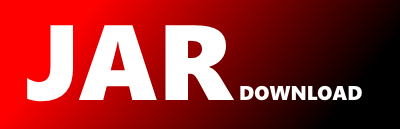
com.fredericboisguerin.excel.writer.ExcelWriterImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of excel-reader-writer Show documentation
Show all versions of excel-reader-writer Show documentation
A library to read/write excel files with simple descriptions of the model
package com.fredericboisguerin.excel.writer;
import com.fredericboisguerin.excel.common.ExcelRow;
import com.fredericboisguerin.excel.common.Information;
import com.fredericboisguerin.excel.description.WorkbookDescription;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by fboisguerin on 11/09/2015.
*/
public class ExcelWriterImpl implements ExcelWriter {
private final WorkbookDescription description;
public ExcelWriterImpl(WorkbookDescription description) {
this.description = description;
}
public InputStream getStream(Map> bookRowsMap)
throws ExcelWriterException {
try {
// Create workbook
final SXSSFWorkbook wb = new SXSSFWorkbook();
wb.setCompressTempFiles(true);
// Reference column indexes in organized maps
// tagSheetMap :
final Map sheetMap = new HashMap<>();
// sheetInfoColIndexMap : >
final Map> sheetInfoColIndexMap = new HashMap<>();
description.getSheetIndexSheetInfoMap().forEach((sheetIdx, sheetDescription) -> {
sheetMap.put(sheetIdx, wb.createSheet(sheetDescription.getName()));
sheetInfoColIndexMap.put(sheetIdx, new HashMap<>());
});
// Create headers cells
// headerRowMap :
final Map headerRowMap = new HashMap<>();
sheetMap.forEach((sheetIdx, sheet) -> {
Font boldFont = wb.createFont();
boldFont.setBold(true);
Row headerRow = sheet.createRow(0);
CellStyle boldStyle = wb.createCellStyle();
boldStyle.setFont(boldFont);
headerRow.setRowStyle(boldStyle);
headerRowMap.put(sheetIdx, headerRow);
});
description.getSheetIndexSheetInfoMap().forEach(
(sheetIdx, sheetDescription) -> sheetDescription.getColumnIndexInfoMap().forEach(
(columnIndex, information) -> {
final Cell header = headerRowMap.get(sheetIdx).createCell(columnIndex);
header.getCellStyle().setAlignment(CellStyle.ALIGN_CENTER);
header.getCellStyle().setVerticalAlignment(CellStyle.VERTICAL_CENTER);
header.setCellValue(information.getLabel());
sheetInfoColIndexMap.get(sheetIdx).put(information, columnIndex);
}));
// Create data cells
final Map tagIndexMap = new HashMap<>();
sheetMap.keySet().forEach(sheetIdx -> tagIndexMap.put(sheetIdx, 1));
bookRowsMap.forEach((sheetIdx, rowList) -> {
final Sheet sheet = sheetMap.get(sheetIdx);
for (ExcelRow excelRow : rowList) {
final int indexNewRow = tagIndexMap.get(sheetIdx);
final Row row = sheet.createRow(indexNewRow);
tagIndexMap.put(sheetIdx, indexNewRow + 1);
// Informations
excelRow.getInformationValuesMap().forEach((information, informationValue) -> {
Integer colIndex = sheetInfoColIndexMap.get(sheetIdx).get(information);
if (colIndex != null) {
final Cell cell = row.createCell(colIndex);
if (informationValue != null) {
switch (information.getType()) {
case STRING:
cell.setCellType(Cell.CELL_TYPE_STRING);
cell.setCellValue((String) informationValue);
break;
case DATE:
cell.setCellValue((Date) informationValue);
setCellStyleDate(wb, cell);
break;
case BOOLEAN:
cell.setCellType(Cell.CELL_TYPE_BOOLEAN);
cell.setCellValue((Boolean) informationValue);
break;
case LONG:
cell.setCellType(Cell.CELL_TYPE_NUMERIC);
cell.setCellValue((Long) informationValue);
break;
case DOUBLE:
cell.setCellType(Cell.CELL_TYPE_NUMERIC);
cell.setCellValue((Double) informationValue);
break;
default:
cell.setCellValue(informationValue.toString());
}
}
}
});
}
});
// Autosize columns
sheetInfoColIndexMap.forEach((tag, informationColIndexMap) ->
informationColIndexMap.forEach((information, integer) ->
sheetMap.get(tag).autoSizeColumn(integer)));
// Export POI
final ByteArrayOutputStream bos = new ByteArrayOutputStream();
wb.write(bos);
bos.close();
wb.dispose();
return new ByteArrayInputStream(bos.toByteArray());
} catch (Exception e) {
e.printStackTrace();
throw new ExcelWriterException(e);
}
}
private static void setCellStyleDate(Workbook wb, Cell cell) {
CellStyle cellStyle = wb.createCellStyle();
CreationHelper createHelper = wb.getCreationHelper();
cellStyle.setDataFormat(createHelper.createDataFormat().getFormat("dd/mm/yyyy"));
cell.setCellStyle(cellStyle);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy