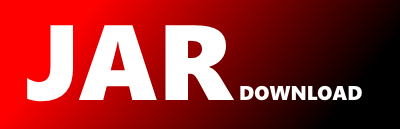
com.fredericboisguerin.excel.converter.DateConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of excel-reader-writer Show documentation
Show all versions of excel-reader-writer Show documentation
A library to read/write excel files with simple descriptions of the model
The newest version!
package com.fredericboisguerin.excel.converter;
import com.fredericboisguerin.excel.StringUtils;
import org.apache.poi.ss.usermodel.DateUtil;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Created by fboisguerin on 22/07/2015.
*/
class DateConverter extends AbstractConverter {
private static final DateConverter INSTANCE = new DateConverter();
private static final SimpleDateFormat SIMPLE_DATE_FORMAT = new SimpleDateFormat("dd/MM/yyyy");
private static final String YEAR_PATTERN = "([1-2][0-9]{3})";
private static final String DATE_PATTERN = ".*([0-9]{2}/[0-9]{2}/[0-9]{4}).*";
public static DateConverter getInstance() {
return INSTANCE;
}
private DateConverter() {
super(Date.class);
}
@Override
protected Date innerConvert(String s) {
if (StringUtils.isEmpty(s)) {
return null;
} else if (s.matches(DATE_PATTERN)) {
return convertStringToDate(firstOccurenceOf(s, DATE_PATTERN));
} else if (s.matches(YEAR_PATTERN)) {
return convertStringToDate("01/01/" + firstOccurenceOf(s, YEAR_PATTERN));
} else {
return DateUtil.getJavaDate(Double.parseDouble(s));
}
}
private static Date convertStringToDate(String dateInString) {
try {
return SIMPLE_DATE_FORMAT.parse(dateInString);
} catch (ParseException e) {
return null;
}
}
public static String firstOccurenceOf(String s, String pattern) {
Pattern p = Pattern.compile(pattern);
Matcher m = p.matcher(s);
if (m.matches()) {
return m.group(1);
} else {
throw new IllegalArgumentException("String does not match pattern");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy