
SheetDescription sheetDescription) {
final List excelRowList = new ArrayList<>();
mBeans.forEach(bufferItem -> {
ExcelRow excelRow = new ExcelRow();
excelRow.getInformationValuesMap().putAll(getInformationValues(bufferItem, sheetDescription));
excelRowList.add(excelRow);
});
return excelRowList;
}
private static Map> convertWbToInstrumentList(Map> mBeans,
WorkbookDescription wbDescription) {
final Map> excelRowList = new HashMap<>();
mBeans.forEach(
(sheetIdx, items) -> excelRowList.put(sheetIdx,
convertSheetToInstrumentList(items, wbDescription.getSheetIndexSheetInfoMap().get(sheetIdx)))
);
return excelRowList;
}
@Override
public Map> readWorkbook(InputStream inputStream)
throws ExcelReaderException {
Map> mBeans = new HashMap<>();
Map jxlsBeans = new HashMap();
XLSReader xlsReader = getWorkbookReader(wbDescription, mBeans, jxlsBeans);
try {
xlsReader.read(inputStream, jxlsBeans);
return convertWbToInstrumentList(mBeans, wbDescription);
} catch (Exception e) {
throw new ExcelReaderException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy