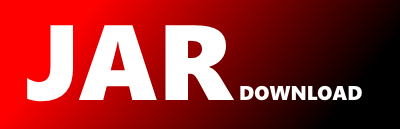
com.freedomotic.bus.BootStatus Maven / Gradle / Ivy
/**
*
* Copyright (c) 2009-2014 Freedomotic team
* http://freedomotic.com
*
* This file is part of Freedomotic
*
* This Program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2, or (at your option)
* any later version.
*
* This Program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Freedomotic; see the file COPYING. If not, see
* .
*/
package com.freedomotic.bus;
import com.freedomotic.app.Freedomotic;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Logger;
/**
* Bus boot statuses enumeration
*
* @author Freedomotic Team
*
*/
public enum BootStatus {
/**
*
*/
STOPPED(0),
/**
*
*/
BOOTING(1),
/**
*
*/
FAILED(2),
/**
*
*/
STARTED(3),
/**
*
*/
STOPPING(4);
private static final Logger LOG = Logger.getLogger(BootStatus.class.getName());
private static final Map lookup = new HashMap();
private static final EnumSet initStatuses = EnumSet.of(STOPPED, BOOTING);
private static final EnumSet destroyStatuses = EnumSet.of(STOPPED, STOPPING);
private static BootStatus currentStatus = STOPPED; // initial status
private static Throwable throwable;
static {
for (BootStatus s : EnumSet.allOf(BootStatus.class)){
lookup.put(s.getCode(), s);
}
}
private int code;
private BootStatus(int code) {
this.code = code;
}
/**
* Getter method for code
* @return the code (as int value)
*/
public int getCode() {
return code;
}
/**
* Translates an int code into his {@link BootStatus} enumeration
* @param code the int value of code
* @return the enumerated object
*/
public static BootStatus get(int code) {
return lookup.get(code);
}
/**
* Setter method for this current status holder
*
* @param status the status to set
*/
public static void setCurrentStatus(BootStatus status) {
currentStatus = status;
}
/**
* Getter method for this current status holder
* @return the current status
*/
public static BootStatus getCurrentStatus() {
return currentStatus;
}
/**
* Getter method for the fault status of this holder
* @return null
or a {@link Throwable}
*/
// TODO Java8 Return an Optional
public static Throwable getThrowable() {
return throwable;
}
/**
* Setter method for the fault status of this holder
* @param t The throwable
*/
public static void setThrowable(Throwable t) {
BootStatus.throwable = t;
BootStatus.currentStatus = BootStatus.FAILED;
// FIXME LCG stop bootstrap
LOG.severe(Freedomotic.getStackTraceInfo(t));
}
private static boolean isAnInitialStatus(BootStatus status) {
return initStatuses.contains(status);
}
/**
* Holder current status is... method.
*
* @return true
if current status is an ... status.
* false
otherwise.
*/
public static boolean isStarting() {
return isAnInitialStatus(currentStatus);
}
private static boolean isAnDestroyStatus(BootStatus status) {
return destroyStatuses.contains(status);
}
/**
* Holder current status is... method.
*
* @return true
if current status is an ... status.
* false
otherwise.
*/
public static boolean isStopping() {
return isAnDestroyStatus(currentStatus);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy